JavaScript Kung Fu: Mastering the Fundamentals (Episode 2)
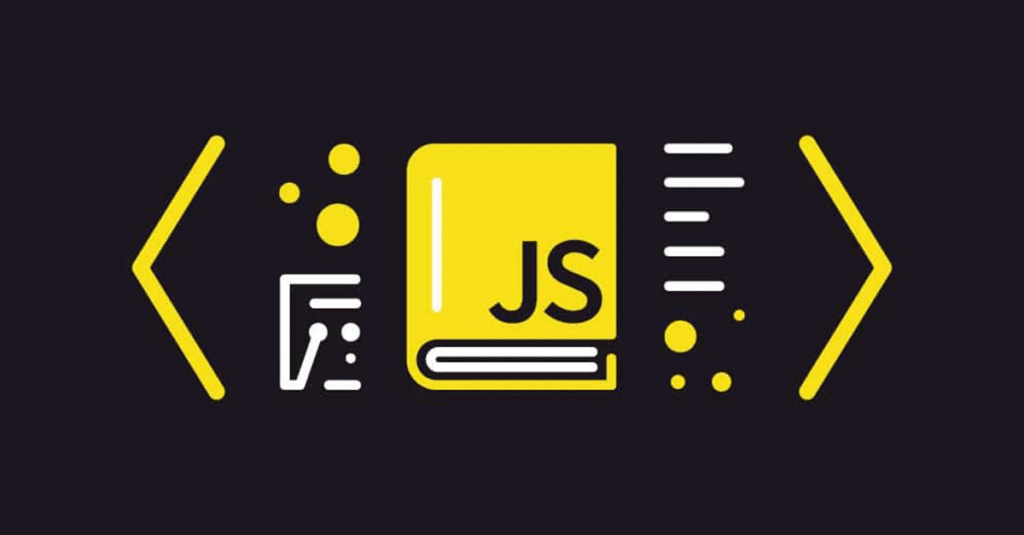
The Fundamentals of JavaScript
JavaScript is a versatile language that enables the creation of dynamic applications and interactive web content used daily. Understanding its fundamental concepts is essential for building a strong foundation. For beginners, JavaScript may initially appear complex. However, in this episode, we will cover three key areas: variables and data types, operators and expressions, and control flow with if statements and loops. These fundamental building blocks will provide you with the foundation needed to excel in software development.
Variables and Data Types
Variables
Imagine a carpenter needing a toolbox. Variables are similar to the compartments or containers within that toolbox, storing information that can be used, referenced, and manipulated throughout your program. You can name these compartments using descriptive terms. You declare a variable using the var
, let
, or const
keywords:
var: This is the old way to declare variables, with function scope.
let: It was introduced in ES6, allows block level scope.
const: Also introduced in ES6, used to declare variables that shouldn't change (constant).
Example:
//various ways to declare a variables
//var
var me = "Julius Adedeji";
// let
let profession = "software engineer";
//const
const yearsOfExperience = 5;
Data Types
What kind of information can you store in a variable? That is where data types come in. JavaScript offers various data types like:
- String: This represent text enclosed in a single or double quotes.
//string
let greeting = "Good morning, Julius";
- Number: It is a representation of numerical values, both integer and floating point numbers.
//Integer number
//A whole number with no fractional part
//It can be a positive number, a negative number or zero. Integers are stored as 32-bit or 64-bit values, depending on the platform.
let a = 20;
//Floating point number
//This data type is used to represent numbers that have a fractional part or to represent very large or small numbers
let b = 20.55;
- Boolean: Represents a logical entity and can have two values which are:
true or false
//true
let isTrue = true;
//false
let isFalse = false;
- Null: This represents the intentional absence of any object value.
//null
let notAnumber = null;
- Undefined: It is an indication that a variable has been declared but has not yet been assigned to a value.
let notDefined;
- Object: Objects are collections of key-value pairs. it represents complex data structures
let techBro = {
name : "Julius",
yearsOfExperience : 5
};
- Array: An array is a powerful data structure that allow you store an ordered collection of item under a single variable name.
let laptopItems = ["mouse", "battery", "bag"];
Operators and Expression
Operators are symbols that perform operations on variables and values, and JavaScript supports various types of operators. Continuing with the carpenter analogy, operators are your tools. They are used to perform calculations and comparisons on your data. Here are some essential operators:
- Arithmetic Operators: It is used to perform arithmetic on numbers.
//Addition arithmetic
let sum = 13 + 13; //26
//Subtration Arithmetic
let difference = 10 - 5; //5
//Multiplication
let product = 10 * 2; //20
//Quotient
let quotient = 10 / 2; //5
//Remainder
let remainder = 10 % 3; //1
- Assignment Operators: It is Used to assign values to variable.
//This line declares a variable named x and assigns the initial value of 10 to it.
let x = 10;
//The value 5 is added to the current value of x (which is 10) and the result (15) is then stored back into x.
x += 5; // x = x + 5
//Similar to the previous line, this uses the -= operator. It subtracts 3 from the current value of x (which is now 15) and stores the result (12) back into x.
x -= 3; // x = x - 3
//This line employs the *= operator. It multiplies the current value of x (12) by 2 and stores the result (24) back into x.
x *= 2; // x = x * 2
// this line uses the /= operator. It divides the current value of x (24) by 2 and stores the result (12) back into x.
x /= 2; // x = x / 2
//the above code snippet starts with x as 10 and then performs a series of calculations using shorthand assignment operators, ultimately ending with x having a value of 12.
- Comparison Operators:
//== checks for equality in value, but it can sometimes be misleading because it might also consider type conversions. Here, both operands (10) have the same value and type (number), so the comparison is true, and isEqual is assigned true
let isEqual = 10 == 10; // true
//=== checks for both value and type equality. In this case, even though the values appear similar (both represent the number 10), their types are different (number vs. string).
let isIdentical = 10 === "10"; // false
// compares the values of 10 and 5. Since 10 is indeed greater than 5, the comparison is true, and isGreater is assigned true
let isGreater = 10 > 5; // true
//It compares the values of 10 and 5. Here, 10 is not less than 5, so the comparison is false, and isLess is assigned false.
let isLess = 10 < 5; // false
- Logical Operators: These are used to perform logical operations.
//This line declares a variable andOperator and uses the && (AND) operator. The && operator only returns true if both operands are true.
let andOperator = true && false; // false
//This line uses the || (OR) operator. The || operator returns true if at least one operand is true.
let orOperator = true || false; // true
//This line uses the ! (NOT) operator, which is a unary operator (it takes only one operand). The ! operator inverts the truth value of its operand.
let notOperator = !true; // false
Control Flow
Control flow statements are used to control the execution of code based on certain conditions.
If Statements
The if
statement runs a block of code if a specified condition is true. Optionally, you can use else
to run a block of code if the condition is false.
Example:
let age = 18; // Declares a variable 'age' and assigns the value 18
// The following code block is an if-else statement
if (age >= 18) { // Checks if the value of 'age' is greater than or equal to 18
console.log("You are an adult."); // If the condition is true, this message is logged to the console
} else {
console.log("You are a minor."); // If the condition is false, this message is logged to the console
}
You can also use else if
to test multiple conditions:
let score = 85; // Declares a variable 'score' and assigns the value 85
// The following code block is a multi-way if-else if-else statement
if (score >= 90) {
console.log("Grade: A"); // Check for A grade (score >= 90)
} else if (score >= 80) {
console.log("Grade: B"); // Check for B grade if not A (score >= 80)
} else if (score >= 70) {
console.log("Grade: C"); // Check for C grade if not A or B (score >= 70)
} else {
console.log("Grade: F"); // Default case for score below 70
}
Loops
Loops are used to repeatedly execute a block of code as long as a specified condition is true.
- For Loop: The most commonly used loop in JavaScript
for (let i = 0; i < 5; i++) {
console.log(i);
}
- While Loop: Executes a block of code as long as a specified condition is true.
let i = 0;
while (i < 5) {
console.log(i);
i++;
}
- Do-While Loop: Similar to the
while
loop, but it executes the block of code at least once before checking the condition.
let i = 0;
do {
console.log(i);
i++;
} while (i < 5);
Conclusion
Understanding these fundamental concepts will give you a solid foundation in JavaScript, allowing you to write efficient and effective code. In the next sections, we will explore more advanced topics and practical applications of JavaScript. Thank you for reading.
Subscribe to my newsletter
Read articles from Julius Adedeji directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Julius Adedeji
Julius Adedeji
I am a Full Stack Engineer who delivers end-to-end solutions that solve complex business challenges with precision and expertise. I create scalable, robust, and user-centric applications by Leveraging a deep understanding of various programming languages and frameworks (JavaScript, TypeScript, Python, ReactJs, NextJS, MySql, Flask and Django). I'm well versed in both front-end and back-end development. I thrive in dynamic environments and relish excelling both independently and as a key player in collaborative teams. I am passionate about delivering impactful results and driving business growth by turning data insights into innovative software solutions in a rapidly evolving tech landscape. The proclivity to continuously learn and implement nascent industry technology interventions sets me apart.