Comprehensive API Guidelines for Professional Development
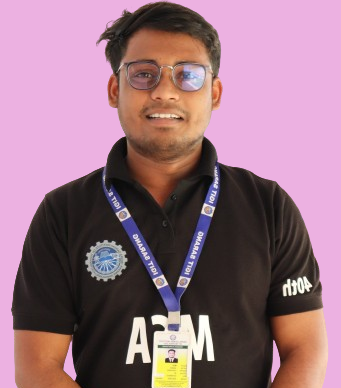
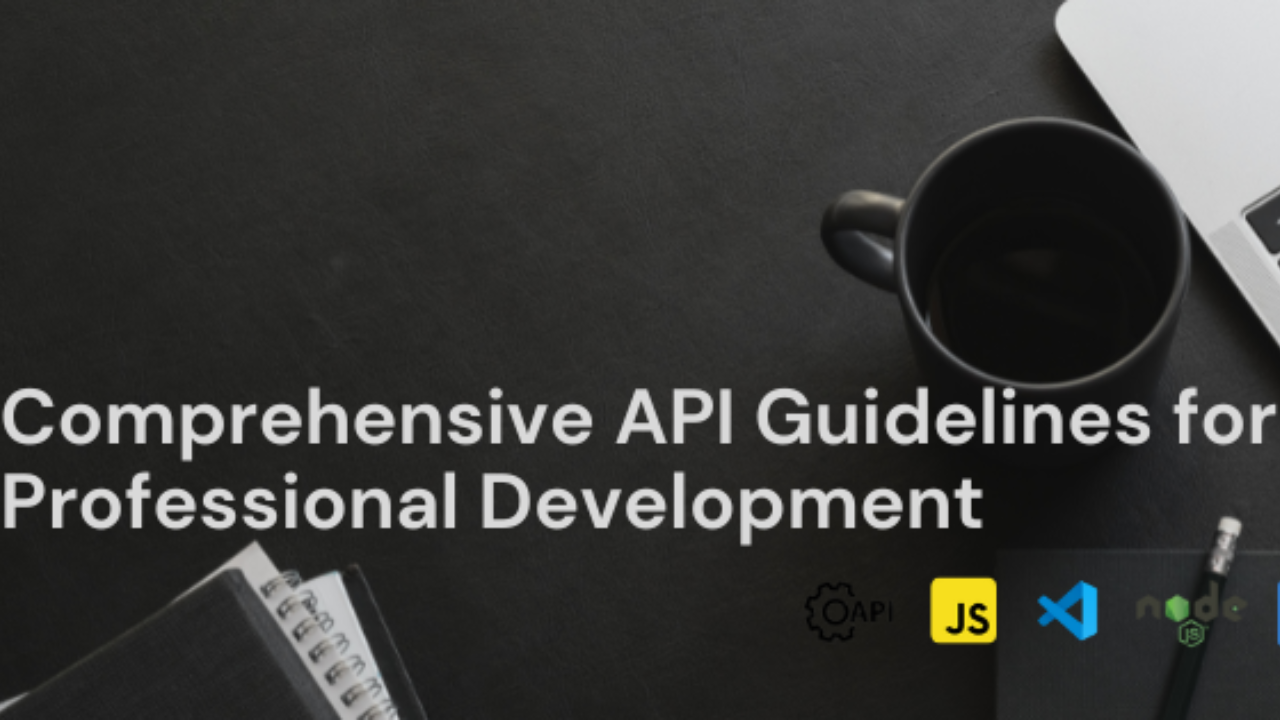
Introduction
In today's interconnected digital landscape, APIs (Application Programming Interfaces) play a critical role in enabling communication between different software systems. Whether you're developing a new API or working with existing ones, adhering to best practices ensures your APIs are reliable, secure, and easy to use. This article provides comprehensive guidelines for professional API development, focusing on design, implementation, documentation, and security.
API Design Principles
1. Consistency
Consistency is key to creating APIs that are easy to understand and use. Consistent naming conventions, endpoints, and response structures help developers quickly become familiar with your API.
Naming Conventions: Use clear and descriptive names for endpoints, parameters, and methods. For example, use
/users
to represent user-related operations.HTTP Methods: Follow RESTful conventions by using appropriate HTTP methods:
GET
for retrieving resources.POST
for creating resources.PUT
for updating resources.DELETE
for deleting resources.
2. Versioning
Version your API to ensure backward compatibility and smooth transitions when making changes.
URI Versioning: Include the version number in the API path, e.g.,
/api/v1/users
.Header Versioning: Alternatively, use request headers to specify the version.
3. Statelessness
APIs should be stateless, meaning each request from the client contains all the information the server needs to fulfill that request. This simplifies scalability and reliability.
4. Resource-Oriented Design
Design your API around resources rather than actions. Resources are typically nouns (e.g., users
, orders
), and actions are represented by HTTP methods.
API Implementation Best Practices
1. Proper Use of HTTP Status Codes
Use standard HTTP status codes to indicate the outcome of API requests:
200 OK: The request was successful.
201 Created: A new resource was successfully created.
204 No Content: The request was successful, but there is no content to return.
400 Bad Request: The request was malformed or invalid.
401 Unauthorized: Authentication is required or has failed.
403 Forbidden: The request is authenticated but the user does not have the necessary permissions.
404 Not Found: The requested resource was not found.
500 Internal Server Error: An error occurred on the server.
2. Error Handling
Provide meaningful error messages and include relevant details to help developers diagnose issues.
Error Format: Use a consistent error format, such as JSON, with fields like
error_code
,message
, anddetails
.Example Error Response:
{ "error_code": "INVALID_REQUEST", "message": "The request parameters are invalid.", "details": "Email is required." }
3. Pagination
For endpoints that return large sets of data, implement pagination to improve performance and usability.
Common Parameters: Use parameters like
page
andlimit
to control pagination.Response Structure: Include metadata about the total count and current page.
{ "data": [ ... ], "meta": { "total": 100, "page": 1, "limit": 10 } }
API Documentation
1. Comprehensive Documentation
Provide detailed and up-to-date documentation to help developers understand and use your API effectively.
Endpoint Descriptions: Clearly describe each endpoint, including the URL, HTTP method, and a summary of its purpose.
Parameters and Responses: Document all request parameters, response fields, and data types.
Examples: Include request and response examples for common use cases.
2. Interactive Documentation
Use tools like Swagger (OpenAPI) to create interactive API documentation that allows developers to test endpoints directly from the documentation interface.
API Security
1. Authentication and Authorization
Implement robust authentication and authorization mechanisms to protect your API.
Token-Based Authentication: Use OAuth 2.0, JWT, or API keys for secure authentication.
Role-Based Access Control (RBAC): Restrict access to endpoints based on user roles and permissions.
2. Data Encryption
Encrypt sensitive data in transit and at rest to protect against data breaches.
HTTPS: Use HTTPS to encrypt data transmitted between clients and servers.
Encryption Algorithms: Use strong encryption algorithms (e.g., AES-256) for data at rest.
3. Rate Limiting
Implement rate limiting to prevent abuse and ensure fair usage of your API.
Throttling: Limit the number of requests a user can make within a given time period.
Response Headers: Include rate limit information in response headers to inform users of their rate limits.
Conclusion
Following these guidelines will help you develop professional, reliable, and secure APIs. Consistent design principles, thorough documentation, and robust security measures are essential to creating APIs that meet the needs of your users and stand the test of time. By adhering to these best practices, you'll ensure your APIs are well-regarded and widely adopted by the developer community.
Subscribe to my newsletter
Read articles from Prasanta kumar Sahoo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
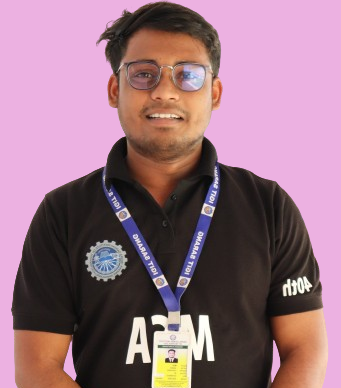
Prasanta kumar Sahoo
Prasanta kumar Sahoo
Trying to learn something new Everyday.