Mastering Asynchronous JavaScript: A Comprehensive Guide to Building Responsive Web Apps
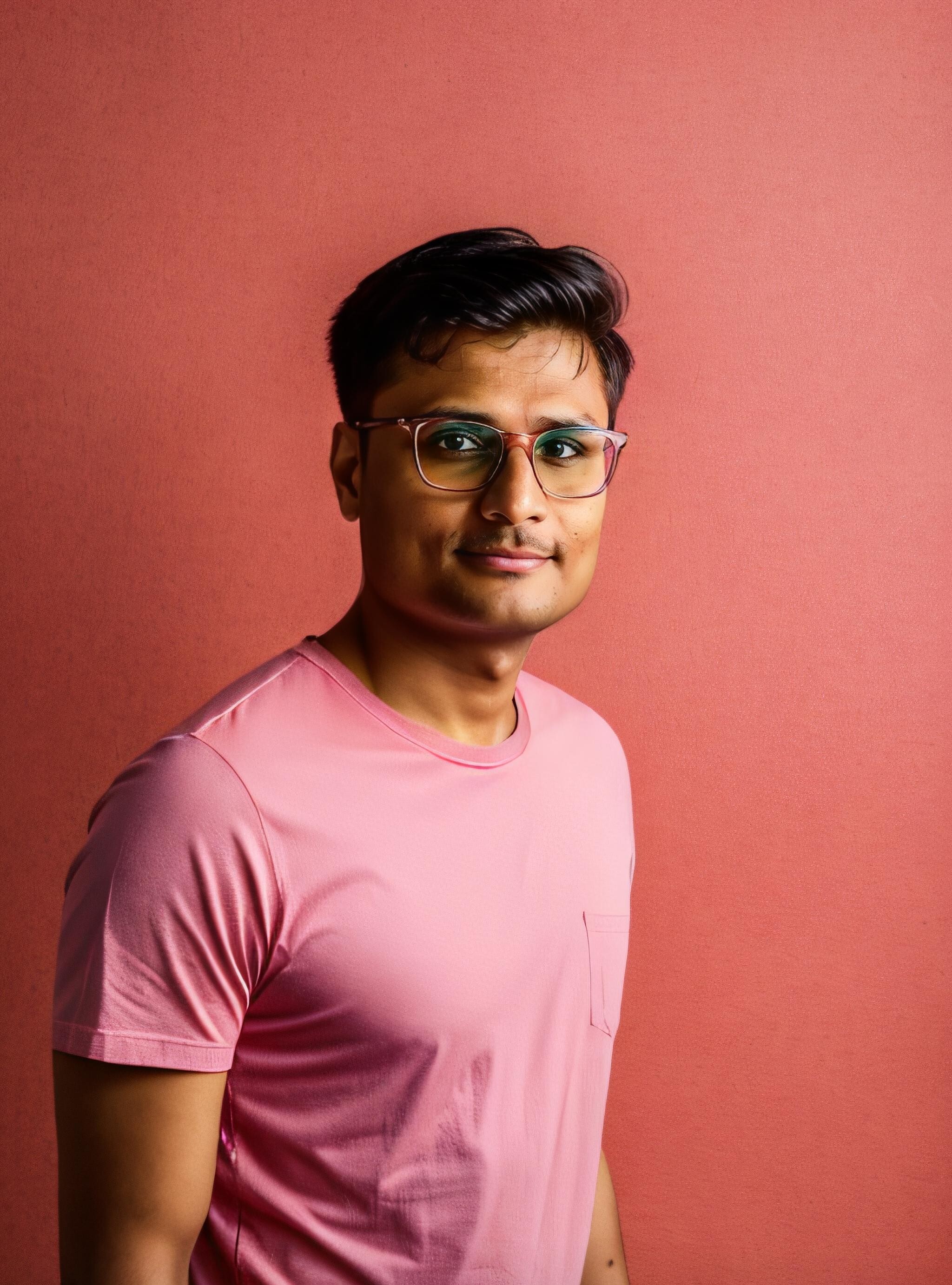
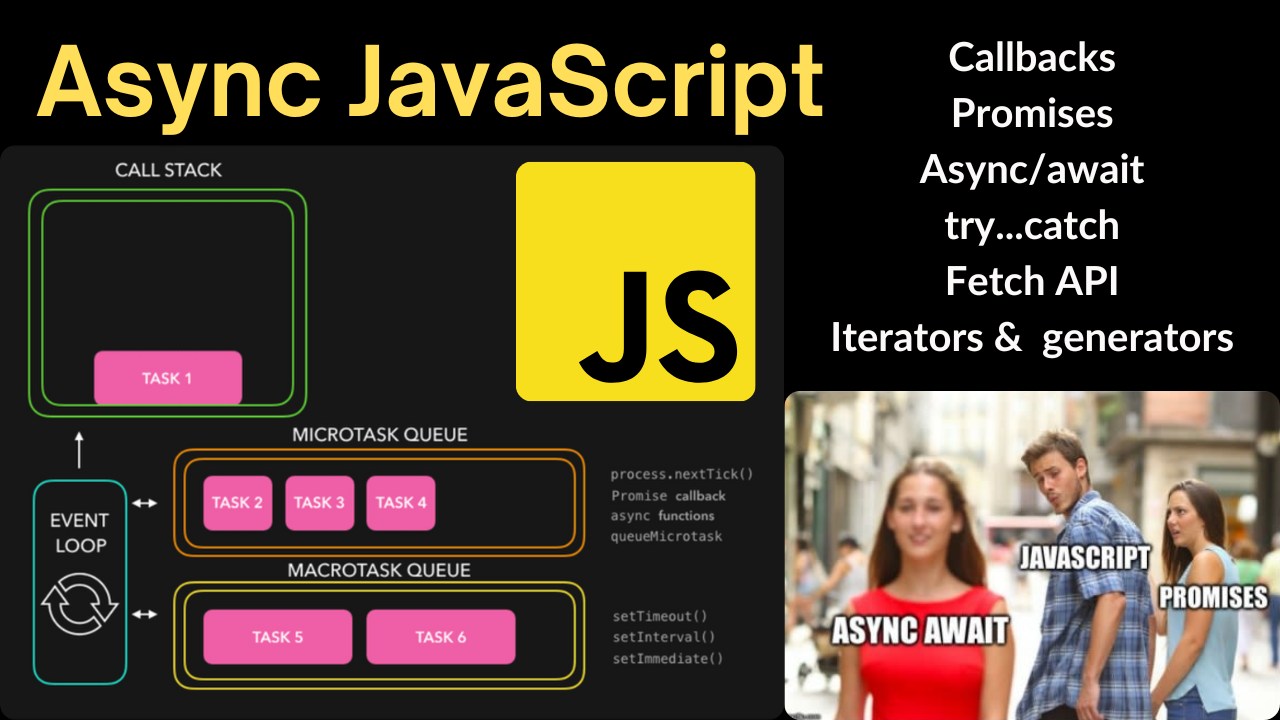
In this article, we’ll explore the world of asynchronous programming and learn how to handle non-blocking operations in JavaScript. We’ll cover the differences between synchronous and asynchronous code, callbacks, promises, async/await, error handling with try…catch, the Fetch API, and async iterators and generators.
Synchronous vs. Asynchronous Code
Synchronous code executes in a sequential manner, one operation at a time.
Asynchronous code allows operations to run in parallel, without blocking the main thread.
JavaScript is traditionally single-threaded, but asynchronous operations are essential for building responsive and efficient web applications.
Callbacks
Callbacks are functions passed as arguments to other functions, to be executed when an asynchronous operation completes.
They are the traditional way of handling asynchronous code in JavaScript.
function fetchData(callback) {
// Simulating an asynchronous operation
setTimeout(() => {
const data = { message: 'Hello, World!' };
callback(null, data);
}, 2000);
}
fetchData((error, data) => {
if (error) {
console.error(error);
} else {
console.log(data.message);
}
});
Promises
Promises are objects that represent the eventual completion or failure of an asynchronous operation.
They provide a more structured and readable way to handle asynchronous code.
function fetchDataPromise() {
return new Promise((resolve, reject) => {
// Simulating an asynchronous operation
setTimeout(() => {
const data = { message: 'Asynchronous data' };
resolve(data);
}, 2000);
});
}
fetchDataPromise()
.then(data => {
console.log(data.message);
})
.catch(error => {
console.error(error);
});
Fetch API
- The Fetch API is a modern and more straightforward way to make HTTP requests in JavaScript.
async function fetchDataWithFetchAPI() {
try {
const response = await fetch('https://dummyjson.com/todo/3');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
fetchDataWithFetchAPI();
In this example, we refactor the previous fetchData
function to use the modern Fetch API and make an HTTP GET request to https://api.example.com/data
. We handle the response asynchronously using async/await
and parse the JSON response.
Async/Await
Async/await is a modern syntax for working with promises in a more concise and synchronous-like manner.
The
async
keyword is used to define an asynchronous function that can use theawait
keyword to pause execution until a promise is resolved.
async function fetchDataAsync() {
const response = await fetch('<https://dummyjson.com/todo/1>');
const data = await response.json();
console.log(data);
}
fetchDataAsync();
Handling Errors with Try…Catch
The
try...catch
statement is used to handle errors in JavaScript, including errors thrown by asynchronous operations.The
try
block contains the code that may throw an error and thecatch
block contains the code to handle the error.
async function fetchTodo() {
try {
const response = await fetch('https://dummyjson.com/todo/2');
// Check if the response is successful
if (!response.ok) {
throw new Error(`HTTP error ${response.status}`);
}
const data = await response.json();
console.log(data);
} catch (error) {
// Handle any errors that occurred during the fetch or parsing
console.error('Error:', error);
}
}
fetchTodo();
In this example, we wrap the asynchronous fetch
operation and the parsing of the response in a try
block. If any error occurs during these operations, it will be caught in the catch
block, and we can handle the error accordingly (e.g., log the error message).
Async Iterators and Generators
Async iterators and generators are advanced concepts in JavaScript that allow you to work with asynchronous sequences of data.
They are particularly useful when dealing with streaming data or infinite data sources.
async function* generateNumbers() {
yield 1;
yield 2;
yield 3;
yield 4;
yield 5;
}
// Using an async iterator to consume the generator
async function consumeNumbers() {
const numberGenerator = generateNumbers();
for await (const num of numberGenerator) {
console.log(num);
}
}
consumeNumbers();
In this example, we define an async generator function generateNumbers
that yields a sequence of numbers asynchronously. We then create an async function consumeNumbers
that uses a for await...of
loop to consume the values generated by the async iterator.
Async iterators and generators are useful when working with streaming data or infinite data sources, as they allow you to handle the data in chunks asynchronously, without having to load the entire data set into memory at once.
You now have a solid understanding of asynchronous programming in Javascript. Keep practicing and experimenting with asynchronous JavaScript to solidify your knowledge.
Subscribe to my newsletter
Read articles from Vatsal Bhesaniya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
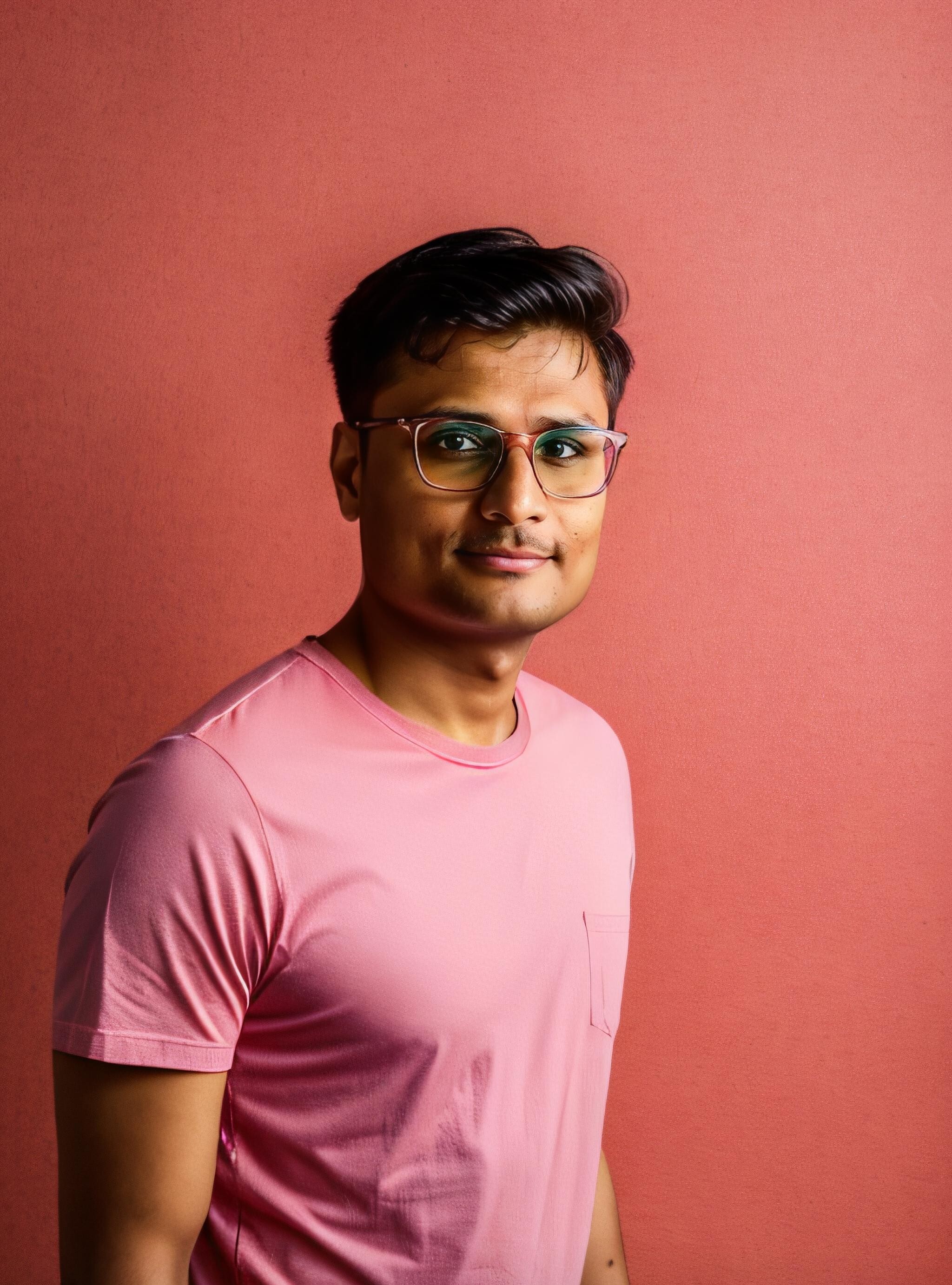