Understanding Linting, Linters, and ESLint: A Guide for JavaScript Developers

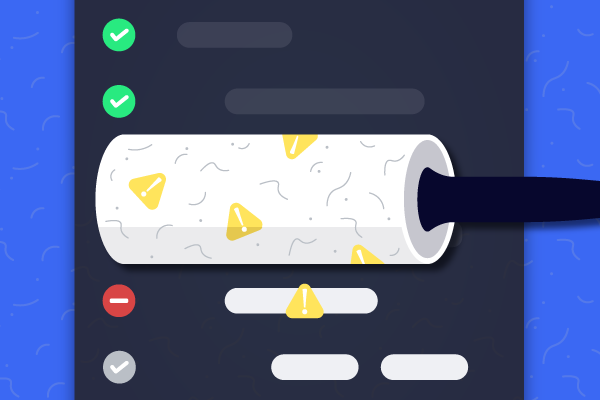
As a software engineer, you have likely encountered terms like "linting," "linters," and "ESLint" in your development workflow. These concepts are crucial for maintaining high code quality and ensuring consistency in your codebase. This blog post explores the principles of linting, how linters work, and why ESLint is an essential tool for JavaScript developers.
Linting
Linting is the process of analyzing code to find potential errors, stylistic issues, and areas for improvement. It helps developers maintain a consistent coding style, catch common mistakes early, and follow best practices. Linting is especially useful in large projects with multiple contributors, where keeping a uniform code style can be challenging.
Linters
A linter is a tool that performs linting. It scans your source code and highlights issues based on a set of predefined rules or guidelines. Linters can identify a wide range of issues, including:
Syntax errors
Coding Style violations
Potential bugs
Code complexity issues
Deprecated or unsafe code patterns
By integrating a linter into our development workflow, we can catch and address problems early, reducing the likelihood of bugs making it into production.
ESLint
ESLint is one of the most popular linters for JavaScript and its ecosystem. Created by Nicholas C. Zakas in 2013, it has become an essential tool for JavaScript developers. ESLint is highly configurable and supports ECMAScript 6 (ES6) and newer versions, as well as frameworks like React, Vue, and Angular.
Key Features of ESLint
Highly Configurable: ESLint allows you to customize rules according to your project's needs. You can enable, disable, or modify rules to match your coding standards.
Plugin Support: ESLint's extensibility is one of its strongest features. You can extend its functionality using plugins, which add or modify rules and support different environments.
Integrations: ESLint integrates seamlessly with popular code editors like VSCode, Sublime Text, and Atom, providing real-time feedback as you write code.
Autofixing: ESLint can automatically fix certain types of issues, saving you time and effort in maintaining code quality.
Community and Ecosystem: With a large and active community, ESLint has a wealth of shared configurations and plugins that you can use to enforce best practices in your projects.
Setting Up ESLint
Setting up ESLint in your project is straightforward. Here's a step-by-step guide to getting started:
Install ESLint*: You can install ESLint using npm or yarn:*
npm install eslint --save-dev # or yarn add eslint --dev
Initialize ESLint*: Run the following command to create an ESLint configuration file:*
npx eslint --init
This command will prompt you with a series of questions to tailor the configuration to your project's needs.
Configure ESLint*: The configuration file (
.eslintrc.js
or.eslintrc.json
) defines the rules ESLint will enforce. Here's an example of a basic configuration:*module.exports = { "env": { "browser": true, "es6": true }, "extends": "eslint:recommended", "rules": { "no-console": "warn", "indent": ["error", 2], "quotes": ["error", "single"] } };
Lint Your Code*: You can now lint your code by running:*
npx eslint index.js
For a whole project, you might run:
npx eslint . --ext .js,.jsx
Best Practices for Using ESLint
Integrate with Version Control*: Incorporate a linting step into your CI/CD pipeline to ensure code quality before merging changes.*
Use Prettier for Formatting*: Combine ESLint with Prettier, a code formatter, to address code style issues. There are plugins available to integrate Prettier with ESLint seamlessly.*
Adopt a Shared Configuration*: In larger teams, utilize shared ESLint configurations to maintain consistency. This can be a custom configuration specific to your team or an existing one like Airbnb's.*
Regularly Update*: Keep ESLint and its plugins up to date to take advantage of the latest features and bug fixes.*
Conclusion
Linting and linters are essential in modern software development for ensuring code quality and consistency. ESLint, with its many features and flexibility, is a great choice for JavaScript developers. By integrating ESLint into your workflow, you can catch errors early, enforce coding standards, and keep your codebase clean.
Happy linting!
Subscribe to my newsletter
Read articles from bikal adhikari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
