Understanding React's Virtual DOM: The Algorithm Behind Efficient Updates

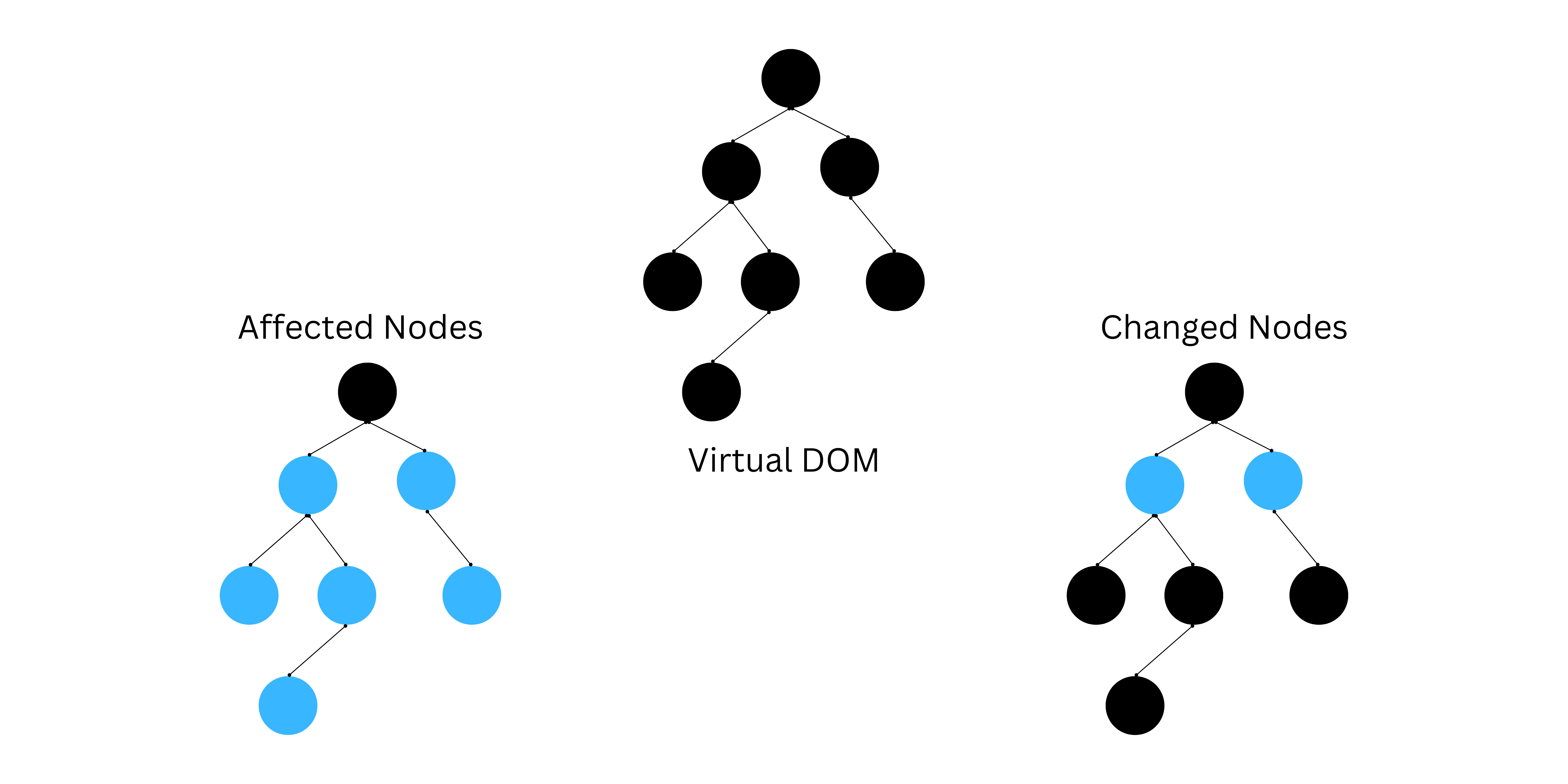
We all know about the amazing JavaScript framework React.js and that how it is declarative is more efficient than writing plain old javascript and uses a virtual DOM. But what is this virtual DOM and how does this make React so powerful. Let's find out.
What is a DOM?
Before going into Virtual DOM and its complexities let's first understand what is a DOM. A DOM or Document Object Model is a representation of the HTML document as a tree of objects, each representing a part of the document.
What is a Virtual DOM?
A virtual DOM is a feature in React.js and just like the real DOM it is also the tree like representation of the HTML document. React uses this to efficiently update the UI. Unlike the Real DOM which is slow to update, the virtual DOM is fast and updates the UI efficiently. The difference is that when a change occurs on the web page then:
(a) In case of real DOM that entire node gets destroyed along with all of its children and a new node is created from scratch.
(b) In case of virtual DOM only the nodes which have been changed gets updated in the real DOM and the children of that node are not affected.
How does the Virtual DOM works?
When a React application is built then it creates virtual DOM which is a copy of the original DOM. Whenever there is any change in the state of a component it reflects in the virtual DOM first. Then React compares the changes in virtual DOM with the previous version and only updates the changed components and does not create the whole node from scratch using an algorithm called "diffing".
This is how React manages to update the DOM with minimal changes avoiding unnecessary re-renders.
What is "Diffing"?
You must be wondering what is this "diffing" algorithm. Let me put some light on this topic. Diffing is React's algorithm to update the real DOM efficiently. This algorithm has a Time Complexity of O(n) meaning that it can easily compare large trees efficiently. This works as follows:
Tree Comparison: React compares the tree created by Virtual DOM and the Real DOM.
Element Comparison: It compares elements from the root and makes its way to the bottom traversing through each child.
Updating Nodes: React identifies changes and updates and then accordingly add/remove elements/attributes from the node.
Reconciliation Process
This process is how React handle's changes and updates the Real DOM based on the changes in the Virtual DOM. Here's an in depth analysis of this process:
Element type: React first checks if the element type has changed (example: from <div> to <span>). If it has then it destroys that entire node and reconstructs that node from scratch. This is because React thinks that different element generates different DOM tree even if it is exactly the same.
Attributes & Properties: It then checks if any attributes of an element has changed. If it has changed then it only updates only that attribute node.
Child Nodes: Then React recursively applies these changes to each child node of the DOM tree.
Minimizing Re-renders
React uses the following measures to minimize re-rendering:
Keys in lists: Keys play an important role in minimizing re-renders. This is because when React checks for changes in lists then it does this by comparing keys of previous version and current version and if there are any changes then it only updates those elements.
Pure Components and Memoization: React provides PureComponent & memo to minimize unnecessary re-renders by performing a shallow comparison of props and states.
Batching Updates: React batches multiple updates into a single render pass, minimizing the number of DOM manipulations.
Performance benefits of Virtual DOM
The Virtual DOM provides several performance benefits:
Efficient Updates: By updating only the changed parts of the DOM, React reduces the time it takes to render the changes on the screen.
Improved overall user experience: Since React performs re-renders very quickly, the website load time is reduced significantly thus making the experience much smoother.
Optimized usage of resources: Efficient DOM updates lead to less computational overhead eventually leading to minimal usage of resources.
Limitations of Virtual DOM
Although the Virtual DOM is very efficient, it also has some limitations:
Complexity: The diffing algorithm and reconciliation adds complexity to the React library as they need to keep a track of Real DOM and Virtual DOM to apply changes.
Excessive memory overhead: Maintaining a Virtual DOM requires additional memory as the Virtual DOM creates a copy of the Real DOM along with all of its elements and attributes.
Not always Optimal: In some cases, using React is not optimal, especially in websites which include only static content.
Conclusion
React is a powerful frontend web development framework. The concept of Virtual DOM is a powerful concept which minimizes unnecessary re-renders and improves React application's performance. Through the use of efficient algorithms React ensures that the application is performing at its best, providing a better user experience.
Subscribe to my newsletter
Read articles from Sparsh Shandilya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
