Python List Comprehensions: Write Cleaner, Faster Code
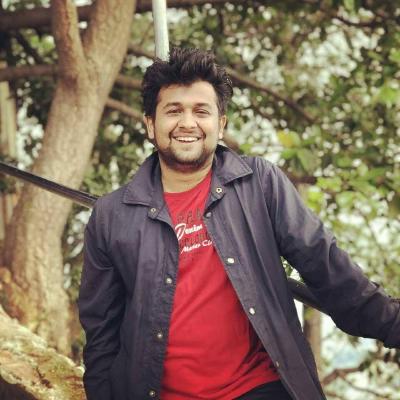
Introduction
List comprehensions are one of Python's most distinctive features. They offer a succinct way to create lists and transform existing lists. In this article, we will delve deep into the world of list comprehensions, exploring their syntax, use cases, and benefits. So, buckle up and let's dive into the magic of list comprehensions!
What are list comprehensions?
List comprehensions provide a concise way to generate lists in Python. They are more readable and faster than traditional for-loops and map() functions. A basic list comprehension consists of the following parts:
An expression that produces elements of the new list.
A
for
clause to iterate over an existing collection.Optional
if
clauses to filter elements.
The general syntax is:
[expression for item in iterable if condition]
Let's start with a simple example to warm up.
Basic Example
Suppose we want to create a list of squares for the numbers from 0 to 9.
Traditional loop
squares = []
for i in range(10):
squares.append(i ** 2)
print(squares)
List Comprehension
squares = [i ** 2 for i in range(10)]
print(squares)
Both methods produce the same result: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
, but the list comprehension is more compact and readable.
Adding Conditions
You can add conditions to your list comprehensions to filter elements.
Example: Even Squares
Suppose we only want the squares of even numbers.
Traditional Loop
even_squares = []
for i in range(10):
if i % 2 == 0:
even_squares.append(i ** 2)
print(even_squares)
List Comprehension
even_squares = [i ** 2 for i in range(10) if i % 2 == 0]
print(even_squares)
The result is [0, 4, 16, 36, 64]
.
Nested List Comprehensions
List comprehensions can also be nested. This is particularly useful when working with multi-dimensional data structures like matrices.
Example: Flattening a Matrix
Suppose we have a matrix and we want to flatten it into a single list.
Traditional Loop
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened = []
for row in matrix:
for num in row:
flattened.append(num)
print(flattened)
List Comprehension
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
flattened = [num for row in matrix for num in row]
print(flattened)
The result is [1, 2, 3, 4, 5, 6, 7, 8, 9]
.
List Comprehensions with Functions
You can also use functions within your list comprehensions to create more complex transformations.
Example: Applying a Function
Let's define a simple function and apply it to a list using a list comprehension.
def double(x):
return x * 2
doubled = [double(i) for i in range(10)]
print(doubled)
The result is [0, 2, 4, 6, 8, 10, 12, 14, 16, 18]
.
Combining Multiple Conditions
List comprehensions can handle multiple conditions, allowing for complex filtering logic.
Example: Prime Numbers
Let's generate a list of prime numbers between 2 and 50.
def is_prime(n):
if n <= 1:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
primes = [x for x in range(2, 51) if is_prime(x)]
print(primes)
The result is [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47]
.
List Comprehensions with Multiple Iterables
You can also create list comprehensions that iterate over multiple iterables.
Example: Cartesian Product
Let's generate the Cartesian product of two lists.
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
cartesian_product = [(x, y) for x in list1 for y in list2]
print(cartesian_product)
The result is [(1, 'a'), (1, 'b'), (1, 'c'), (2, 'a'), (2, 'b'), (2, 'c'), (3, 'a'), (3, 'b'), (3, 'c')]
.
Practical Applications
List comprehensions are not just for neat tricks; they have practical uses in real-world applications.
Example: Reading a File
Suppose we want to read lines from a file and strip any leading or trailing whitespace.
with open('example.txt', 'r') as file:
lines = [line.strip() for line in file]
print(lines)
Example: Extracting Specific Data
Let's say we have a list of dictionaries representing people and we want to extract their names.
people = [
{'name': 'Alice', 'age': 30},
{'name': 'Bob', 'age': 25},
{'name': 'Charlie', 'age': 35},
]
names = [person['name'] for person in people]
print(names)
The result is ['Alice', 'Bob', 'Charlie']
.
Performance Considerations
List comprehensions are not just more readable; they are often more efficient than traditional loops. This is because they are optimized for Python's internal implementation.
Example: Timing List Comprehensions vs. Loops
Let's compare the performance of list comprehensions and traditional loops using the timeit
module.
import timeit
# List comprehension
comprehension_time = timeit.timeit('[i ** 2 for i in range(1000)]', number=1000)
# Traditional loop
loop_time = timeit.timeit('''
squares = []
for i in range(1000):
squares.append(i ** 2)
''', number=1000)
print(f"List Comprehension Time: {comprehension_time}")
print(f"Loop Time: {loop_time}")
Generally, you will find that list comprehensions are faster, though the exact difference can depend on the specific use case and data size.
Conclusion
List comprehensions are a powerful feature in Python that can make your code more readable and efficient. By understanding and utilizing their full potential, you can write cleaner and more Pythonic code. From basic examples to more complex use cases, list comprehensions offer a versatile tool for any Python programmer.
And remember, while list comprehensions can make your code shorter, they should also make it clearer. If you find that a list comprehension is becoming too complex, it might be a good idea to refactor it into a function or a traditional loop for the sake of readability.
If you found this lesson interesting and rewarding please give it a like and follow me.If you have any questions please comment on the post.If you want to follow me on linkedin then go to this link and follow or even connect with me.I would be happy to discuss anything related to python.
Happy coding!
Subscribe to my newsletter
Read articles from Pravesh Chapagain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
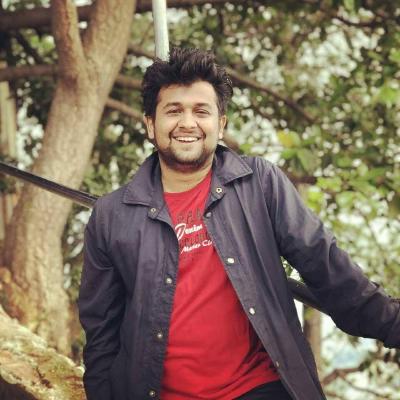