Mastering Python Lambda Expressions: A Guide with Examples

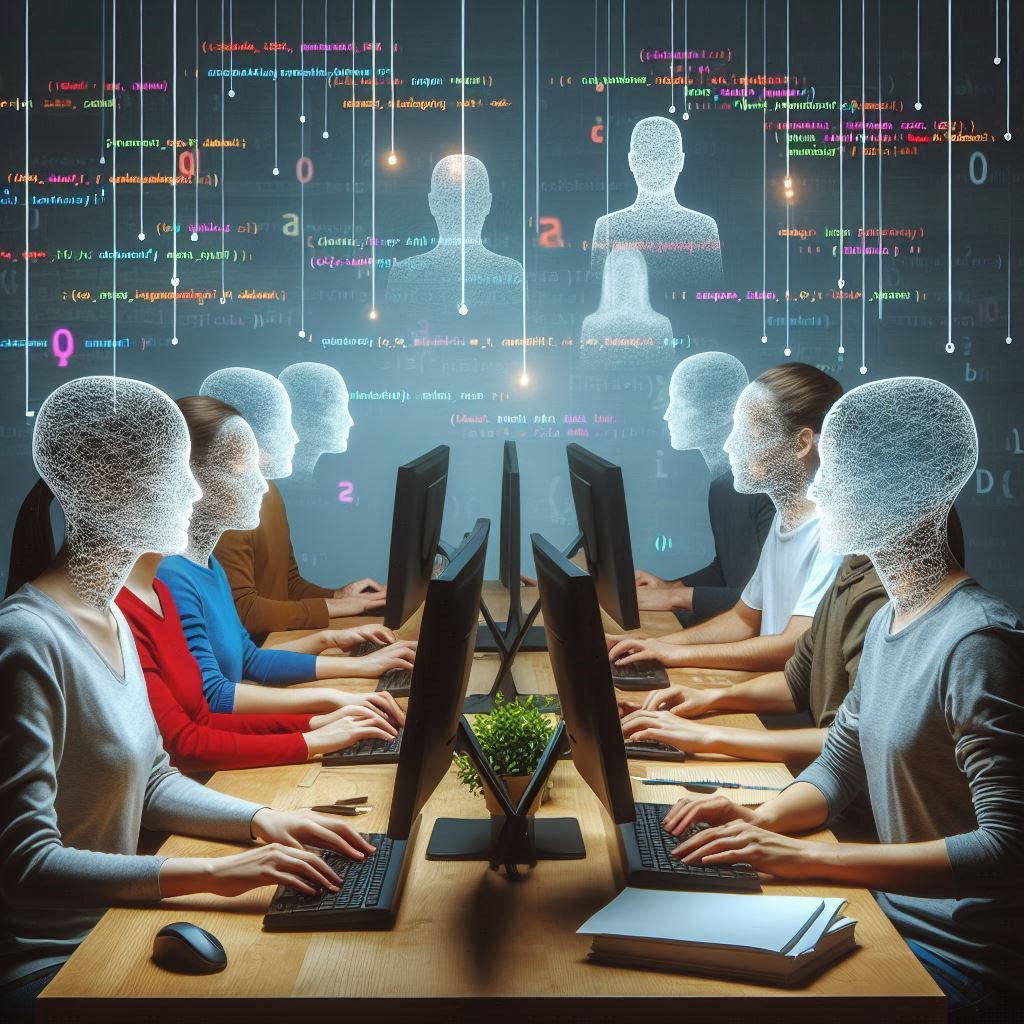
Python is a versatile programming language known for its readability and simplicity. One of its powerful features is the lambda function, an anonymous function expressed as a single statement. In this blog post, we'll delve into what lambda functions are, explore their usefulness, and demonstrate their application through several examples.
What is a Lambda Function?
A lambda function in Python is a small, anonymous function defined with the lambda
keyword. Unlike regular functions defined using def
, a lambda function can have any number of arguments but only one expression. The expression is evaluated and returned. Lambda functions are particularly useful in scenarios where a simple function is needed temporarily, often as an argument to higher-order functions like map()
, filter()
, and reduce()
.
Syntax
The basic syntax of a lambda function is:
lambda arguments: expression
Example 1: Filtering Data
In this example, we use a lambda function to filter out integers from a list that are greater than the average integer value of the list.
#Create a list
data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
#Find the average of the list
avg = sum(data) / len(data) #Output: 5.5
#Applies the filter function to each element in the list.
#If x is greater than the average it will be included in the result.
result = list(filter(lambda x: x > avg, data))
print(result)
Output:
[6, 7, 8, 9, 10]
In-Depth Explanation of the Output
The list
data
contains integers from 1 to 10.The average value of the list is
5.5
.The
filter()
function, combined with the lambda functionlambda x: x > avg
, checks each element ofdata
to see if it is greater than5.5
.1
is not greater than5.5
(excluded)2
is not greater than5.5
(excluded)3
is not greater than5.5
(excluded)4
is not greater than5.5
(excluded)5
is not greater than5.5
(excluded)6
is greater than5.5
(included)7
is greater than5.5
(included)8
is greater than5.5
(included)9
is greater than5.5
(included)10
is greater than5.5
(included)
Therefore, the resulting list is
[6, 7, 8, 9, 10]
.
Example 2: String Multiplication
In this example, we will use a lambda function to create a custom function that repeats a word a specified number of times.
echo_word = (lambda word1, echo: word1 * echo)
result = echo_word('hey', 5)
print(result)
Output:
heyheyheyheyhey
In-Depth Explanation of the Output
The lambda function
lambda word1, echo: word1 * echo
is defined to take two arguments and return the first argument repeated the number of times specified by the second argument.When
echo_word('hey', 5)
is called:word1
is'hey'
echo
is5
The expression
'hey' * 5
is evaluated.In Python, multiplying a string by an integer repeats the string that many times.
Therefore,
'hey' * 5
results in'heyheyheyheyhey'
.
The resulting string is stored in the variable
result
.Finally,
print(result)
outputs the repeated string'heyheyheyheyhey'
.
Example 3: Modifying List Elements
Here, we use a lambda function to add "!!!" to each item in the list of spells.
spells = ['expecto patronum', 'avada kedavra', 'wingardium leviosa']
shout_spells = list(map(lambda item: item + '!!!', spells))
print(shout_spells)
Output:
['expecto patronum!!!', 'avada kedavra!!!', 'wingardium leviosa!!!']
In-Depth Explanation of the Output
Original List:
['expecto patronum', 'avada kedavra', 'wingardium leviosa']
- The
spells
list contains three spell names.
- The
Lambda Function:
lambda item: item + '!!!'
- This lambda function is designed to take a single input (
item
) and append "!!!" to it.
- This lambda function is designed to take a single input (
Map Function:
map(lambda item: item + '!!!', spells)
The
map
function applies the lambda function to each item in thespells
list:'expecto patronum'
becomes'expecto patronum!!!'
'avada kedavra'
becomes'avada kedavra!!!'
'wingardium leviosa'
becomes'wingardium leviosa!!!'
Converting to List:
list(map(...))
- The map object is converted to a list, resulting in
['expecto patronum!!!', 'avada kedavra!!!', 'wingardium leviosa!!!']
.
- The map object is converted to a list, resulting in
Print Statement:
print(shout_spells)
- The final list is printed, showing each spell with "!!!" appended to it.
Example 4: Reducing a List to a Single String
In this example, the reduce()
function is used with a lambda function to combine all elements of a list into a single string.
from functools import reduce
stark = ['Eddard', 'Catelyn', 'Robb', 'Sansa', 'Arya', 'Bran', 'Rickon']
result = reduce(lambda item1, item2: item1 + item2, stark)
print(result)
Output:
EddardCatelynRobbSansaAryaBranRickon
In-Depth Explanation of the Output
Original List:
['Eddard', 'Catelyn', 'Robb', 'Sansa', 'Arya', 'Bran', 'Rickon']
- The
stark
list contains the names of the Stark family members.
- The
Lambda Function:
lambda item1, item2: item1 + item2
- This lambda function concatenates two strings.
Reduce Function:
reduce(lambda item1, item2: item1 + item2, stark)
The
reduce
function applies the lambda function to the list elements cumulatively:'Eddard'
+'Catelyn'
->'EddardCatelyn'
'EddardCatelyn'
+'Robb'
->'EddardCatelynRobb'
'EddardCatelynRobb'
+'Sansa'
->'EddardCatelynRobbSansa'
'EddardCatelynRobbSansa'
+'Arya'
->'EddardCatelynRobbSansaArya'
'EddardCatelynRobbSansaArya'
+'Bran'
->'EddardCatelynRobbSansaAryaBran'
'EddardCatelynRobbSansaAryaBran'
+'Rickon'
->'EddardCatelynRobbSansaAryaBranRickon'
The final result is the concatenated string
'EddardCatelynRobbSansaAryaBranRickon'
.
Conclusion
Lambda functions are a powerful feature in Python that can make your code more concise and readable, especially when working with higher-order functions like map()
, filter()
, and reduce()
. By understanding and utilizing lambda functions, you can write more efficient and expressive code. Whether you're filtering data, modifying list elements, or reducing lists, lambda functions provide a compact way to perform these operations. Mastering lambda expressions will undoubtedly enhance your Python programming skills and allow you to handle functional programming tasks with ease.
Subscribe to my newsletter
Read articles from Emeron Marcelle directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Emeron Marcelle
Emeron Marcelle
As a doctoral scholar in Information Technology, I am deeply immersed in the world of artificial intelligence, with a specific focus on advancing the field. Fueled by a strong passion for Machine Learning and Artificial Intelligence, I am dedicated to acquiring the skills necessary to drive growth and innovation in this dynamic field. With a commitment to continuous learning and a desire to contribute innovative ideas, I am on a path to make meaningful contributions to the ever-evolving landscape of Machine Learning.