Secure Dynamic HTML in Oracle APEX: Best Practices and Pitfalls
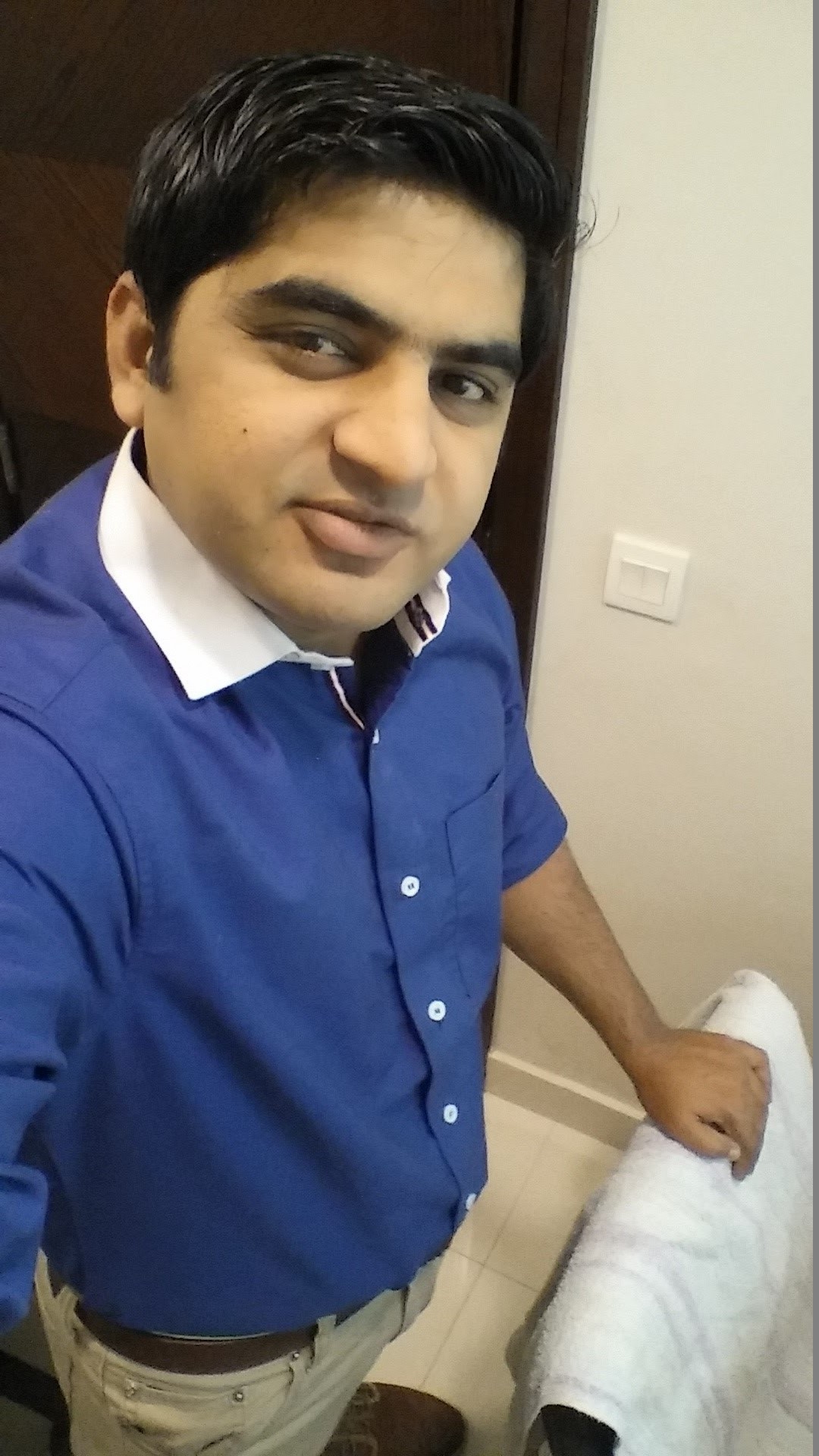
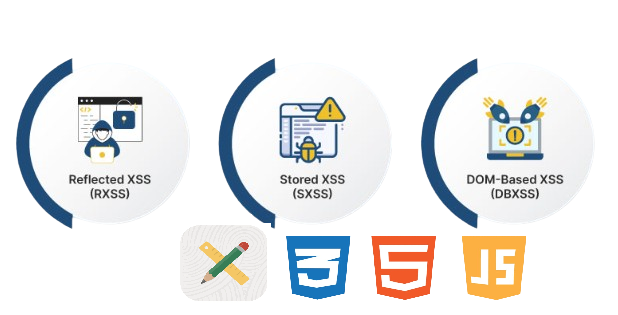
Introduction
In web applications, generating dynamic HTML content securely is crucial to prevent security vulnerabilities, especially Cross-Site Scripting (XSS) attacks. Oracle APEX offers various tools and methods to ensure secure HTML generation. One common approach is to embed HTML directly in SQL queries. However, this can pose significant security risks if not handled properly. This blog post will discuss both the insecure and secure methods of generating HTML content, highlighting the importance of escaping special characters. We will also cover when to use database-level HTML generation and when to avoid it.
What is an XSS Attack?
Cross-Site Scripting (XSS) is a type of security vulnerability typically found in web applications. It allows attackers to inject malicious scripts into web pages viewed by other users. These scripts can steal cookies, session tokens, or other sensitive information, manipulate the appearance of the web page, or perform actions on behalf of the user without their consent. XSS attacks are broadly categorized into three types:
Stored XSS: The malicious script is stored on the server (e.g., in a database) and executed when the user accesses the stored content.
Reflected XSS: The malicious script is reflected off a web server, such as in an error message or search result, and executed immediately.
DOM-based XSS: The malicious script is executed as a result of modifying the DOM environment in the victim’s browser.
Preventing XSS attacks requires careful validation and escaping of any user input that can be rendered as part of the HTML.
Example of Generating HTML Directly in SQL (Insecure Method)
Consider the following SQL query where HTML content is embedded directly:
SELECT
some_column,
other_column,
'<a href="javascript:void(0);" id="' || CERTIFICATE_NUM || '" class="pdf">' ||
'<span class="fa fa-file-pdf-o" style="background-color:#d21e27;color:#fff;padding: 6px;border-radius: 6px;"></span>' ||
'</a>' AS pdf_button
FROM
your_table
This query generates a PDF download button for each row. However, if CERTIFICATE_NUM
contains malicious data, such as a script, it could lead to an XSS attack. For example, if CERTIFICATE_NUM
is 123"><script>alert('XSS')</script>
, the generated HTML would be:
<a href="javascript:void(0);" id="123"><script>alert('XSS')</script>" class="pdf">
<span class="fa fa-file-pdf-o" style="background-color:#d21e27;color:#fff;padding: 6px;border-radius: 6px;"></span>
</a>
When rendered in a browser, this would execute the script, leading to an XSS attack.
Secure Method: Using PL/SQL Function with Escaping
To prevent such vulnerabilities, you can use PL/SQL functions combined with HTF.ESCAPE_SC
to escape special characters. Here’s how you can do it:
- Create a PL/SQL Function to Generate HTML Safely
CREATE OR REPLACE FUNCTION generate_pdf_button(p_certificate_num VARCHAR2)
RETURN VARCHAR2 IS
l_html VARCHAR2(4000);
BEGIN
-- Use HTF.ESCAPE_SC to escape special characters
l_html := '<a href="javascript:void(0);" id="' || htf.escape_sc(p_certificate_num) || '" class="pdf">' ||
'<span class="fa fa-file-pdf-o" style="background-color:#d21e27;color:#fff;padding: 6px;border-radius: 6px;">' ||
'</span></a>';
RETURN l_html;
END generate_pdf_button;
/
- Use the Function in Your SQL Query
SELECT
some_column,
other_column,
generate_pdf_button(CERTIFICATE_NUM) AS pdf_button
FROM
your_table
Example with Escaping Malicious Data
If CERTIFICATE_NUM
is 123"><script>alert('XSS')</script>
, the function will escape the special characters, and the generated HTML will be:
<a href="javascript:void(0);" id="123"><script>alert('XSS')</script>" class="pdf">
<span class="fa fa-file-pdf-o" style="background-color:#d21e27;color:#fff;padding: 6px;border-radius: 6px;"></span>
</a>
Here’s the breakdown of the escaped characters:
"
becomes"
>
becomes>
<
becomes<
'
becomes'
When the above escaped HTML is rendered in the browser, it will not execute the script, thereby preventing the XSS attack. Instead, it will safely display the link without interpreting any malicious code.
When to Use Database-Level HTML Generation
Use Database-Level HTML Generation When:
Dynamic Content is Simple: If the HTML content you need to generate is straightforward and doesn't require complex logic, generating it at the database level can simplify your application.
Centralized Logic: If you want to centralize the HTML generation logic to ensure consistency across your application.
Performance Considerations: Generating HTML in the database can reduce the load on your application server, especially for data-intensive applications.
Avoid Database-Level HTML Generation When:
Complex Layouts and Styles: If the HTML content involves complex layouts, extensive styling, or JavaScript, it's often better to handle this at the application level where you have more control over the presentation.
Separation of Concerns: Mixing HTML generation with data retrieval can make your PL/SQL code harder to maintain. Keeping the presentation logic in the application layer can lead to cleaner, more maintainable code.
Frequent UI Changes: If the HTML structure is subject to frequent changes, it’s easier to manage and update in the application layer rather than modifying database functions repeatedly.
Conclusion
Using HTF.ESCAPE_SC
in your PL/SQL function to escape special characters effectively mitigates the risk of XSS attacks. Disabling escaping in regions can be risky if not handled properly. By ensuring that all dynamic content is sanitized before being rendered, you can maintain the security of your Oracle APEX applications. Always prioritize security by escaping special characters, especially when dealing with user-generated content. Determine whether to generate HTML at the database or application level based on the complexity and maintainability of your code.
Subscribe to my newsletter
Read articles from Azam Sajid directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
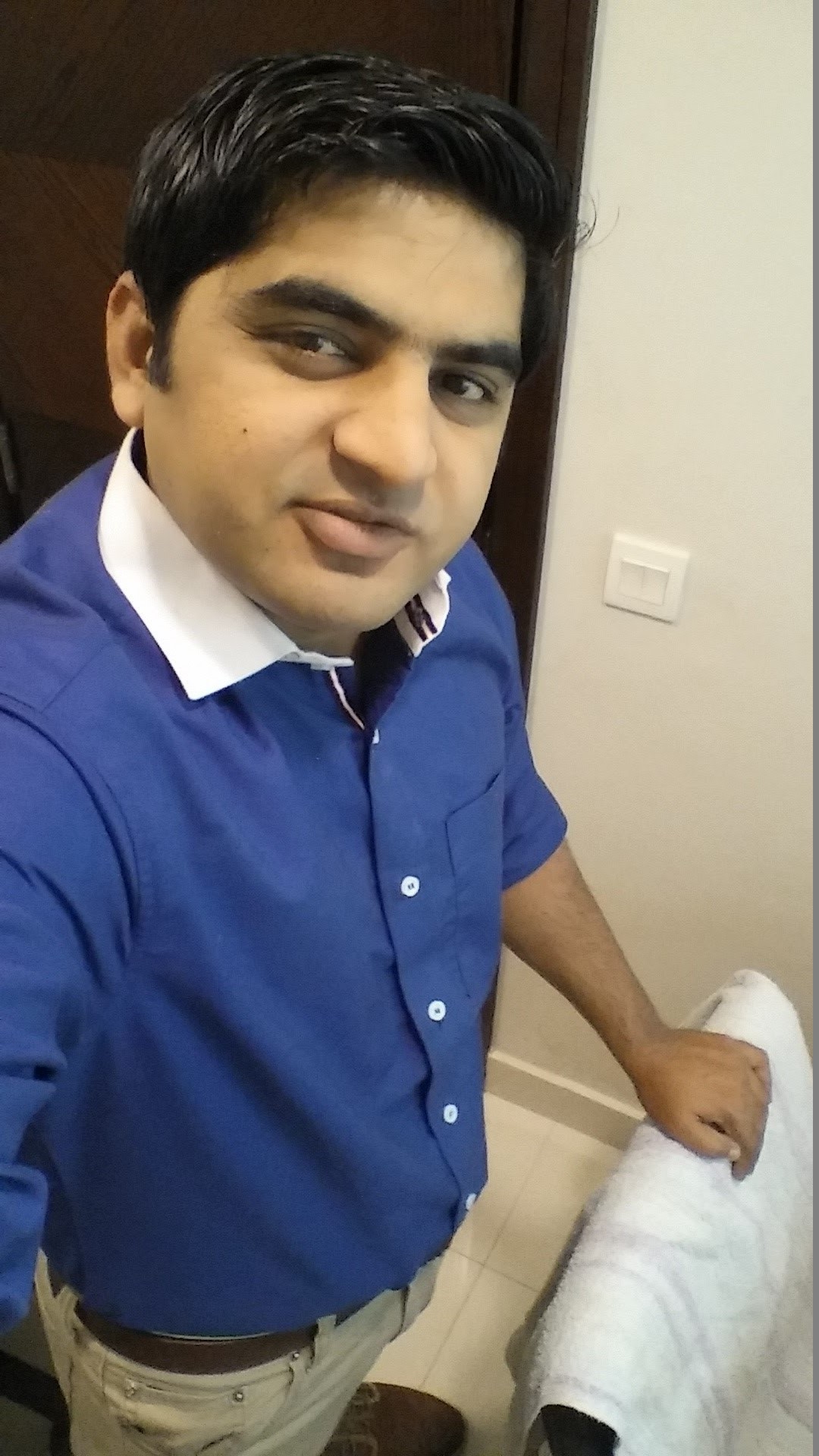
Azam Sajid
Azam Sajid
Experienced Oracle full-stack developer specializing in customizing ERP solutions, developing and training on Oracle APEX applications, and providing data analytics and business intelligence services. Passionate about leveraging technology to drive business success and innovation. Let's connect and create impactful solutions together