Understanding Data Structures in Go
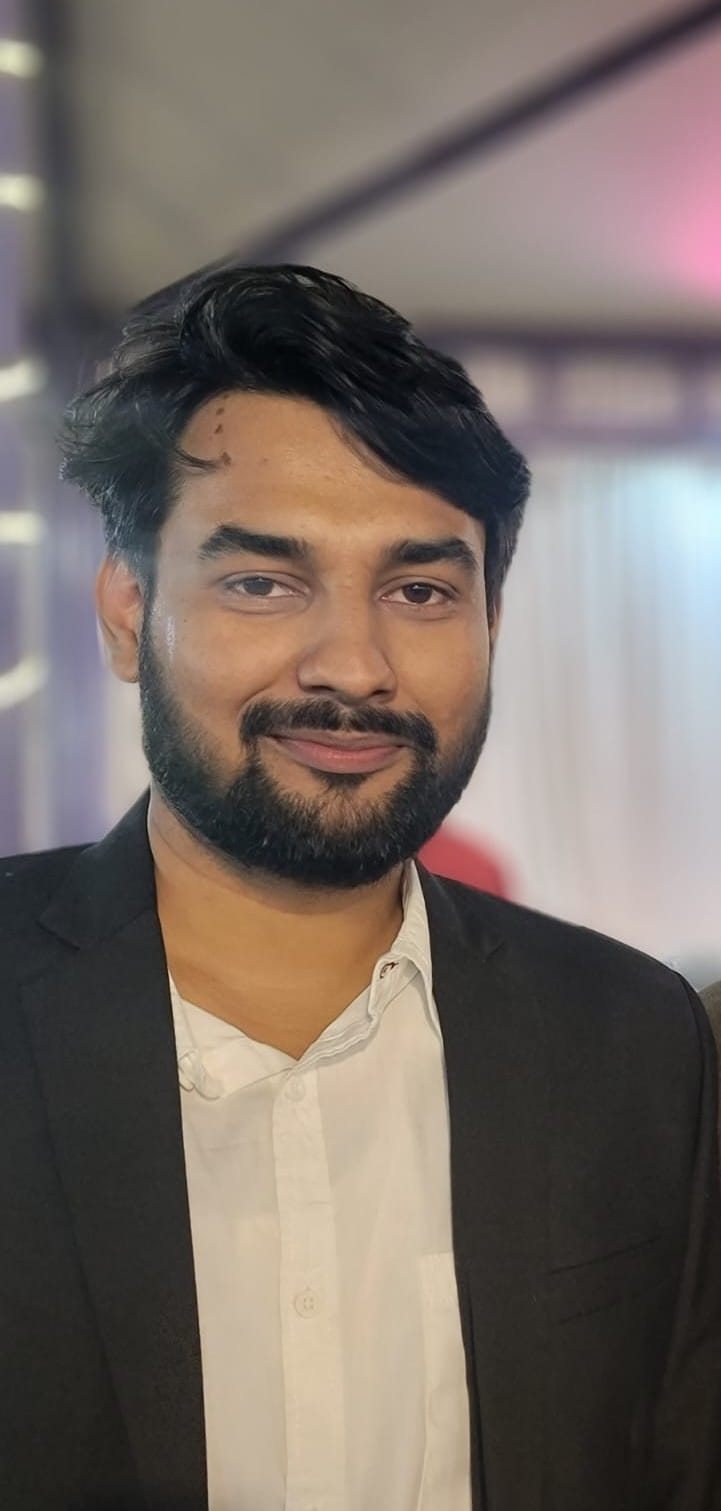
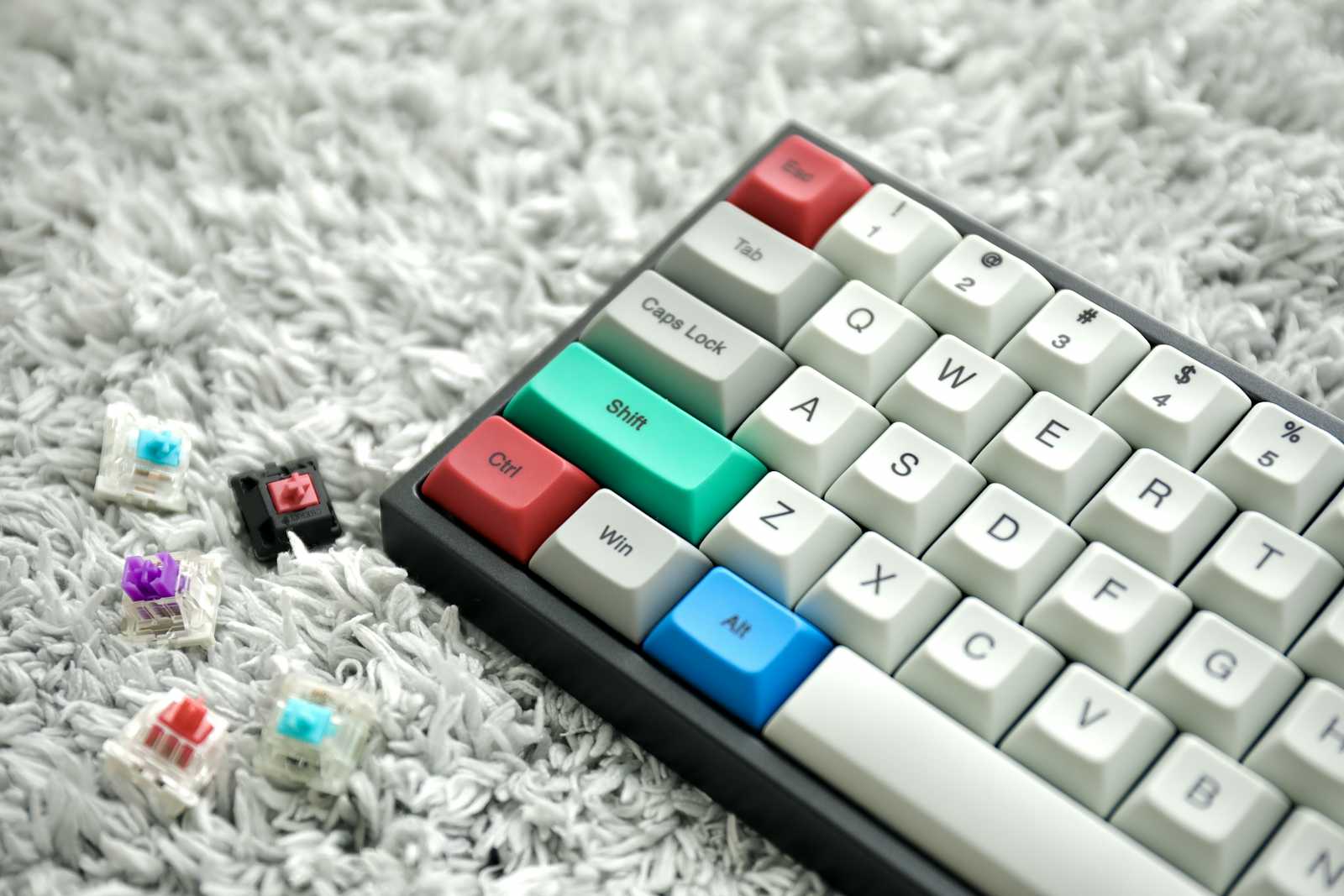
Data structures are crucial for organizing and storing data efficiently in any programming language. In Go (Golang), several fundamental data structures are available to developers, including arrays, slices, maps, and structs. This article will explore these data structures, providing examples to illustrate their usage.
Arrays and Slices
Arrays:
An array is a fixed-size collection of elements of the same type. The size of an array is defined at the time of its declaration and cannot be changed.
Example:
package main
import "fmt"
func main() {
var numbers [5]int
numbers[0] = 10
numbers[1] = 20
numbers[2] = 30
numbers[3] = 40
numbers[4] = 50
fmt.Println(numbers) // Output: [10 20 30 40 50]
}
In this example, we declare an array numbers
of size 5 and assign values to each element.
Slices:
Slices are a more flexible and powerful data structure than arrays. Unlike arrays, slices can grow and shrink dynamically. Slices are built on top of arrays and provide a more convenient and efficient way to work with sequences of data.
Example:
package main
import "fmt"
func main() {
numbers := []int{10, 20, 30, 40, 50}
fmt.Println(numbers) // Output: [10 20 30 40 50]
// Adding an element to the slice
numbers = append(numbers, 60)
fmt.Println(numbers) // Output: [10 20 30 40 50 60]
// Slicing the slice
subSlice := numbers[1:4]
fmt.Println(subSlice) // Output: [20 30 40]
}
In this example, we create a slice numbers
and demonstrate appending an element and slicing the slice.
Maps
Maps in Go are unordered collections of key-value pairs. They provide an efficient way to associate values with unique keys.
Example:
package main
import "fmt"
func main() {
// Creating a map
colors := make(map[string]string)
// Adding key-value pairs
colors["red"] = "#FF0000"
colors["green"] = "#00FF00"
colors["blue"] = "#0000FF"
fmt.Println(colors) // Output: map[blue:#0000FF green:#00FF00 red:#FF0000]
// Accessing a value
fmt.Println("The hex code for red is", colors["red"]) // Output: The hex code for red is #FF0000
// Deleting a key-value pair
delete(colors, "green")
fmt.Println(colors) // Output: map[blue:#0000FF red:#FF0000]
}
In this example, we create a map colors
, add key-value pairs, access a value, and delete a key-value pair.
Structs
Structs are composite data structures that group together variables under a single name. These variables, known as fields, can be of different types. Structs are particularly useful for representing complex data types and defining custom types.
Example:
package main
import "fmt"
// Defining a struct
type Person struct {
Name string
Age int
}
func main() {
// Creating a new instance of the struct
person1 := Person{Name: "Alice", Age: 25}
person2 := Person{"Bob", 30}
fmt.Println(person1) // Output: {Alice 25}
fmt.Println(person2) // Output: {Bob 30}
// Accessing struct fields
fmt.Println("Name:", person1.Name) // Output: Name: Alice
fmt.Println("Age:", person1.Age) // Output: Age: 25
// Modifying struct fields
person1.Age = 26
fmt.Println("Updated Age:", person1.Age) // Output: Updated Age: 26
}
In this example, we define a Person
struct with Name
and Age
fields, create instances of the struct, access and modify its fields.
Conclusion
Go provides several essential data structures, including arrays, slices, maps, and structs, each with unique features and capabilities. Arrays offer fixed-size collections, while slices provide dynamic and flexible sequences. Maps allow efficient key-value pair associations, and structs enable the grouping of different data types under a single name. Mastering these data structures is crucial for writing efficient and robust Go programs. Keep practicing and experimenting with these data structures to enhance your Go programming skills.
Subscribe to my newsletter
Read articles from Lokesh Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
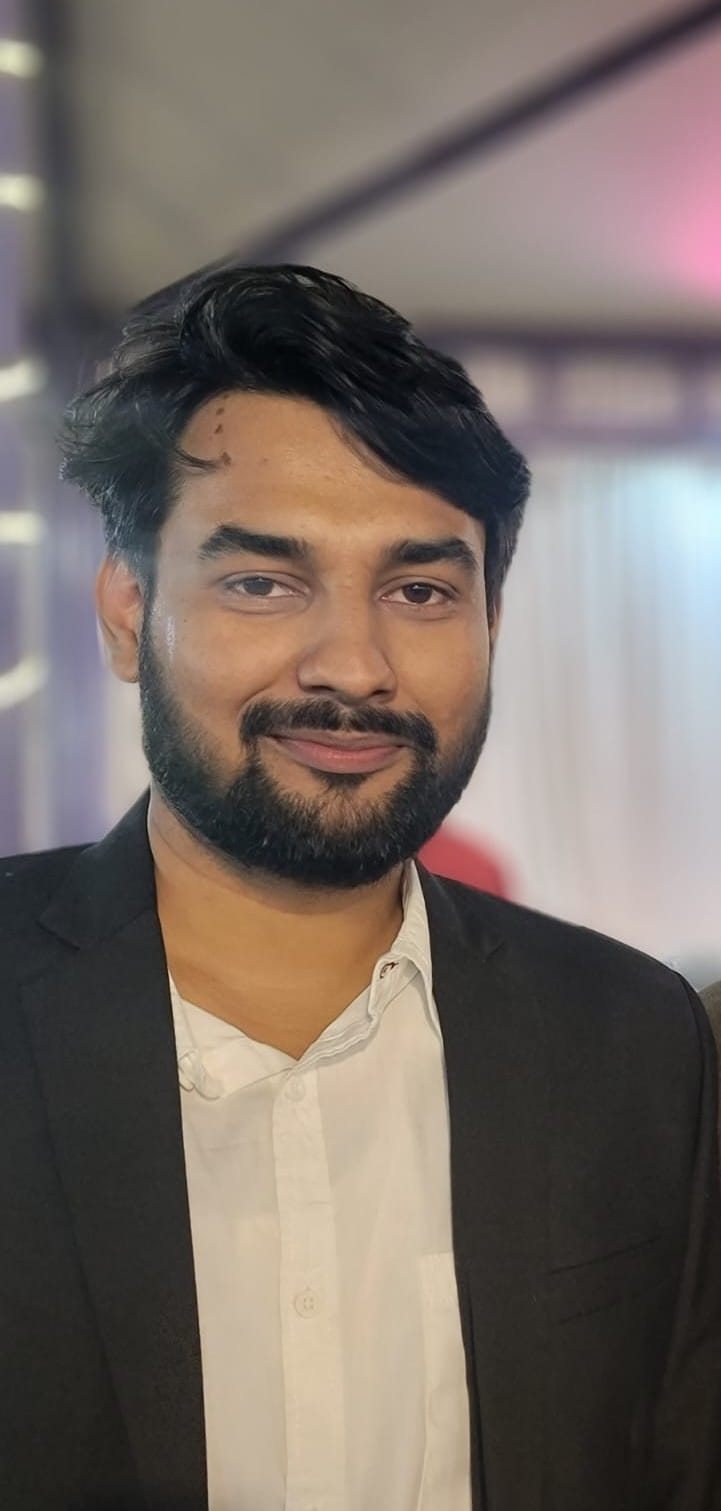
Lokesh Jha
Lokesh Jha
I am a senior software developer and technical writer who loves to learn new things. I recently started writing articles about what I've learned so that others in the community can gain the same knowledge. I primarily work with Node.js, TypeScript, and JavaScript, but I also have past experience with Java and C++. Currently, I'm learning Go and may explore Web3 in the future.