Graph Algorithms: Exploring Shortest Path and Minimum Spanning Tree
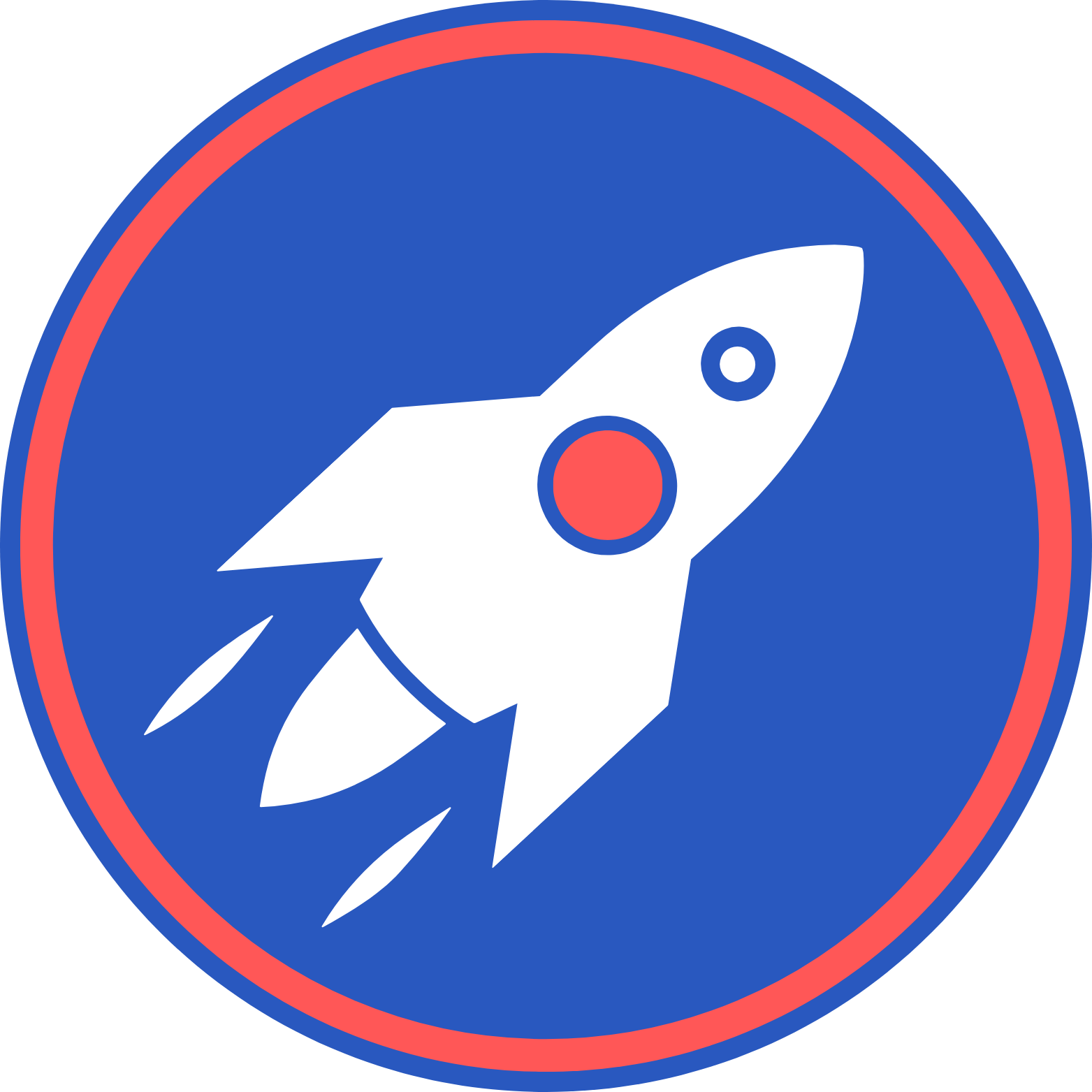
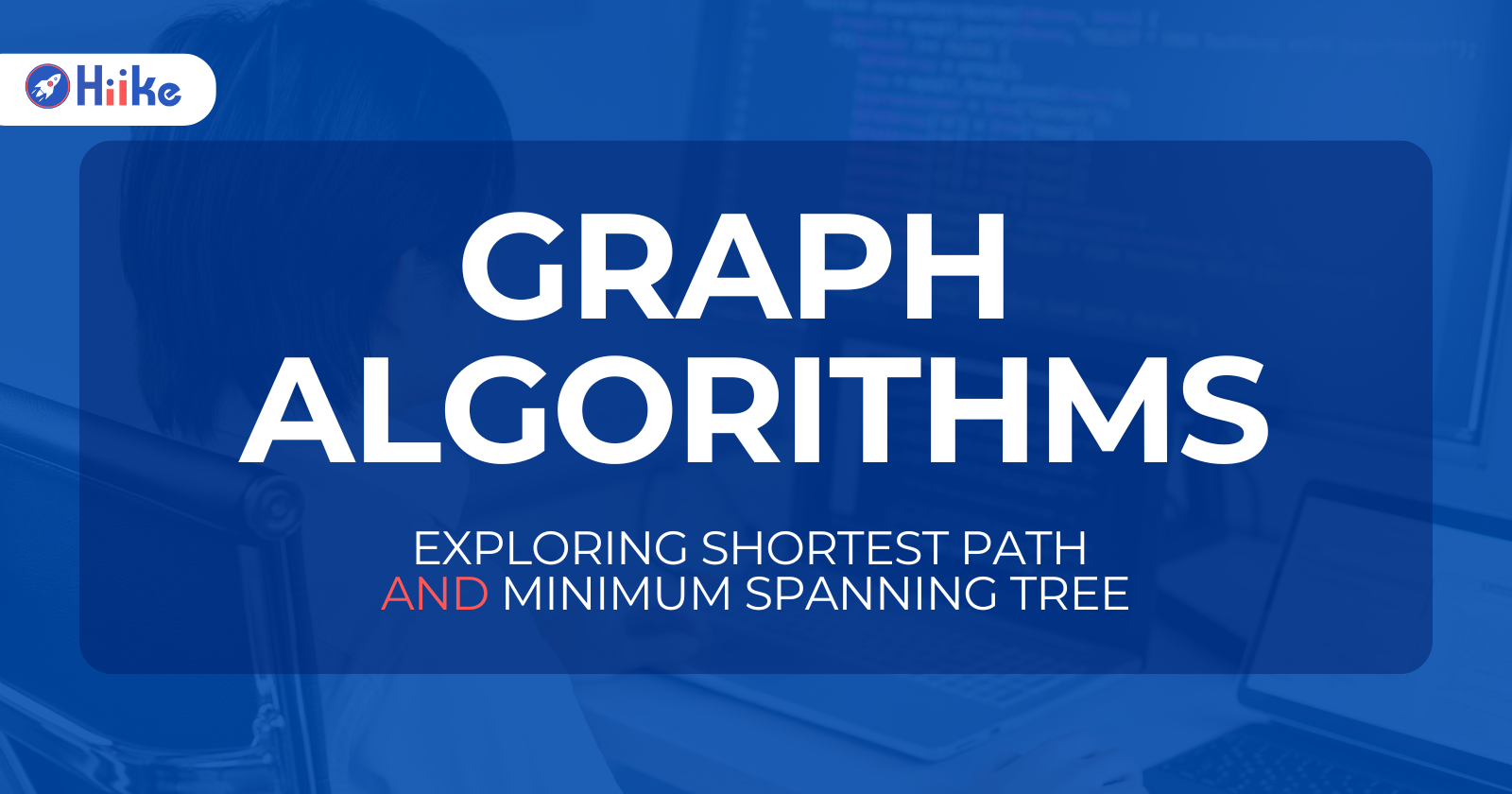
Graph algorithms are fundamental in computer science and have numerous applications in various fields, from networking to logistics. Two of the most important types of graph algorithms are those that find the shortest path and those that compute the minimum spanning tree. In this guide, we'll explore these algorithms, providing detailed explanations, practical examples, and discussing their applications and use cases.
Introduction to Graph Algorithms
Graphs are mathematical structures used to model pairwise relations between objects. They consist of vertices (nodes) and edges (connections between nodes). Graph algorithms help solve problems related to these structures, such as finding the shortest path between nodes or determining a minimum spanning tree.
Key Concepts:
Vertices (Nodes): The fundamental units of the graph.
Edges (Connections): The links between vertices.
Weighted Graphs: Graphs where edges have weights or costs.
Directed Graphs: Graphs where edges have a direction.
Explanation of Shortest Path Algorithms
Shortest path algorithms find the path between two vertices in a graph such that the sum of the weights of its constituent edges is minimized.
Common Shortest Path Algorithms:
Dijkstra's Algorithm
Dijkstra's algorithm finds the shortest path from a single source vertex to all other vertices in a graph with non-negative weights.
Steps:
Initialize distances from the source to all vertices as infinite, except for the source itself (distance 0).
Use a priority queue to select the vertex with the smallest distance.
Update the distance of neighboring vertices.
Repeat until all vertices have been visited.
Example:
import heapq
def dijkstra(graph, start):
distances = {vertex: float('infinity') for vertex in graph}
distances[start] = 0
priority_queue = [(0, start)]
while priority_queue:
current_distance, current_vertex = heapq.heappop(priority_queue)
if current_distance > distances[current_vertex]:
continue for neighbor, weight in graph[current_vertex].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(priority_queue, (distance, neighbor))
return distances
Bellman-Ford Algorithm
The Bellman-Ford algorithm computes shortest paths from a single source vertex to all other vertices in a graph, even if some edges have negative weights.
Steps:
Initialize distances from the source to all vertices as infinite, except for the source itself (distance 0).
Relax all edges |V| - 1 times, where |V| is the number of vertices.
Check for negative-weight cycles.
Example:
def bellman_ford(graph, start):
distances = {vertex: float('infinity') for vertex in graph}
distances[start] = 0
for _ in range(len(graph) - 1):
for vertex in graph:
for neighbor, weight in graph[vertex].items():
if distances[vertex] + weight < distances[neighbor]:
distances[neighbor] = distances[vertex] + weight
for vertex in graph:
for neighbor, weight in graph[vertex].items():
if distances[vertex] + weight < distances[neighbor]:
raise ValueError("Graph contains a negative-weight cycle")
return distances
Detailed Look at Minimum Spanning Tree Algorithms
Minimum spanning tree (MST) algorithms find a subset of edges that connect all vertices in the graph with the minimum possible total edge weight.
Common MST Algorithms:
Kruskal's Algorithm
Kruskal's algorithm is a greedy algorithm that finds an MST by sorting all edges and adding the smallest edge to the spanning tree, ensuring no cycles are formed.
Steps:
Sort all edges by weight.
Initialize a forest where each vertex is its own tree.
Add the smallest edge to the forest, merging two trees, until only one tree remains.
Example:
class DisjointSet:
def init(self, vertices):
self.parent = {v: v for v in vertices}
self.rank = {v: 0 for v in vertices}
def find(self, vertex):
if self.parent[vertex] != vertex:
self.parent[vertex] = self.find(self.parent[vertex])
return self.parent[vertex]
def union(self, vertex1, vertex2):
root1 = self.find(vertex1)
root2 = self.find(vertex2)
if root1 != root2:
if self.rank[root1] > self.rank[root2]:
self.parent[root2] = root1
else:
self.parent[root1] = root2
if self.rank[root1] == self.rank[root2]:
self.rank[root2] += 1
def kruskal(graph):
edges = sorted(graph['edges'], key=lambda edge: edge[2])
disjoint_set = DisjointSet(graph['vertices'])
mst = []
for edge in edges:
vertex1, vertex2, weight = edge
if disjoint_set.find(vertex1) != disjoint_set.find(vertex2):
disjoint_set.union(vertex1, vertex2)
mst.append(edge)
return mst
Prim's Algorithm
Prim's algorithm is another greedy algorithm that finds an MST by starting with a single vertex and expanding the MST by adding the smallest edge from the existing tree to a new vertex.
Steps:
Start with an arbitrary vertex.
Use a priority queue to select the smallest edge that connects a vertex in the tree to a vertex outside the tree.
Add the selected edge and vertex to the tree.
Repeat until all vertices are included.
Example:
import heapq
def prim(graph, start):
mst = []
visited = set()
priority_queue = [(0, start, None)]
while priority_queue:
weight, vertex, parent = heapq.heappop(priority_queue)
if vertex not in visited:
visited.add(vertex)
if parent is not None:
mst.append((parent, vertex, weight))
for neighbor, edge_weight in graph[vertex].items():
if neighbor not in visited:
heapq.heappush(priority_queue, (edge_weight, neighbor, vertex))
return mst
Practical Examples and Exercises
Exercise 1: Implement Dijkstra's Algorithm
Given a graph represented as an adjacency list, implement Dijkstra's algorithm to find the shortest path from a given source node to all other nodes.
Exercise 2: Implement Kruskal's Algorithm
Given a list of edges and their weights, implement Kruskal's algorithm to find the minimum spanning tree of the graph.
Exercise 3: Compare Shortest Path Algorithms
Compare the performance of Dijkstra's and Bellman-Ford algorithms on a graph with both positive and negative weights.
Applications and Use Cases
Shortest Path Algorithms
Navigation Systems: Finding the shortest route between two locations.
Network Routing: Optimizing data packet paths in a network.
Project Scheduling: Identifying the critical path in project management.
Minimum Spanning Tree Algorithms
Network Design: Laying out cables or pipelines with minimum cost.
Clustering: Grouping data points into clusters in machine learning.
Image Processing: Constructing the minimum spanning tree for image segmentation.
Conclusion
Graph algorithms for finding the shortest path and minimum spanning tree are essential tools in a developer's toolkit. Understanding these algorithms enables you to tackle a variety of complex problems in different domains efficiently. At Hiike, we provide comprehensive training in data structures and algorithms, including in-depth coverage of graph algorithms.
Our Top 30 Program is designed to equip you with the skills needed to excel in tech interviews and real-world applications. Join Hiike today and take the first step towards mastering graph algorithms and advancing your career in the tech industry.
Subscribe to my newsletter
Read articles from Hiike directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
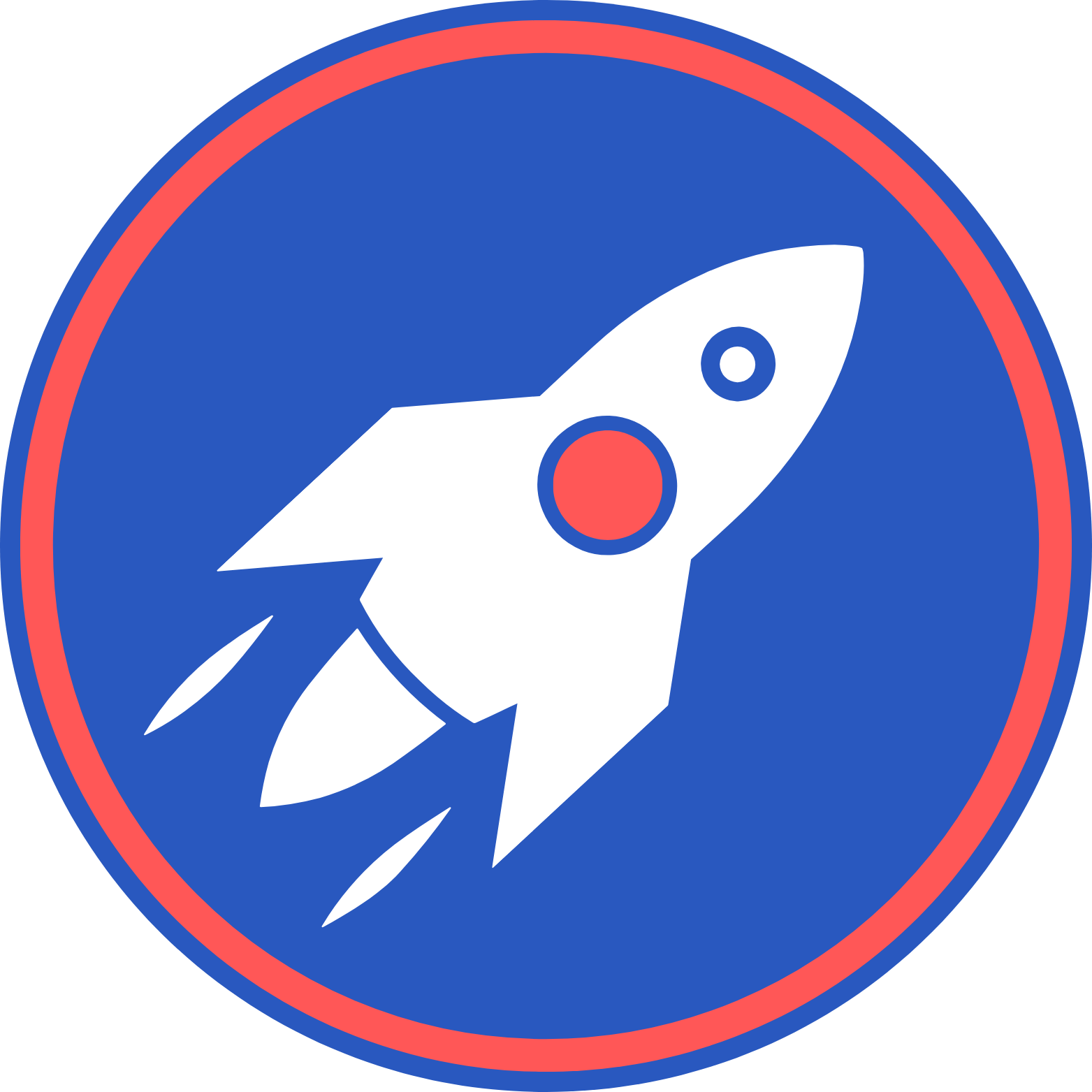
Hiike
Hiike
๐๐๐ข๐ข๐ค๐ - Crafting Your Tech Odyssey! ๐ Join Hiike for immersive learning, expert mentorship, and accelerated career growth. Our IITian-led courses and vibrant community ensure you excel in Data Structures, Algorithms, and System Design. Transform your tech career with Hiike today!