Unlocking the Secrets: Counting Digits in an Integer Made Easy

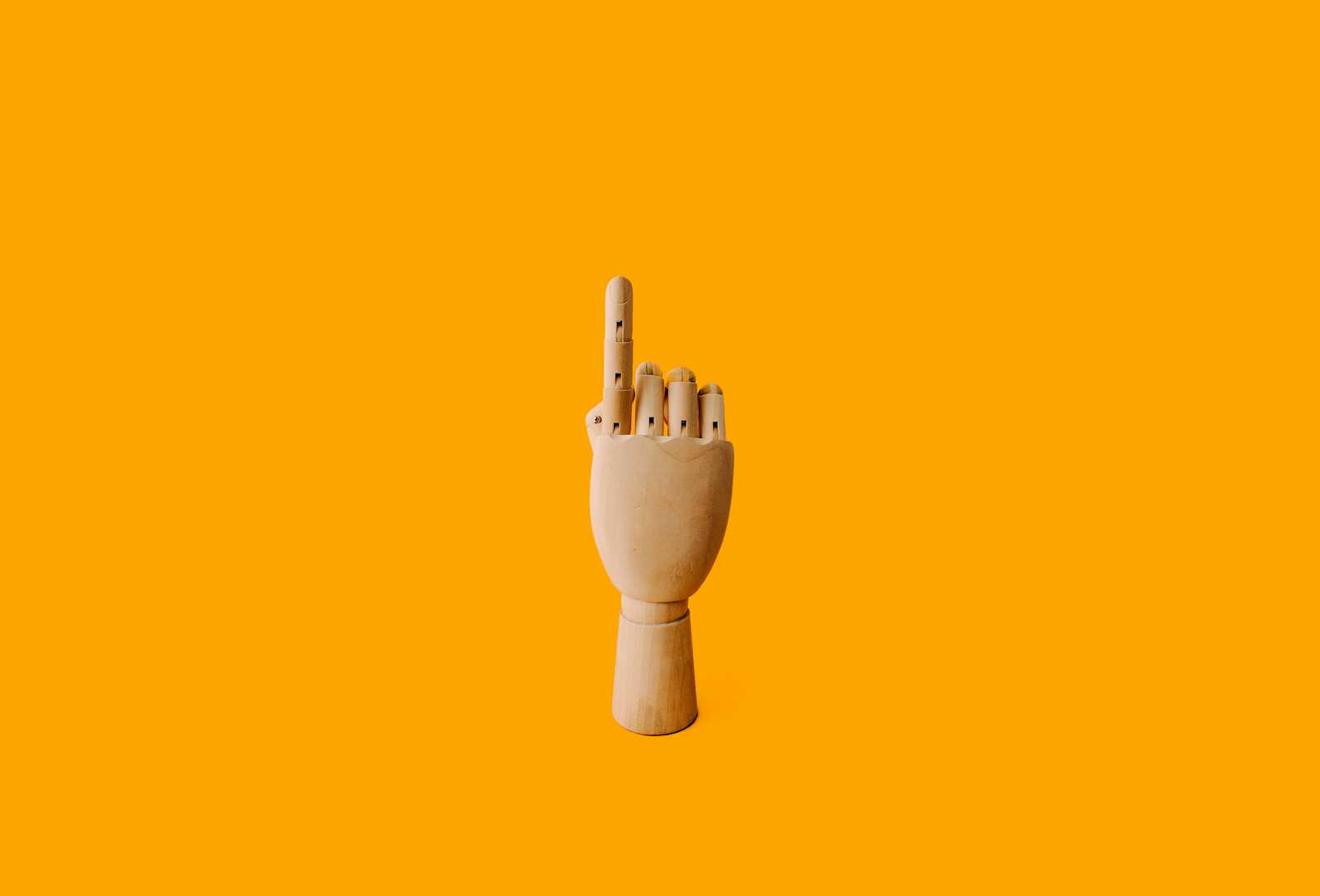
Hello coders! In this article, I will explain how to count the number of digits in an integer. We will start with a simple loop method and then explore a faster technique using logarithms. Let's dive in and make counting digits easy and efficient!
Before we dive in, let's first understand what a logarithm is.
๐ What is a Logarithm?
Imagine you have a secret treasure chest, and you want to keep track of how many times you need to multiply a certain number to reach the treasure amount. Letโs say you multiply 2 by itself, and then by itself again, and again until you reach 16. You find that you need to multiply 2 by itself 4 times to get to 16 (because 2 x 2 x 2 x 2 = 16).
The number of times you multiply 2 (4 times in this case) is the "logarithm" of 16 with base 2. So, a logarithm helps you figure out "how many times" you need to multiply a certain number to get a certain amount.
For example:
- To get 64, you need to multiply 2 by itself 6 times (because 2 x 2 x 2 x 2 x 2 x 2 = 64), so the logarithm of 64 with base 2 is 6.
This is the idea behind using logarithms to count steps in reaching a number!
๐ป Method 1: Using a Loop
The straightforward way to count the digits of a number is to repeatedly divide the number by 10 until it becomes 0. Each division reduces the number of digits by one.
def count_digits_loop(n):
if n == 0:
return 1
count = 0
while n != 0:
n //= 10
count += 1
return count
# Example usage:
number = 12345
print(count_digits_loop(number)) # Output: 5
๐ How It Works:
Initialization: Start with
count
set to 0.Division and Counting: In each iteration of the loop, divide
n
by 10 and incrementcount
.Termination: The loop continues until
n
becomes 0.Special Case: If
n
is 0, it directly returns 1, since 0 has 1 digit.
๐ Another Example with Loop
Let's try another example to make it clearer:
number = 789
print(count_digits_loop(number)) # Output: 3
Hereโs what happens:
789 // 10 = 78
(count = 1)78 // 10 = 7
(count = 2)7 // 10 = 0
(count = 3)Final count is 3, so the number 789 has 3 digits.
๐ Method 2: Using Logarithms
A more efficient way to count the digits is to use logarithms. The base-10 logarithm of a number gives the power to which 10 must be raised to get that number. By taking the floor of the logarithm and adding 1, we get the number of digits.
import math
def count_digits_log(n):
if n == 0:
return 1
return int(math.log10(n)) + 1
# Example usage:
number = 12345
print(count_digits_log(number)) # Output: 5
๐ How It Works:
Logarithm Calculation:
math.log10(n)
computes the base-10 logarithm ofn
.Floor and Increment:
int(math.log10(n))
takes the floor of the logarithm, effectively giving the largest integer less than or equal to the logarithm value.Adding One: Adding 1 accounts for the zero-indexing of logarithms.
Special Case: If
n
is 0, it directly returns 1, since 0 has 1 digit andlog10(0)
is undefined.
๐ Another Example with Logarithm
Let's try another example to make it clearer:
number = 789
print(count_digits_log(number)) # Output: 3
Hereโs what happens:
log10(789) โ 2.897
int(2.897) = 2
Adding 1 gives
2 + 1 = 3
The number 789 has 3 digits.
๐ Comparison
Loop Method: Simple and easy to understand. However, it may be slower for very large numbers.
Logarithm Method: More efficient, especially for large numbers, since it reduces the problem to a single mathematical operation.
๐ Conclusion
In conclusion, counting the digits in an integer can be achieved through both simple and advanced techniques. The loop method offers simplicity and ease of understanding, making it ideal for beginners and small numbers. On the other hand, the logarithm method provides a more efficient solution for larger numbers, leveraging mathematical operations to expedite the process. Depending on your needs and the size of the numbers you are working with, you can choose the method that best fits your requirements. Experiment with both approaches in your coding projects to gain a deeper understanding and see the performance differences firsthand. Happy coding!
Subscribe to my newsletter
Read articles from RITIK Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
