Encapsulation in Object oriented programming
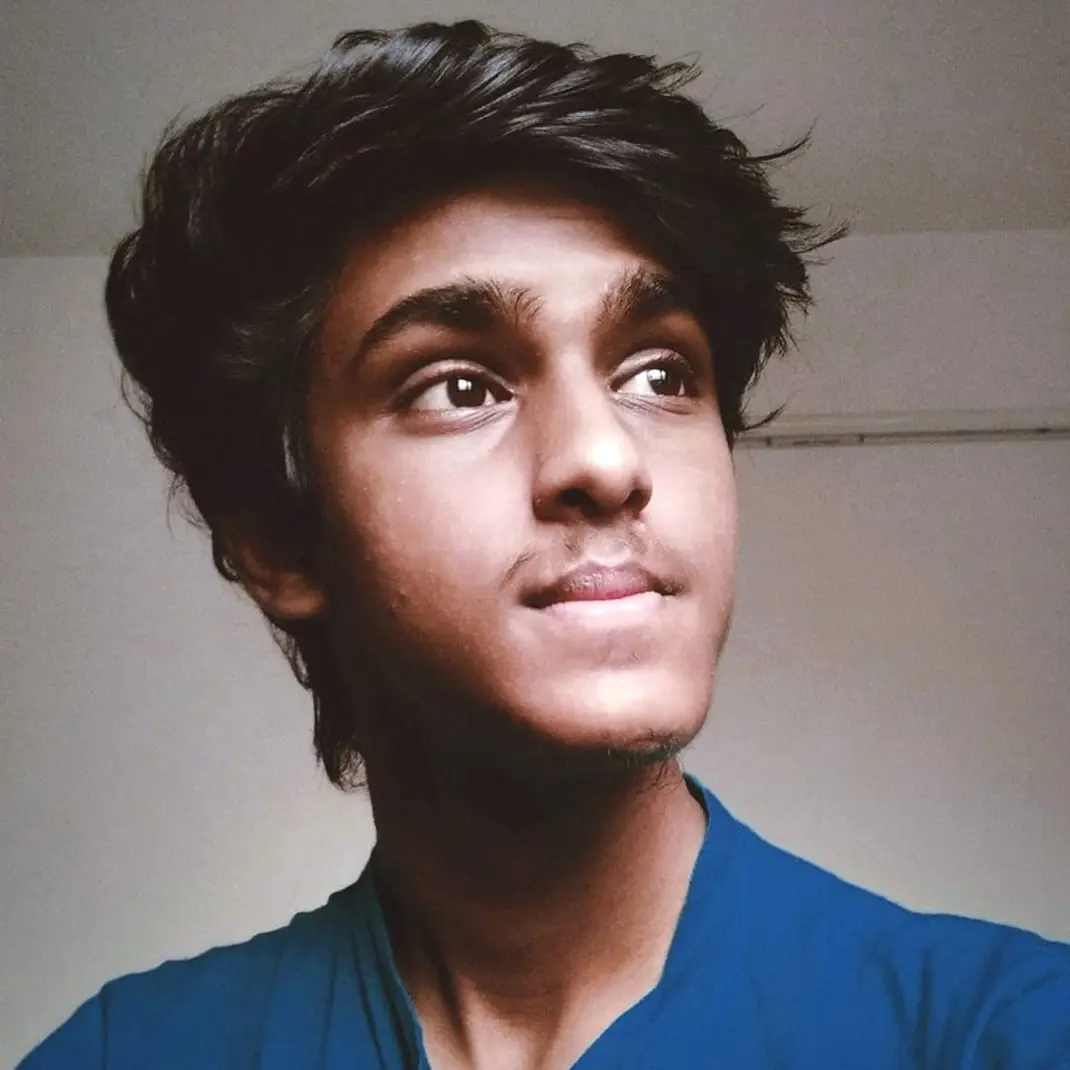
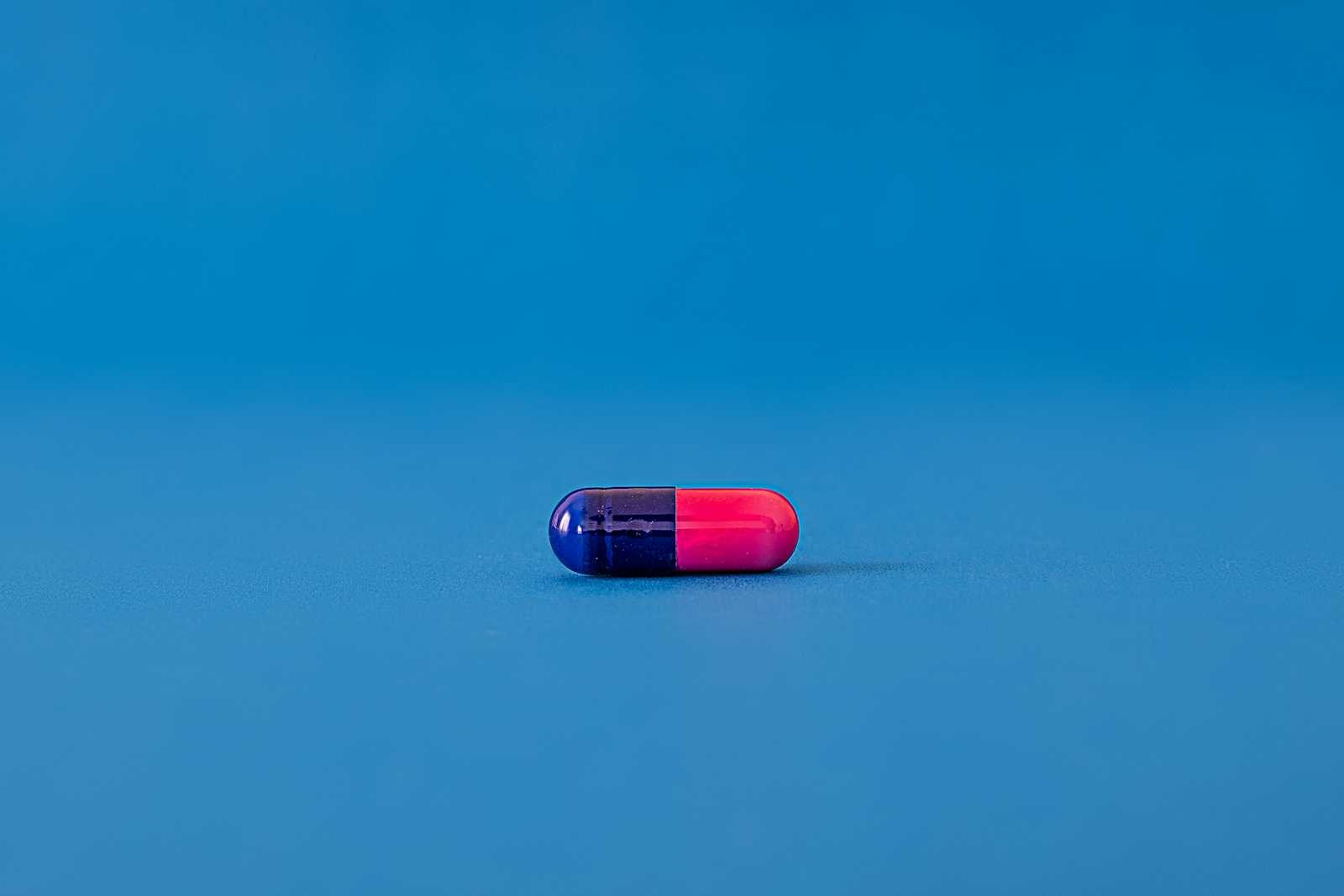
Introduction to Encapsulation
Encapsulation is one of the main pillars of object oriented programming. It is a process of hiding a data member by declaring it as a private data member thereby enhancing its security.
Private data members can only be accessed within the specific class where they are declared.
Encapsulation is used to achieve data security, robust, maintainable, and secure software design in OOP. Encapsulation is a way of binding data members and member functions together.
Example of Encapsulation in Java
public class Student {
private int age, rollno; // Private data members
private String name;
public void setAge(int age) { // Public setter for age
if (age >= 5) {
this.age = age;
} else {
System.out.println("Student should be above 5 years");
}
}
public void setRollNo(int rollno) { // Public setter for roll no
if (rollno > 0) {
this.rollno = rollno;
} else {
System.out.println("Roll number should be positive");
}
}
public void setName(String name) { // Public setter for name
this.name = name;
}
public int getAge() { // Public getter for age
return age;
}
public int getRollNo() { // Public getter for roll no
return rollno;
}
public String getName() { // Public getter for name
return name;
}
}
Explanation
In the Student
class, we declare age
, rollno
, and name
as private data members to achieve encapsulation. This ensures that the data members and member functions are bound to each other within the class.
setAge(int age)
: Ensures the student's age is at least 5 years.
setRollNo(int rollno)
: Ensures the roll number is positive.
setName(String name)
: Assigns the provided name to the student.
We then use public setter and getter methods to make the data members accessible outside the class.
In the below lines of code lets set the value for the private data members.
Encapsulation Implementation Class
public class StudApp {
public static void main(String[] args) {
Student s1 = new Student(); // Create a new Student object
// Set the values for the private data members using setter methods
s1.setRollNo(12); // Set the roll number to 12
s1.setName("Rakesh"); // Set the name to Rakesh
s1.setAge(7); // Set the age to 7
// Get the values of the private data members using getter methods
int s1RollNo = s1.getRollNo(); // Get the roll number
String s1Name = s1.getName(); // Get the name
int s1Age = s1.getAge(); // Get the age
// Print the values of the data members
System.out.println("RollNo: " + s1RollNo); // Output: 12
System.out.println("Name: " + s1Name); // Output: Rakesh
System.out.println("Age: " + s1Age); // Output: 7
}
}
Explanation
Create a new Student object:
Student s1 = new Student();
: Instantiates a newStudent
object nameds1
.
Set the values for the private data members using setter methods:
s1.setRollNo(12);
: Sets the roll number to 12.s1.setName("Rakesh");
: Sets the name to "Rakesh".s1.setAge(7);
: Sets the age to 7.
Get the values of the private data members using getter methods:
String s1Name = s1.getName();
: Retrieves the name of the student.int s1Age = s1.getAge();
: Retrieves the age of the student.int s1Rollno = s1.getRollNo();
: Retrieves the roll number of the student.
Print the values of the data members:
System.out.println(s1Rollno);
: Prints the roll number.System.out.println(s1Name);
: Prints the name.System.out.println(s1Age);
: Prints the age.
This code demonstrates how to create an instance of the Student
class, set the values of its private data members using public setter methods, retrieve these values using public getter methods, and print the values to the console.
Expected Output
Advantages of Encapsulation in Java
Encapsulation, a fundamental concept in object-oriented programming (OOP), offers several key benefits:
Data Protection:
By making data members private, encapsulation hides the internal state of objects from external access, enhancing security.
This prevents accidental or unauthorized modifications.
Controlled Access:
Public getter and setter methods allow controlled access to private data members.
This ensures that any changes to the data are made in a controlled and predictable manner.
Improved Maintainability:
Encapsulation keeps related data and methods together, making the code easier to manage and maintain.
Changes to the internal implementation of a class do not affect other parts of the program that use the class.
Simplified Code:
Encapsulation provides a clear and simple interface for interacting with objects, making the code easier to understand and use.
This abstraction hides complex implementation details from the user.
Data Validation:
Setter methods can include validation logic to ensure that only valid data is assigned to private variables.
This helps maintain the integrity and correctness of the data.
Conclusion
Encapsulation is achieved by declaring data members (age, rollno, name) as private within the Student class. This protects their integrity and ensures controlled access via setter and getter methods.
Student Class:
Private Data Members:
age
,rollno
, andname
are private for internal state protection.Setter Methods: Validate and set data, like ensuring age is valid.
Getter Methods: Provide controlled access to retrieve data.
StudApp Class:
Setting Values: Uses setters to initialize
Student
object data.Getting Values: Retrieves and prints data using getters.
This example demonstrates how encapsulation promotes data security and maintainability in Java, ensuring reliable software design through controlled data access and modification.
Subscribe to my newsletter
Read articles from Sridhar K directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
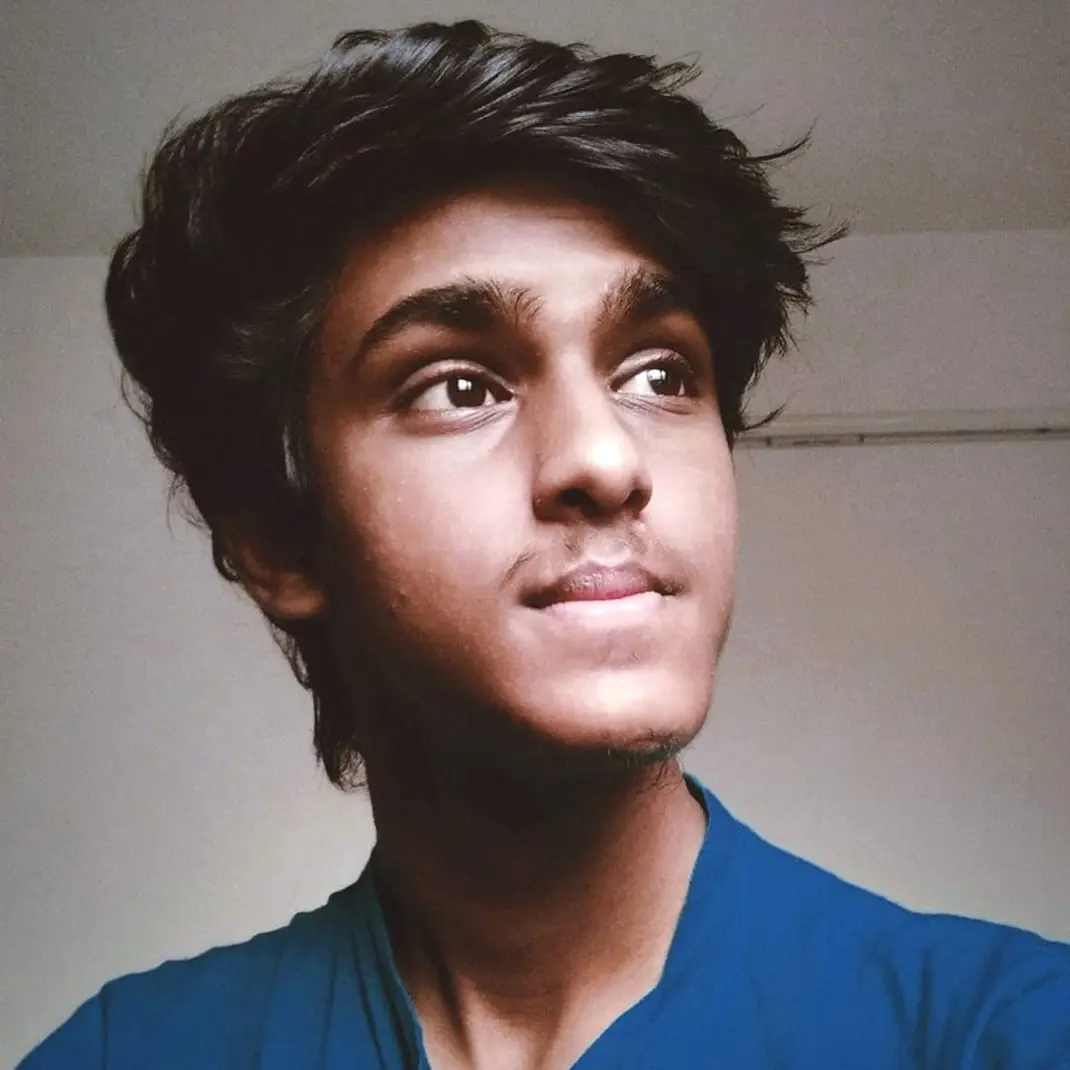
Sridhar K
Sridhar K
I am a fresher Software developer with a strong foundation in Java, HTML, CSS, and JavaScript. I have experience with various libraries and frameworks, including Spring Boot, Express.js, Node.js, Hibernate, MySQL, and PostgreSQL. I am eager to leverage my technical skills and knowledge to contribute effectively to a dynamic development team. I am committed to continuous learning and am excited to begin my career in a challenging developer role.