SASS for Beginners: A Step-by-Step Guide
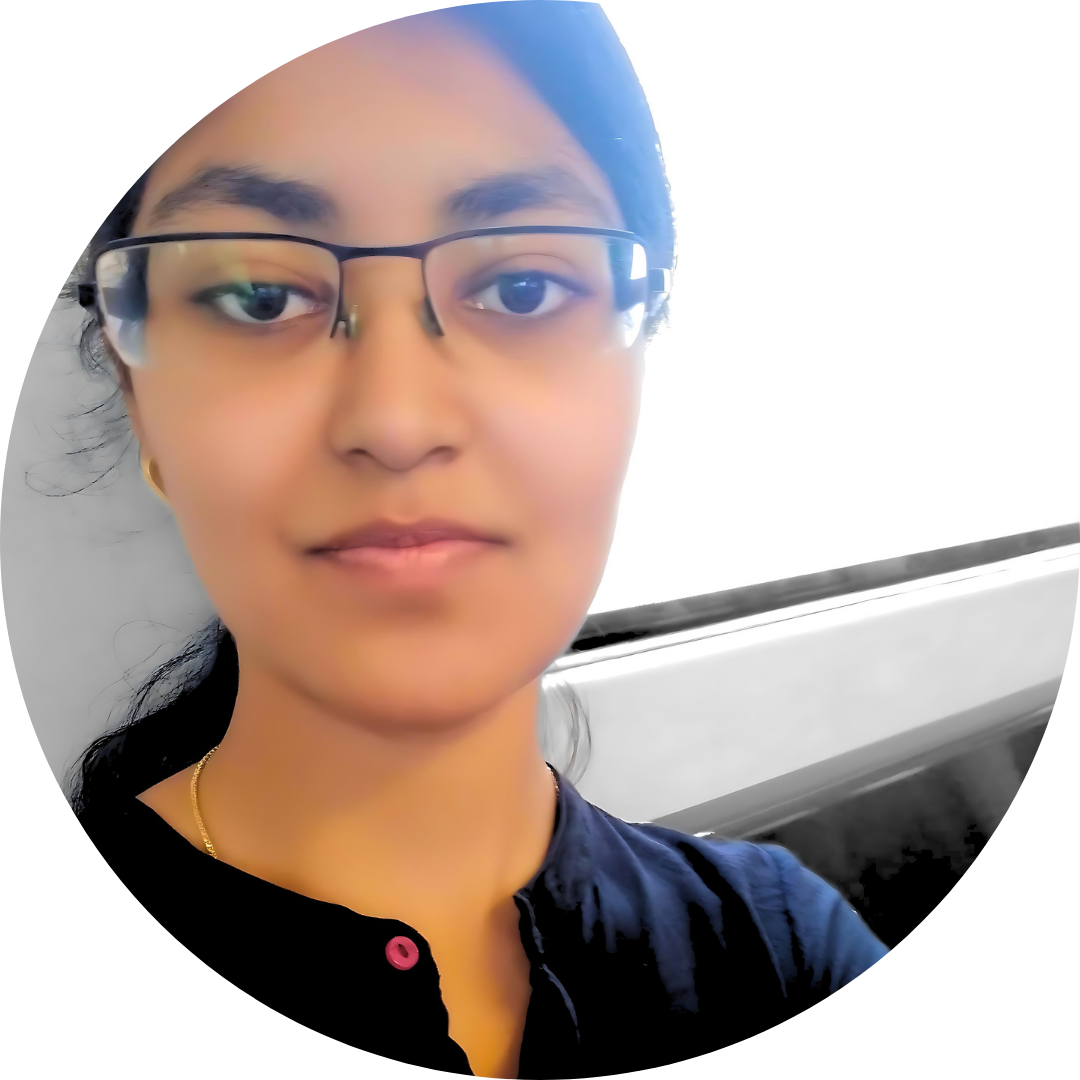
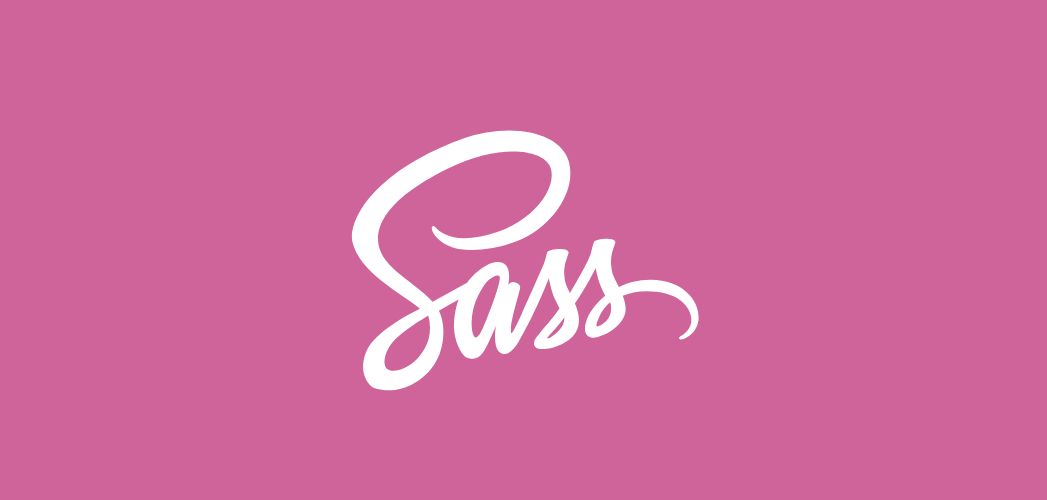
introduction
sass is a css preprocessor -- meaning sass cannot be run by browser, sass code should be converted to css to make it run on browser
Sass has two syntaxes:
SCSS (Sassy CSS)
Sass (indented syntax)
SCSS is more popular and resembles regular CSS.
BOOTSTRAP library uses SASS
how to use as a beginner
as a beginner you can compile sass in your basic html project using sass compiling vscode extensions
- your css code will be generated in a dist folder , link that style.css file to index.html
in huge projects sass is converted to css in build process by bundlers like webpack, parcel
why sass?
Sass allows you to write more maintainable, scalable, and easier-to-read stylesheets.
It extends CSS with features like variables, nested rules, mixins, and functions, making your CSS more dynamic and organized.
sass nesting
Nesting in Sass refers to the way you can nest CSS selectors within one another to mimic the hierarchical structure of your HTML.
This helps in writing more readable and organized styles
// SCSS syntax
nav {
ul {
margin: 0;
padding: 0;
list-style: none;
li {
display: inline-block;
margin-right: 5px;
a {
text-decoration: none;
}
}
}
}
sass partials
- Partials are Sass files named with a leading underscore (e.g.,
_partial.scss
).
The underscore tells Sass that the file is a partial and should not be compiled into a standalone CSS file. Instead, these partials are imported into other Sass files.
Example partial files:
_variables.scss
: Contains all your variables._mixins.scss
: Contains mixins._reset.scss
: Contains CSS reset rules._header.scss
: Contains styles for the header component.
Benefits of Using Partials
Organization: Clearly defined structure makes it easy to find and manage styles.
Reusability: Common styles and components can be reused across different projects.
Maintainability: Updates and modifications are simpler as you only need to edit specific partials.
Collaboration: Multiple developers can work on different parts of the styles without causing conflicts.
the 7-1 pattern
The 7-1 pattern in Sass is a well-organized structure for managing large projects
Basically, you have all your partials stuffed into 7 different folders
all the partials are imported into main.scss and it is compiled into a CSS stylesheet.
// main.scss
/* while using/importing partials no need to use
underscore,scss extension */
// 1. Abstracts
@use 'abstracts/variables';
@use 'abstracts/mixins';
// 2. Vendors
@use 'vendors/bootstrap';
// 3. Base
@use 'base/reset';
@use 'base/typography';
// 4. Layout
@use 'layout/header';
@use 'layout/footer';
// 5. Components
@use 'components/buttons';
// 6. Pages
@use 'pages/contact';
// 7. Themes
@use 'themes/theme';
Here's a breakdown of the 7 folders and the main file:
1. abstracts: Contains functions, mixins, and variables.
2. base: Holds global styles such as resets, typography rules, and base elements.
3. components: Includes small, reusable parts of the design like buttons, forms, and cards.
4. layout: Deals with the overall structure of the site, such as the grid system, header, footer, and sidebars.
5. pages: Styles specific to individual pages.
6. themes: Contains styles for different themes.
7. vendors: Stores styles from external libraries and frameworks.
@import
v/s @use
in sass
both @import
and @use
are used to include styles from Sass partials, but they have different purposes and behaviors
@use
is recommended over@import
@import
cons
Global Scope: All variables, mixins, and functions are available globally, which can lead to naming conflicts and unintentional overrides.
Compilation Time: each
@import
statement in Sass causes the compiler to load and process the imported file immediately. This can lead to redundant processing of files if the same file is imported multiple times across different Sass files.Dependency Management:
@import
can lead to tangled dependencies and difficulties in managing which files are being imported where, especially in large projects.Deprecation:
@import
is being deprecated in favor of@use
and@forward
// main.scss
@import 'variables';
/*here all styles in variables partial can be accessed by all lines
which are after this import so even the mixins partial can access those
styles*/
@import 'mixins';
.button {
color: $primary-color;
@include border-radius(5px);
}
@use
pros
Namespace: By default,
@use
adds all the members (variables, mixins, functions) under a namespace, preventing naming conflicts.Explicit Imports: You can control what gets imported and make your stylesheets more modular and maintainable.
Single Load: With
@use
, Sass only loads the imported file once per build process, regardless of how many times it is imported across different files. This reduces redundancy and speeds up the compilation process.
// main.scss
@use 'variables' as *;
/*here mixins partial cannot access styles of variables partial only
main.scss file has access to variables partial*/
@use 'mixins' as mx;
.button {
color: $primary-color;
@include mx.border-radius(5px); // mx is namespace
}
Combining @use
and @forward
For large projects, you might want to combine @use
with @forward
to create a more organized structure. @forward
allows you to re-export members from a module:
// _all.scss
@forward 'variables';
@forward 'mixins';
// main.scss
@use 'all';
.button {
color: all.$primary-color;
@include all.border-radius(5px);
}
Sass variables
they store values that you want to reuse throughout your stylesheet
you can assign a wide variety of data types like numbers, strings, colors, boolean, lists, maps, null, calculations to Sass variables, making them incredibly versatile for handling different kinds of data
// Numbers
$base-font-size: 16px;
$line-height: 1.5;
$width-percentage: 75%;
// Strings
$font-family: 'Helvetica, sans-serif';
// Colors
$primary-color: #3498db;
// Booleans
$is-responsive: true;
// Lists
$font-stack: Helvetica, Arial, sans-serif;
// Maps
$theme-colors: (
primary: #3498db,
secondary: #2ecc71,
accent: #e74c3c
);
// Null
$optional-border: null;
// Calculation Expressions
$base-spacing: 10px;
$double-spacing: $base-spacing * 2;
Sass lists
groups multiple values together
// Comma-separated list
$colors: red, green, blue;
//iterating through sass list
@each $color in $colors {
.card-#{$color} { //class selector
background-color : $color;
}
}
Sass maps
//sass map with key as number and value as a string of image path
$card-images : (
1 : '../../images/desktop/image-deep-earth.jpg',
2 : '../../images/desktop/image-night-arcade.jpg',
3 : '../../images/desktop/image-soccer-team.jpg',
4 : '../../images/desktop/image-grid.jpg',
)
//iterating through sass map
@each $card-num,$card-image in $card-images{
.card-#{$card-num}{ //class selector
background-image: url($card-image);
}
}
mixins
Mixins are reusable pieces of code that can be included in other selectors.
@mixin border-radius($radius) {
-webkit-border-radius: $radius;
-moz-border-radius: $radius;
border-radius: $radius;
}
button {
@include border-radius(10px);
}
functions
Sass allows you to define custom functions to reuse code and logic.
// Define a base font size for rem calculations
$base-font-size: 16px;
// Function to convert pixels to rems
@function px-to-rem($px) {
@return $px / $base-font-size * 1rem;
}
// Example of using the function to create spacing variables
$spacing-small: px-to-rem(8px);
Extending/Inheriting Styles
The @extend
directive lets you share a set of CSS properties across several selectors
%message-shared {
border: 1px solid #ccc;
padding: 10px;
color: #333;
}
.message {
@extend %message-shared;
}
my html, scss project
checkout a basic html,scss project done by me to get a overview on how to use scss
Subscribe to my newsletter
Read articles from prasanna pinnam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
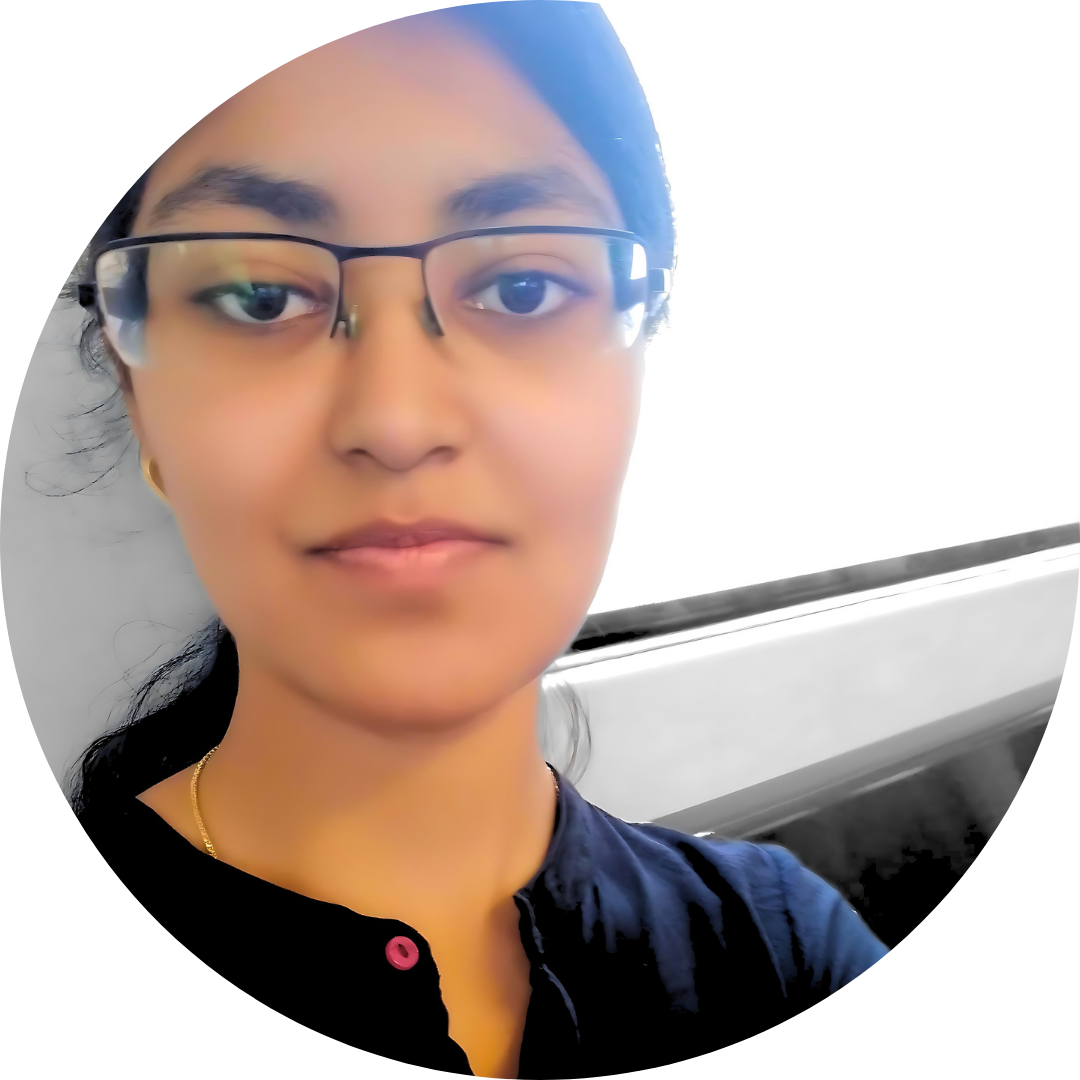