Step-by-Step Guide to Packaging and Publishing Python Code

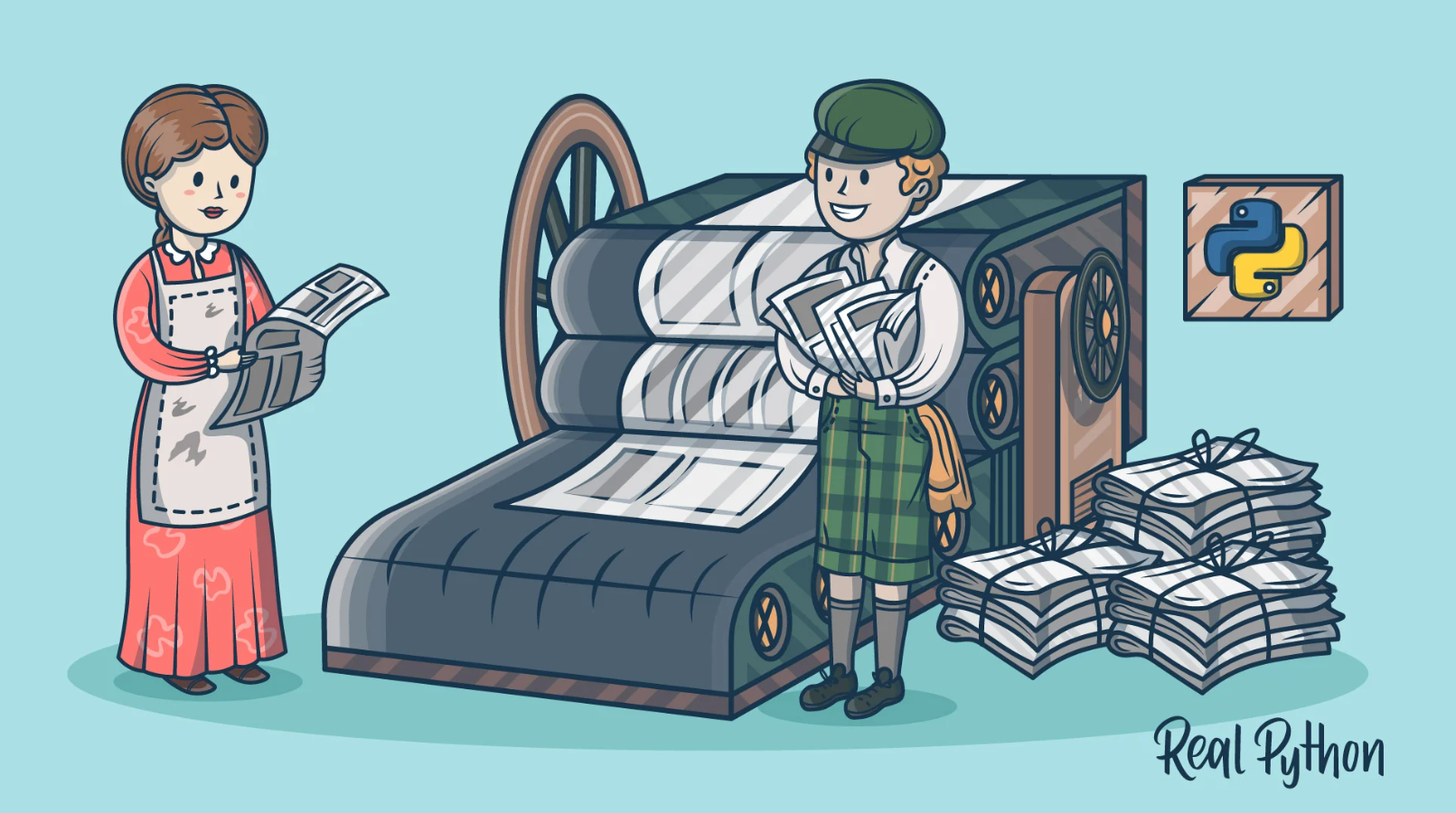
If you're a Python developer, you might be wondering how to share your code with others. Packaging and publishing your Python code allows other developers to use your libraries and tools easily. This post will walk you through the steps to package and publish a Python library. We’ll also cover terms like "wheel" and "egg info" and provide some important tips to consider when sharing your code.
Why Package Your Python Code?
Packaging your Python code can make it easier to manage and reuse, especially in larger projects. If you have common tools or functions that you use across different scripts or projects, packaging them can save you time and avoid redundancy. Furthermore, publishing these packages allows others to benefit from your work, contributing to the larger Python community.
Setting Up Your Project
Project Structure
Before anything, let's look at a simple project structure. Here’s a basic setup for an ID generator library:
IDGenerator/
├── IDGenerator
│ ├── __init__.py
│ ├── id_generator.py
│ └─�� utils.py
├── tests/
│ ├── __init__.py
│ └── test_id_generator.py
├── README.md
├── LICENSE
└── setup.py
IDGenerator: Contains your Python modules.
tests: Contains test modules.
README.md: Describes your project.
LICENSE: Provides the licensing information.
setup.py: Contains packaging information.
Writing the Code
Inside the id_generator.py
file, you might have a few functions to generate IDs, passwords, and so on. In utils.py
, you can place utility functions. Adding tests is also crucial to ensure your code works as expected.
Writing Tests
Here's an example of a test case for the ID generator:
import unittest
from IDGenerator import generate_password, generate_uuid
class TestIDGenerator(unittest.TestCase):
def test_generate_password(self):
self.assertTrue(len(generate_password(10)) == 10)
def test_generate_uuid(self):
self.assertTrue(isinstance(generate_uuid(), str))
if __name__ == "__main__":
unittest.main()
Packaging Your Code
The setup.py
File
Your setup.py
is the core of your package. Here’s an example setup file to package the ID generator:
from setuptools import setup, find_packages
with open("README.md", "r") as fh:
long_description = fh.read()
setup(
name="IDGenerator",
version="0.1.0",
author="Your Name",
author_email="your.email@example.com",
description="A library for generating various types of IDs",
long_description=long_description,
long_description_content_type="text/markdown",
url="https://github.com/yourusername/idgenerator",
packages=find_packages(),
classifiers=[
"Programming Language :: Python :: 3",
"License :: OSI Approved :: MIT License",
"Operating System :: OS Independent",
],
python_requires='>=3.6',
)
Building the Package
To build your package, navigate to your project directory and run:
python setup.py sdist bdist_wheel
This command will generate distribution archives in a dist/
directory.
Publishing Your Package
Create an Account on PyPI
First, you need an account on PyPI. Register and get your credentials.
Uploading Using Twine
Twine is a tool for uploading packages. Install it using:
pip install twine
To upload your package to PyPI, run:
twine upload dist/*
Enter your PyPI username and password when prompted.
Testing Your Package
Before uploading to the main PyPI repository, it’s wise to test on TestPyPI:
twine upload --repository-url https://test.pypi.org/legacy/ dist/*
Once you’re satisfied with your package on TestPyPI, you can upload to the main repository.
Important Considerations
Code Quality: Ensure your code is well-written, tested, and documented.
Documentation: Include a detailed README and consider writing additional documentation.
Licensing: Choose the correct license for your project. Tools like choosealicense.com can help.
Versioning: Use semantic versioning (e.g., 1.0.0) to clearly communicate updates and changes.
Classifiers: Use classifiers in
setup.py
to make your package easily discoverable based on different criteria.
Conclusion
Packaging and publishing your Python code can significantly improve your development workflow and help the community. By following this guide, you should be well on your way to sharing your useful tools and libraries with others. Happy coding!
Subscribe to my newsletter
Read articles from Hrithik Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Hrithik Singh
Hrithik Singh
I am a graduate in computer science and engineering. With over a year of working as a fullstack developer in a legal company and joining lots of hackathons, I've gained a bunch of skills and improved my problem solving skills. I know my way around C, C++, and JavaScript, Python, and I'm pretty good with frameworks like Node.js, ReactJs, and NextJs, handling both front and back-end stuff.