Understanding Dockerfiles
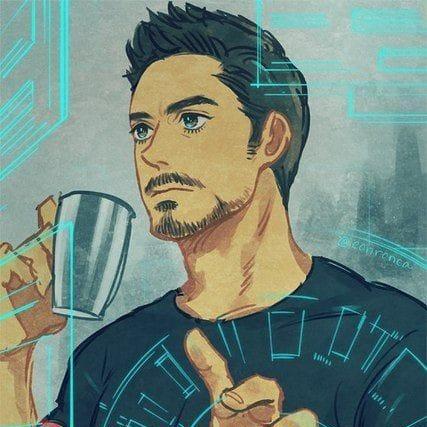
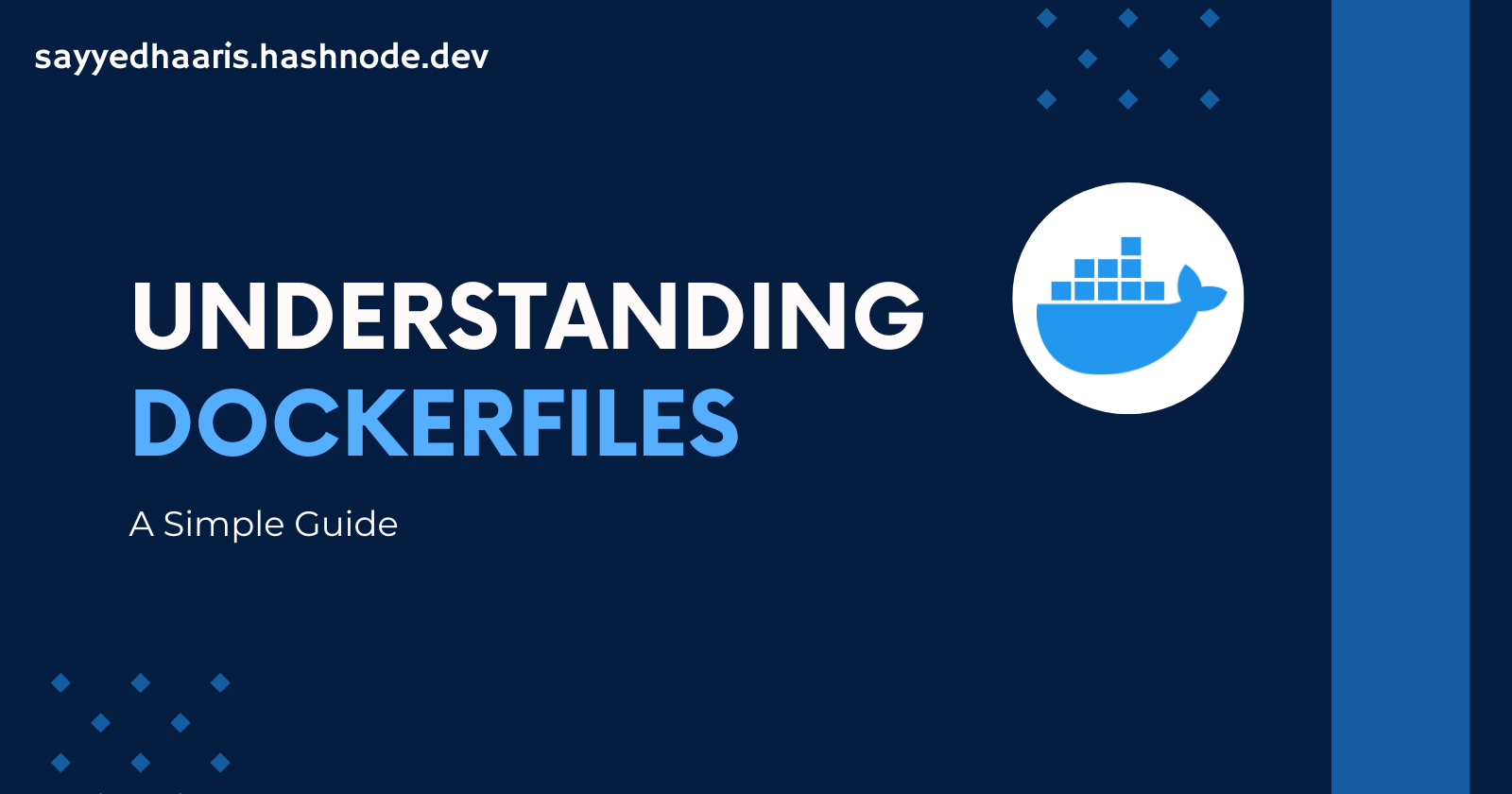
In the world of containerization, Docker stands out as a powerful tool for automating the deployment of applications inside lightweight, portable containers. At the heart of Docker's containerization process lies the Dockerfile, a simple text file that contains a series of instructions to build a Docker image. Understanding Dockerfiles is crucial to leveraging the full potential of Docker.
What is a Dockerfile?
A Dockerfile is a script that contains a list of commands that Docker uses to assemble an image. The instructions within the Dockerfile specify the base image, application dependencies, configuration settings, and the command to run the application. By defining these steps, a Dockerfile enables the creation of consistent and reproducible Docker images.
Dockerfile Instructions
Below is an explanation of each Dockerfile instruction along with examples:
ADD: Adds files, directories, or remote files to the image.
ADD local-file.txt /path/in/container/ ADD http://example.com/file.zip /path/in/container/
ARG: Defines a variable that users can pass at build-time to the builder with the
docker build
command.ARG VERSION=1.0 FROM ubuntu:${VERSION}
CMD: Specifies the default command that should run when a container is started.
CMD ["executable","param1","param2"] CMD ["echo","Hello, World!"]
COPY: Copies new files or directories from
src
and adds them to the filesystem of the container at the pathdest
.COPY local-file.txt /path/in/container/ COPY . /app
ENTRYPOINT: Configures a container that will run as an executable.
ENTRYPOINT ["executable","param1","param2"] ENTRYPOINT ["python","app.py"]
ENV: Sets the environment variable
key
to the valuevalue
.ENV MY_VAR my_value ENV PATH /my/custom/path:$MY_VAR
EXPOSE: Informs Docker that the container listens on the specified network ports at runtime.
EXPOSE 80 EXPOSE 8080
FROM: Sets the base image for subsequent instructions.
FROM ubuntu:20.04 FROM python:3.9
HEALTHCHECK: Tells Docker how to test a container to check that it is still working.
HEALTHCHECK --interval=30s --timeout=10s \ CMD curl -f http://localhost/ || exit 1
LABEL: Adds metadata to an image.
LABEL version="1.0" LABEL description="This is my Docker image"
MAINTAINER: Specifies the author of the image.
MAINTAINER Your Name <your-email@example.com>
ONBUILD: Adds a trigger instruction to the image that will be executed later when the image is used as the base for another build.
ONBUILD ADD . /app/src ONBUILD RUN /usr/local/bin/python-build --dir /app/src
RUN: Executes any commands in a new layer on top of the current image and commits the results.
RUN apt-get update && apt-get install -y python3 RUN mkdir -p /app && chown user:user /app
SHELL: Sets the default shell for the shell form of commands.
SHELL ["/bin/bash", "-c"]
STOPSIGNAL: Sets the system call signal that will be sent to the container to exit.
STOPSIGNAL SIGKILL
USER: Sets the user name or UID to use when running the image and for any RUN, CMD, and ENTRYPOINT instructions.
USER myuser USER 1001
VOLUME: Creates a mount point with the specified path and marks it as holding externally mounted volumes from native host or other containers.
VOLUME ["/data"] VOLUME /var/lib/mysql
WORKDIR: Sets the working directory for any
RUN
,CMD
,ENTRYPOINT
,COPY
, andADD
instructions that follow it in the Dockerfile.WORKDIR /app
Example Dockerfile
Let's write a Dockerfile for a simple Java program that prints "Hello, World!".
1: Create the Java Program
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
2: Create the Dockerfile
# Use a base image with OpenJDK 17
FROM openjdk:17
# Metadata
LABEL version="1.0"
LABEL description="A simple Java program Docker image"
LABEL maintainer="Your Name <your-email@example.com>"
# Set environment variables
ENV APP_HOME=/usr/src/app
# Create app directory and set working directory
WORKDIR $APP_HOME
# Copy Java program to the container
COPY HelloWorld.java .
# Compile the Java program
RUN javac HelloWorld.java
# Set the default user (optional, requires you to add the user in RUN)
RUN useradd -ms /bin/bash appuser
USER appuser
# Change default shell (optional)
SHELL ["/bin/bash", "-c"]
# Entry point for the container
ENTRYPOINT ["java"]
# Default command to run when the container starts
CMD ["HelloWorld"]
# Stop signal
STOPSIGNAL SIGINT
3: Build and Run the Docker Image
$ docker build -t my-java-app .
$ docker run my-java-app
Best Practices for Dockerfiles
Choose the Right Base Image
Use official base images from trusted sources.
Prefer slim versions to reduce image size.
Specify version tags for consistency.
Minimize Layers
Combine commands in
RUN
statements to reduce layers.Order instructions to leverage Docker's build cache effectively.
Use Multi-Stage Builds
Separate build environments from runtime to keep images small.
Copy only necessary artifacts into the final image.
Optimize Image Size
Clean up package manager caches and unnecessary files.
Use
.dockerignore
to exclude unneeded files.
Use ENV for Configuration
Set environment variables for application configuration.
Allows flexibility without modifying Dockerfile.
Run as Non-Root User
- Enhances container security by running processes as non-root.
Document Exposed Ports
- Use
EXPOSE
to document which ports are intended to be published.
- Use
Implement HEALTHCHECK
- Define a
HEALTHCHECK
to monitor container health.
- Define a
Clean Up Intermediate Images
- Remove unused images and containers to free up disk space.
Conclusion
Dockerfiles provide a powerful way to automate the creation of Docker images, ensuring your applications run consistently across different environments. By mastering Dockerfiles, you can optimize your build processes, improve deployment consistency, and take full advantage of Docker's capabilities. Whether you are containerizing a simple Java application or a complex microservices architecture, understanding and utilizing Dockerfiles effectively is a critical skill in modern software development.
Happy containerizing!
Subscribe to my newsletter
Read articles from Haaris Sayyed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
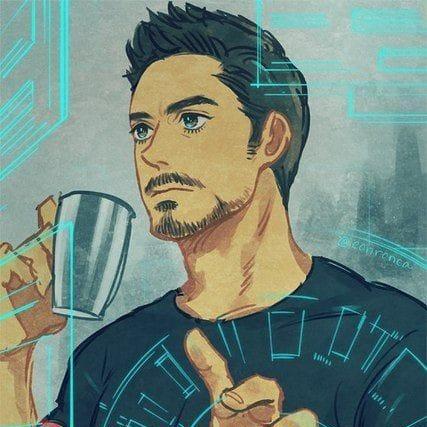