React Native: Touchable Opacity vs Button Element Explained
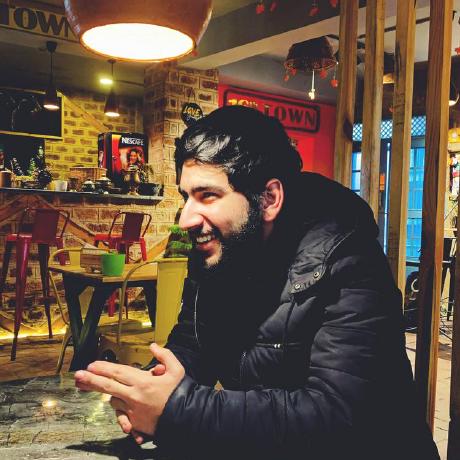
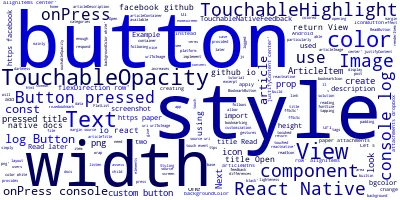
In the realm of mobile app development, React Native offers a variety of components to cater to different interactive needs. Two commonly used components for handling touch events are TouchableOpacity
and Button
. Both serve to provide interactive elements, but they come with their own sets of features and use cases. This article delves into the differences, advantages, and best use scenarios for TouchableOpacity
and Button
.
TouchableOpacity
TouchableOpacity
is a wrapper for making views respond properly to touches. When a touch is detected, the opacity of the wrapped view is decreased, dimming the view. This feedback gives users a visual cue that their touch has been registered. Here's a closer look at TouchableOpacity
Features and Benefits
Flexibility:
TouchableOpacity
can wrap any React Native component, making it incredibly versatile. This means you can use it to make text, images, or even complex views respond to touch events.Visual Feedback: The opacity change on touch provides immediate feedback, enhancing user experience.
Customizability: Since it’s a wrapper, you can style the content inside as you wish. This gives developers more control over the design and functionality.
import React from 'react';
import { TouchableOpacity, Text, StyleSheet } from 'react-native';
const App = () => (
<TouchableOpacity style={styles.button} onPress={() => alert('Button Pressed!')}>
<Text style={styles.text}>Touchable Opacity Button</Text>
</TouchableOpacity>
);
const styles = StyleSheet.create({
button: {
backgroundColor: '#2196F3',
padding: 10,
borderRadius: 5,
},
text: {
color: '#fff',
textAlign: 'center',
},
});
export default App;
Button
The Button
component in React Native is a basic, ready-to-use button with a pre-defined look and feel. It is designed to be simple and easy to implement, providing essential functionality without requiring extensive customization.
Features and Benefits
Simplicity:
Button
is straightforward to use. You only need to provide a title and an onPress function.Cross-Platform Consistency:
Button
provides a consistent look and feel across both iOS and Android, adhering to platform-specific design guidelines.Ease of Use: For developers looking for a quick and easy way to implement a button,
Button
is ideal.
import React from 'react';
import { Button, View, StyleSheet } from 'react-native';
const App = () => (
<View style={styles.container}>
<Button
title="Press Me"
onPress={() => alert('Button Pressed!')}
color="#841584"
/>
</View>
);
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
export default App;
Comparison
Use Case Scenarios
Custom Designs: If your application requires custom-designed buttons or touchable areas,
TouchableOpacity
is the way to go. It allows for greater flexibility in terms of design and layout.Simplicity and Speed: If you need a button with a standard look and feel quickly,
Button
is the most efficient choice. It requires minimal setup and provides a consistent user experience across platforms.
Performance
Both TouchableOpacity
and Button
are optimized for performance in React Native. However, TouchableOpacity
might have a slight edge in scenarios where complex touch handling and animations are involved, as it offers more control over the touchable area.
Customizability
TouchableOpacity: Offers high customizability. You can style and animate the content inside the touchable area as you wish.
Button: Limited in terms of customization. You can only set the title, color, and handle the press event.
Conclusion
Choosing between TouchableOpacity
and Button
in React Native largely depends on your specific needs. TouchableOpacity
is ideal for custom touchable elements requiring specific designs and interactions, while Button
is perfect for quick, standard button implementations. Understanding the strengths and limitations of each component allows developers to make informed decisions, ensuring the best user experience and development efficiency.
In practice, many applications use both components, leveraging the simplicity of Button
for standard actions and the flexibility of TouchableOpacity
for more customized interactions. As React Native continues to evolve, these components will likely see further enhancements, making them even more powerful and versatile for mobile app development.
Subscribe to my newsletter
Read articles from Syed Dayim Shah directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
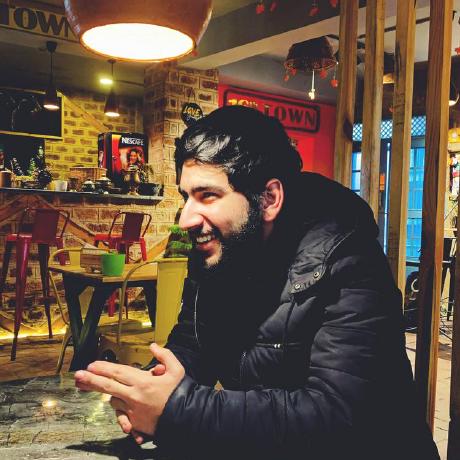
Syed Dayim Shah
Syed Dayim Shah
Hello, My name is Syed Dayim Shah. I Specialize in Software, Mobile Apps and API development/Integration. Python and JavaScript are my go-to Languages. I'm also a DSA Lover. I am currently working as a Developer. I've done Computer Science Engineering. I am interested in Gardening. I like to play Chess and badminton. In Computer gaming i like RPG and Racing games. Thank you