How Inheritance Works in Java Programming
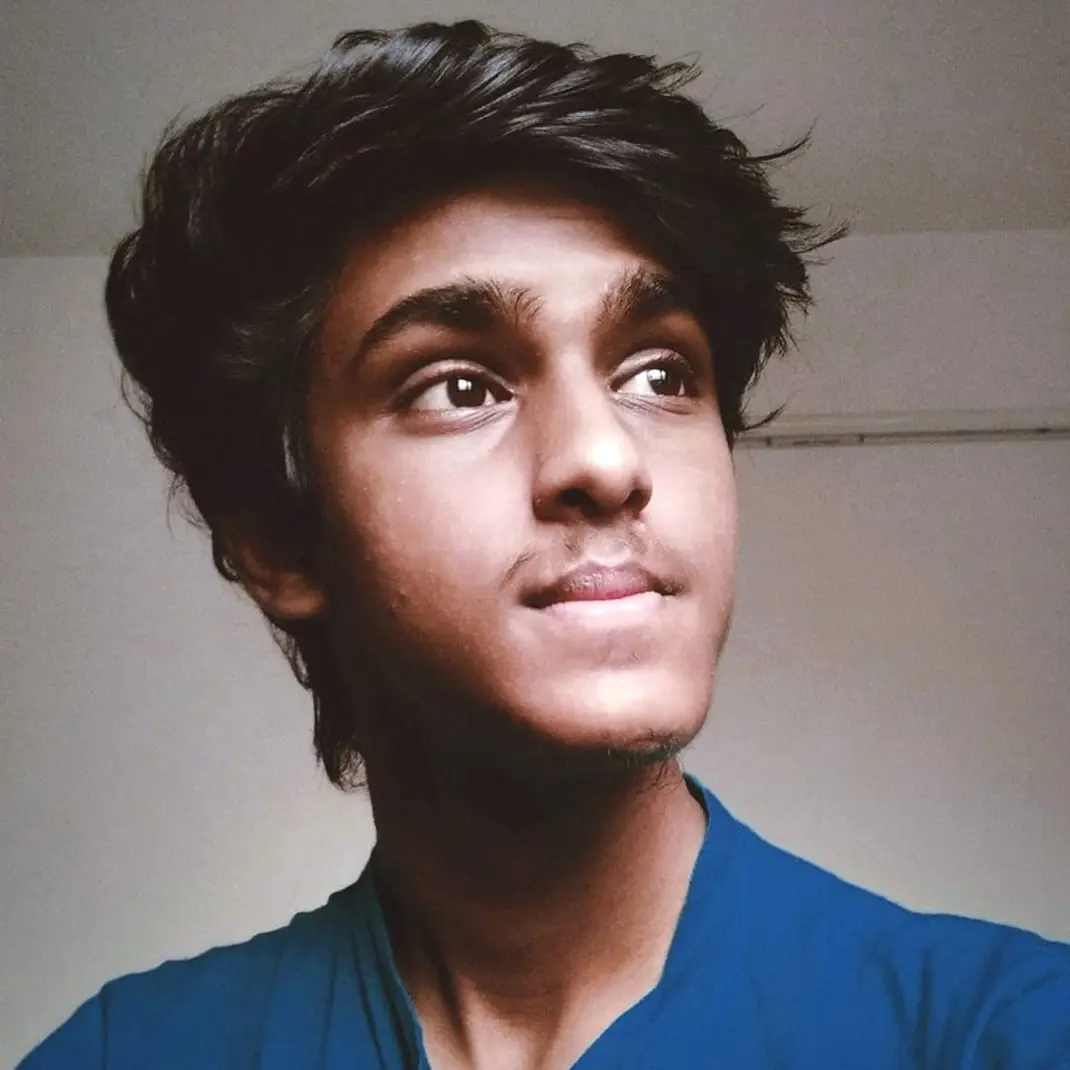

Inheritance
Inheritance is a core concept in Java that allows a class to inherit the properties and methods of a base class, enabling code reusability and hierarchical classification. This allows a child class to inherit and extend the functionality of a parent class.
Inheritance is followed in order to reduce code redundancy. Code redundancy is nothing but the repetition of lines of code in a program. Hence, inheritance is a main pillar that allows us to write and manage our code effectively.
Inheritance can be achieved through the extends
keyword. A child class should use the extends
keyword in order to acquire the parent class's data members and member functions.
Example for Inheritance
Fig. 1: A Simple Example of Inheritance
The Robot
class serves as the parent class, defining fundamental behaviors for all robot types. It includes methods such as talk()
, walk()
, and charge()
.
There are three specialized child classes inheriting from Robot
:
Chef Robot Class:
Inherits methods:
talk()
,walk()
,charge()
.Child specific method:
cook()
.Description: The
ChefRobot
class extendsRobot
, inheriting basic functionalities for communication, movement, and power management. It specializes further with thecook()
method, allowing it to prepare meals autonomously.
Doctor Robot Class:
Inherits methods:
talk()
,walk()
,charge()
.Child Specific method:
surgery()
.Description: The
DoctorRobot
class extendsRobot
, inheriting essential capabilities for communication, mobility, and energy replenishment. It is equipped with thesurgery()
method, enabling it to perform intricate medical procedures with precision.
Teacher Robot Class:
Inherits methods:
talk()
,walk()
,charge()
.Child Specific method:
teach()
.Description: The
TeacherRobot
class extendsRobot
, inheriting core functionalities including communication, locomotion, and power management. It introduces theteach()
method, allowing it to deliver educational content and interact with students effectively.
These child classes demonstrate the concept of inheritance in object-oriented programming, where common functionalities are inherited from a parent class (Robot
), and specialized behaviors are defined in each child class (ChefRobot
, DoctorRobot
, TeacherRobot
). This approach promotes code reusability, modularity, and extensibility in software design.
Code for Inheritance
Parent Robot Class
public class Robot { // Parent Class
public void talk() {
System.out.println("Robot is talking"); // talk method
}
public void walk() {
System.out.println("Robot is walking"); // walk method
}
public void charge() {
System.out.println("Robot is charging"); // charge method
}
}
Code Snippet of Robot (parent) class
This is a Robot class with the methods of talk()walk()charge()
.
Child Chef Robot class:
class ChefRobot extends Robot{ // extends keyword is used
//to implement inheritance
public void cook() {
System.out.println("Chef robot is cooking");
}
}
Code Snippet of ChefRobot (child) class
ChefRobot
class is a child class of Robot
Class. This class has a child specific method cook()
.
Child Doctor Robot class:
class DoctorRobot extends Robot{//extends keyword is used to implement
//inheritance
public void surgery() { // child specific method
System.out.println("Doctor robot is doing a surgery");
}
}
Code Snippet of DoctorRobot (child) Class
DoctorRobot
class which is a child class of Robot
Class. This class has a child specific method surgery()
.
Child Teacher Robot class:
class TeacherRobot extends Robot{ // extends keyword is used
//to implement inheritance
public void teach() { // Child specific method
System.out.println("Teacher robot is teaching");
}
}
Code snippet of TeacherRobot (child) Class
TeacherRobot
class is a child class of Robot
Class. This class has a child specific method teach()
.
Robot App Class:
public class RobotApp {
public static void main(String[] args) {
Robot r1=new Robot();// creating an object for Robot Class
r1.walk(); // calling walk method of Robot Class
r1.talk(); // calling talk method of Robot Class
r1.charge(); // calling charge method of Robot Class
ChefRobot cr=new ChefRobot();// creating am object for chef robo
cr.walk(); // calling walk method of ChefRobo class
cr.talk(); // calling talk method of ChefRobo class
cr.charge(); // calling charge method of ChefRobo class
cr.cook(); // calling child specific cook method of ChefRobo class
DoctorRobot dr=new DoctorRobot();// creating an object for doc robo
dr.walk();// calling walk method of DoctorRobo class
dr.talk();// calling talk method of DoctorRobo class
dr.charge();// calling charge method of DoctorRobo class
dr.surgery();// calling child specific method of DoctorRobo class
TeacherRobot tr=new TeacherRobot();// creating an object for teacher robo
tr.walk(); // calling walk method of TeacherRobo class
tr.talk(); // calling talk method of TeacherRobo class
tr.charge(); // calling charge method of TeacherRobo class
tr.teach();// calling child specific method of TeacherRobo class
}
}
Code Snippet of RobotApp (implementation) Class
The RobotApp
class demonstrates the usage of inheritance and polymorphism in Java through different types of robots inheriting from the Robot
class.
Robot Class Usage:
- An object
r1
of typeRobot
is created and used to call methodswalk()
,talk()
, andcharge()
, which are defined in theRobot
class itself.
- An object
ChefRobot Class Usage:
An object
cr
of typeChefRobot
is created and used to call inherited methodswalk()
,talk()
,charge()
from theRobot
class.Additionally, the
cook()
method, specific toChefRobot
, is called usingcr.cook()
.
DoctorRobot Class Usage:
An object
dr
of typeDoctorRobot
is created and used to call inherited methodswalk()
,talk()
,charge()
from theRobot
class.The
surgery()
method, specific toDoctorRobot
, is called usingdr.surgery
()
.
TeacherRobot Class Usage:
An object
tr
of typeTeacherRobot
is created and used to call inherited methodswalk()
,talk()
,charge()
from theRobot
class.The
teach()
method, specific toTeacherRobot
, is called usingtr.teach()
.
Each robot type demonstrates the concept of inheritance, where they inherit common behaviors (walk()
, talk()
, charge()
) from the Robot
class and implement their own specific behaviors (cook()
, surgery()
, teach()
).
This approach promotes code reuse, enhances maintainability, and allows for specialized functionalities tailored to different types of robots in a modular and organized manner.
Expected Output:
Fig. 7: Snapshot of output of the program.
Note Private data members of a class can never be inherited by a child class. In other words Private data members of a class will never participate in inheritance.
Example:
public class Parent {
// Public field that can be inherited
String eye_color = "Grey";
// Private field that cannot be inherited
private int atm_pin = 1234;
}
public class Child extends Parent {
// Inherits eye_color field from Parent class
// Does not inherit atm_pin as it is private in Parent class
}
public class ParentApp {
public static void main(String[] args) {
// Creating an instance of the Child class
Child c = new Child();
// Accessing inherited field from Parent class
System.out.println(c.eye_color); // This will print "Grey"
// Trying to access private field from Parent class (will cause an error)
System.out.println(c.atm_pin); // ERROR: because private data members can never be inherited
}
}
The eye_color
field in the Parent
class is declared with default visibility (package-private), making it accessible within the same package and inheritable by subclasses in the same package.
The atm_pin
field in the Parent
class is private, meaning it is not accessible outside the Parent
class, including in subclasses. Therefore, attempting to access atm_pin
from an instance of Child
will result in a compilation error.
Advantages of Inheritance
Code Reusability: Inherited methods and attributes can be reused in derived classes, reducing redundancy and promoting cleaner, more maintainable code.
Extensibility: Derived classes can extend the functionality of base classes by adding new methods and attributes without modifying the existing code, thus supporting incremental development.
Polymorphism: Inheritance facilitates polymorphic behavior, where objects of derived classes can be treated as objects of their base class. This allows for flexibility in designing and implementing different behaviors for related objects.
Organization and Structure: Inheritance hierarchies provide a natural way to organize and structure code, making it easier to understand and maintain complex systems.
Promotes Code Consistency: By defining common attributes and behaviors in a base class, inheritance helps enforce consistency across derived classes, ensuring that they adhere to the same interface and behavior.
In summary inheritance enhances code reusability, promotes extensibility, facilitates polymorphism, improves code organization, and promotes consistency in object-oriented design.
Interested in learning about the types of inheritance? Feel free to read my blog on the topic: Understanding Different Types of Inheritance in Java.
Conclusion
In conclusion, inheritance is a fundamental feature of object-oriented programming that promotes code reuse, extensibility, and polymorphic behavior. It allows for efficient organization of code and enhances software design by facilitating hierarchical relationships among classes. Adopting inheritance can lead to more maintainable and scalable software systems.
Subscribe to my newsletter
Read articles from Sridhar K directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
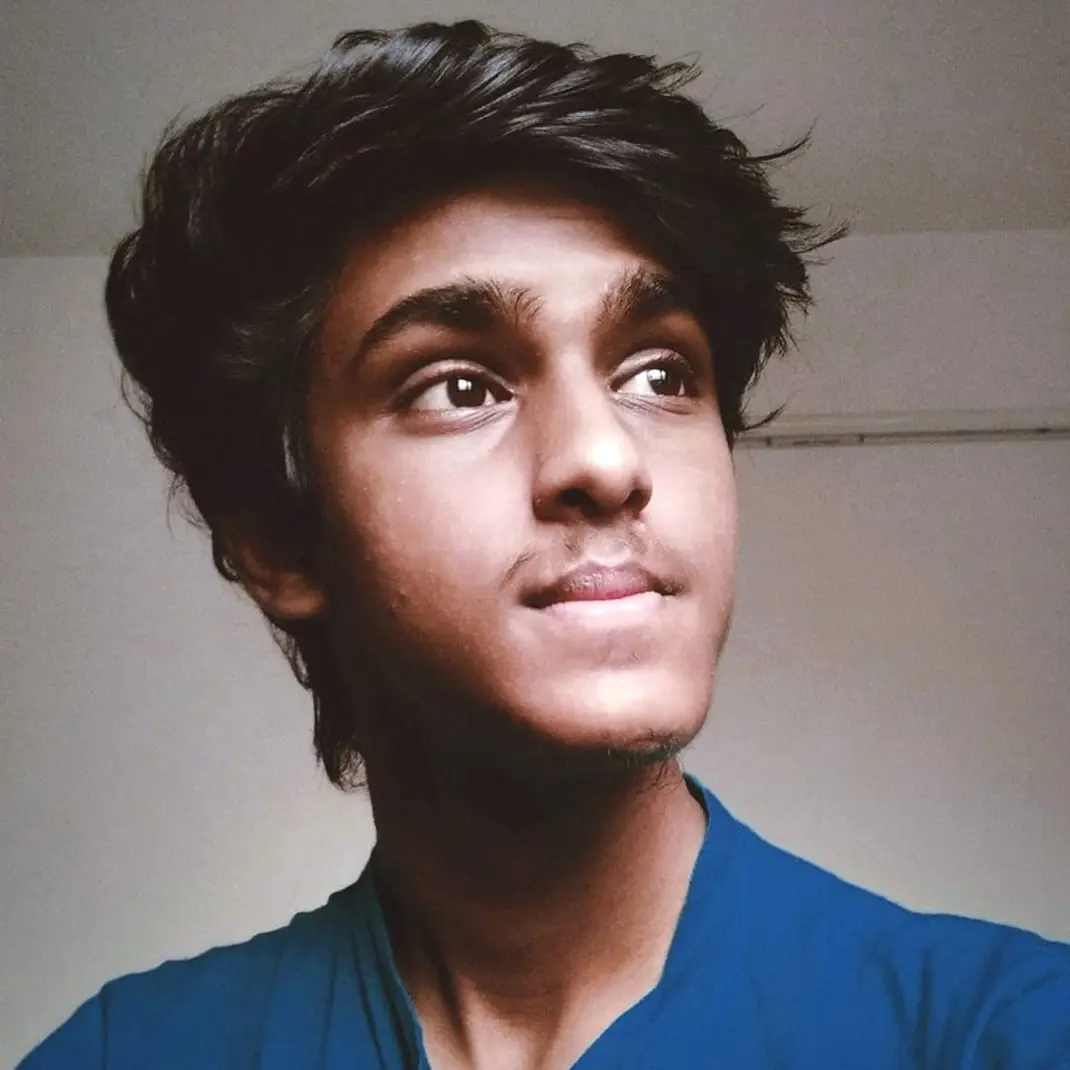
Sridhar K
Sridhar K
I am a fresher Software developer with a strong foundation in Java, HTML, CSS, and JavaScript. I have experience with various libraries and frameworks, including Spring Boot, Express.js, Node.js, Hibernate, MySQL, and PostgreSQL. I am eager to leverage my technical skills and knowledge to contribute effectively to a dynamic development team. I am committed to continuous learning and am excited to begin my career in a challenging developer role.