What is Database Sharding and How Does It Work?

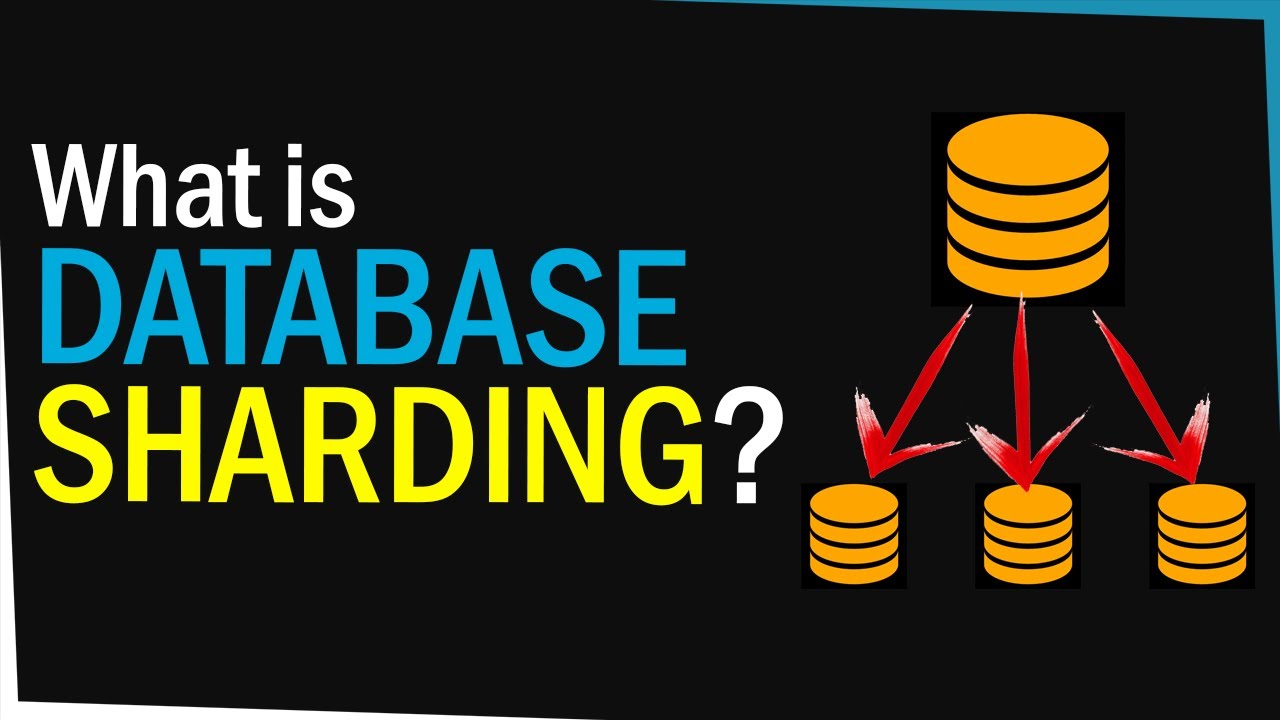
In today's data-driven world, modern applications face the ever-growing challenge of managing massive volumes of information. Traditional monolithic databases struggle with bottlenecks, leading to sluggish performance and limited scalability. Enter database sharding—a powerful solution designed to enhance scalability and boost performance by distributing data across multiple shards.
What is Database sharding?
Database sharding is a technique for horizontal scaling of databases, where the data is split across multiple database instances, or shards, to improve performance and reduce the impact of large amounts of data on a single database. (ref: Database Sharding)
Each shard is essentially a separate database instance or server that contains a subset of the overall data. The goal of sharding is to distribute the data and database load across multiple shards, allowing for improved scalability, performance, and fault tolerance in a distributed system.
Key components of sharding:
Shard Key: This is the attribute used to distribute data across different shards. It's like a label that determines which shard a particular piece of data belongs to. Choosing the right shard key is crucial for even data distribution and efficient querying. Common shard keys include user ID, product category, or date.
Shards: These are individual databases that hold a subset of the overall data. Imagine a large library divided into multiple sections - each section (shard) focuses on a specific category of books. The total data is distributed across these shards based on the shard key.
Shard Map (or Catalog): This acts like a directory, keeping track of which shard stores data for a specific shard key value. When your application needs to access data, it consults the shard map to locate the appropriate shard. Think of it as a library card catalogue that tells you which section (shard) to find a particular book (data) based on its title or author (shard key).
Shard Router: This is a component within your application or a separate service that interacts with the shard map. It receives the shard key from your application logic and uses the shard map to determine the appropriate shard for the desired operation (read or write). The shard router then directs the request to the specific shard.
Benefits of Sharding
Enhanced Scalability: Sharding allows for horizontal scaling by distributing data across multiple databases (shards). As data volume increases, additional shards can be seamlessly integrated, ensuring the application can accommodate growth without performance sacrifices.
Improved Performance: Sharding optimizes query execution by enabling queries to target specific data subsets within individual shards. This reduces the amount of data scanned, resulting in significantly faster response times and a more responsive user experience.
Increased Availability: Sharding introduces a degree of fault tolerance. If one shard encounters an issue, other shards remain unaffected, mitigating the impact on overall system availability. This enhances application resilience and ensures service continuity.
Implementation in Node.js
Now that we have a foundational understanding of database sharding and its benefits, let's dive into a practical implementation example using Node.js and MongoDB.
Create Two Shards (Database Instances)
In this example, we have created two database instances, both hosted on Atlas. You can also use Docker or self-host if you prefer.
Define the shard map
To define the shard map, create a simple JavaScript object that maps product categories to their respective shard connection strings.
const shardMap = {
'Clothing': 'database url for clothing products',
'Electronics': 'database url for electronics products',
'default': 'database url for all other products'
}
Define the function that returns the proper shard based on the category
Create a function that takes a product category as input and returns the appropriate shard connection.
// Fetch the right shard
const fetchShard = (category) => {
// get shard credentials
const credentials = shardMap?.[category]
// connect with the server
return new MongoClient(credentials ?? shardMap?.default);
}
Define the API routes for uploading products
Set up an API route to handle product uploads. This route will use the fetchShard
function to determine the correct shard for storing the product.
app.post('/products', async (req, res) => {
const {products} = req.body;
for (const product of products) {
// get database connection for each product
const client = await fetchShard(product?.category)
const db = client.db('database-sharding')
const productCollection = db.collection("products");
await productCollection.insertOne(product)
}
res.send("ok")
})
Define the API route that returns products based on category
Set up an API route to fetch products by category. This route will also use the fetchShard
function to determine the correct shard to query.
app.get('/products/:category', async (req, res) => {
const {category} = req.params;
try {
// find out the correct shard
const client = await fetchShard(category)
// connect to the database
const db = client.db('database-sharding')
const productCollection = db.collection("products");
const products = await productCollection.find({category}).toArray();
res.send(products)
} catch (err) {
console.log(err)
res.status(500).json({message: 'Error fetching products'});
}
});
Test out our application
To test the application, you can use tools like Postman or Curl to send HTTP requests to the API endpoints. For example:
To upload products:
To fetch products by category:
Considerations and Best Practices for Sharding
Database sharding offers significant advantages in terms of scalability and performance, but it's important to be aware of its complexities and best practices for successful implementation:
Increased Application Complexity: Sharding introduces an additional layer of abstraction between your application and the database. You'll need to manage shard routing, shard key selection, and potential consistency issues across shards.
Shard Key Selection: Choosing the right shard key is critical. It should evenly distribute data across shards and align with your most frequent query patterns. A poorly chosen shard key can lead to bottlenecks and negate the benefits of sharding.
Scalability Considerations: While sharding enables horizontal scaling, it's not a magic bullet. Adding new shards comes with its own management overhead. Evaluate your data access patterns and growth projections to determine if sharding is the most suitable solution for your specific needs.
By understanding the benefits and considerations of sharding, you can make informed decisions to optimize your Node.js applications for scalability and performance as your data demands continue to evolve. Remember, sharding is a powerful tool, but like any powerful tool, it requires careful planning and execution to reap the maximum rewards.
Subscribe to my newsletter
Read articles from Gaurav Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Gaurav Kumar
Gaurav Kumar
Hey! I'm Gaurav, a tech writer who's all about MERN, Next.js, and GraphQL. I love breaking down complex concepts into easy-to-understand articles. I've got a solid grip on MongoDB, Express.js, React, Node.js, and Next.js to supercharge app performance and user experiences. And let me tell you, GraphQL is a game-changer for data retrieval and manipulation. I've had the opportunity to share my knowledge through tutorials and best practices in top tech publications. My mission? Empowering developers and building a supportive community. Let's level up together with my insightful articles that'll help you create top-notch applications!