Building a React To-Do App with Add, Edit, Delete, and Enter Key Features
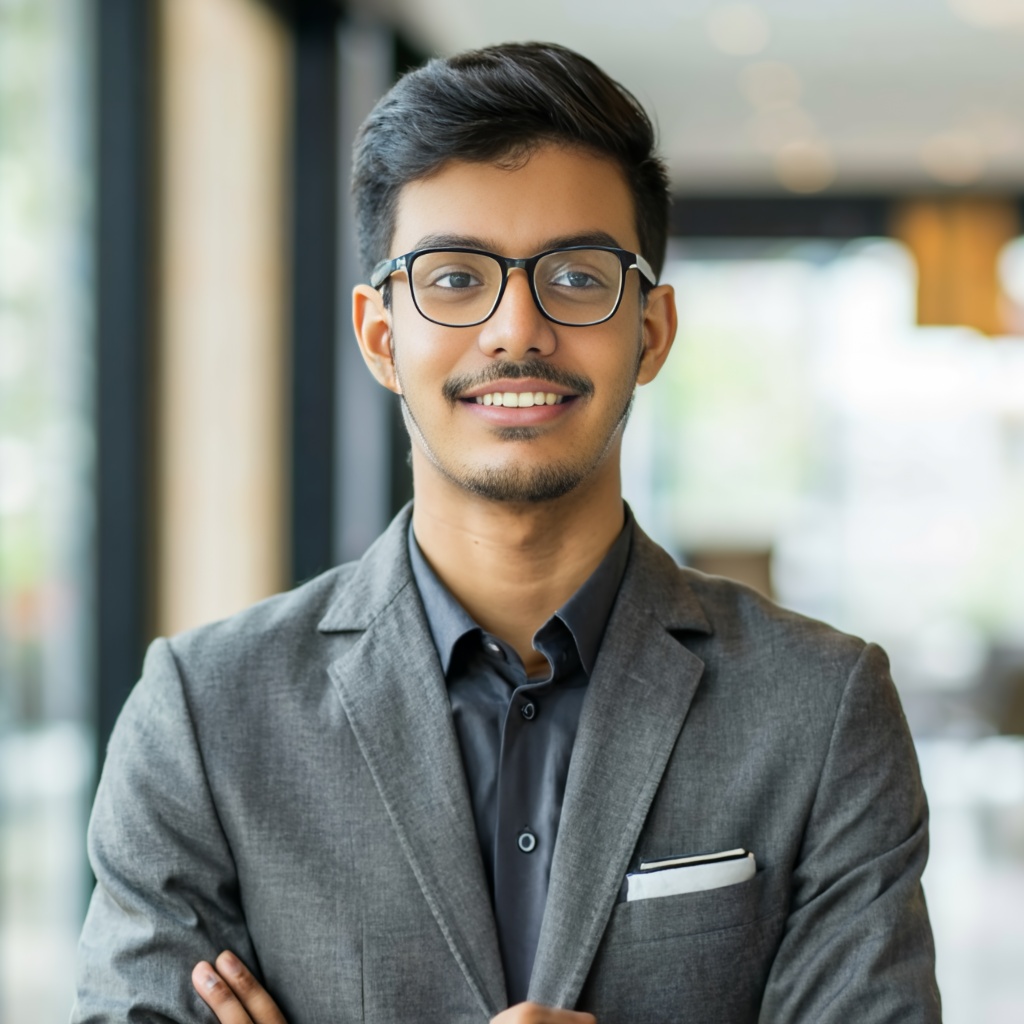
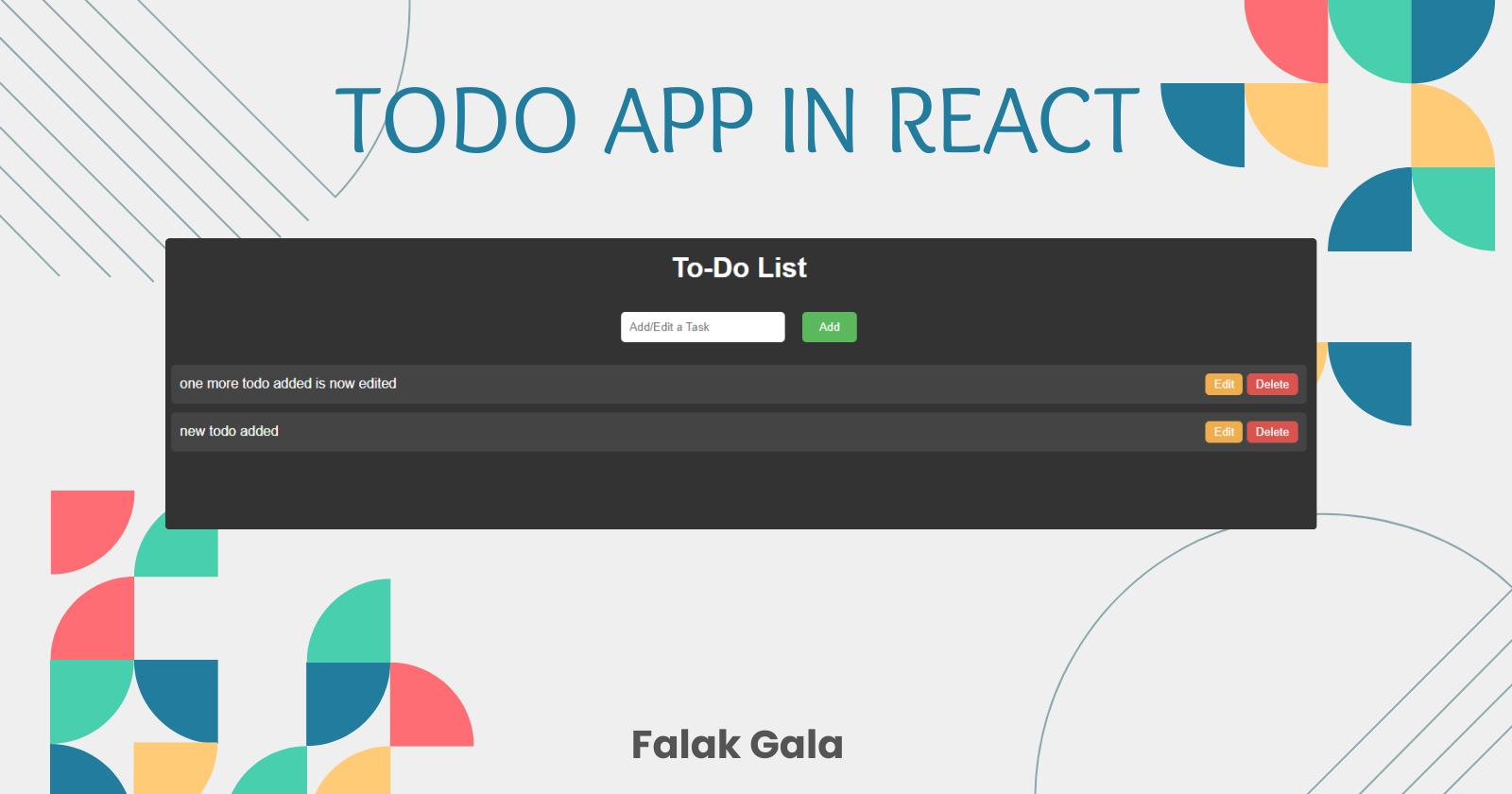
In this blog post, we will walk through the creation of a simple yet functional To-Do app using React. This app will include features for adding, editing, and deleting tasks, with new tasks appearing at the top of the list. Additionally, we will implement functionality to add or edit tasks by pressing the Enter key.
Step 1: Setup and Initial State
First, set up the React component and initialize the state. We need to import useState
from React and create the initial state for tasks, the new task input, and the task being edited.
import { useState } from 'react';
const App = () => {
const [tasks, setTasks] = useState([]); // State to manage the list of tasks
const [newTask, setNewTask] = useState(''); // State to manage the input value for new or edited tasks
const [editedTask, setEditedTask] = useState(null); // State to track which task is being edited (null means no task is being edited)
Step 2: Adding and Editing Tasks
Create a function to handle adding new tasks or editing existing ones. If a task is being edited, update the existing task. Otherwise, add the new task to the top of the list.
// Function to handle adding or editing a task
const handleAddTask = () => {
if (newTask.trim() !== '') {
if (editedTask !== null) {
const updatedTasks = [...tasks];
updatedTasks[editedTask] = {
text: newTask,
completed: tasks[editedTask].completed,
};
setTasks(updatedTasks);
setEditedTask(null);
} else {
// Adding new task to the top of the list
setTasks([{ text: newTask, completed: false }, ...tasks]);
}
setNewTask('');
}
};
Step 3: Deleting Tasks
Create a function to handle deleting a task by removing it from the tasks array based on the index.
// Function to handle deleting a task
const handleDeleteTask = (index) => {
const updatedTasks = [...tasks];
updatedTasks.splice(index, 1);
setTasks(updatedTasks);
};
Step 4: Editing Tasks
To edit a task, set the task's index in the editedTask
state and populate the input field with the task's text.
// Function to handle setting a task for editing
const handleEditTask = (index) => {
setEditedTask(index);
setNewTask(tasks[index].text);
};
Step 5: Handling Enter Key Press
Add an event listener to the input field to handle the Enter key press, which triggers the task addition or edit function.
// Function to handle Enter key press for adding/editing tasks
const handleKeyPress = (e) => {
if (e.key === 'Enter') {
handleAddTask();
}
};
Step 6: Rendering the Component
Render the component, including the input field, Add/Edit button, and the list of tasks. Apply some inline styles for a better visual presentation.
return (
<div style={styles.container}>
<h1>To-Do List</h1>
<div>
<input
type="text"
value={newTask}
placeholder="Add/Edit a Task"
onChange={(e) => setNewTask(e.target.value)}
onKeyUp={handleKeyPress} // Add event listener for key press
style={styles.input}
/>
<button type="button" onClick={handleAddTask} style={styles.button}>
{editedTask !== null ? 'Edit' : 'Add'}
</button>
</div>
<ul style={styles.list}>
{tasks.map((task, index) => (
<li
key={index}
className={task.completed ? 'completed' : ''}
style={styles.listItem}>
<span>{task.text}</span>
<div>
<button
onClick={() => handleEditTask(index)}
style={styles.editButton}>
Edit
</button>
<button
onClick={() => handleDeleteTask(index)}
style={styles.deleteButton}>
Delete
</button>
</div>
</li>
))}
</ul>
</div>
);
};
Final Output
Summary
Developed a To-Do app using React that allows users to add, edit, and delete tasks.
Implemented the functionality to add new tasks at the top of the list and handle task additions or edits with the Enter key.
Connect with me on Twitter ๐๐ป
๐ Falak097
Check out my GitHub for complete code reference and more such React, JS and front-end development projects.
Subscribe to my newsletter
Read articles from Falak Gala directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
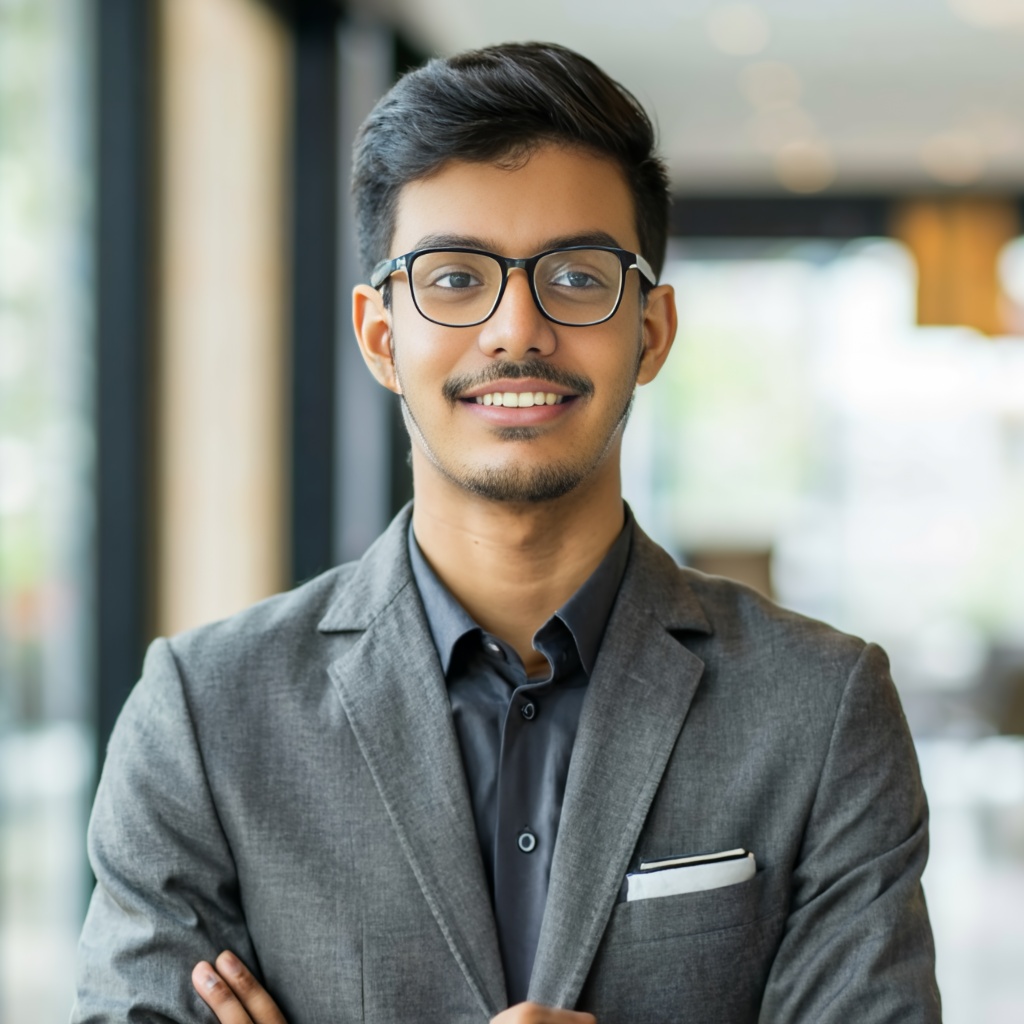