Python Unit Testing: Mocking
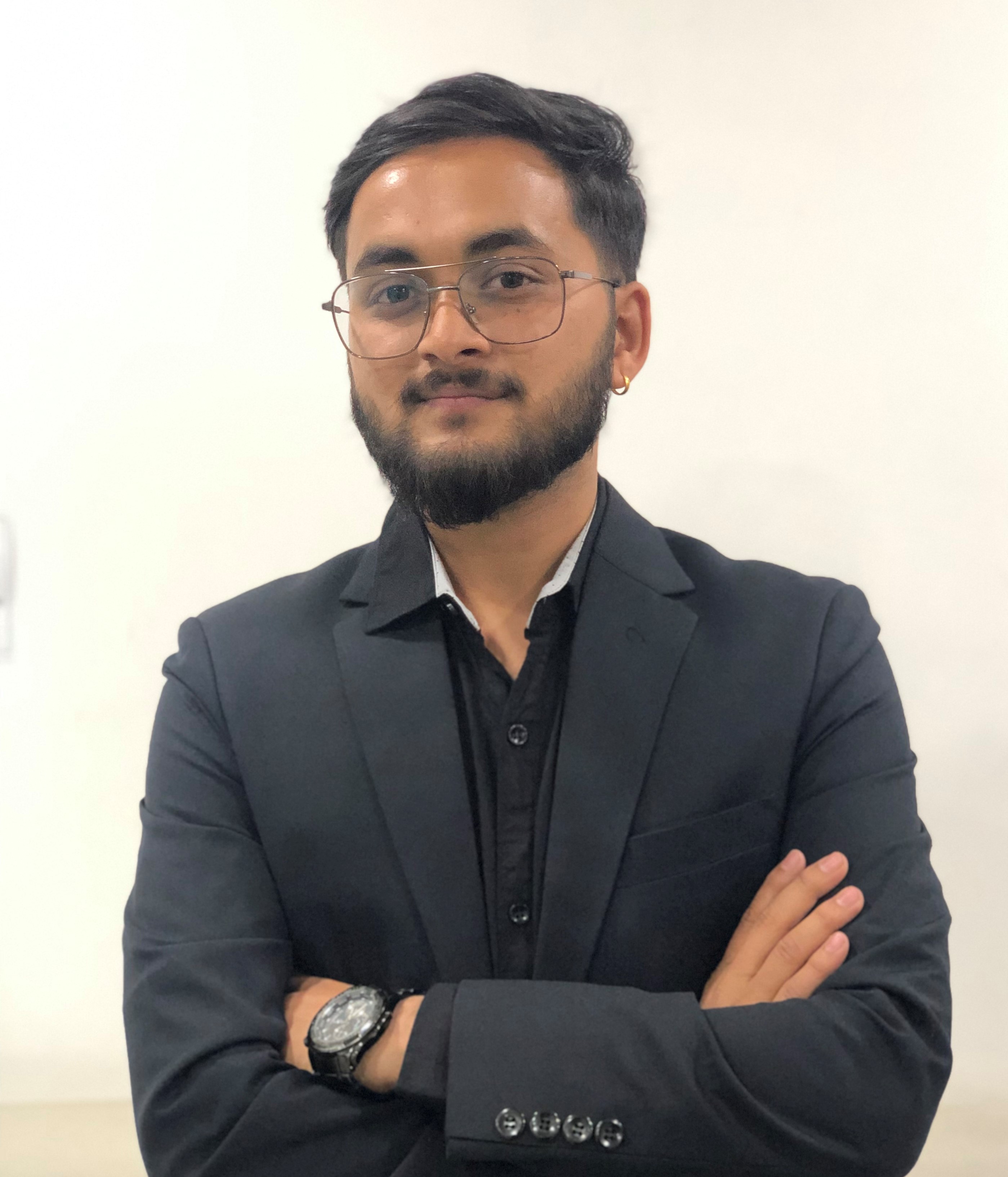
Table of contents
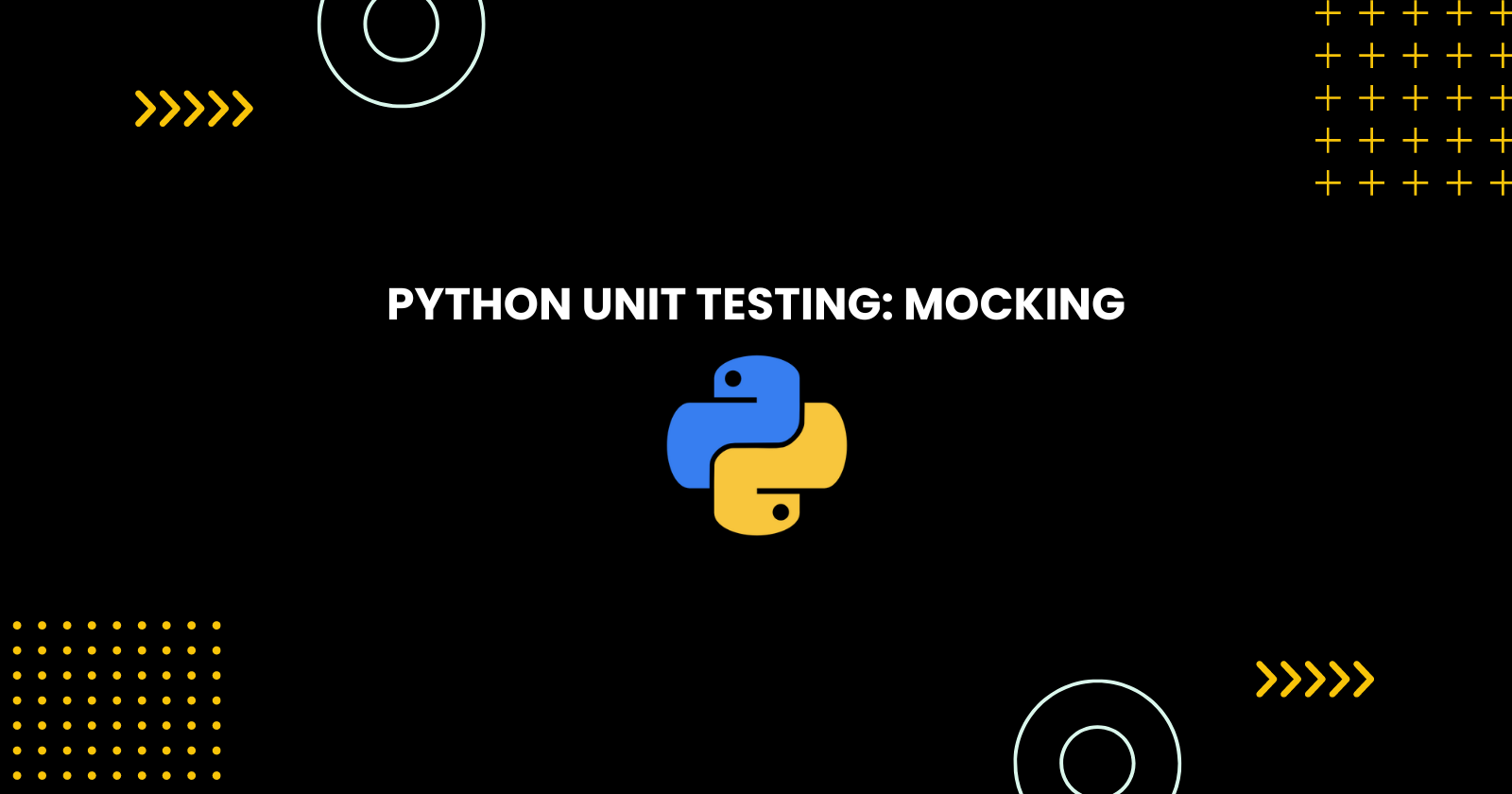
Mocking means replacing real objects with pretend ones during testing, especially in unit tests. This technique lets to create different scenarios without using real resources, which saves time and effort. The Mock() object from the unittest.mock class is crucial here. It allows to define how these fake objects should behave and helps catch potential bugs or errors in the code.
Mock() object
The Mock() object from the unittest.mock class is at the heart of mocking in Python. It serves as a versatile tool for creating mock objects that behave like real ones but don't have actual functionality. We can set attributes, define return values for methods, and even assert how these mock objects are used in tests.
Example:
from unittest.mock import Mock
# Create a mock object
mock_obj = Mock()
# Set an attribute
mock_obj.attribute = 'value'
# Define a method's return value
mock_obj.some_method.return_value = 10
# Usage in a test scenario
result = mock_obj.some_method()
assert result == 10
mock_obj is a mock object where attribute is set to 'value', and some_method() is mocked to return 10. This allows to simulate different behaviors without accessing real resources.
patch()
The patch function from unittest.mock acts as a decorator or a context manager. It temporarily replaces objects or functions during testing, allowing to control their behavior within a specific scope.
Example:
from unittest.mock import patch
# Example of patching a function
def original_function():
return 'Real function'
@patch('__main__.original_function')
def test_mocked_function(mock_function):
mock_function.return_value = 'Mocked function'
result = original_function()
assert result == 'Mocked function'
patch temporarily replaces original_function with a mock version defined within test_mocked_function. This ensures that the test test_mocked_function runs with the mocked behavior, validating expected outcomes without altering the original function's behavior outside the test.
side_effect
The side_effect attribute of a mock object allows to define what happens when the mock object is called. It can be set to a function, an exception, or a sequence of return values, making it versatile for testing different scenarios.
Example:
from unittest.mock import MagicMock
# Example using side_effect with MagicMock
mock = MagicMock()
# Define side_effect as a sequence
mock.side_effect = [1, 2, 3]
print(mock()) # Output: 1
print(mock()) # Output: 2
print(mock()) # Output: 3
mock() is called three times, and each time it returns the next value from the side_effect list [1, 2, 3]. This demonstrates how side_effect can be used to simulate different return values sequentially during testing.
Magicmock()
MagicMock() is a subclass of Mock() that automatically provides all attributes and methods. It's useful when you need a mock object that behaves like a real one without needing to manually define every aspect.
from unittest.mock import MagicMock
# Example of MagicMock usage
mock_obj = MagicMock()
# Set a method's return value
mock_obj.some_method.return_value = 'Mocked result'
result = mock_obj.some_method()
print(result) # Output: 'Mocked result'
mock_obj is a MagicMock object where some_method() is mocked to return 'Mocked result'. This simplifies mocking by automatically providing all necessary attributes and methods of the mocked object.
By leveraging these mocking techniques in Python, It helps to effectively isolate components for testing, simulate diverse scenarios, and ensure robustness in their codebases.
Subscribe to my newsletter
Read articles from Sushil Tiwari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
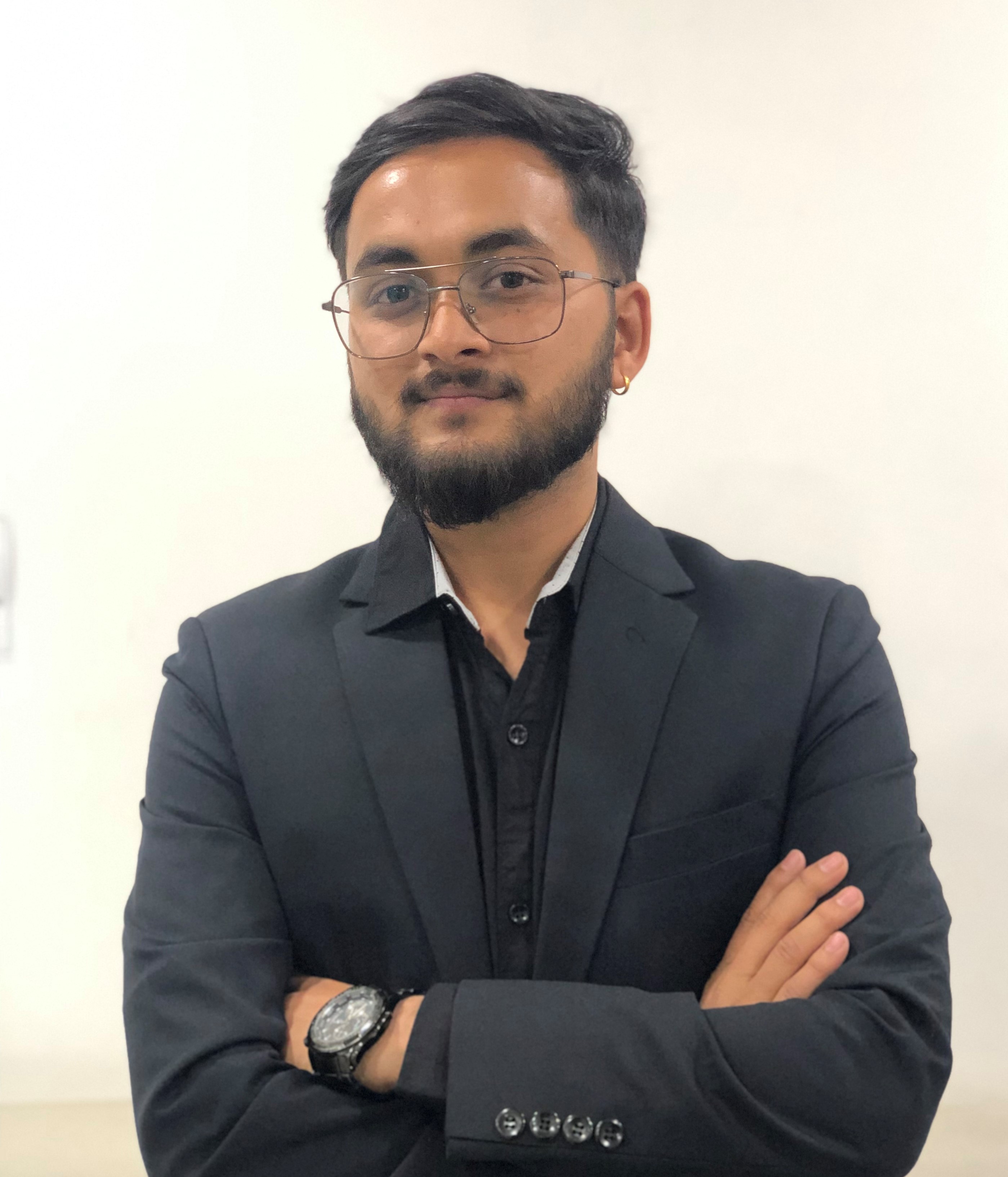