JS Constructor , Inhiritance , Polymorphism.
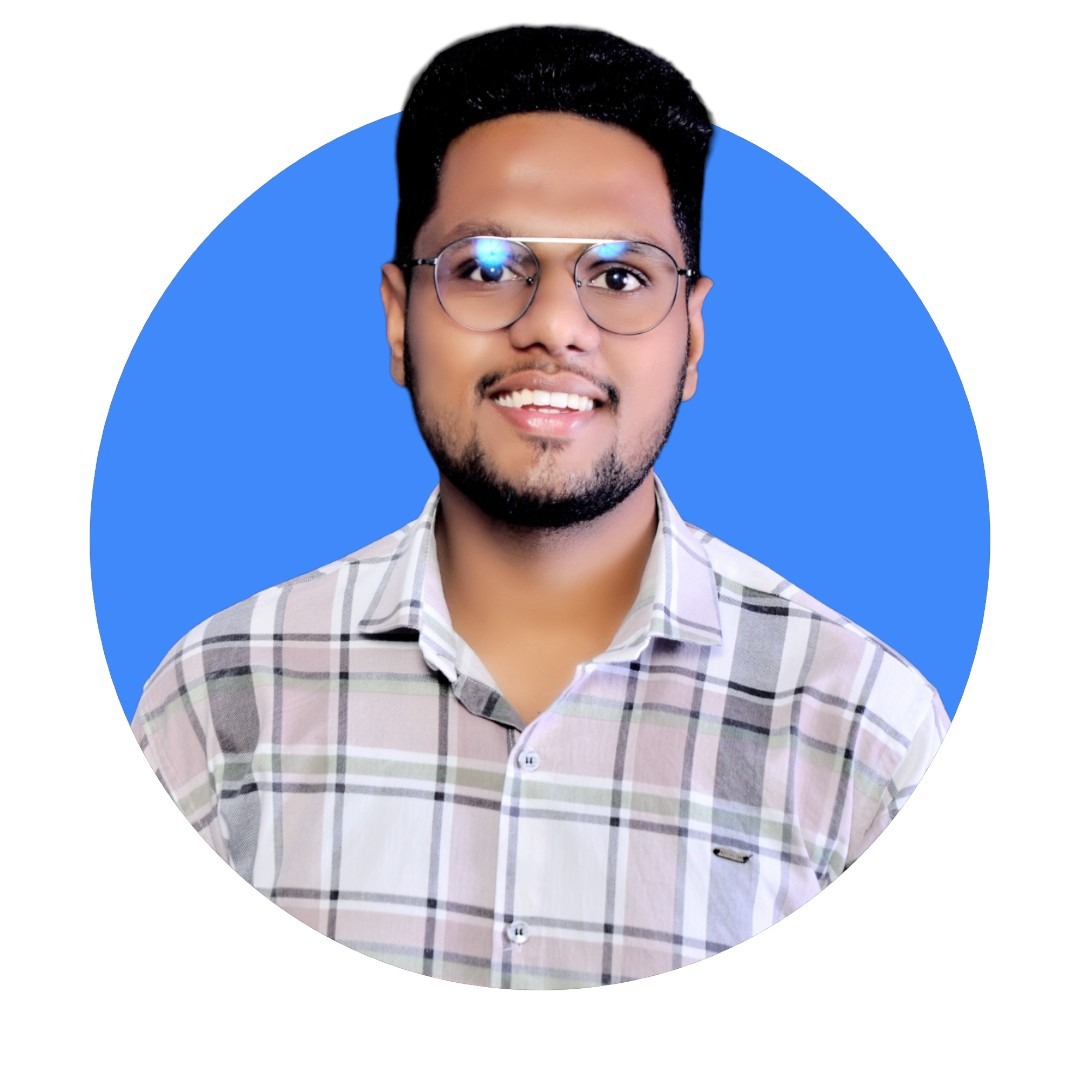
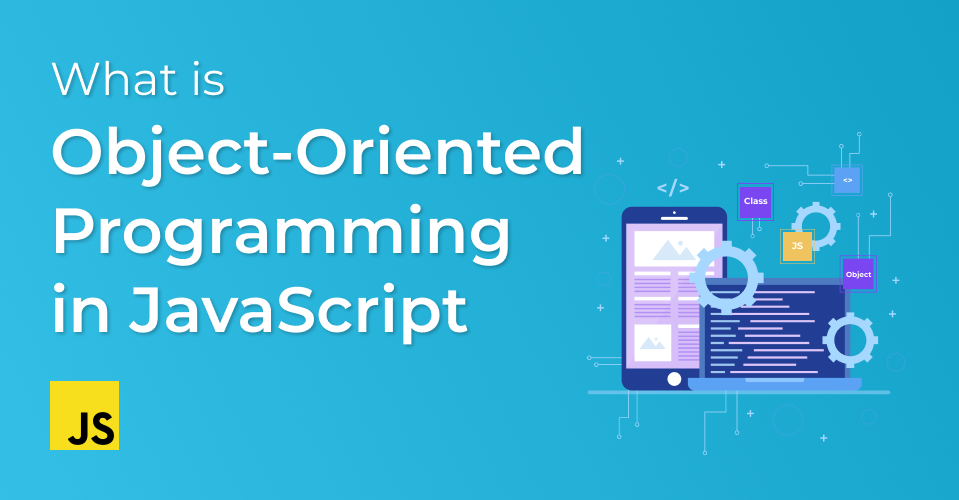
JavaScript Constructor :
A JavaScript constructor method is a special type of method
Constructor is used to initialize and create an object.
It is called when memory is allocated for an object.
Points to remember :
The constructor keyword is used to declare a constructor method.
The class can contain one constructor method only.
JavaScript allows us to use parent class constructor through super keyword.
Example:
<!DOCTYPE html>
<html>
<body>
<script>
class Employee {
constructor() {
this.id=101;
this.name = "Martin Roy";
}
}
var emp = new Employee();
document.writeln(emp.id+" "+emp.name);
</script>
</body>
</html>
Output:
101 Martin Roy
Advantages of Constructors in JavaScript
Encapsulation:
- Constructors allow you to encapsulate and initialize object properties, ensuring that each instance of the object has its own set of properties.
Code Reusability:
- Constructors promote code reuse by allowing you to create multiple instances of an object with the same properties and methods.
Inheritance:
- Using the class syntax and extends keyword, constructors facilitate inheritance, allowing you to create a hierarchical class structure and reuse code across different classes.
Initialization Logic:
- Constructors enable you to include complex initialization logic when an object is created, ensuring that the object is in a consistent state.
Cleaner Syntax with ES6 Classes:
- The introduction of the class keyword in ES6 (ECMAScript 2015) provides a cleaner and more intuitive syntax for creating constructors, making the code more readable and maintainable.
Method Binding:
- Methods defined within a class using a constructor are automatically bound to the instance, avoiding issues related to the this keyword.
Disadvantages of Constructors in JavaScript
Memory Usage:
- If methods are defined within the constructor function, they are recreated for every instance of the object, leading to increased memory usage. This can be mitigated by using prototypes for method definitions.
Complexity:
- Constructors can add complexity to the code, especially when dealing with inheritance and the super keyword, making it harder to debug and maintain.
Performance Overhead:
- The use of constructors and the new keyword can introduce performance overhead, especially in performance-critical applications. This overhead comes from the creation and initialization of objects.
Learning Curve:
- Understanding how constructors work, especially with the new ES6 class syntax and inheritance, can have a steep learning curve for beginners.
Inflexibility:
- Once an object is created using a constructor, its structure is relatively fixed. Modifying the object's structure after creation can be less straightforward compared to using plain objects.
JavaScript Inheritance :
The JavaScript inheritance is a mechanism that allows us to create new classes on the basis of already existing classes.
It provides flexibility to the child class to reuse the methods and variables of a parent class.
The JavaScript extends keyword is used to create a child class on the basis of a parent class.
It facilitates child class to acquire all the properties and behavior of its parent class.
Points to remember :
It maintains an IS-A relationship.
The extends keyword is used in class expressions or class declarations.
Using extends keyword, we can acquire all the properties and behavior of the inbuilt object as well as custom classes.
We can also use a prototype-based approach to achieve inheritance.
Example:
<!DOCTYPE html>
<html>
<body>
<script>
class Bike
{
constructor()
{
this.company="Honda";
}
}
class Vehicle extends Bike {
constructor(name,price) {
super();
this.name=name;
this.price=price;
}
}
var v = new Vehicle("Shine","70000");
document.writeln(v.company+" "+v.name+" "+v.price);
</script>
</body>
</html>
Output:
Honda Shine 70000
Prototypal Inheritance
JavaScript's inheritance system is based on prototypes. Each object in JavaScript has an internal link to another object called its prototype. When you try to access a property or method on an object, JavaScript will look for it on the object itself first, and if it doesn't find it, it will look at the object's prototype, and so on up the prototype chain.
Example:
<script>
//Constructor function
function Bike(company)
{
this.company=company;
}
Bike.prototype.getCompany=function()
{
return this.company;
}
//Another constructor function
function Vehicle(name,price) {
this.name=name;
this.price=price;
}
var bike = new Bike("Honda");
Vehicle.prototype=bike; //Now Bike treats as a parent of Vehicle.
var vehicle=new Vehicle("Shine",70000);
document.writeln(vehicle.getCompany()+" "+vehicle.name+" "+vehicle.price);
</script>
Output:
Honda Shine 70000
Advantages of Inheritance in JavaScript
Code Reusability:
- Inheritance allows you to reuse existing code. You can define a base class with common functionality and create derived classes that extend the base class, adding or overriding functionality as needed.
Modularity:
- It promotes modular design. Common functionality can be abstracted into base classes, and specific functionality can be added to derived classes, making the codebase more organized and maintainable.
Ease of Maintenance:
- With inheritance, updates to common functionality need to be made in only one place (the base class). This can reduce the chances of bugs and inconsistencies.
Clear Hierarchical Relationships:
- Inheritance can model real-world relationships and hierarchies, making it easier to understand and reason about the structure and behavior of the system.
Polymorphism:
- Inheritance supports polymorphism, allowing objects of different classes to be treated as objects of a common superclass. This enables the design of flexible and extensible systems.
Disadvantages of Inheritance in JavaScript
Complexity:
- Inheritance can introduce complexity into the codebase. Understanding and debugging code that involves multiple levels of inheritance can be challenging, especially in large applications.
Tight Coupling:
- Inheritance creates a tight coupling between the base class and derived classes. Changes to the base class can inadvertently affect all derived classes, potentially introducing bugs.
Inflexibility:
- Once a class hierarchy is established, it can be difficult to change. Adding new functionality might require significant refactoring if the existing hierarchy doesn't accommodate it well.
Overhead:
- Inheritance can introduce performance overhead. Each object in the inheritance chain may need to be traversed to find a property or method, which can slow down the application.
Misuse and Overuse:
- Inheritance can be misused or overused, leading to inappropriate class hierarchies and fragile code. Not all relationships should be modeled using inheritance; sometimes composition or other design patterns are more suitable.
Difficulty in Understanding:
- New developers may find it difficult to understand deep inheritance hierarchies, especially if they involve multiple levels and extensive use of method overriding.
JavaScript Polymorphism :
The polymorphism is a core concept of an object-oriented paradigm
that provides a way to perform a single action in different forms.
It provides an ability to call the same method on different JavaScript objects.
As JavaScript is not a type-safe language, we can pass any type of data members with the methods.
Example:
Let's see an example where a child class object invokes the parent class method.
<!DOCTYPE html>
<html>
<body>
<script>
class A
{
display()
{
document.writeln("A is invoked");
}
}
class B extends A
{
}
var b=new B();
b.display();
</script>
</body>
</html>
Output:
A is invoked
Advantages of Polymorphism in JavaScript
Code Reusability:
- Polymorphism promotes code reuse by allowing you to write generic code that can work with objects of different types. This reduces code duplication and improves maintainability.
Flexibility and Extensibility:
- Polymorphic code is more flexible and easier to extend. New classes can be added with minimal changes to existing code, as long as they conform to the expected interface or superclass.
Simplified Code:
- Polymorphism can simplify complex conditional logic by allowing you to define different behaviors for different object types through method overriding or interface implementation.
Decoupling:
- Polymorphism decouples client code from specific class implementations. This means that changes to one part of the system are less likely to affect other parts, promoting a more modular architecture.
Dynamic Binding:
- Methods can be dynamically bound at runtime, allowing for more dynamic and flexible code execution. This is particularly useful in scenarios where the exact type of objects is determined at runtime.
Enhanced Readability and Maintainability:
- By allowing objects to be treated uniformly, polymorphism can make code easier to read and maintain. It clarifies the intent of the code, as behaviors are abstracted to a higher level.
Disadvantages of Polymorphism in JavaScript
Performance Overhead:
- Polymorphism can introduce performance overhead due to dynamic method resolution and the potential need for additional indirection.
Complexity:
- While polymorphism can simplify code in some respects, it can also introduce complexity, especially when overused or when the hierarchy of polymorphic types becomes deep and difficult to manage.
Debugging Difficulties:
- Polymorphic code can be harder to debug and trace because the actual method that gets executed might be determined at runtime, making it harder to follow the program flow.
Learning Curve:
- Understanding and correctly implementing polymorphism requires a solid grasp of object-oriented principles, which can present a steep learning curve for beginners.
Potential for Misuse:
- Polymorphism can be misused, leading to poor design choices. For example, creating overly complex class hierarchies or using polymorphism in situations where simpler solutions would suffice.
Whats Next ?
Javascript Object Such As Date ,Math, Number,Boolean.
Subscribe to my newsletter
Read articles from Aditya Gadhave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
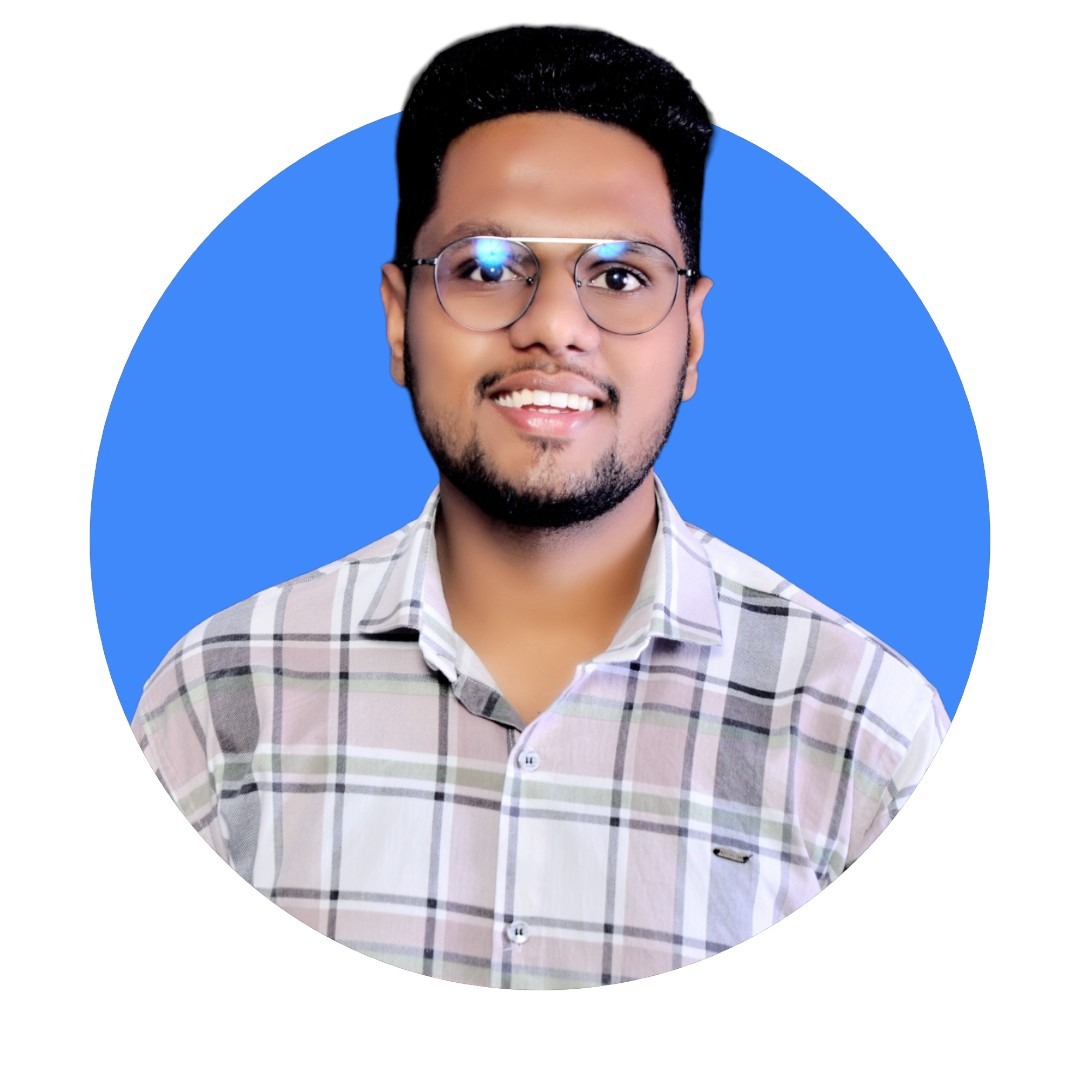
Aditya Gadhave
Aditya Gadhave
๐ Hello! I'm Aditya Gadhave, an enthusiastic Computer Engineering Undergraduate Student. My passion for technology has led me on an exciting journey where I'm honing my skills and making meaningful contributions.