Prefix and Postfix Operators in JavaScript
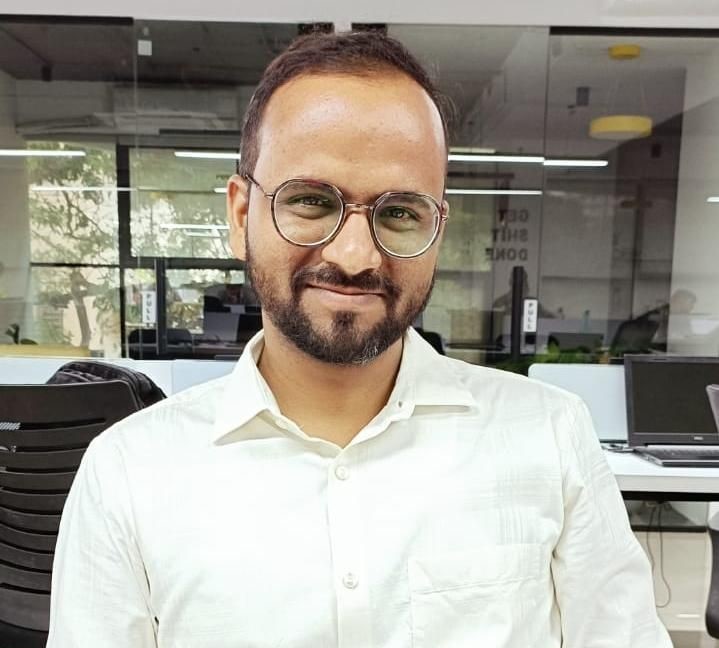
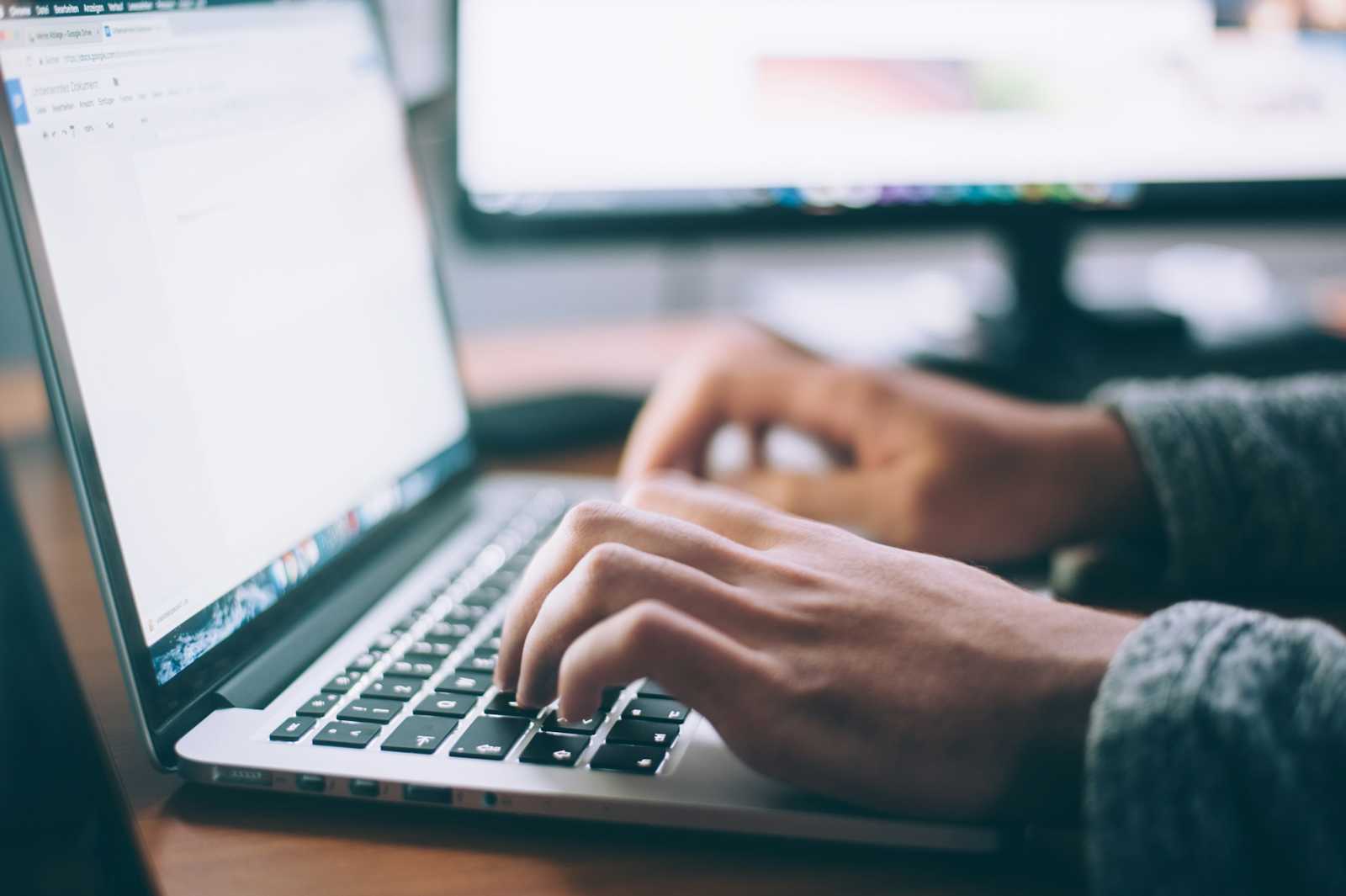
In JavaScript, the increment (++) and decrement (--) operators can be used in two distinct ways: prefix and postfix. These operators are used to increase or decrease the value of a variable by one, but the position of the operator (before or after the variable) changes the order of operations, which affects both the stored value and the value returned by the expression.
Prefix Operators
When the increment or decrement operator is placed before the variable, it is called a prefix operator. With a prefix operator, the variable is incremented or decremented first, and then its new value is used in the expression.
Prefix Increment (++)
The prefix increment operator increases the value of a variable by one and returns the new value.
Example:
let x = 5;
let y = ++x; // x is incremented first, then y is assigned the new value of x
console.log(x); // Output: 6
console.log(y); // Output: 6
In this example:
x
starts with the value of 5.The
++x
operation incrementsx
to 6.y
is then assigned the value ofx
, which is now 6.
Prefix Decrement (--)
The prefix decrement operator decreases the value of a variable by one and returns the new value.
Example:
let m = 5;
let n = --m; // m is decremented first, then n is assigned the new value of m
console.log(m); // Output: 4
console.log(n); // Output: 4
In this example:
m
starts with the value of 5.The
--m
operation decrementsm
to 4.n
is then assigned the value ofm
, which is now 4.
Postfix Operators
When the increment or decrement operator is placed after the variable, it is called a postfix operator. With a postfix operator, the current value of the variable is used in the expression first, and then the variable is incremented or decremented.
Postfix Increment (++)
The postfix increment operator increases the value of a variable by one but returns the original value before the increment.
Example:
let a = 5;
let b = a++; // b is assigned the value of a first, then a is incremented
console.log(a); // Output: 6
console.log(b); // Output: 5
In this example:
a
starts with the value of 5.The
a++
operation assigns the current value ofa
(which is 5) tob
.Then,
a
is incremented to 6.
Postfix Decrement (--)
The postfix decrement operator decreases the value of a variable by one but returns the original value before the decrement.
Example:
let p = 5;
let q = p--; // q is assigned the value of p first, then p is decremented
console.log(p); // Output: 4
console.log(q); // Output: 5
In this example:
p
starts with the value of 5.The
p--
operation assigns the current value ofp
(which is 5) toq
.Then,
p
is decremented to 4.
Key Differences
Prefix (++x or --x): The variable is incremented/decremented first, and the updated value is used in the expression.
Postfix (x++ or x--): The current value of the variable is used in the expression first, and then the variable is incremented/decremented.
Practical Usage
Understanding these differences is crucial in scenarios such as loops, where the timing of the increment or decrement can impact the loop's behavior.
Example with Loops:
Using prefix in a loop:
for (let i = 0; i < 5; ++i) {
console.log(i); // Outputs: 0, 1, 2, 3, 4
}
Using postfix in a loop:
for (let i = 0; i < 5; i++) {
console.log(i); // Outputs: 0, 1, 2, 3, 4
}
In both cases, the output is the same because the increment operation happens at the end of each loop iteration. However, understanding the difference between prefix and postfix can be essential in more complex expressions.
By mastering the prefix and postfix operators, you can write more precise and effective JavaScript code, ensuring that your operations perform exactly as intended.
Subscribe to my newsletter
Read articles from Indrajeet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
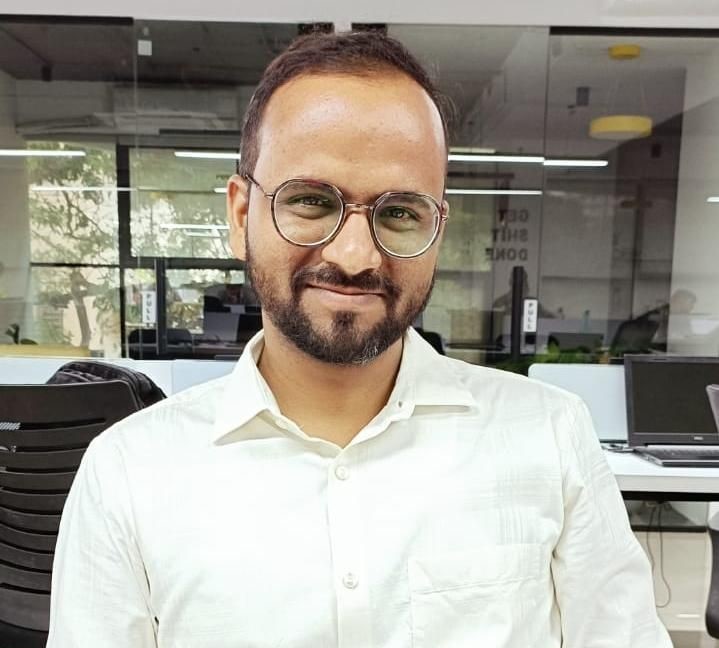
Indrajeet
Indrajeet
Hello there! My name is Indrajeet, and I am a skilled web developer passionate about creating innovative, user-friendly, and dynamic web applications. 📌My Expertise advanced proficiency in angular front-End Development. Back-End Development Leveraging the power of .NET, I build secure, robust, and scalable server-side architectures.