Step-by-Step Guide to Swap Two Numbers in Java :

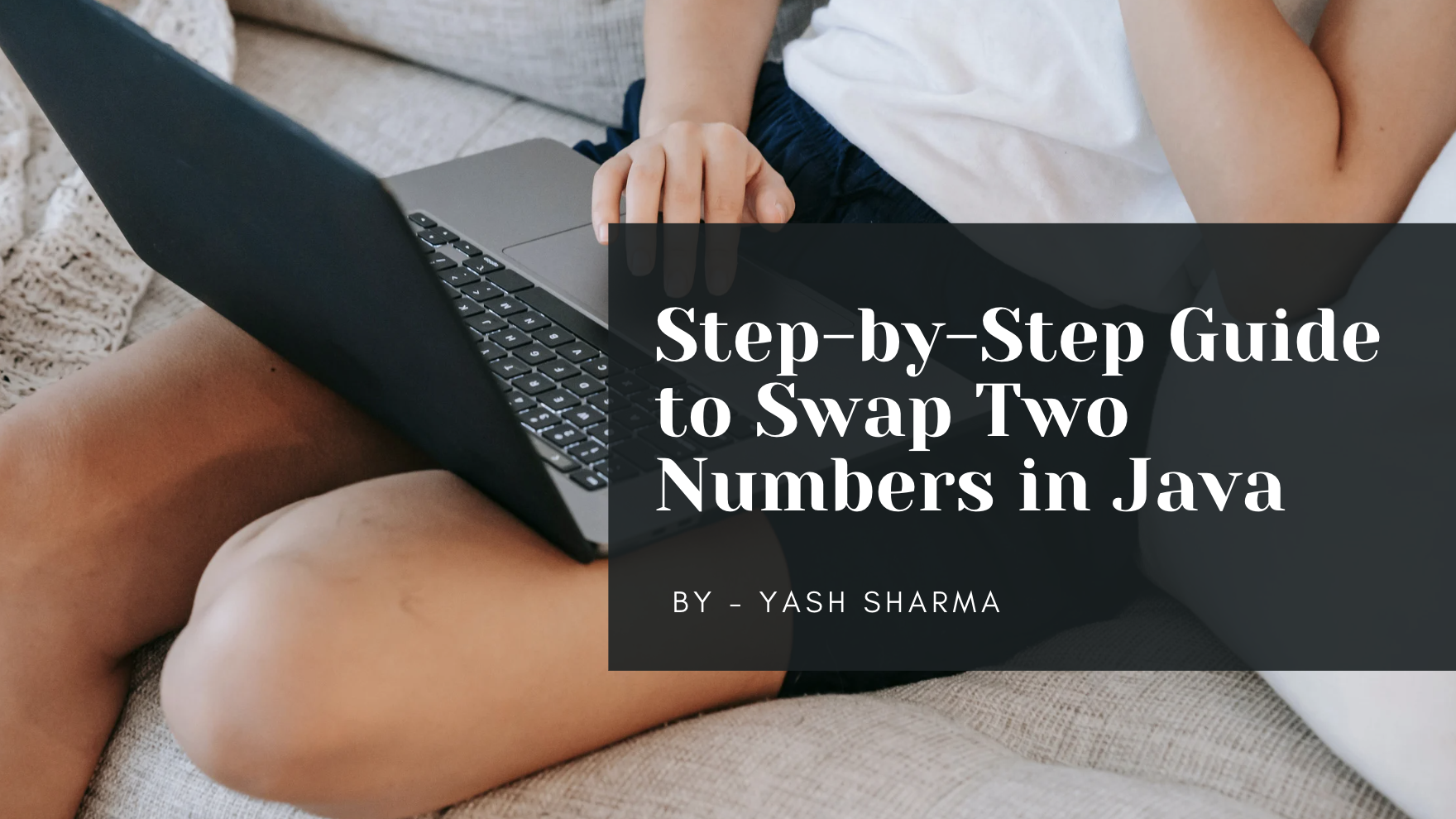
Swapping Two Numbers in Java
Problem Description
We are given two variables, a
and b
, with initial values. The task is to swap the values of these two variables.
Examples
Example 1:
Input:
a = 10
,b = 20
Output:
a = 20
,b = 10
Example 2:
Input:
a = "Geeks"
,b = "gfg"
Output:
a = "gfg"
,b = "Geeks"
Incorrect Approach
javaCopy codeint a = 10;
int b = 20;
a = b;
b = a;
This approach fails because:
When
a = b
is executed,a
becomes20
.When
b = a
is executed,b
also becomes20
.Both
a
andb
end up being20
, which is incorrect.
Correct Approach
To swap two values correctly, we need an extra temporary variable.
Steps:
Initialization:
javaCopy codeint a = 10; int b = 20; int temp = 0; // temporary variable
Swapping Process:
Store the value of
a
intemp
:javaCopy codetemp = a; // temp = 10
Assign the value of
b
toa
:javaCopy codea = b; // a = 20
Assign the value stored in
temp
tob
:javaCopy codeb = temp; // b = 10
Output:
javaCopy codeSystem.out.println("a = " + a); // a = 20 System.out.println("b = " + b); // b = 10
Java Implementation
javaCopy codepublic class SwapNumbers {
public static void main(String[] args) {
// Initialize variables
int a = 10;
int b = 20;
// Temporary variable for swapping
int temp = a;
a = b;
b = temp;
// Print the swapped values
System.out.println("a = " + a); // a = 20
System.out.println("b = " + b); // b = 10
}
}
Explanation with Memory Blocks
Initial Values:
a
points to10
.b
points to20
.
After
temp = a
:temp
points to10
(value ofa
).
After
a = b
:a
points to20
(value ofb
).
After
b = temp
:b
points to10
(value stored intemp
).
Interview Questions
Why can't we directly assign
a = b
andb = a
to swap values?- Direct assignment loses the original value of
a
, making it impossible to correctly assignb
.
- Direct assignment loses the original value of
Can you swap values without using a temporary variable?
- Yes, by using arithmetic or bitwise operations.
How would you swap two strings in Java?
The process is the same as with numbers:
javaCopy codeString a = "Geeks"; String b = "gfg"; String temp = a; a = b; b = temp;
What are other methods to swap two variables in Java?
Using arithmetic operations:
javaCopy codea = a + b; b = a - b; a = a - b;
Using bitwise XOR:
javaCopy codea = a ^ b; b = a ^ b; a = a ^ b;
Why might you prefer using a temporary variable for swapping in interviews?
- It's straightforward and clear, minimizing the risk of errors in complex operations.
This guide should help you understand the concept of swapping values in Java, along with common pitfalls and alternative methods.
Subscribe to my newsletter
Read articles from Yash Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Yash Sharma
Yash Sharma
Final-year CSE student at Chandigarh University passionate about web development and data structures and algorithm. I write articles on Java and DSA in Java, sharing insights and coding tips. Exploring my journey into teaching while refining my skills in software development.