Understanding Null, Undefined, and Undeclared in JavaScript
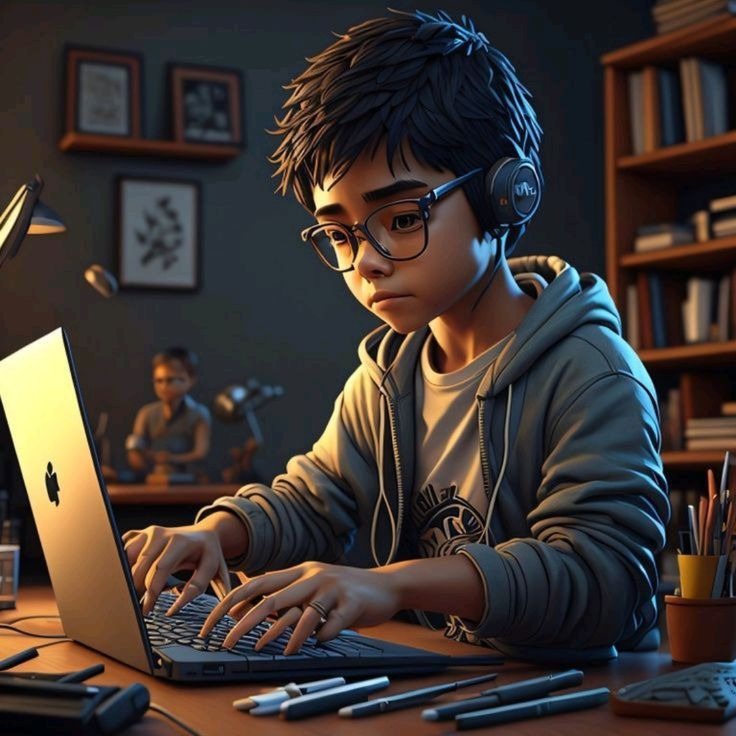
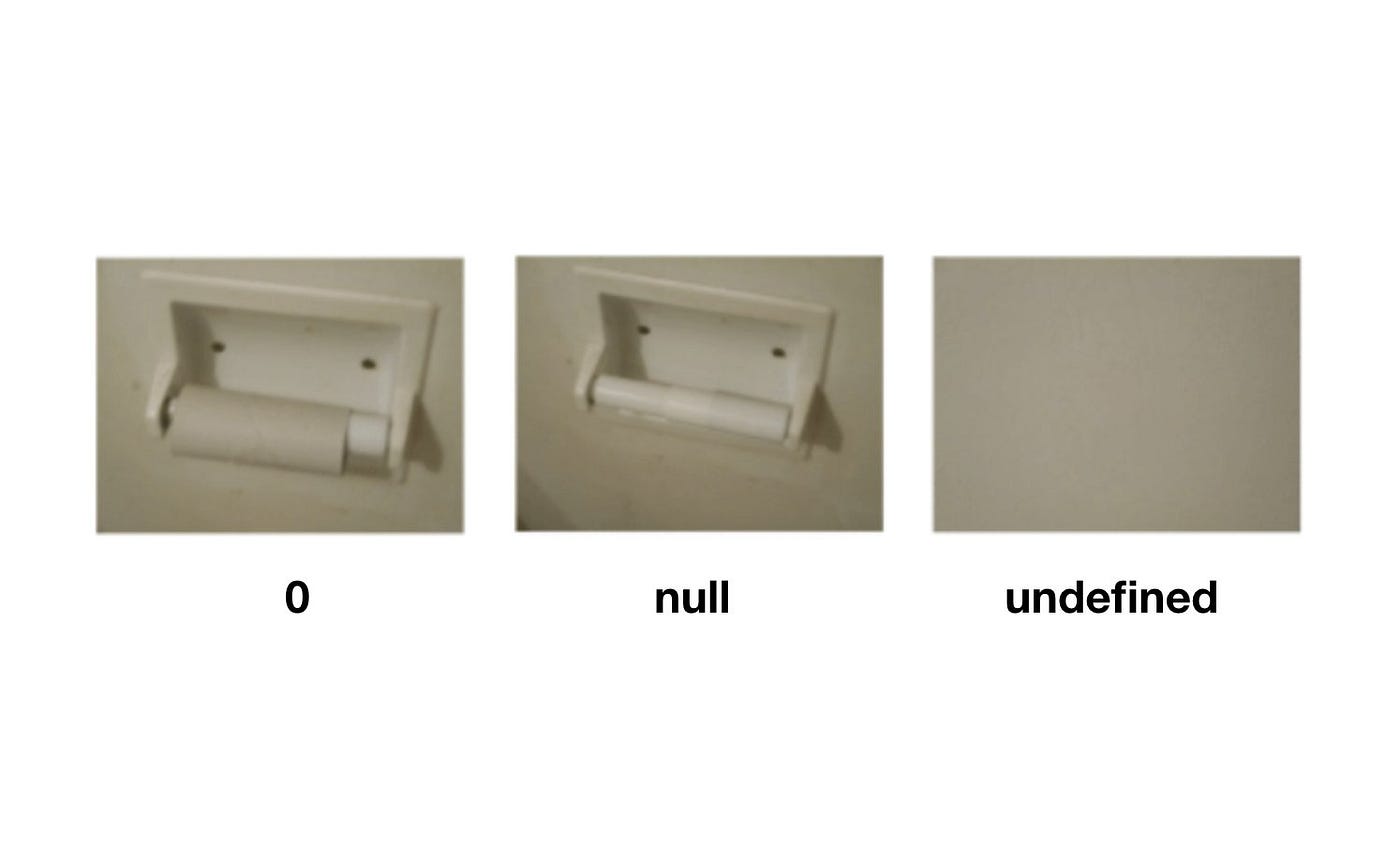
JavaScript is a versatile and dynamic language, but it can sometimes be confusing, especially when dealing with null
, undefined
, and undeclared
variables. Understanding the differences between these three concepts is crucial for writing clean and bug-free code. In this blog, we'll explore the definitions, differences, and use cases for null
, undefined
, and undeclared
in JavaScript.
1. Understanding undefined
Definition: undefined
is a primitive value automatically assigned to variables that have been declared but not initialized with any value.
Example:
let x;
console.log(x); // Output: undefined
Key Points:
A variable declared but not assigned a value will have the value
undefined
.Functions return
undefined
if no return value is specified.Accessing a non-existent object property results in
undefined
.
Use Case:
- Use
undefined
to indicate that a variable has not been initialized or to check if a function parameter was provided.
2. Understanding null
Definition: null
is an assignment value that represents the intentional absence of any object value. It's one of JavaScript's primitive values.
Example:
let y = null;
console.log(y); // Output: null
Key Points:
null
must be explicitly assigned to a variable.null
is used to explicitly indicate "no value".The type of
null
is "object" (this is a quirk of JavaScript).
Use Case:
- Use
null
to explicitly indicate that a variable should be empty or have no value.
3. Understanding undeclared
Definition: undeclared
variables are those that have not been declared in any scope.
Example:
console.log(z); // ReferenceError: z is not defined
Key Points:
Accessing an undeclared variable results in a
ReferenceError
.Using
let
orconst
without declaring a variable is a common source ofReferenceError
.
Use Case:
- Avoid using undeclared variables. Always declare variables using
let
,const
, orvar
.
Differences at a Glance
Aspect | undefined | null | undeclared |
Definition | Default value for uninitialized variables | Explicitly assigned to indicate no value | Variables not declared |
Assignment | Automatic | Manual | N/A |
Type | undefined | object | N/A |
When to Use | Uninitialized state | Explicitly no value | Avoid using |
Error Handling | Safe to access | Safe to access | Throws ReferenceError when accessed |
Practical Examples
Let's see some practical examples to solidify our understanding:
Example with undefined
:
function greet(name) {
console.log(`Hello, ${name}`);
}
greet(); // Output: Hello, undefined
In this case, name
is undefined
because no argument was passed to the function.
Example with null
:
let person = {
name: 'Deepak',
age: null
};
console.log(person.age); // Output: null
Here, age
is explicitly set to null
, indicating that the age is intentionally left empty.
Example with undeclared
:
function showMessage() {
console.log(message);
}
showMessage(); // ReferenceError: message is not defined
Since message
is not declared within the function or globally, accessing it results in a ReferenceError
.
Conclusion
Understanding the differences between null
, undefined
, and undeclared
variables is essential for effective JavaScript programming. undefined
signifies uninitialized variables, null
explicitly denotes the absence of a value, and undeclared
indicates variables that have not been declared in the scope. By using these concepts appropriately, you can write more robust and maintainable JavaScript code.
Thought-Provoking Questions
How can you leverage strict mode (
'use strict'
) to avoid issues with undeclared variables?What are some common debugging techniques for handling
undefined
andnull
values in large codebases?How do different JavaScript frameworks and libraries handle
null
andundefined
values, and what best practices can be adopted from them?
By mastering these fundamental concepts, you'll enhance your JavaScript skills and be better prepared to tackle more advanced programming challenges.
Feel free to share your thoughts and experiences with null
, undefined
, and undeclared
variables in the comments below! Happy coding!
Subscribe to my newsletter
Read articles from Raaj Aryan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
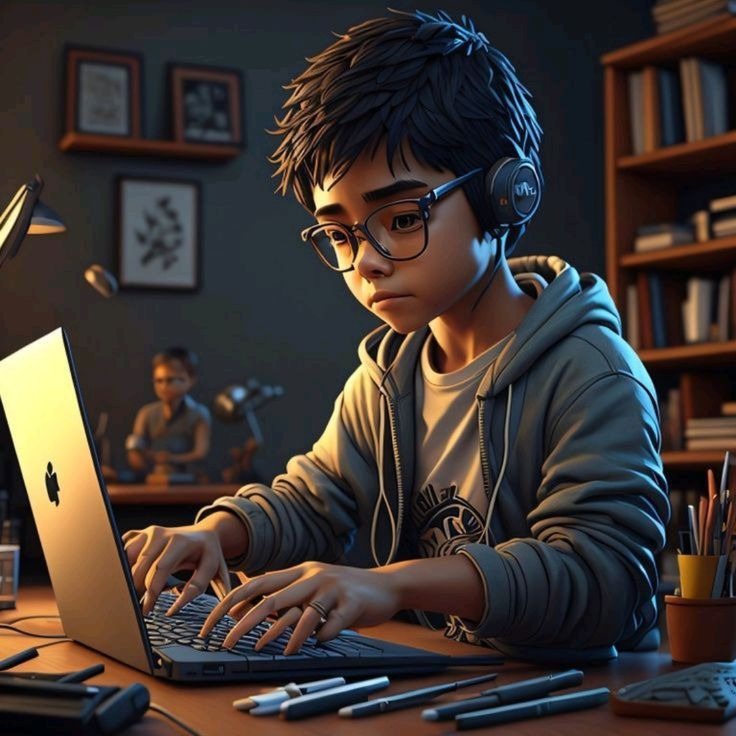
Raaj Aryan
Raaj Aryan
MERN Stack Developer • Open Source Contributor • DSA With Java • Freelancer • Youtuber • Problem-solving •