Big O Notation: A Practical Guide for Beginners
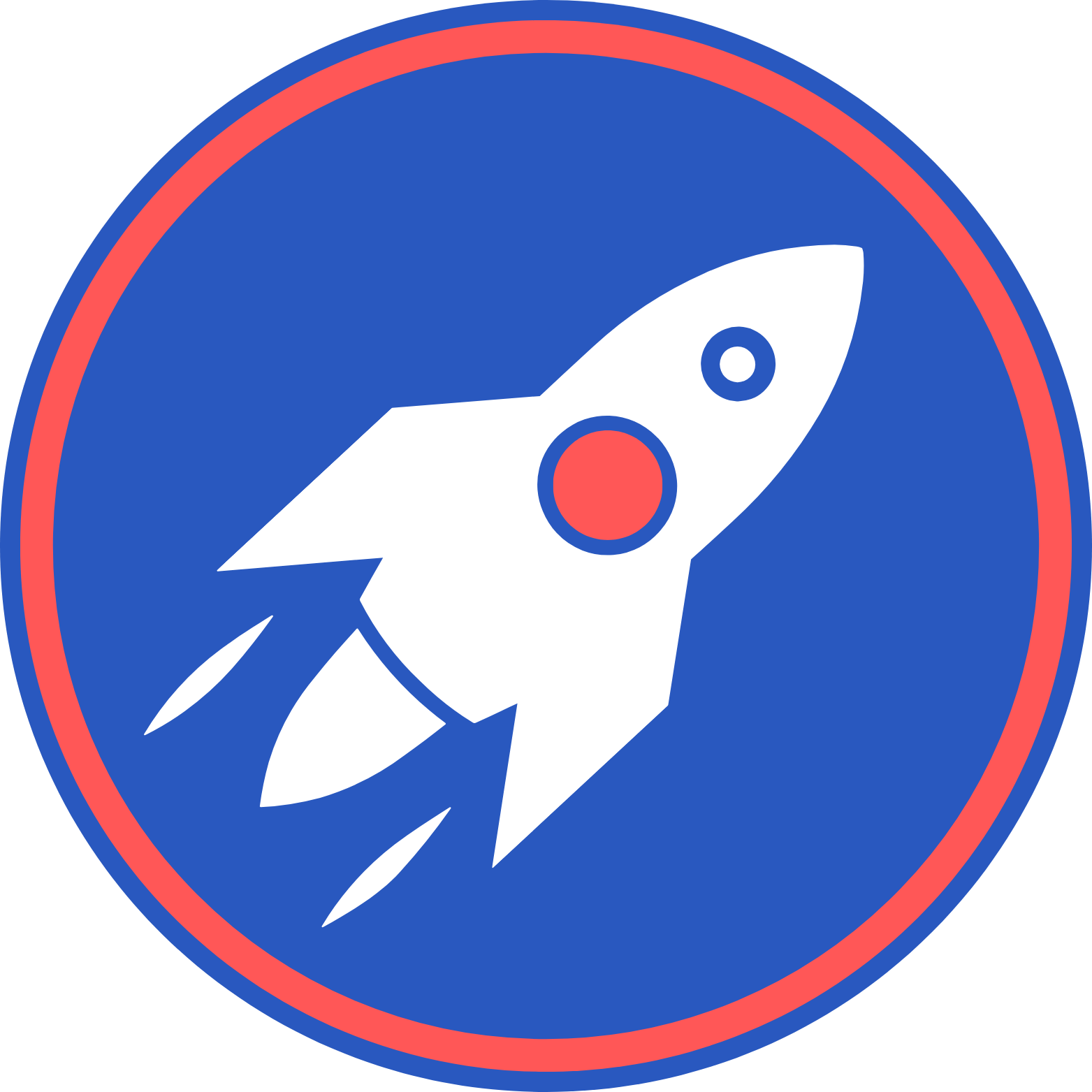
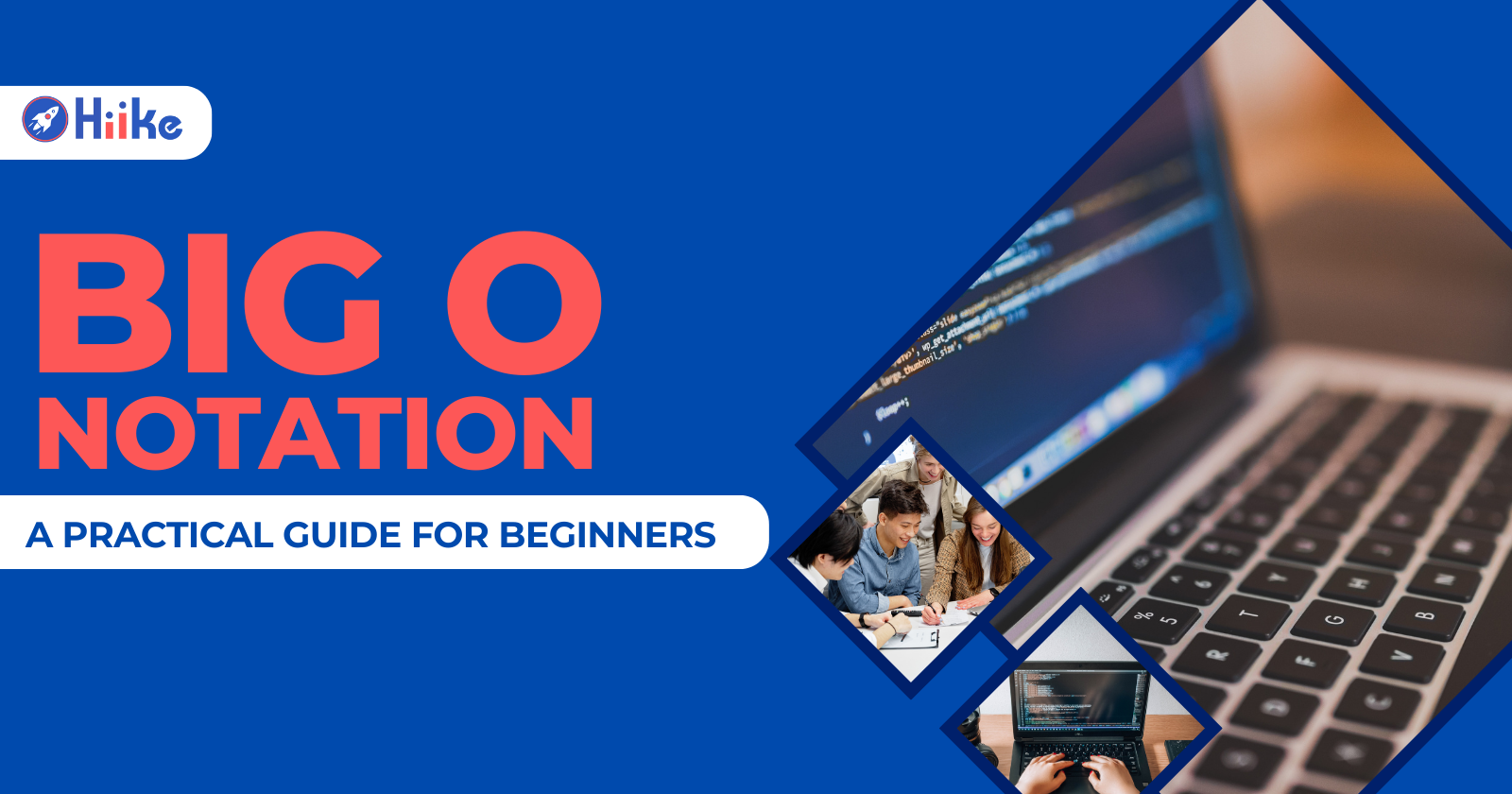
Understanding the efficiency of algorithms is crucial for software developers, and Big O notation is the standard mathematical language used to describe the performance of algorithms. This guide will break down the basics of Big O notation, provide practical examples, and offer tips to help you grasp this fundamental concept.
Explanation of Big O Notation
Big O notation is a way to describe the efficiency of an algorithm in terms of time (time complexity) and space (space complexity). It focuses on the worst-case scenario, providing an upper bound on the running time or memory usage of an algorithm as the input size grows.
Key Concepts:
Time Complexity: Measures the amount of time an algorithm takes to complete as a function of the input size (n).
Space Complexity: Measures the amount of memory an algorithm uses as a function of the input size (n).
Common Big O Notations:
O(1): Constant time – The algorithm's performance is independent of the input size.
O(log n): Logarithmic time – The algorithm's performance grows logarithmically with the input size.
O(n): Linear time – The algorithm's performance grows linearly with the input size.
O(n log n): Linearithmic time – The algorithm's performance grows in a combination of linear and logarithmic time.
O(n^2): Quadratic time – The algorithm's performance grows quadratically with the input size.
Practical Examples and Exercises
Let's look at some practical examples to illustrate these concepts.
Example 1: Constant Time – O(1)
A function that retrieves the first element of an array operates in constant time:
def get_first_element(arr):
return arr[0]
Example 2: Linear Time – O(n)
A function that sums all elements of an array operates in linear time:
def sum_elements(arr):
total = 0
for num in arr:
total += num
return total
Example 3: Quadratic Time – O(n^2)
A function that checks for duplicates in an array using two nested loops operates in quadratic time:
def has_duplicates(arr):
for i in range(len(arr)):
for j in range(i + 1, len(arr)):
if arr[i] == arr[j]:
return True
return False
Comparison of Time Complexities
Understanding how different time complexities compare can help you choose the most efficient algorithm for a given problem.
Complexity | Name | Example |
O(1) | Constant | Accessing an element in an array |
O(log n) | Logarithmic | Binary search |
O(n) | Linear | Finding an item in an unsorted list |
O(n log n) | Linearithmic | Merge sort, Quick sort |
O(n^2) | Quadratic | Bubble sort, Selection sort |
Common Algorithms and Their Time Complexities
Here are some common algorithms and their associated time complexities:
Binary Search: O(log n)
Merge Sort: O(n log n)
Quick Sort: O(n log n)
Bubble Sort: O(n^2)
Insertion Sort: O(n^2)
Fibonacci (Recursive): O(2^n)
Fibonacci (Dynamic Programming): O(n)
Tips for Understanding and Using Big O Notation
Focus on the Dominant Term: When analyzing an algorithm, focus on the term that grows the fastest as the input size increases. For example, in O(n + n^2), the dominant term is n^2, so the complexity is O(n^2).
Drop Constants and Lower Order Terms: Big O notation describes the asymptotic behavior, so constants and non-dominant terms are not considered. For instance, O(3n) simplifies to O(n).
Analyze Worst-Case Scenario: Big O notation typically describes the worst-case scenario, giving an upper bound on the time or space required.
Practice, Practice, Practice: The best way to get comfortable with Big O notation is through practice. Analyze different algorithms, compare their efficiencies, and solve related problems.
Conclusion
Mastering Big O notation is essential for anyone serious about software development. It provides a framework for analyzing and comparing the efficiency of algorithms, helping you make informed decisions about which algorithms to use in different scenarios.
At Hiike, we understand the importance of these foundational concepts. Our Top 30 Program is designed to equip you with the skills and knowledge needed to excel in the tech industry. Through comprehensive courses in Data Structures, Algorithms, and System Design, we ensure that you are thoroughly prepared for the challenges of technical interviews and real-world problem-solving. Join Hiike today and take the first step towards mastering data structures, algorithms, and system design!
Subscribe to my newsletter
Read articles from Hiike directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
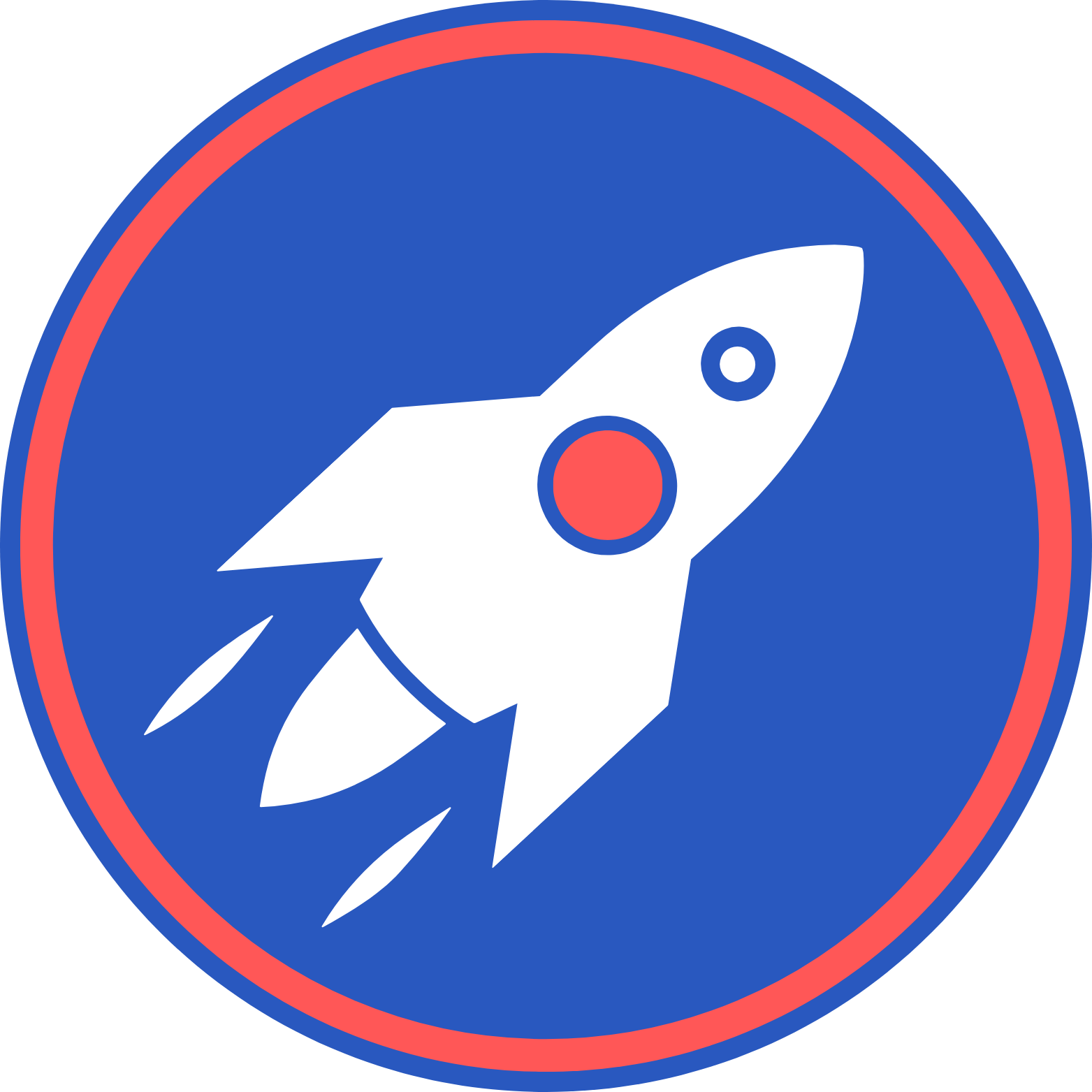
Hiike
Hiike
🌟𝐇𝐢𝐢𝐤𝐞 - Crafting Your Tech Odyssey! 🚀 Join Hiike for immersive learning, expert mentorship, and accelerated career growth. Our IITian-led courses and vibrant community ensure you excel in Data Structures, Algorithms, and System Design. Transform your tech career with Hiike today!