Python's Turtle: A Place where Creativity meets Code
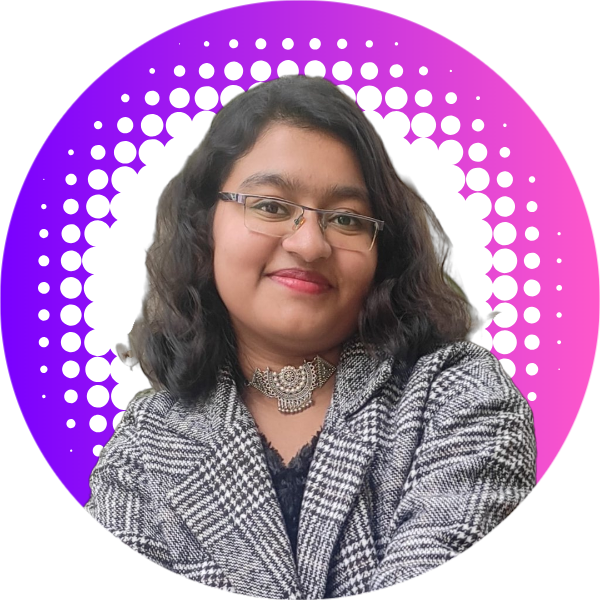
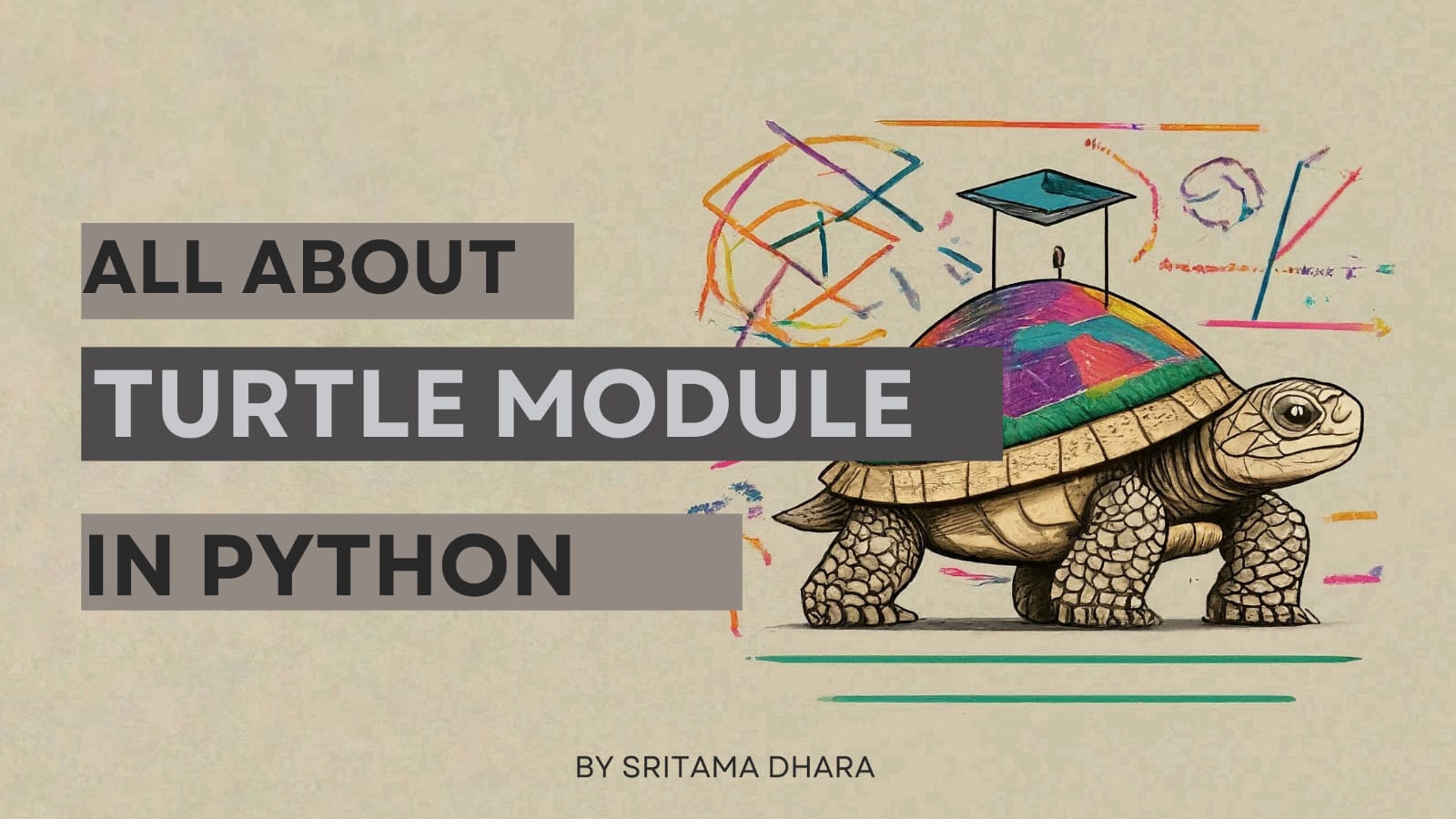
What is Turtle?
Do you remember MS LOGO from your childhood? Turtle is almost the same!
Turtle is a pre-installed Python library that enables users to create a variety of shapes and patterns, just through the use of simple commands. Imagine Turtle as a playful pet turtle with a pen attached to it's shell. This spunky little artist scoots around a virtual canvas, leaving a trail of vibrant lies and intricate shapes. Turtle graphics is particularly useful for beginners as it is fun, interactive and provide instant visual feedbacks.
Getting Started
Installing Turtle
The beauty of Turtle is that it comes pre-installed in most Python environments. If you have Python installed in your system, you're ready to embark on your colourful journey.
Importing Turtle
To use the Turtle module, you need to import it into your Python script. Here’s how you can do it:
import turtle
Creating a Turtle Object
To unleash your potentials, you'll need a trusty companion: the Turtle itself. In order to create your own pet artist, you need to create an object, that will be doing all the drawings.
my_turtle = turtle.Turtle()
Basic Turtle Commands
Method | Description | Example |
forward() or fd() | Moves the turtle forward by the specified distance | my_turtle.fd(100) |
backward() or bk() | Moves the turtle backwards by specified distance | my_turtle.bk(100) |
right() or rt() | Turns the turtle clockwise through a specific angle | my_turtle.rt(90) |
left() or lt() | Turns the turtle anticlockwise through a specific angle | my_turtle.lt(90) |
penup() | Picks up the turtle's pen | my_turtle.penup() |
pendown() | Puts down the turtle's pen | my_turtle.pendown() |
goto() | Moves the turtle to a specific coordinate(x,y) | my_turtle.goto(70,50) |
home() | Returns turtle to starting position(0,0) | my_turtle.home() |
color() | Changes the colour of the turtle's pen | my_turtle.color('blue') |
fillcolor() | Specifies the colour to fill a polygon | my_turtle.fillcolor('red') |
begin_fill() | Specifies the beginning position to fill a polygon | my_turtle.begin_fill() |
end_fill() | Specifies the end position after filling a polygon | my_turtle.end_fill() |
stamp() | Leaves a stamp of the turtle at the current location | my_turtle.stamp() |
clear() | Clears the screen but leaves the turtle properties the same | my_turtle.clear() |
reset() | Resets everything including the turtle's properties | my_turtle.reset() |
How to Draw Two Parallel Lines
import turtle
t = turtle.Turtle()
t.forward(150)
t.right(90)
t.penup()
t.forward(50)
t.right(90)
t.pendown()
t.forward(150)
How to Draw a Square
import turtle
t = turtle.Turtle()
t.forward(100)
t.right(90)
t.forward(100)
t.right(90)
t.forward(100)
t.right(90)
t.forward(100)
t.right(90)
or by using a loop
import turtle
t = turtle.Turtle()
for i in range(4):
t.forward(100)
t.right(90)
range() can be used to draw polygons with any number of sides. Just specify the number of sides within the range and change the right() or left() angle accordingly.
How to Draw a Star
import turtle
t = turtle.Turtle()
for i in range(5):
t.forward(100)
t.right(144)
How to Fill a Shape
import turtle
t = turtle.Turtle()
t.color('red')
for i in range(6):
t.forward(100)
t.right(60)
t.fillcolor('yellow')
t.begin_fill()
for j in range(6):
t.forward(100)
t.right(60)
t.end_fill()
How to Draw a Circle
import turtle
t = turtle.Turtle()
t.circle(50) #specify the diameter of the circle
How to Draw a Spiral
import turtle
t = turtle.Turtle()
t.speed(10) #specifies the speed of the turtle
for i in range(100):
t.forward(i * 3)
t.right(90)
Conclusion
Turtle graphics is a wonderful way to get started with programming. It combines creativity with coding, making the learning process enjoyable and visually rewarding. As you become more comfortable with Turtle, you can explore its advanced features to create stunning and complex graphics.
For more information, visit: https://docs.python.org/3/library/turtle.html
Thank you for reading this blog. Hope you got to learn something new today! Have a wonderful day ahead!
Subscribe to my newsletter
Read articles from Sritama Dhara directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
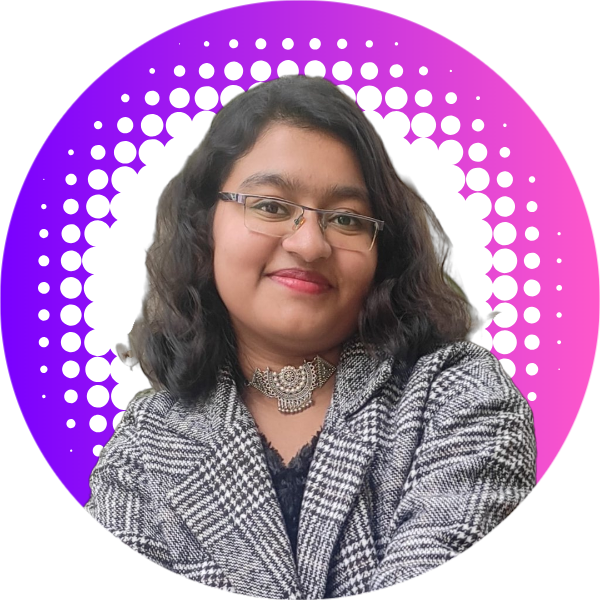
Sritama Dhara
Sritama Dhara
Student, currently pursuing B.Tech in Computer Science and Engineering