10 Python Programs for Beginners: Simple Explanations and Examples
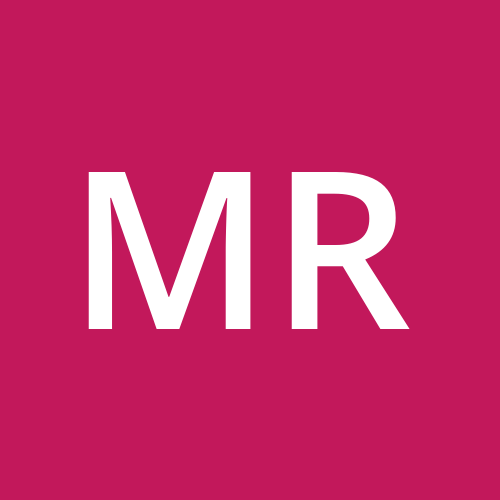
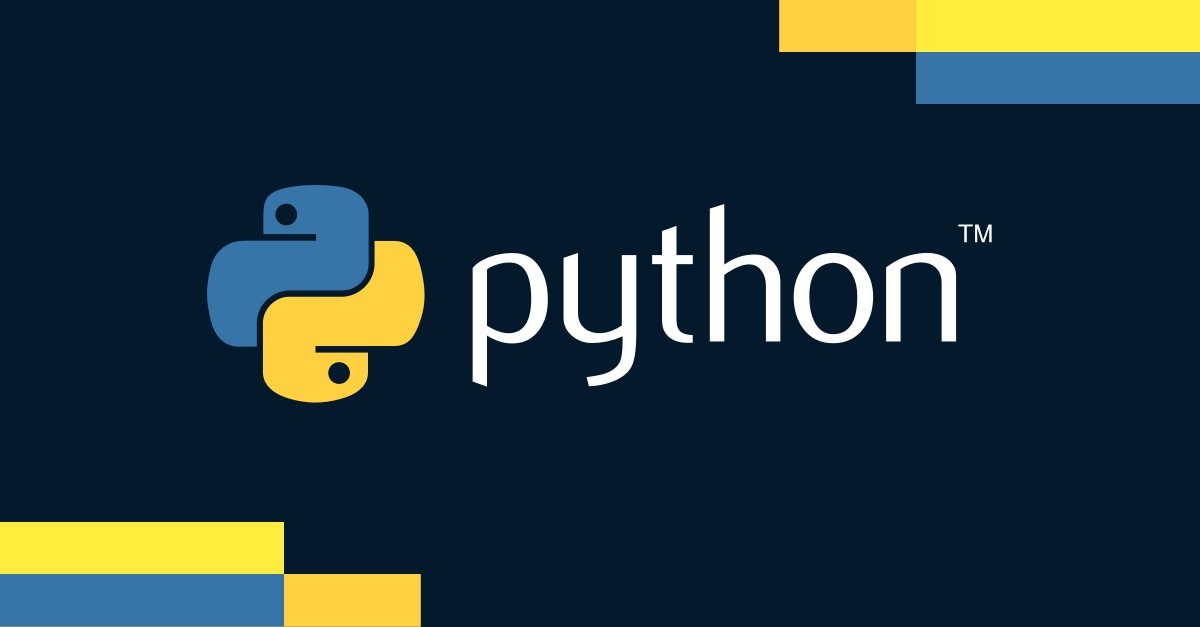
Hey, my friend, congratulations on starting to learn Python. I'm happy to share my knowledge with you. Let's dive into some basic and popular Python problems. "Sit back, relax, and enjoy."
Sure! Here's a more detailed explanation of each program in simple English:
1. Greeting Function
Let's start with a simple greeting function. This program asks for your name and then greets you.
def greet():
user = input("Enter Your Name!: ")
user = user.capitalize()
print(f"Hello {user}, Nice to meet you")
result = greet()
print(result)
Explanation:
The function
greet()
asks the user to enter their name using theinput()
function.It then capitalizes the first letter of the name using the
capitalize()
method to ensure the name looks nice.The function prints a friendly greeting message that includes the user's name.
Since
greet()
doesn't return any value, the variableresult
will beNone
, which is why "None" is printed at the end.
2. Fahrenheit to Celsius Converter
This program converts a temperature from Fahrenheit to Celsius.
def f_to_c():
f = int(input("Enter a temperature in Fahrenheit: "))
s = (f - 32) * 5.0 / 9.0
return s
celcius = f_to_c()
print(f"The converted temperature in Celsius is: {celcius:.2f}")
Explanation:
The function
f_to_c()
asks the user to enter a temperature in Fahrenheit using theinput()
function and converts it to an integer.It then calculates the Celsius equivalent using the formula
(F - 32) * 5/9
.The function returns the Celsius temperature.
The result is printed with two decimal places using the format specifier
:.2f
.
3. Count Vowels
This program counts the number of vowels in a given string.
def no_vowels():
word = input("Enter a word or a sentence: ")
vowels = ['a', 'e', 'i', 'o', 'u']
count = 0
for char in word:
if char in vowels:
count += 1
return count
result = no_vowels()
print(f"The total number of vowels in the given string is {result}")
Explanation:
The function
no_vowels()
asks the user to enter a word or sentence.It initializes a list of vowels
['a', 'e', 'i', 'o', 'u']
and a countercount
set to 0.It then loops through each character in the input word.
If the character is a vowel (i.e., it is in the vowels list), the counter is incremented by 1.
The function returns the total count of vowels in the input string.
4. Find the Maximum
This program finds the largest number in a list of numbers.
def find_max():
numbers = input("Enter a list of numbers separated by spaces: ")
numbers = list(map(int, numbers.split()))
return max(numbers)
result = find_max()
print(f"The maximum number in the given list is {result}")
Explanation:
The function
find_max()
asks the user to enter a list of numbers separated by spaces.It converts the input string into a list of integers using
map(int, numbers.split())
.It then finds the maximum number in the list using the
max()
function.The function returns the maximum number.
5. String Reversal
This program reverses a given string.
def reverse():
sent = input("Enter a string: ")
return sent[::-1]
result = reverse()
print(result)
Explanation:
The function
reverse()
asks the user to enter a string.It reverses the string using slicing
[::-1]
, which means it starts from the end of the string and goes backwards.The function returns the reversed string.
6. Check Even or Odd
This program checks if a given number is even or odd.
def evenorodd():
a = int(input("Enter any number to find if it's even or odd: "))
if a % 2 == 0:
return "Even"
else:
return "Odd"
print(evenorodd())
Explanation:
The function
evenorodd()
asks the user to enter a number and converts it to an integer.It checks if the number is divisible by 2 using the modulus operator
%
.If the number is divisible by 2 (i.e.,
a % 2 == 0
), the function returns "Even".Otherwise, it returns "Odd".
7. Factorial
This program calculates the factorial of a non-negative integer.
def factorial():
n = int(input("Enter a number to find its factorial: "))
result = 1
for i in range(1, n + 1):
result *= i
return result
print(factorial())
Explanation:
The function
factorial()
asks the user to enter a non-negative integer and converts it to an integer.It initializes a variable
result
to 1.It then uses a for loop to multiply
result
by each number from 1 ton
.The function returns the factorial of the number.
8. Palindrome Check
This program checks if a given string is a palindrome.
def palindrome():
n = input("Enter a string to check if it's a palindrome: ")
if n == n[::-1]:
return "The given string is a palindrome"
else:
return "The given string is not a palindrome"
print(palindrome())
Explanation:
The function
palindrome()
asks the user to enter a string.It checks if the string is the same forwards and backwards by comparing it to its reversed version (
n[::-1]
).The function returns "The given string is a palindrome" if they are the same.
Otherwise, it returns "The given string is not a palindrome".
9. Fibonacci Sequence
This program returns the nth number in the Fibonacci sequence.
def fibonacci(n):
if n == 0:
return 0
elif n == 1:
return 1
else:
return fibonacci(n - 1) + fibonacci(n - 2)
print(fibonacci(9))
Explanation:
The function
fibonacci(n)
calculates the nth Fibonacci number using recursion.If
n
is 0, it returns 0.If
n
is 1, it returns 1.Otherwise, it calls itself twice: once with
n-1
and once withn-2
, and returns the sum of those two calls.The
print(fibonacci(9))
statement prints the 9th Fibonacci number.
10. Sum of Numbers in a List
This program calculates the sum of all the numbers in a given list.
def sum_of_numbers():
numbers = input("Enter a list of numbers separated by spaces: ")
numbers = list(map(int, numbers.split()))
return sum(numbers)
result = sum_of_numbers()
print(f"The sum of the numbers in the list is {result}")
Explanation:
The function
sum_of_numbers()
asks the user to enter a list of numbers separated by spaces.It converts the input string into a list of integers using
map(int, numbers.split())
.It calculates the sum of all numbers in the list using the
sum()
function.The function returns the sum.
These 10 simple Python programs cover various basic concepts and provide a good foundation for you, my friend. For more interesting content like this, make sure to subscribe to my blog. Happy coding!
Subscribe to my newsletter
Read articles from Mugil Raja directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
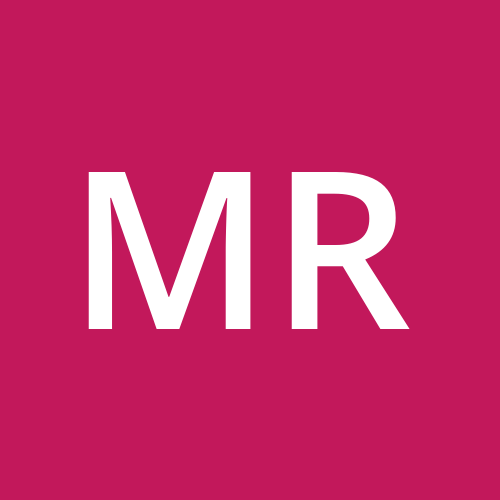