How to Use Wrapper Classes in Java: A Simple Tutorial

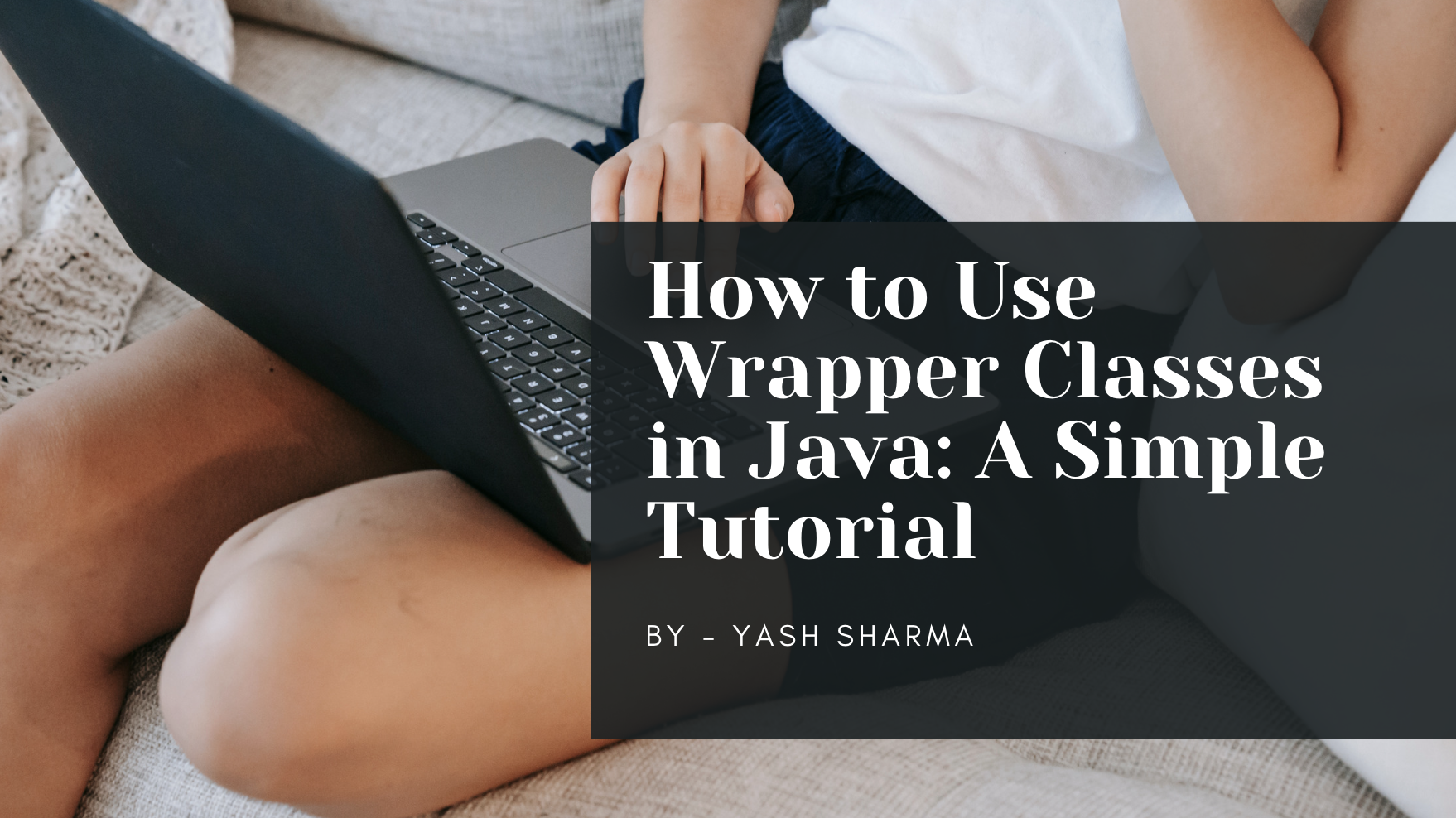
Wrapper Classes in Java
Overview
In Java, there are two main types of data: primitive types and non-primitive types.
Primitive Types: Directly hold values. Examples include
int
,char
,byte
,short
,long
,float
,double
, andboolean
.Non-Primitive Types: Objects created from classes. They are references to memory locations holding the actual data. Examples include
String
, arrays, and user-defined objects.
Wrapper Classes
Java provides a built-in class for each primitive type, known as wrapper classes, which enable primitive values to be treated as objects. These wrapper classes follow a naming convention where the first letter is capitalized.
byte
->Byte
short
->Short
int
->Integer
long
->Long
float
->Float
double
->Double
char
->Character
boolean
->Boolean
Examples
Primitive Type:
int x1 = 10; // x1 is a primitive type
Wrapper Class:
Integer x2 = new Integer(10); // x2 is a reference to an Integer object
Need for Wrapper Classes
Object-Oriented Programming: Java is fundamentally object-oriented. Many features and frameworks require objects rather than primitive types.
Generics: Generics in Java allow you to define classes, interfaces, and methods with a placeholder for types. Generics work only with objects, not primitive types.
ArrayList<Integer> list = new ArrayList<>(); // Cannot use ArrayList<int>
Collections Framework: Java collections such as
ArrayList
,HashSet
, andHashMap
require objects. Therefore, primitive types need to be wrapped in their respective wrapper classes to be used in collections.
Efficiency and Legacy
Efficiency: Primitive types are more efficient in terms of performance and memory usage as they directly store values. Wrapper classes, being objects, introduce additional overhead.
Legacy: Java inherited primitive types from C and C++ to maintain efficiency. The syntax and efficiency reasons from these languages influenced Java's design.
Practical Situations for Wrapper Classes
Collections: When using collections, wrapper classes are necessary.
ArrayList<Integer> integerList = new ArrayList<>();
Methods expecting Objects: Some Java methods are designed to work with objects, necessitating the use of wrapper classes.
Interview Questions
What are wrapper classes in Java?
- Wrapper classes in Java are object representations of primitive data types, allowing primitives to be treated as objects.
Why do we need wrapper classes in Java?
- Wrapper classes are needed for compatibility with Java's object-oriented features, especially generics and collections, which do not work with primitive types.
Can you give examples of primitive types and their corresponding wrapper classes?
int
->Integer
char
->Character
boolean
->Boolean
What are the advantages of using primitive types over wrapper classes?
- Primitive types offer better performance and memory efficiency compared to wrapper classes.
Explain the role of wrapper classes in Java collections.
- Collections in Java, such as
ArrayList
,HashSet
, andHashMap
, require objects. Wrapper classes enable the use of primitive data types within these collections.
- Collections in Java, such as
Why does Java support primitive types if object-oriented programming prefers objects?
- Java supports primitive types for performance and efficiency reasons. Primitive types provide a way to perform basic operations quickly without the overhead associated with objects.
By understanding these concepts, you can effectively use both primitive types and wrapper classes in Java, balancing efficiency and object-oriented programming needs.
Subscribe to my newsletter
Read articles from Yash Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Yash Sharma
Yash Sharma
Final-year CSE student at Chandigarh University passionate about web development and data structures and algorithm. I write articles on Java and DSA in Java, sharing insights and coding tips. Exploring my journey into teaching while refining my skills in software development.