Learn to Combine WebAssembly and JavaScript: A Practical Guide
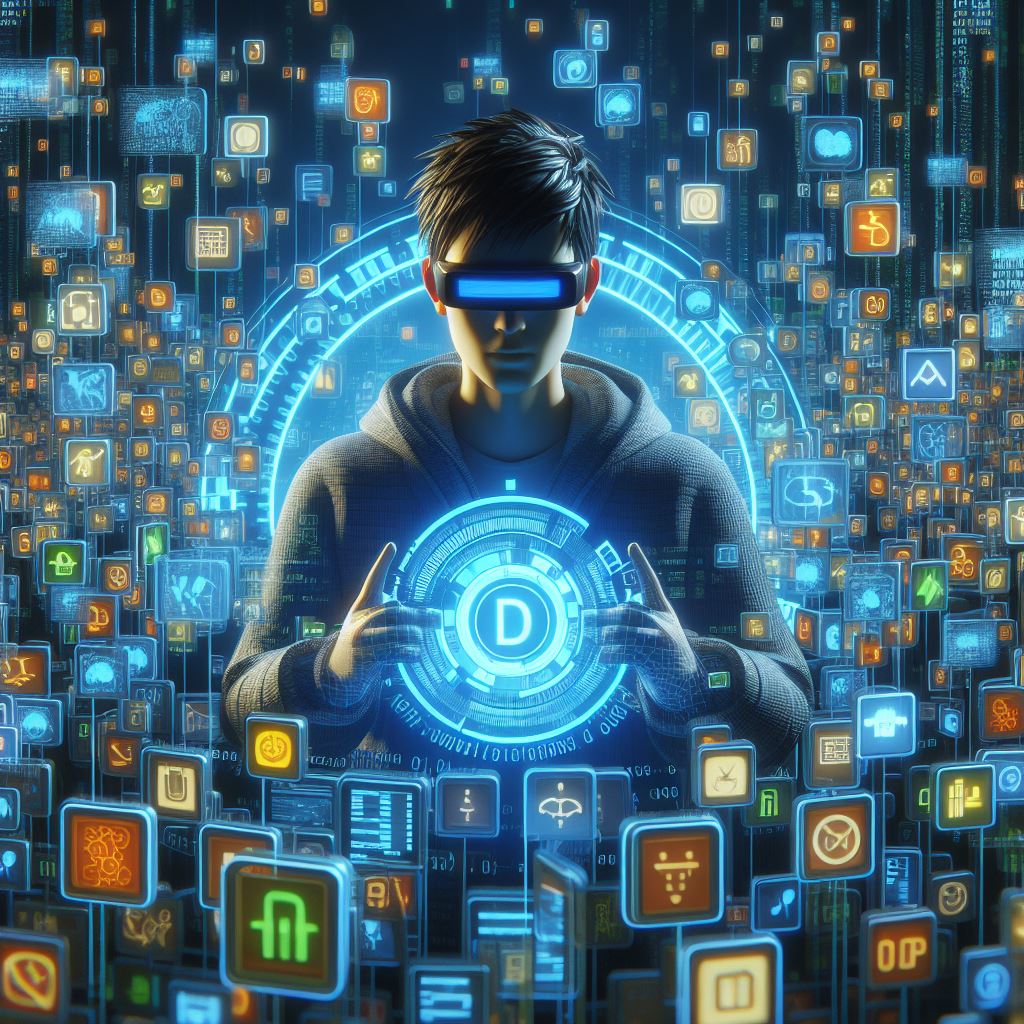
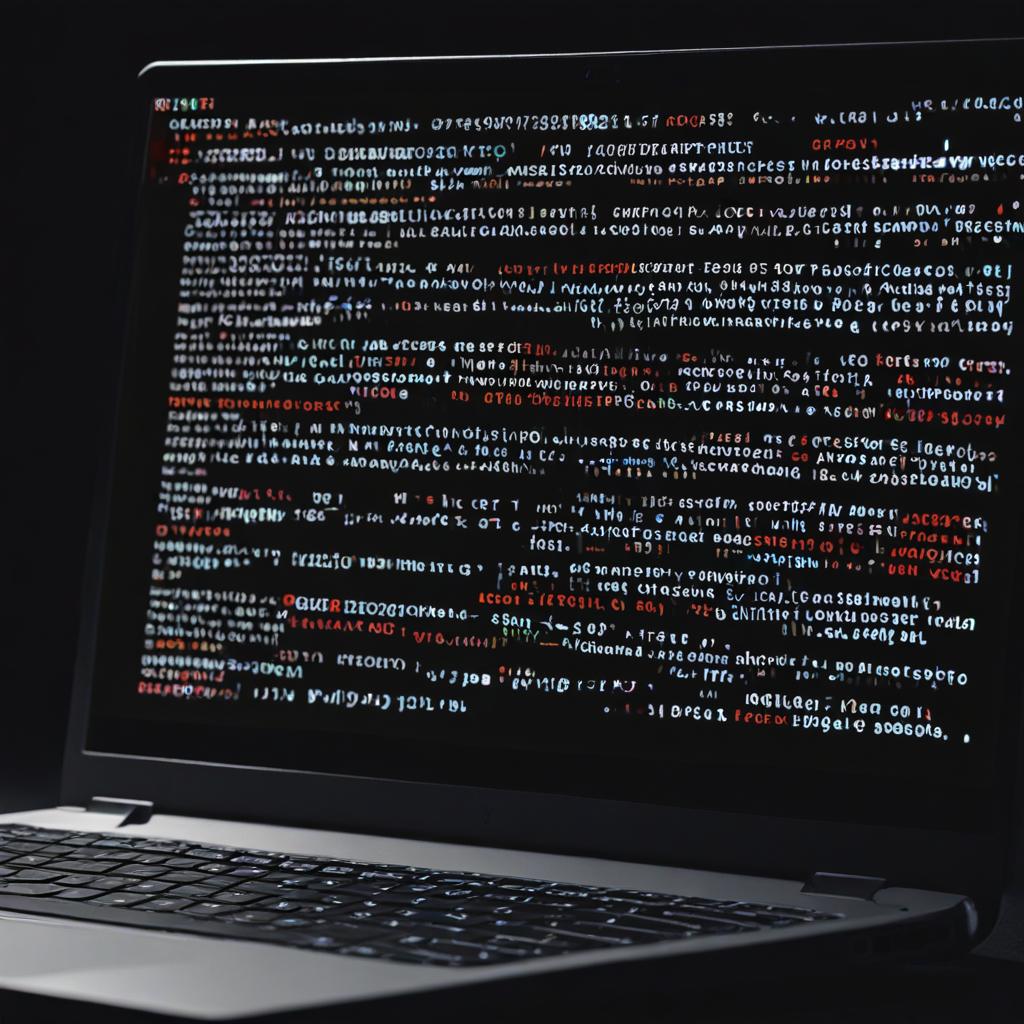
Integrating WebAssembly with JavaScript: A Fun and Informative Guide
Welcome to the future of web development, where performance meets versatility! Today, we're diving into the exciting world of WebAssembly (Wasm) and exploring how it can be integrated with JavaScript to create powerful, high-performance web applications. Whether you're a seasoned developer or just starting, this guide will provide you with a clear and fun introduction to WebAssembly and its integration with JavaScript.
What is WebAssembly?
WebAssembly, or Wasm, is a binary instruction format designed for safe and efficient execution on the web. It enables high-performance applications by allowing code written in multiple languages (like C, C++, and Rust) to run on the web at near-native speed. Think of WebAssembly as a superhero, swooping in to save the day when JavaScript alone isn't powerful enough to handle computationally intensive tasks.
Why Use WebAssembly?
Before we dive into the how, let’s understand the why. Here are some compelling reasons to integrate WebAssembly with JavaScript:
Performance: WebAssembly is designed to be fast. It can execute code at speeds comparable to native applications, making it perfect for performance-critical tasks.
Efficiency: WebAssembly's compact binary format ensures efficient transmission over the network and quick loading times.
Language Flexibility: Write code in languages like C, C++, and Rust, and run it on the web alongside JavaScript.
Compatibility: WebAssembly is designed to work with JavaScript, making it easy to integrate into existing web projects.
Getting Started with WebAssembly
Let's roll up our sleeves and get our hands dirty with some code! We'll start with a simple example: integrating a WebAssembly module written in C with a JavaScript application.
Step 1: Writing the C Code
First, we need to write a simple C function. Create a file named hello.c with the following content:
c
Copy code
#include <stdio.h> |
Step 2: Compiling to WebAssembly
Next, we'll use Emscripten, a powerful toolchain for compiling C and C++ to WebAssembly. Make sure you have Emscripten installed on your machine. If not, follow the installation instructions here.
Once Emscripten is set up, compile your C code to WebAssembly with the following command:
bash
Copy code
emcc hello.c -s WASM=1 -o hello.html |
This command generates several files, but the ones we're interested in are hello.wasm (the WebAssembly binary) and hello.js (the JavaScript glue code).
Step 3: Setting Up the HTML File
Now, let's create an HTML file to load and run our WebAssembly module. Create a file named index.html with the following content:
html
Copy code
<!DOCTYPE html> |
Step 4: Running the Example
To see our WebAssembly module in action, you'll need to serve the files using a local web server. You can use Python's built-in HTTP server for this:
bash
Copy code
python3 -m http.server |
Navigate to http://localhost:8000 in your web browser, and you should see "Hello from WebAssembly!" printed in the console.
Advanced Integration: Passing Data Between JavaScript and WebAssembly
Now that we've got the basics down, let's explore a more advanced example: passing data between JavaScript and WebAssembly. We'll write a simple function in C that adds two numbers and returns the result to JavaScript.
Step 1: Writing the C Code
Create a file named math.c with the following content:
c
Copy code
int add(int a, int b) { |
Step 2: Compiling to WebAssembly
Compile the C code to WebAssembly:
bash
Copy code
emcc math.c -s WASM=1 -s EXPORTED_FUNCTIONS='["_add"]' -o math.js |
Step 3: Setting Up the HTML and JavaScript
Create an HTML file named index.html with the following content:
html
Copy code
<!DOCTYPE html> |
Step 4: Running the Example
Serve the files using a local web server and navigate to http://localhost:8000 in your web browser. You should see "5 + 3 = 8" printed in the console.
Conclusion: Embracing the Power of WebAssembly
Congratulations! You've just scratched the surface of what WebAssembly can do. By integrating WebAssembly with JavaScript, you can create web applications that are both powerful and efficient. Whether you're optimizing performance-critical sections of your code or leveraging existing C/C++ libraries, WebAssembly opens up a world of possibilities for web development.
So, what are you waiting for? Dive into the world of WebAssembly, experiment with different languages, and start building the next generation of high-performance web applications. Happy coding!
Subscribe to my newsletter
Read articles from Reagan Mwangi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
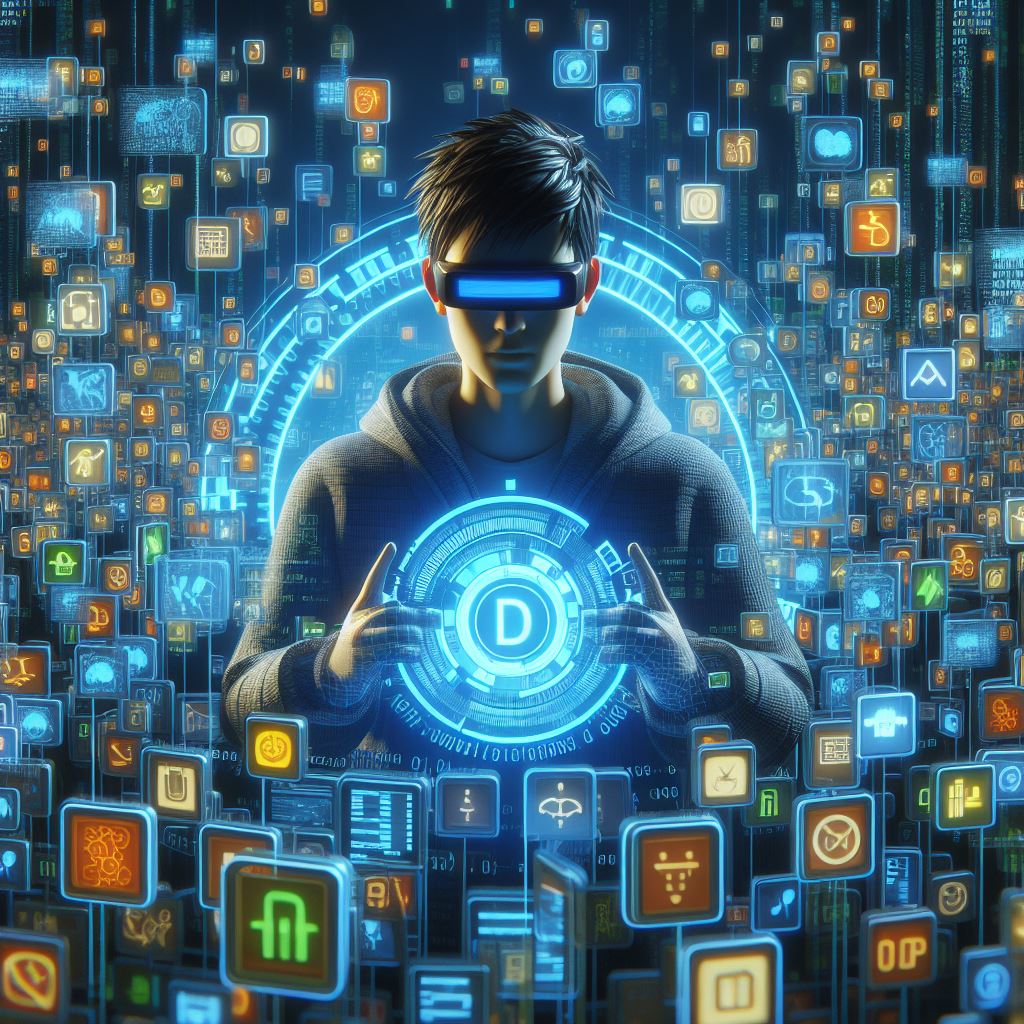