Django Portfolio
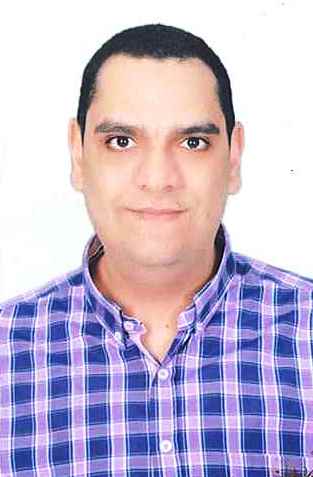
Advanced Django Blog
A blog project built using:
Project Goals
- Django Backend
Authenticated users can:
Create, Read, Update and Delete (CRUD) blog posts and tags on the website.
Add comments on blog posts, but the comments will not be visible until the website admin approves it.
Like blog posts or add them to their favorites post list (currently handled by HTMX without page refresh).
Access their profile where they can update their information, see all their added blog posts or see their favorite posts.
Access a GraphQL endpoint and run several Queries and CRUD mutations.
Access a REST API endpoint and perform CRUD operations
All Users can read or search for blog posts.
- Vue.js 3 Frontend
Users can register for a new account or login to existing account this method is using Django Rest Framework Session Authentication.
Perform CRUD operations using both REST API and GraphQL endpoints for blog posts, tags and comments.
Add or remove posts from their favorite posts list using both REST API and GraphQL.
Like or Unlike blog posts.
Search for blog posts.
Get started with this project
Without using Docker:
Make sure that both PostgreSQL database and Mailpit Email client are installed and running in your system.
Create a PostgreSQL database, This project uses PostgreSQL version 14.
Export database default url using the following terminal command, In this example PostgreSQL is installed locally, database name is django-blog-backend, and database admin credentials are => username: admin / password: 1234:
export DATABASE_URL=postgres://admin:1234@127.0.0.1:5432/django-blog-backend
Open the terminal or CMD to create a virtual environment like Python virtual environment (venv) or pipenv and activate it.
python -m venv venv
Activate the virtual environment, if you are using Linux:
source venv/bin/activate
If you are using Windows
venv/Scripts/activate
Or Git bash on windows
source venv/Scripts/activate
Change directory to django_blog_backend
cd django_blog_backend
Install the requirements from the local.txt file
python -m pip install -r requirements/local.txt
Migrate the data into the database
python manage.py makemigrations
python manage.py migrate
Create a super user
python manage.py createsuperuser
Run the project
python manage.py runserver
Before logging in to the Django website make sure that Mailpit email client is running in your system to recieve an email activation link.
Using Docker
Make sure that both [Docker](Docker) and [docker-compose](docker-compose) are installed in your system
Clone the repository: git clone https://github.com/MoustafaShaaban/Advanced_Django_Blog.git
Change directory to blog_backend directory
cd blog_backend
Build the docker image to develop the project locally using docker-compose
docker-compose -f local.yml build
Create the database by running the following commands
docker-compose -f local.yml run --rm django python manage.py migrate
Create a super user
docker-compose -f local.yml run --rm django python manage.py createsuperuser
Run the tests using pytest
docker compose -f local.yml run --rm django test blog_backend/blog
Now run the project
docker-compose -f local.yml up
Open the web browser and navigate to `http://localhost:8000/` to see the result.
Open a new terminal window and change directory to vue-frontend project
cd vue-frontend
Install the dependencies
npm install
Run the vite development server
npm run dev
Open the web browser and navigate to `http://localhost:5173/` to see the result.
GraphQL Queries and Mutations Examples
query ReturnAllPosts {
allPosts {
id
title
content
updatedAt
comments {
id
comment
user {
username
}
}
tag {
id
name
}
}
}
query returnPostBySlug($slug: String!) {
postBySlug(slug: $slug) {
id
title
content
author {
username
avatar
}
updatedAt
comments {
id
comment
user {
username
}
}
tag {
id
name
}
}
}
and in the variables:
{
"slug: "post-1"
}
query ReturnMyPost {
myPostsWithFilters {
edges {
node {
id
title
content
updatedAt
comments {
id
comment
user {
username
}
}
tag {
id
name
}
}
}
}
}
query PostByTitle {
allPostsWithFilters(title: "Post Number 1") {
edges {
node {
id
title
updatedAt
content
comments {
id
comment
}
}
}
}
}
query AllComments {
allComments {
id
user {
name
}
comment
post {
id
title
content
updatedAt
}
}
}
query AllTags {
allTags {
id
name
slug
}
}
mutation createTag {
createTag(input: {
name: "Python"
}) {
tag {
id
name
}
}
}
mutation UpdateTag {
updateTag(id: 1, name: "Python") {
tag {
id
name
}
}
}
mutation DeleteTag {
deleteTag(id: 6) {
success
}
}
mutation CreatePost {
createPost(input: {
title: "Post number 1",
content: "Post number 1 content",
tags: [
{ slug: "python" }
]
}) {
post {
id
content
}
}
}
mutation CreateComment {
createComment(inputs: {
postSlug: "post-1",
comment: "Great post",
}) {
post {
title,
comments {
comment
user {
username
}
}
}
}
}
query PostsByAuthor {
postsByAuthor(author: "admin") {
id
title
updatedAt
tag {
name
}
content
comments {
id
user {
username
}
comment
}
}
}
query PostsByTag {
postsByTag(tag: "Python") {
id
title
updatedAt
tag {
name
}
content
comments {
id
user {
username
}
comment
}
}
}
query PostsByTitle {
postByTitle(title: "Post number 1") {
id
title
updatedAt
tag {
name
}
content
comments {
id
user {
username
}
comment
}
}
}
query PostsByTitleWithDjangoFilters {
allPostsWithFilters(title_Icontains: "Post number") {
edges {
node {
id
title
updatedAt
tag {
name
}
content
comments {
id
user {
username
}
comment
}
}
}
}
}
query PostsByTitleWithDjangoFilters {
allPostsWithFilters(title_Istartswith: "Post number") {
edges {
node {
id
title
updatedAt
tag {
name
}
content
comments {
id
user {
username
}
comment
}
}
}
}
}
Django and Folium
Project Goals
Use Django admin site to import data from different sources (CSV, JSON, ...) into the database.
Importing and Exporting the data from the website directly (without using the Django Admin Site).
Use the power of Folium to visualize data generated from Django Database on a Leaflet JS map.
Visualize data using Folium's Simple Markers and Marker Cluster.
Data Sources
The data used in this project is downloaded from the Seattle City Open Data website, The following Datasets are used:
Project Preview
Libraries and Packages used
To get started with this project
Clone the repository: git clone https://github.com/MoustafaShaaban/Django_and_Folium.git
Change directory to django_and_folium
cd django_and_folium
Open the terminal or CMD to create a virtual environment like Python virtual environment (venv) or pipenv and activate it.
python -m venv venv
Create the venvsource venv/bin/activate
On Linuxvenv/Scripts/activate
On Windowssource venv/Scripts/activate
Git Bash on Windows
Install requirements.txt:
python -m pip install -r requirements/local.txt
Create the database by running the following commands:
python
manage.py
makemigrations
python
manage.py
migrate
Create a super user:
python
manage.py
createsuperuser
Login to the admin site with your super user and add the data in 'seattle/data' folder using the import functionality in each model.
Run the project:
python
manage.py
runserver
Version 2:
In this version I improved the code by combining all the data in one Django Function View to show it in one map.
Added Layer Control functionality to switch between different layers.
Changed the default style of the Markers and used font awesome icons:
* h-square
Icon for Hospitals Layer. * graduation-cap
Icon for Public Schools Layer. * book
Icon for Public Libraries Layer.
Version 2 Preview
Version 3:
In this version I added the support of Importing and Exporting the data from the website directly (without using the Django Admin Site).
Version 3 Preview
References:
Django Geoapp + Vue.js 3
Project Goals
Use Django admin site to import data from different sources (CSV, JSON, ...) into the database.
Use the power of Folium to visualize data generated from Django Database on a Leaflet JS map.
Visualize data using Folium's Simple Markers.
Users can register for an account, login, and update their information (handled by Cookiecutter Django)
Authenticated users can add, import, or export data using django forms.
Use Vue.js 3 (using Vite ) and axios to fetch the data from the backend and display it in a Bootstrap Table.
Project Files
Project Preview
Libraries and Packages used
Subscribe to my newsletter
Read articles from Moustafa Shaaban directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
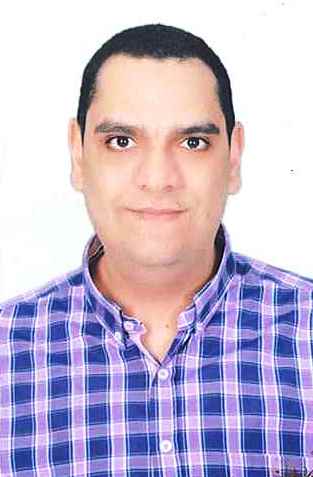
Moustafa Shaaban
Moustafa Shaaban
Software Developer with GIS background with experience in building Web applications I am seeking to apply and expand my knowledge and skills towards working in a collaborative environment to develop quality software solutions that address and solve business problems.