How to Use Methods and Interfaces in Go
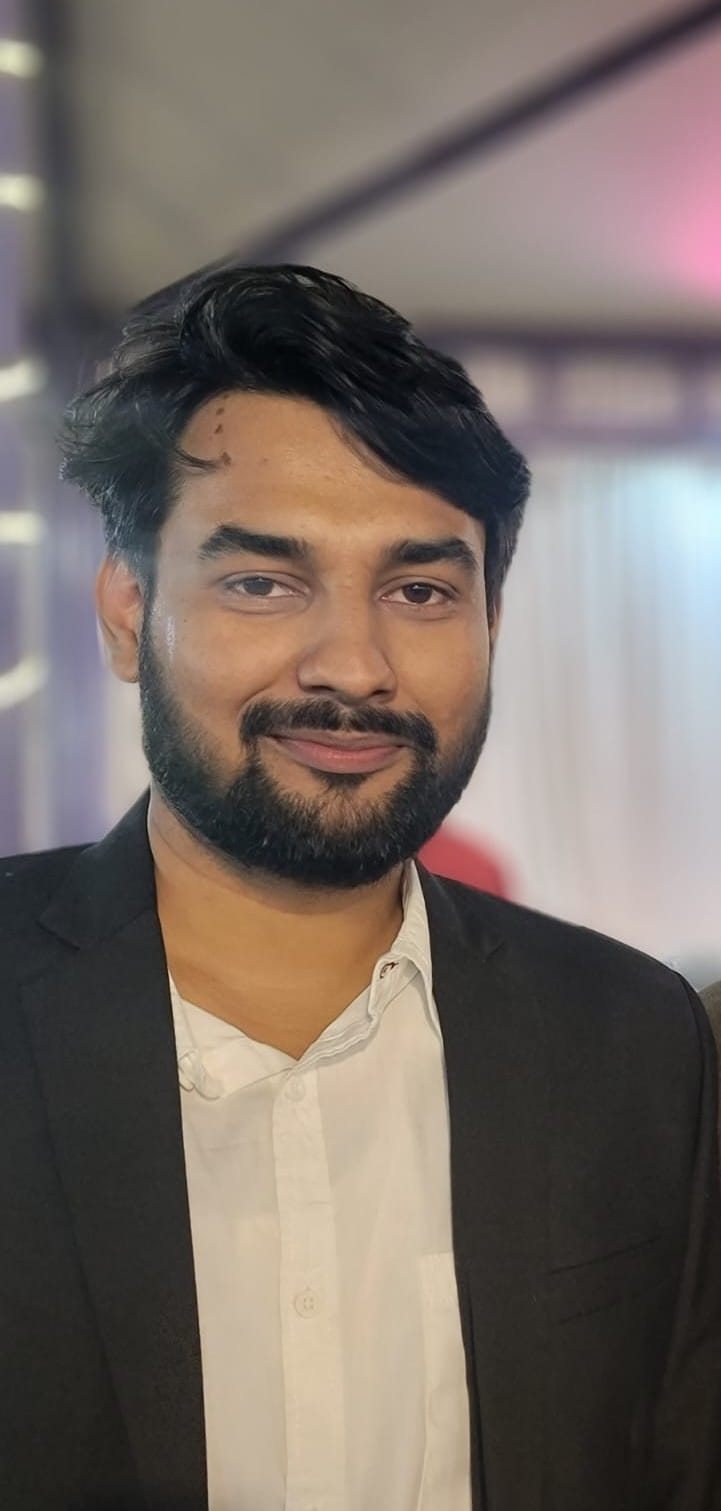
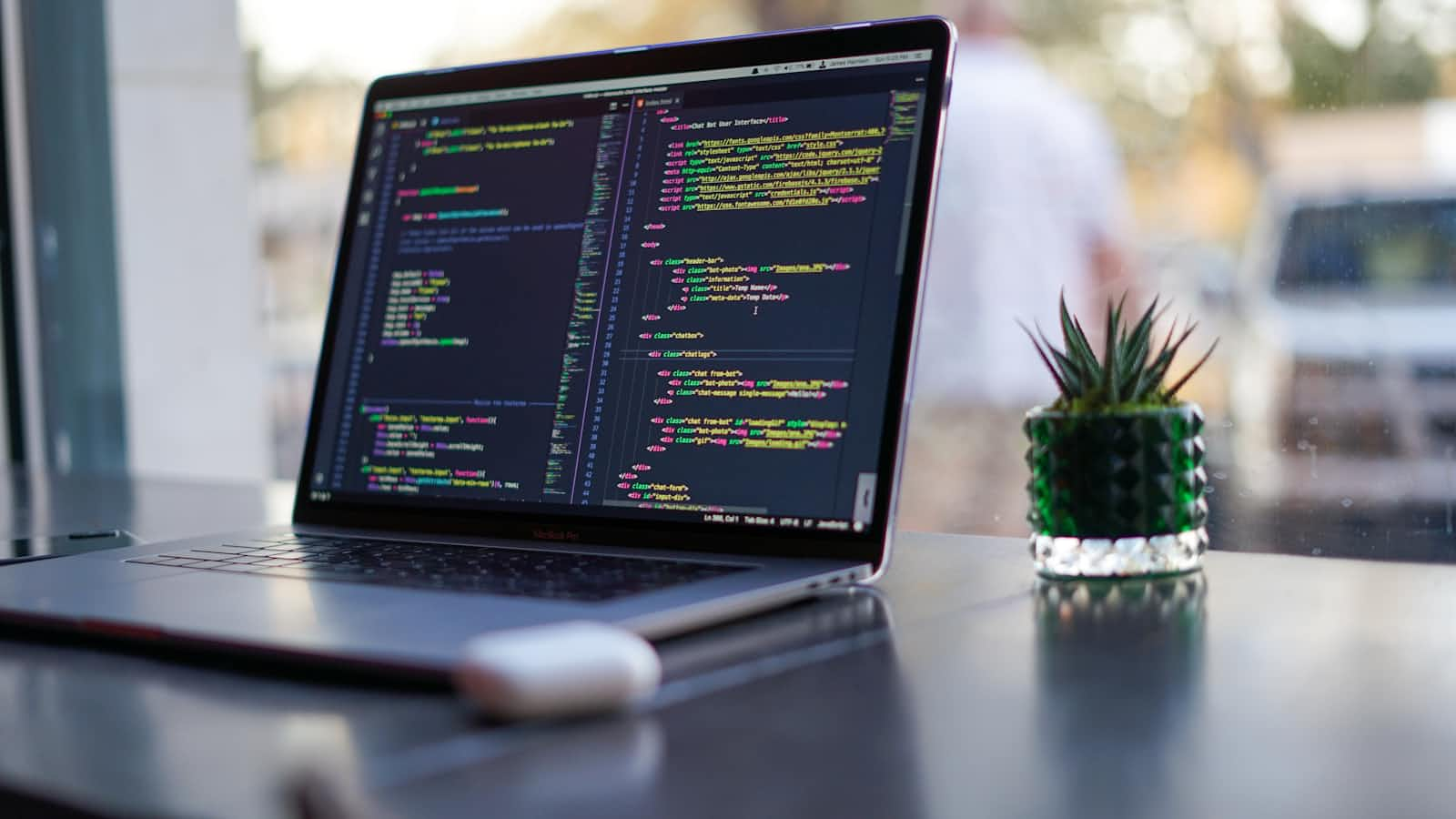
Go (Golang) is a powerful language that provides robust support for object-oriented programming concepts through methods and interfaces. In this article, we'll dive into methods, interfaces, type assertions, and embedding interfaces, helping you to harness the full potential of Go for creating modular and maintainable code.
Method Receivers
Method Receivers:
In Go, methods are functions with a special receiver argument. The receiver appears between the func
keyword and the method name and can be used to call methods on variables of the receiver type.
Example:
package main
import "fmt"
// Defining a struct
type Rectangle struct {
Width, Height float64
}
// Method with a value receiver
func (r Rectangle) Area() float64 {
return r.Width * r.Height
}
// Method with a pointer receiver
func (r *Rectangle) Scale(factor float64) {
r.Width *= factor
r.Height *= factor
}
func main() {
rect := Rectangle{Width: 10, Height: 5}
// Calling method with value receiver
fmt.Println("Area:", rect.Area()) // Output: Area: 50
// Calling method with pointer receiver
rect.Scale(2)
fmt.Println("Scaled Rectangle:", rect) // Output: Scaled Rectangle: {20 10}
}
In this example, Rectangle
has two methods: Area
with a value receiver and Scale
with a pointer receiver. The Area
method calculates the area of the rectangle, while the Scale
method modifies the dimensions of the rectangle.
Interfaces and Type Assertions
Interfaces:
Interfaces in Go are named collections of method signatures. An interface type specifies a set of methods that a type must have to implement the interface. Go interfaces are implicit, meaning that any type that implements the required methods satisfies the interface.
Example:
package main
import "fmt"
// Defining an interface
type Shape interface {
Area() float64
}
// Implementing the interface with Rectangle
type Rectangle struct {
Width, Height float64
}
func (r Rectangle) Area() float64 {
return r.Width * r.Height
}
// Implementing the interface with Circle
type Circle struct {
Radius float64
}
func (c Circle) Area() float64 {
return 3.14 * c.Radius * c.Radius
}
func main() {
var s Shape
s = Rectangle{Width: 10, Height: 5}
fmt.Println("Rectangle Area:", s.Area()) // Output: Rectangle Area: 50
s = Circle{Radius: 7}
fmt.Println("Circle Area:", s.Area()) // Output: Circle Area: 153.86
}
In this example, both Rectangle
and Circle
types implement the Shape
interface by providing an Area
method.
Type Assertions:
Type assertions provide access to an interface value's underlying concrete value. A type assertion can be used to test whether an interface value holds a specific type.
Example:
package main
import "fmt"
// Defining an interface
type Describer interface {
Describe() string
}
type Person struct {
Name string
Age int
}
func (p Person) Describe() string {
return fmt.Sprintf("%s is %d years old.", p.Name, p.Age)
}
func main() {
var d Describer
d = Person{Name: "Alice", Age: 30}
// Type assertion
if p, ok := d.(Person); ok {
fmt.Println("Describer is a Person:", p) // Output: Describer is a Person: {Alice 30}
} else {
fmt.Println("Describer is not a Person")
}
}
In this example, the type assertion checks whether the Describer
interface holds a Person
type and accesses its underlying value if the assertion is successful.
Embedding Interfaces
Embedding Interfaces:
Go allows the composition of interfaces by embedding one interface within another. This feature enables the creation of complex interfaces from simpler ones, promoting code reuse and modularity.
Example:
package main
import "fmt"
// Defining basic interfaces
type Printer interface {
Print()
}
type Scanner interface {
Scan()
}
// Embedding interfaces
type MultiFunctionDevice interface {
Printer
Scanner
}
// Implementing the embedded interface
type OfficePrinter struct{}
func (OfficePrinter) Print() {
fmt.Println("Printing document...")
}
func (OfficePrinter) Scan() {
fmt.Println("Scanning document...")
}
func main() {
var mfd MultiFunctionDevice
mfd = OfficePrinter{}
mfd.Print() // Output: Printing document...
mfd.Scan() // Output: Scanning document...
}
In this example, the MultiFunctionDevice
interface embeds the Printer
and Scanner
interfaces, and the OfficePrinter
type implements all methods required by these interfaces.
Conclusion
Methods and interfaces in Go provide powerful mechanisms for defining and using types with specific behaviors. Method receivers allow you to associate functions with types, while interfaces enable polymorphism and the creation of flexible, reusable code. Type assertions and embedded interfaces further enhance Go's capabilities, making it an excellent language for building scalable and maintainable software. Keep practicing these concepts to fully leverage the power of Go's object-oriented features in your projects.
Subscribe to my newsletter
Read articles from Lokesh Jha directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
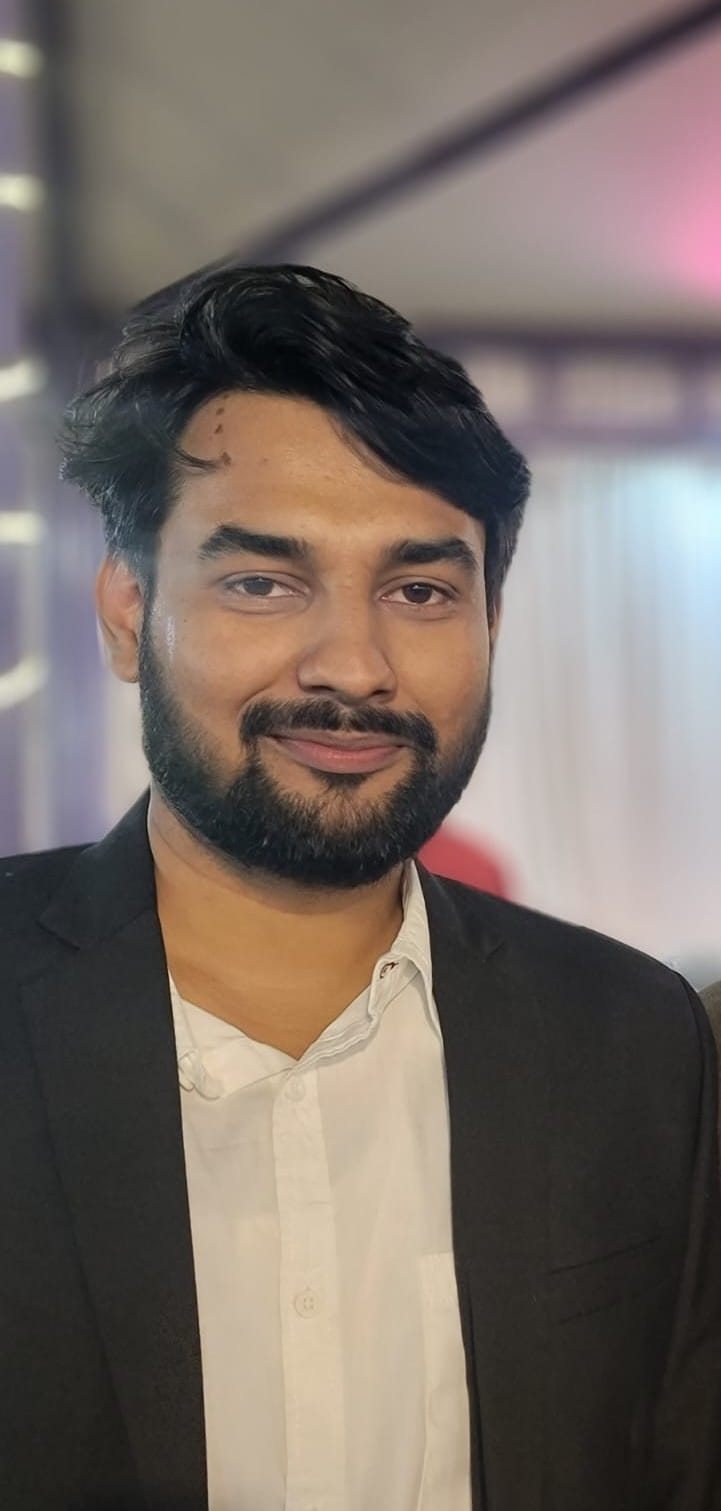
Lokesh Jha
Lokesh Jha
I am a senior software developer and technical writer who loves to learn new things. I recently started writing articles about what I've learned so that others in the community can gain the same knowledge. I primarily work with Node.js, TypeScript, and JavaScript, but I also have past experience with Java and C++. Currently, I'm learning Go and may explore Web3 in the future.