Navigating CLI Projects with Python OOP and SQL

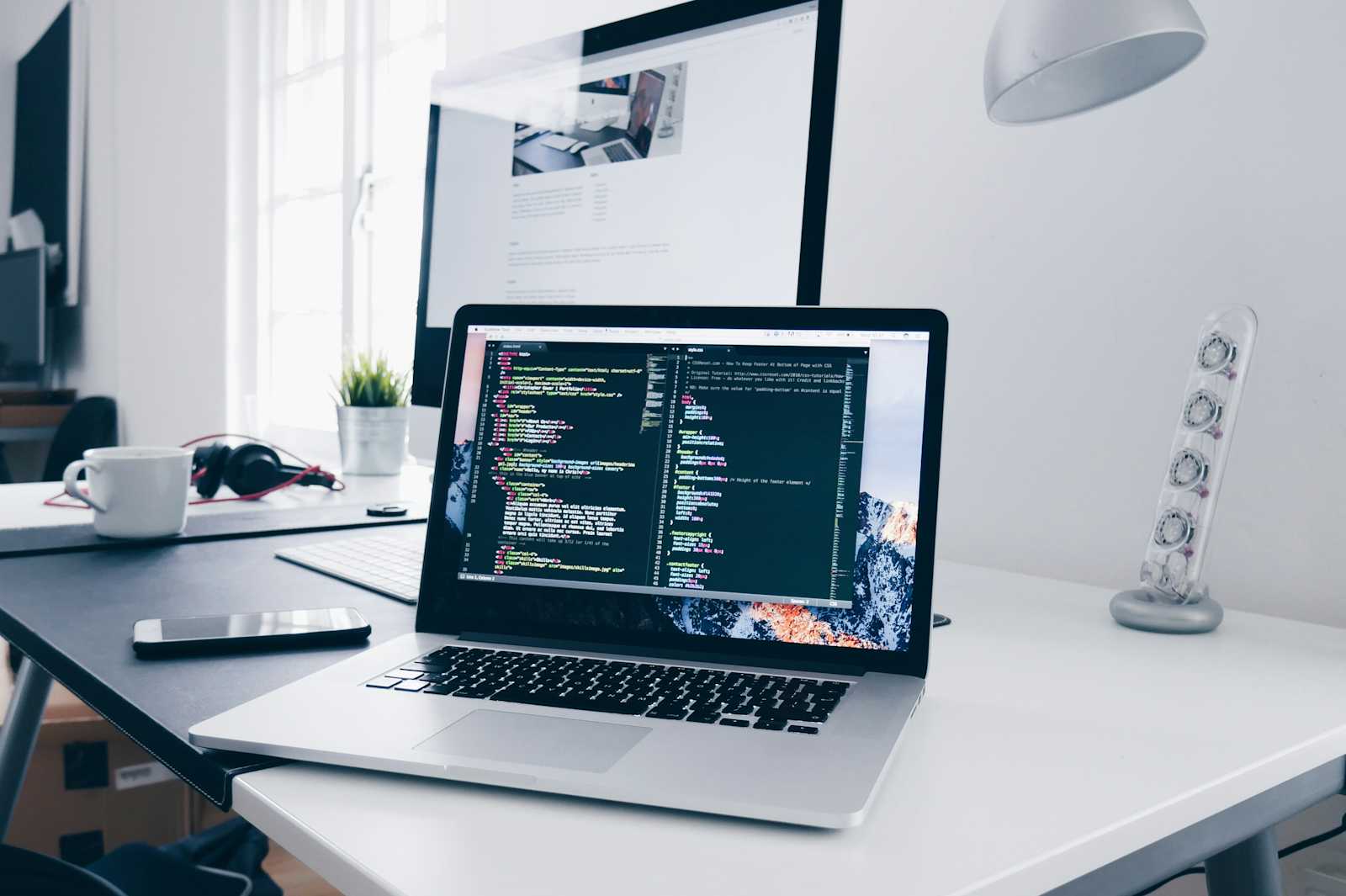
Embarking on a journey with Command Line Interface (CLI) projects can feel like venturing into uncharted territory, especially when you're weaving together Python, Object-Oriented Programming (OOP), and SQL. But fear not! This article will walk you through the essential steps to master these technologies and build a powerful, efficient CLI application.
Why Choose CLI Projects?
CLI projects provide a clean, efficient way to interact with programs. They might seem old-school in our GUI-centric world, but they hold their ground due to their simplicity and speed. Plus, they’re great for automation tasks, server management, and quick scripting.
Python: The Perfect Tool for CLI Projects
Python’s readability and extensive libraries make it an excellent choice for CLI projects. Its robust community and abundant resources mean you’re never far from a solution to any problem you encounter. Moreover, Python’s built-in modules like argparse
for parsing command-line arguments simplify creating user-friendly CLI applications.
Object-Oriented Programming: Organizing Your Code
Object-Oriented Programming (OOP) is a paradigm that uses objects and classes to structure software in a way that is modular and reusable. Here’s why OOP is a game-changer for your CLI projects:
Modularity: Break down your project into manageable chunks.
Reusability: Reuse code across different parts of your project.
Scalability: Easily add new features without breaking existing code.
With OOP, you can create classes that represent the different components of your application. For example, if you’re building a CLI tool for managing a to-do list, you might have classes like Task
, Project
, and User
.
SQL: Managing Your Data
When your CLI project needs to handle data persistently, SQL comes into play. SQL (Structured Query Language) is the standard language for managing and manipulating databases. Python’s sqlite3
library allows you to create a simple, self-contained database that integrates seamlessly with your application.
Bringing It All Together: A Simple Example
Let’s build a simple CLI to-do list manager to illustrate how Python, OOP, and SQL can work together.
Step 1: Setting Up the Project
First, set up your project structure:
todo_cli/
│
├── todo.py
├── models.py
└── database.py
Step 2: Defining the Database
Create database.py
to handle database operations:
import sqlite3
def connect():
return sqlite3.connect('todo.db')
def create_table():
with connect() as conn:
conn.execute('''
CREATE TABLE IF NOT EXISTS tasks (
id INTEGER PRIMARY KEY,
title TEXT NOT NULL,
description TEXT,
completed BOOLEAN NOT NULL CHECK (completed IN (0, 1))
)
''')
create_table()
Step 3: Creating the Models
Define your task model in models.py
:
class Task:
def __init__(self, title, description=''):
self.title = title
self.description = description
self.completed = False
Step 4: Building the CLI
In todo.py
, use argparse
to handle command-line arguments and interact with the database:
import argparse
from models import Task
from database import connect
def add_task(title, description):
with connect() as conn:
conn.execute('INSERT INTO tasks (title, description, completed) VALUES (?, ?, ?)', (title, description, False))
print(f"Task '{title}' added.")
def list_tasks():
with connect() as conn:
cursor = conn.execute('SELECT id, title, description, completed FROM tasks')
for row in cursor:
status = 'Done' if row[3] else 'Pending'
print(f"{row[0]}. {row[1]} - {row[2]} [{status}]")
if __name__ == '__main__':
parser = argparse.ArgumentParser(description='To-Do List CLI')
subparsers = parser.add_subparsers(dest='command')
add_parser = subparsers.add_parser('add', help='Add a new task')
add_parser.add_argument('title', type=str, help='Title of the task')
add_parser.add_argument('description', type=str, nargs='?', default='', help='Description of the task')
list_parser = subparsers.add_parser('list', help='List all tasks')
args = parser.parse_args()
if args.command == 'add':
add_task(args.title, args.description)
elif args.command == 'list':
list_tasks()
Conclusion
Congratulations! You've just built a simple, yet powerful, CLI application using Python, OOP, and SQL. This project is a stepping stone to more complex applications, providing a solid foundation to expand your skills. With Python’s versatility, OOP’s organizational strength, and SQL’s data management capabilities, your CLI projects can handle a wide range of tasks efficiently and effectively.
Happy coding!
Subscribe to my newsletter
Read articles from Dan Koskei directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
