Why developers should use Node.js

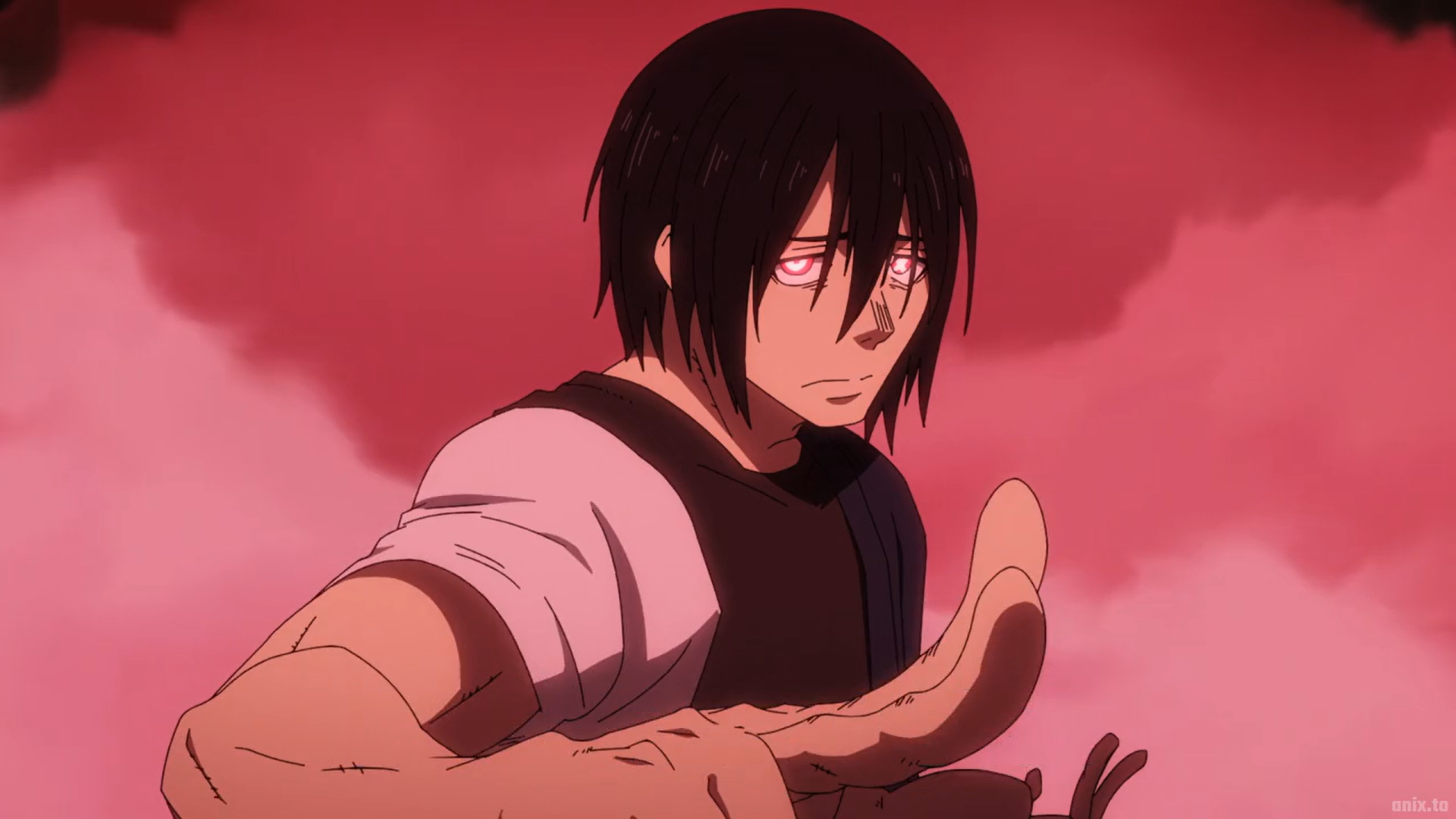
[14]
Introduction
Node.js is a cross-platform runtime environment and library for running JavaScript applications outside the browser. It is used for creating server-side and networking web applications. It is open source and free to use. Many of the basic modules of Node.js are written in JavaScript itself. Node.js is mostly used to run real-time server applications. The official definition goes like "Node.js is a platform built on chrome's JavaScript runtime for easily building fast and scalable network applications. Node.js uses an event-driven, non-blocking I/O model that makes it lightweight and efficient for data-intensive real-time applications that run across distributed devices." Node.js also provides a rich library of various JavaScript modules to simplify the development of Web applications.
Node.js = Runtime Environment + JavaScript Library
Features of Node.js
Following is a list of some of the important features of Node.js that makes it the first choice for software architects.
Extremely fast - Node.js is built on Google Chrome's V8 Engine so it's library is very fast in code execution.
I/O is asynchronous and Event Driven - All APIs of Node.js library are asynchronous i.e., non-blocking. So, a Node.js based server never waits for an API to return data. The server moves to the next API after calling it and a notification mechanism of events of Node.js helps the server to get a response from the previous API call. This is the reason why Node.js is very fast in execution.
Single Threaded - Node.js follows a single threaded model with event looping.
Highly Scalable - Node.js is highly scalable because event mechanism helps the server to respond in a non-blocking way.
No Buffering - Node.js just cuts down the overall processing time while uploading audio and video files (mind you, even though I love Node.js I still don't recommend using it for uploading files that are huge in size for instance files that are > 10 GB). Node.js applications tend not to buffer any data and they simply output the data in chunks.
Open Source - It has an open source community which has produced many excellent modules to add additional capabilities to Node.js applications.
License - Node.js was released under the MIT License.
Node.js Web-based Example
A Node.js web application contains the following three parts:
Importing required modules - The
require
directive is used to load a Node.js module.Create server - You have to establish a server which will listen to client's request similar to Apache HTTP Server.
Read request and return response - Server created in the second step will read HTTP request made by client which can be a browser or console and return the response.
Creating a Node.js Web App
To create a web app, we need to follow the below the mentioned steps:
- The first step is to use
require
directive to load thehttp
module and store the returned HTTP instance into thehttp
variable.
var http = require('http')
- For the second step, you use the created
http
instance and call thehttp.createServer()
method to create server instance and then bind it at port 8081 using thelisten
method associated with the server instance.
var http = require('http')
// Send the HTTP header
// HTTP Status: 200 : OK
http.createServer(function(request, response) {
response.writeHead(200, {'Content-Type': 'text/plain'})
response.end('Hello World\n')
}).listen(8081)
// Printing the message to the console
console.log('Server running at http://127.0.0.1:8081/')
Node.js Console
The Node.js console module provides a simple debugging console similar to JavaScript console mechanism provided by web browsers. There are three console methods that are used to write and stream:
console.log() - This function is used to display any simple message on the console.
console.error() - This function is used to render a error message on the console.
console.warn() - This function is used to display any warning message on the console.
Node.js REPL
The term REPL stands for Read Eval Print and Loop. It specifies a computer environment like a windows console or a Unix/Linux Shell where you can enter the commands and the system responds with an output in an interactive mode.
REPL Environment
The Node.js or node comes bundled with REPL environment where each part of the REPL environment has a specific work.
Read - It reads user's input; parses the input into JavaScript data-structure and stores in memory.
Eval - It takes and evaluates the data structure.
Print - It prints the result.
Loop - It loops the above command until user presses ctrl + c twice.
Node.js Package Manager
Node Package Manager provides two main functionalities:
It provides online repositories for Node.js packages/modules which are searchable on search.nodejs.org.
It also provides command line utility to install Node.js packages, do version management and dependency management of Node.js packages. The
npm
comes bundled with Node.js installables in version after0.6.3
. You can also check the version by opening Node.js command prompt and typing the following command:
npm version
To install a module using npm
:
npm install <module_name>
To uninstall a module using npm
:
npm uninstall <module_name>
Final Notes
Node.js is a powerful tool for building web applications. It lets developers use JavaScript on both the server and client-side. Node.js excels at real-time applications due to its efficient handling of multiple requests simultaneously. With a vast library of tools and constant updates, Node.js is a strong choice for anyone looking to build modern web applications with JavaScript.
If you found this post informative, please hit that like button! It helps spread the knowledge.
Subscribe to my newsletter
Read articles from Pranav Bawgikar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pranav Bawgikar
Pranav Bawgikar
Hiya ๐ I'm Pranav. I'm a recent computer science grad who loves punching keys, napping while coding and lifting weights. This space is a collection of my journey of active learning from blogs, books and papers.