A Guide to Java Methods: Types and Uses
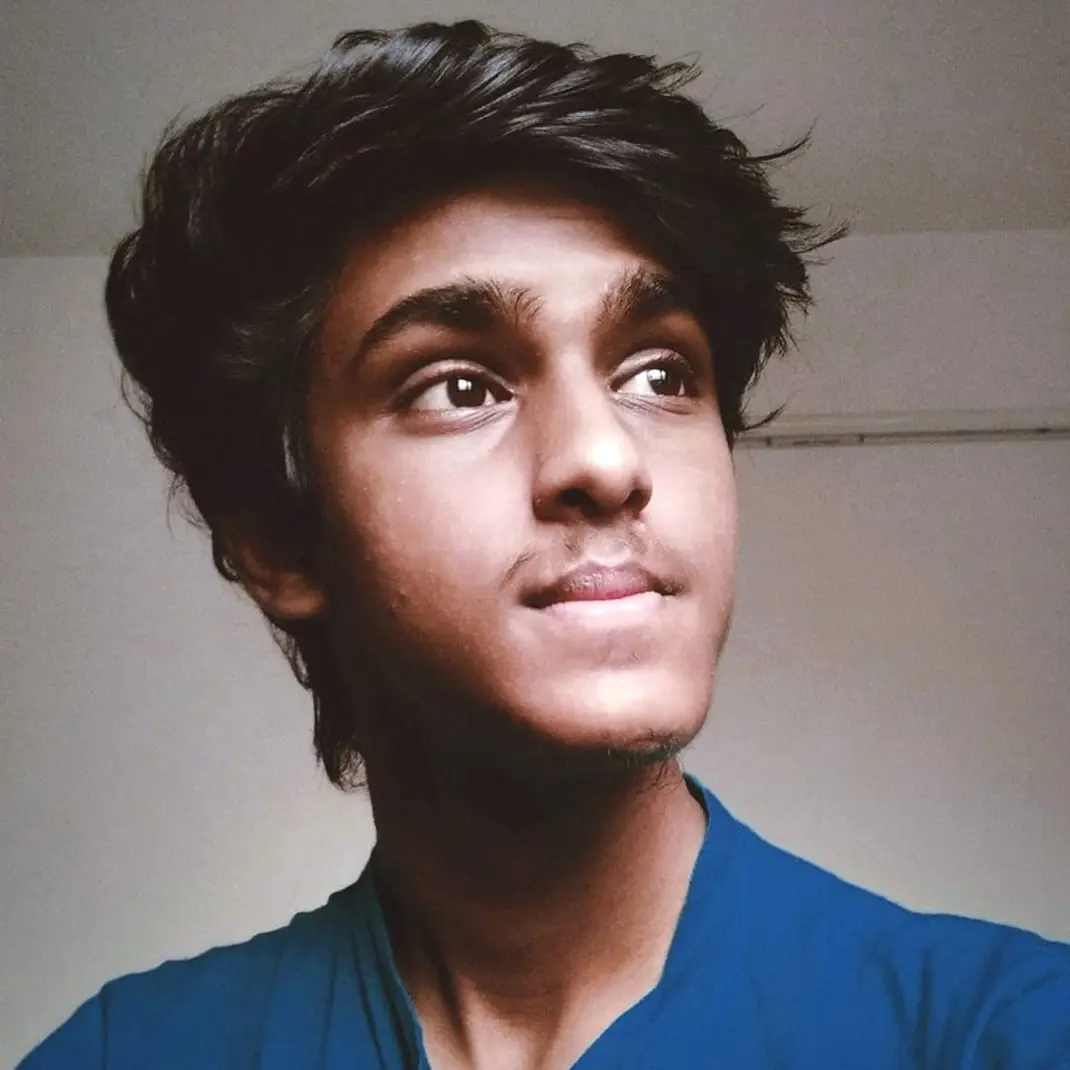
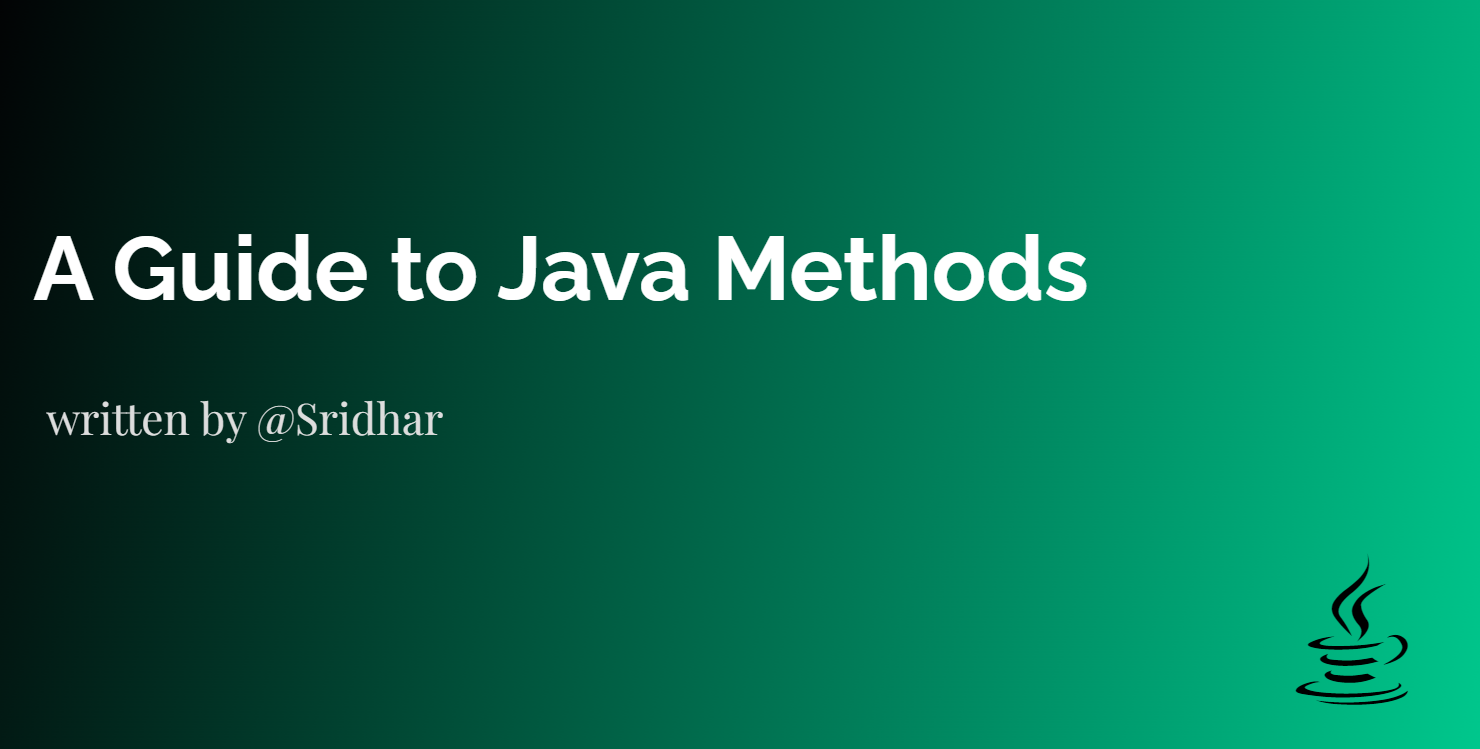
Understanding Different Types of Java Methods
Methods
Methods in Java are specific blocks of code designed to perform a task. They are written once and can be called multiple times as needed by the programmer.
Methods are utilized to reduce code complexity and redundancy, saving developers significant time by eliminating the need to repeatedly write the same lines of code. Instead of duplicating code, simply encapsulate the block of code that needs to be reused in a method. By calling the method by its name, this block of code can be executed any number of times.
Brushing teeth, bathing, eating, and drinking water are essential daily tasks that become second nature to us as we mature. These activities follow routines we develop over time, such as knowing how to brush our teeth or eat without much thought. These can be likened to real-life methods.
In programming, methods function similarly: they act as predefined routines. Once written, methods encapsulate specific tasks and can be invoked whenever needed throughout the program. This approach reduces the need to rewrite the same code, promoting efficiency and consistency in software development.
Syntax
access_modifier return_type methodName(parameters) {
// method body
// return statements
}
This is a syntax to define a method. Here's what each component means:
access_modifier: This determines who can access this method. it could be public, private, or protected.
There are Four types of access_modifiers in java:
public: Accessible from any other class.
private: Accessible only within the same class.
protected: Accessible within the same package and by subclasses (whether they are in the same package or not).
default (no modifier specified): Accessible only within the same package.
return_type: This is the data type of the value that the method returns. void
will be the return type if the method doesn't return any value.
methodName: This is the name of the method, used to call the method to perform a specific task multiple times. CamelCase convention is used here to define a method's name (e.g., methodName
). It is a good practice for a method's name to start with a lowercase letter.
parameters: These are inputs to the method, which are optional for the user to provide. A method may or may not have parameters.
How to call a method
To call a method, simply add ()
to the method name that you want to execute.
access_modifier return_type methodName() {
// method body
// return statements
}
methodName(); // name of the method which you desired to call for an execution
In Java, you call a method by specifying its name followed by parentheses ()
.
methodName
is the name of the method you want to call.The
()
may contain arguments if the method requires parameters.
This is the syntax to call a method with zero parameters.
Syntax for parameterized methods
// Method declaration with parameters
access_modifier return_type methodName(parameter1_type parameter1, parameter2_type parameter2, ...) {
// Method body
// Use parameters in the method logic
}
// to call a parameterized method
methodName(value1, value2);
In this syntax:
methodName
is the name of the method you want to call.value1
andvalue2
are the actual values (or variables containing values) that you pass as arguments to the method.
Parameters (parameter1
, parameter2
, ...) are placeholders in the method declaration that define the types and names of the values that the method expects to receive when it is called. They serve as variables within the method's scope, representing the data that the method will operate on.
Arguments (value1
, value2
) are the actual data that you pass to the method when calling it. These values can be literals, variables, or expressions, and they are substituted for the corresponding parameters defined in the method signature.
When calling methodName(value1, value2);
, you are passing value1
and value2
as arguments to the methodName
method. These arguments are assigned to parameter1
and parameter2
respectively in the method's parameter list. This allows the method to execute its logic using the specific values provided at the time of the method call.
Important note No two methods can have a same name. This makes confusion for the java compiler to execute.
Non Parameterized method
Definition: A non-parameterized method in Java is a method that does not accept any parameters. It is declared with empty parentheses ()
in its method signature, indicating that it does not require any input values to perform its functionality.
Usage: Non-parameterized methods are useful for performing tasks that do not depend on external inputs or for operations that have predefined behaviors or configurations. They are straightforward to call and execute since they do not require any arguments during method invocation.
public class PubgGame {
public static void main(String[] args) {
// Call the pubgGame method directly
pubgGame();
}
public static void pubgGame() { // method declaration
// method body
System.out.println("Boot the game");
System.out.println("Invite friends");
System.out.println("Start the game");
System.out.println("Mark the location");
System.out.println("Invite your friends to jump at marked location");
System.out.println("Take loots for you");
System.out.println("Move to the safe zone");
System.out.println("Go for kills");
System.out.println("Make it to the top ten");
System.out.println("Kill the last team to get winner winner chicken dinner");
}
}
Here, pubgGame
is a zero-parameter method designed to help a newbie player understand the game. The player needs to follow these steps in order to get the "winner winner chicken dinner." The method has void
as its return type because it does not return any value.
pubgGame()
is used to call the pubgGame
method, which will then get executed.
We write it in a method so that it can be called multiple times to execute the block of code, avoiding the need to write it every time and thereby preventing code redundancy.
Output
Boot the game
Invite friends
Start the game
Mark the location
Invite your friends to jump at marked location
Take loots for you
Move to the safe zone
Go for kills
Make it to the top ten
Kill the last team to get winner winner chicken dinner
This is an example for non parameterized method.
Parameterized method
Definition: A parameterized method in Java is declared with one or more parameters inside the parentheses of its method signature. These parameters specify the type and order of values that the method expects to receive when it is invoked.
Usage: Parameterized methods enhance the flexibility and reusability of code by enabling methods to operate on different data based on the values passed during each method call. Parameters act as placeholders for these values, allowing methods to be more versatile.
Let's see an example for parameterized method.
public class Calculator {
// Parameterized static method to add two numbers and print the result
public static void add(int num1, int num2) {
int sum = num1 + num2;
System.out.println("The sum is: " + sum);
}
public static void main(String[] args) {
// Call the static add method directly without instantiating the class
add(5, 10);
}
}
Explanation
Class Definition:
public class Calculator { ... }
defines a class namedCalculator
.
Parameterized Static Method:
public static void add(int num1, int num2) { ... }
defines a static method namedadd
that takes two integer parametersnum1
andnum2
.Inside the method, the sum of
num1
andnum2
is calculated and printed:int sum = num1 + num2;
followed bySystem.out.println("The sum is: " + sum);
.
Main Method:
public static void main(String[] args) { ... }
is the entry point of the Java application.Inside the
main
method, the staticadd
method is called directly with the arguments5
and10
:add(5, 10);
.
Output
The sum is: 15
This is an example for parameterized method.
Rules to be Followed While Defining a method
When declaring a method in Java, there are several rules and conventions to follow to ensure proper syntax and clarity. Here are the key rules to keep in mind:
Method Signature: A method declaration includes its signature, which consists of the method name and its parameters (if any). The signature is followed by the method body enclosed in curly braces
{}
.Method Name:
Must be a valid identifier.
Should use camelCase convention (e.g.,
calculateTotal
,printDetails
).Should be descriptive and indicative of the method's purpose.
Return Type:
Specify the data type of the value returned by the method (
void
if no return value).Use
void
if the method does not return any value.Other valid return types include
int
,double
,String
, etc.
Parameters:
Enclosed in parentheses
()
after the method name.Specify the data type and name of each parameter (e.g.,
int num1, int num2
).Multiple parameters are separated by commas.
Access Modifier:
Specifies the visibility of the method (e.g.,
public
,private
,protected
, or package-private/default).If no access modifier is specified, the method has package-private visibility.
Static Modifier:
- Specifies whether the method belongs to the class itself (
static
) or to instances of the class (non-static).
- Specifies whether the method belongs to the class itself (
Method Body:
Contains the executable code of the method enclosed in
{}
.Code within the method body should follow Java's syntax rules and best practices.
Types of methods in Java
There are four types of methods on the basis of parameters in java, they are
- Method 1:
These type of method doesn't take any parameters
It also doesn't provide any output
void
will be the return type
Example for method 1
public class Method1 {
public static void main(String[] args) {
learnMethods(); // Calling the learnMethods() method directly
}
public static void learnMethods() {
System.out.println("I am learning methods"); // Printing a message
}
}
In this example, learnMethods()
does not take any parameters, nor does it return any value as output. It simply prints a message within its block.
Output
I am learning methods
- Method 2:
These types of methods takes parameters
It doesn't provide any output.
void
will be the return type for this method.
Example for method 2
public class Method2 {
public static void main(String[] args) {
String name = "Developer";
learnMethods(name); // Calling the learnMethods() method with argument
}
public static void learnMethods(String name) {
System.out.println(name + " is learning methods"); // Printing a message with parameter
}
}
In this example, learnMethods()
takes a parameter as input but does not return any output. Therefore, the method has a return type of void
. It simply prints the message within its method block.
Output
Developer is learning methods
- Method 3:
These types of methods Doesn't take any parameters as input
But it generates a return type as output
It has a
return
type.
Example for method 3
public class Method3 {
public static void main(String[] args) {
int result = multiply(); // Calling the multiply() method and storing the result
System.out.println("The multiplication result is: "+result); // Printing the result
}
public static int multiply() {
int a = 2;
int b = 4;
int c = a * b;
return c; // Returning the product of a and b
}
}
In this example, multiply()
does not take any parameters as input. Instead, the method declares integer variables a
and b
with values 2
and 4
respectively. Variable c
is an integer that holds the result of multiplying a
and b
. The method returns this multiplied value.
The return type of multiply()
is int
because it returns an integer value.
In the main
method, result
is a variable of type int
used to store the value returned from multiply()
. Subsequently, result
is printed as the output.
Output
The multiplication result is: 8
- Method 4:
These types of methods takes parameters as input
It also produce output.
It also has a specific return type as it returns some value as output.
Example for method 4:
public class Method4 {
public static void main(String[] args) {
int a = 20;
int b = 4;
int result = multiply(a, b); // Calling the multiply() method and storing the result
System.out.println("The multiplication result is: " + result); // Printing the result
}
public static int multiply(int a, int b) {
return a * b; // Returning the product of a and b
}
}
In the main()
method, we have three integer variables: a
, b
, and result
. a
has a value of 2, and b
has a value of 4. These values are passed to multiply()
, which returns the product of a
and b
. This returned value is stored in the result
variable, which is then printed as output.
This method takes parameters as input, provides output, and has a return type.
Output
The multiplication result is: 80
Let's have a quick overview of what we have discussed about method types
Types | Takes Parameter | Generates a output | Return type |
Method 1 | No | No | void |
Method 2 | Yes | No | void |
Method 3 | No | Yes | Has a return type |
Method 4 | Yes | Yes | Has a return type |
Conclusion
In this overview of Java methods, we explored how methods serve as reusable blocks of code designed to perform specific tasks efficiently. Methods are crucial for reducing code redundancy and organizing logic into manageable parts.
We covered the syntax for declaring methods, which includes defining access modifiers, return types, method names, and parameters. Methods can be categorized based on whether they accept parameters, produce output, and have return types.
We discussed four types of methods:
Method 1: Takes no parameters, produces no output, and has a return type of
void
.Method 2: Takes parameters, produces no output, and has a return type of
void
.Method 3: Takes no parameters, produces output, and has a specific return type.
Method 4: Takes parameters, produces output, and has a specific return type.
Each type serves different programming needs, allowing flexibility in how methods are structured and utilized within Java applications. Understanding these method types enables developers to write efficient and organized code, enhancing readability and maintainability in software development.
Subscribe to my newsletter
Read articles from Sridhar K directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
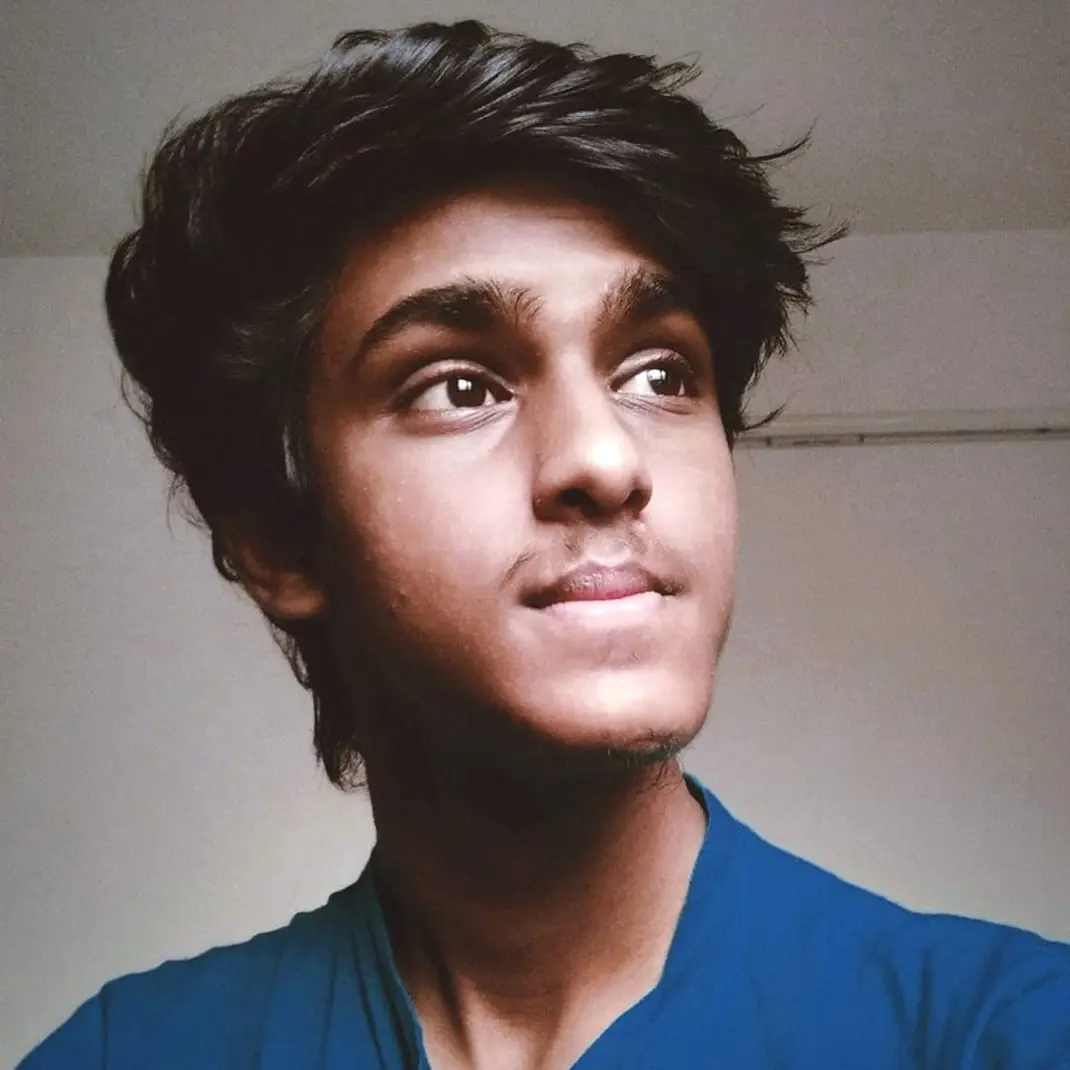
Sridhar K
Sridhar K
I am a fresher Software developer with a strong foundation in Java, HTML, CSS, and JavaScript. I have experience with various libraries and frameworks, including Spring Boot, Express.js, Node.js, Hibernate, MySQL, and PostgreSQL. I am eager to leverage my technical skills and knowledge to contribute effectively to a dynamic development team. I am committed to continuous learning and am excited to begin my career in a challenging developer role.