Object Oriented Programming in Js
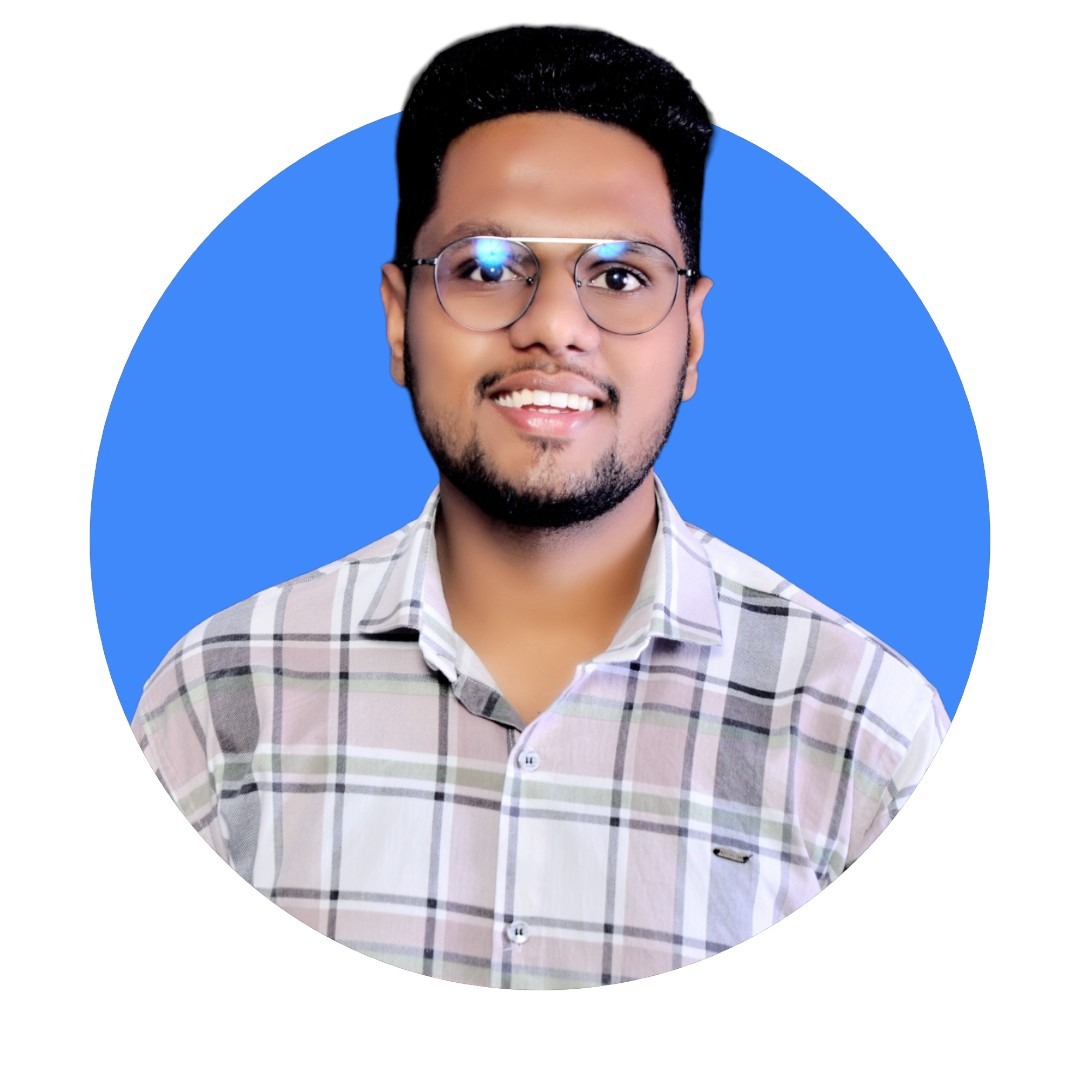
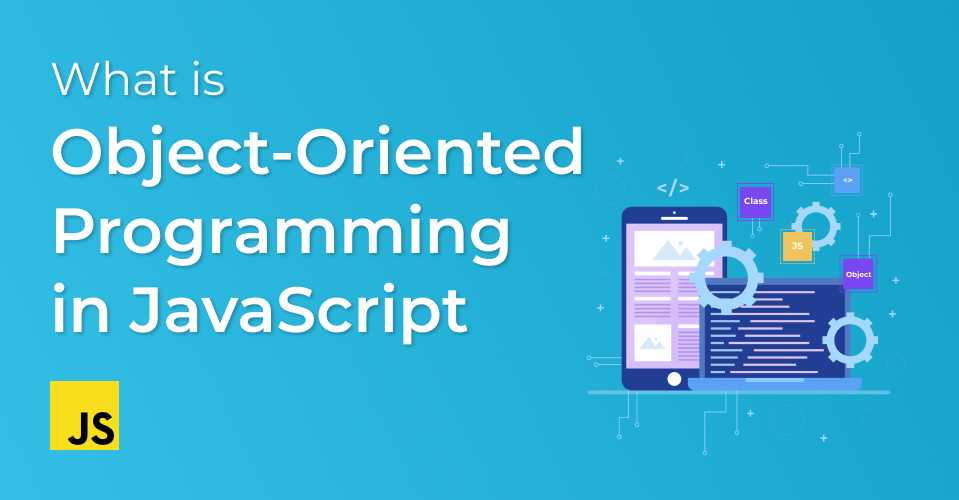
What Is Object-oriented Programming?
Object-oriented Programming treats data as a crucial element in program development and doesn't allow it to flow freely around the system.
It ties data more securely to the function that operates on it and protects it from accidental modification from an outside function.
OOP breaks down a problem into several entities called objects and builds data and functions around these objects.
JavaScript Classes :
In JavaScript, classes are the special type of functions.
We can define the class just like function declarations and function expressions.
The JavaScript class contains various class members within a body including methods or constructor.
The class is executed in strict mode. So, the code containing the silent error or mistake throws an error.
The class syntax contains two components:
Class declarations
Class expressions
Class Declarations :
A class can be defined by using a class declaration.
A class keyword is used to declare a class with any particular name.
According to JavaScript naming conventions, the name of the class always starts with an uppercase letter.
Class Declarations Example
Let's see a simple example of declaring the class.
<!DOCTYPE html>
<html>
<body>
<script>
//Declaring class
class Employee
{
//Initializing an object
constructor(id,name)
{
this.id=id;
this.name=name;
}
//Declaring method
detail()
{
document.writeln(this.id+" "+this.name+"<br>")
}
}
//passing object to a variable
var e1=new Employee(101,"Martin Roy");
var e2=new Employee(102,"Duke William");
e1.detail(); //calling method
e2.detail();
</script>
</body>
</html>
Output:
Class expressions :
Another way to define a class is by using a class expression.
Here, it is not mandatory to assign the name of the class. So, the class expression can be named or unnamed.
The class expression allows us to fetch the class name. However, this will not be possible with class declaration.
Unnamed Class Expression :
The class can be expressed without assigning any name to it.
Let's see an example.
<!DOCTYPE html>
<html>
<body>
<script>
var emp = class {
constructor(id, name) {
this.id = id;
this.name = name;
}
};
document.writeln(emp.name);
</script>
</body>
</html>
JavaScript Objects :
A javaScript object is an entity having state and behavior (properties and method). For example: car, pen, bike, chair, glass, keyboard, monitor etc.
JavaScript is an object-based language. Everything is an object in JavaScript.
JavaScript is template based not class based. Here, we don't create class to get the object. But, we direct create objects.
Creating Objects in JavaScript
There are 3 ways to create objects.
By object literal
By creating instance of Object directly (using new keyword)
By using an object constructor (using new keyword)
JavaScript Abstraction :
An abstraction is a way of hiding the implementation details and showing only the functionality to the users.
In other words, it ignores the irrelevant details and shows only the required one.
We cannot create an instance of Abstract Class.
It reduces the duplication of code.
JavaScript Abstraction Example :
<!DOCTYPE html>
<html>
<body>
<script>
//Creating a constructor function
function Vehicle()
{
this.vehicleName="vehicleName";
throw new Error("You cannot create an instance of Abstract Class");
}
Vehicle.prototype.display=function()
{
return "Vehicle is: "+this.vehicleName;
}
//Creating a constructor function
function Bike(vehicleName)
{
this.vehicleName=vehicleName;
}
//Creating object without using the function constructor
Bike.prototype=Object.create(Vehicle.prototype);
var bike=new Bike("Honda");
document.writeln(bike.display());
</script>
</body>
</html>
Output:
Vehicle is: Honda
JavaScript Encapsulation :
The JavaScript Encapsulation is a process of binding the data (i.e. variables) with the functions acting on that data.
It allows us to control the data and validate it.
To achieve an encapsulation in JavaScrip.
Use var keyword to make data members private.
Use setter methods to set the data and getter methods to get that data.
The encapsulation allows us to handle an object using the following properties:
Read/Write - Here, we use setter methods to write the data and getter methods read that data.
Read Only - In this case, we use getter methods only.
Write Only - In this case, we use setter methods only.
JavaScript Encapsulation Example :
<script>
class Student
{
constructor()
{
var name;
var marks;
}
getName()
{
return this.name;
}
setName(name)
{
this.name=name;
}
getMarks()
{
return this.marks;
}
setMarks(marks)
{
this.marks=marks;
}
}
var stud=new Student();
stud.setName("John");
stud.setMarks(80);
document.writeln(stud.getName()+" "+stud.getMarks());
</script>
Output:
John 80
JavaScript static Method :
The JavaScript provides static methods that belong to the class instead of an instance of that class.
So, an instance is not required to call the static method.
These methods are called directly on the class itself.
Points to remember:
The static keyword is used to declare a static method.
The static method can be of any name.
A class can contain more than one static method.
If we declare more than one static method with a similar name, the JavaScript always invokes the last one.
The static method can be used to create utility functions.
We can use this keyword to call a static method within another static method.
We cannot use this keyword directly to call a static method within the non-static method. In such case, we can call the static method either using the class name or as the property of the constructor.
Example:
<!DOCTYPE html>
<html>
<body>
<script>
class Test
{
static display()
{
return "static method is invoked"
}
}
document.writeln(Test.display());
</script>
</body>
</html>
Output:
static method is invoked
Whats Next?
JS OOP Inhiritance , Constructor.
Subscribe to my newsletter
Read articles from Aditya Gadhave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
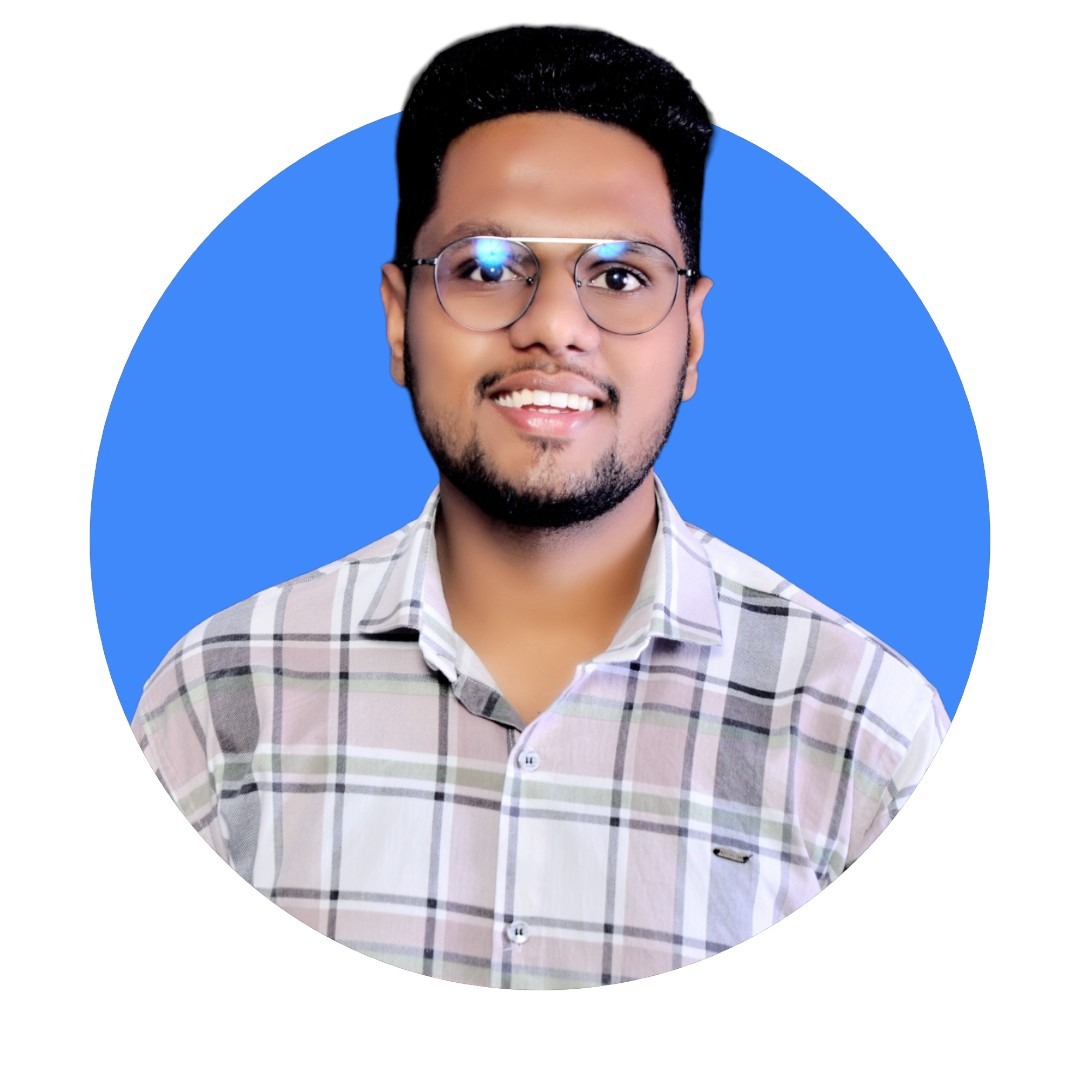
Aditya Gadhave
Aditya Gadhave
๐ Hello! I'm Aditya Gadhave, an enthusiastic Computer Engineering Undergraduate Student. My passion for technology has led me on an exciting journey where I'm honing my skills and making meaningful contributions.