Url launcher using flutter
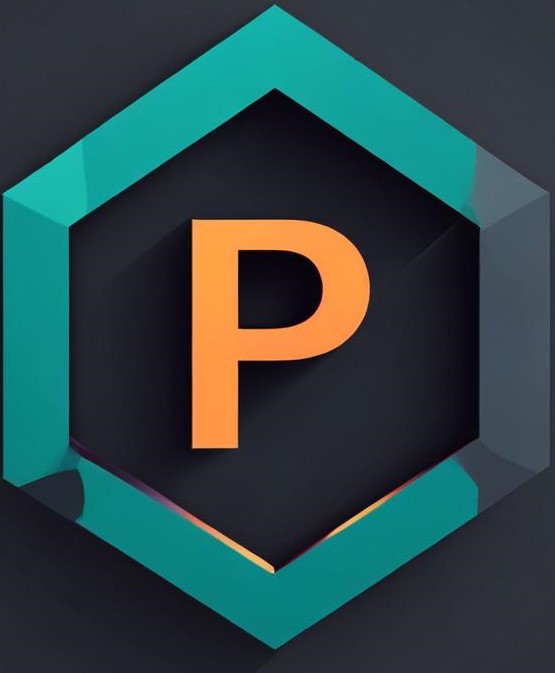
Exploring the URL Launcher Package in Flutter: A Comprehensive Guide
Introduction:
In the world of Flutter app development, integrating external functionalities like opening URLs, making phone calls, sending emails, and more is essential for creating robust and user-friendly applications. This is where the URL Launcher package comes into play. This package provides a simple and efficient way to interact with external resources and services directly from your Flutter app.
What is a URL Launcher?
The URL Launcher package is a Flutter plugin that allows developers to launch various types of URLs and perform actions such as opening web pages, making phone calls, sending emails, and launching map applications. It abstracts the platform-specific code required for these tasks, making it easy to incorporate these functionalities into your Flutter apps regardless of the platform (Android or iOS).
Installation:
To start using the URL Launcher package in your Flutter project, follow these simple steps:
- Add the URL Launcher package dependency under the dependencies section:
→ flutter pub add url_launcher.
- Now add the following permissions in the manifest and info.plist file.
- Manifest file
→ <intent>
<action android:name="android.intent.action.VIEW" />
<data android:scheme="https" />
</intent>
<intent>
<action android:name="android.intent.action.SEND" />
<data android:mimeType="*/*" />
</intent>
<intent>
<action android:name="android.intent.action.PROCESS_TEXT"/>
<data android:mimeType="text/plain"/>
</intent>
<intent>
<action android:name="android.intent.action.VIEW" />
<data android:scheme="sms" />
</intent>
<intent>
<action android:name="android.intent.action.VIEW" />
<data android:scheme="tel" />
</intent>
- Info.plist file
→ <key>LSApplicationQueriesSchemes</key>
<array>
<string>sms</string>
<string>tel</string>
</array>
<key>LSApplicationQueriesSchemes</key>
<array>
<string>mailto</string>
</array>
- Using the following functions you can use the url launcher.
- For call
→ void makePhoneCall() async {
const phoneNumber = 'tel:1234567890';
if (await canLaunch(phoneNumber)) {
await launch(phoneNumber);
} else {
throw 'Could not launch $phoneNumber';
}
}
- For sms
→ void sendSMS() async {
const smsNumber = 'sms:1234567890';
if (await canLaunch(smsNumber)) {
await launch(smsNumber);
} else {
throw 'Could not launch $smsNumber';
}
}
- For email
→ void sendEmail() async {
final Uri emailLaunchUri = Uri(
scheme: 'mailto',
path: 'recipient@example.com', // Enter your recipient email address here
queryParameters: {
'subject': 'Hello', // Enter your email subject here
'body': 'Hello Flutter!' // Enter your email body here
},
);
final url = emailLaunchUri.toString();
if (await canLaunchUrl(emailLaunchUri)) {
await launchUrl(emailLaunchUri);
} else {
throw 'Could not launch $url';
}
}
For detail code please visit the github code
Happy Coding!! 🙂
Subscribe to my newsletter
Read articles from Parth Sheth directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
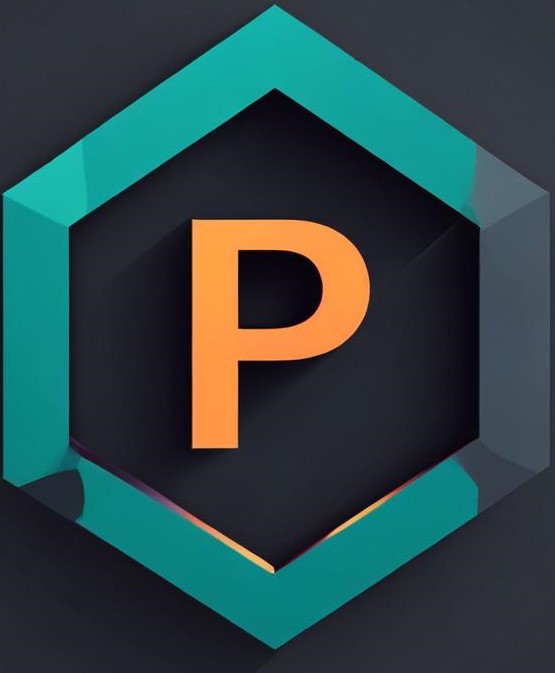
Parth Sheth
Parth Sheth
"🚀 Fluttering through the digital cosmos, I am Parth Sheth, a passionate architect of interactive marvels in the realm of Flutter development. With a fervent love for coding and an insatiable curiosity for the ever-evolving tech landscape, I embark on a perpetual quest to redefine the boundaries of innovation. From sleek UI designs to seamless user experiences, I orchestrate symphonies of code that resonate with elegance and functionality. 💻✨ My journey in Flutter is more than just a profession; it's a thrilling odyssey where creativity meets craftsmanship. Armed with a keen eye for detail and an arsenal of cutting-edge tools, I navigate the complexities of app development with finesse, crafting solutions that not only meet but exceed expectations. 🎨🔧 But beyond the lines of code lies a deeper commitment—to inspire, empower, and elevate. Through my words, my work, and my unwavering dedication to excellence, I strive to ignite the spark of imagination in others, fostering a community where innovation knows no bounds. 🌟💡 So come, fellow adventurers, and join me on this exhilarating expedition into the unknown. Together, let's sculpt the future, one pixel at a time, and unleash the full potential of Flutter's boundless possibilities. Connect with me, and let's embark on this extraordinary voyage—together, we shall chart new horizons and redefine what's possible in the digital landscape. 🌍🔍"