Python and IoT: Building Smart Devices with MicroPython
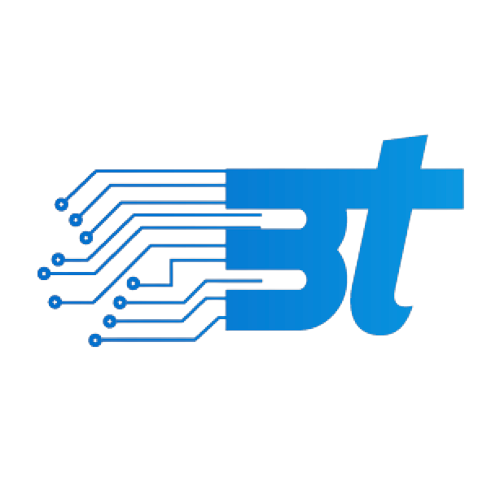
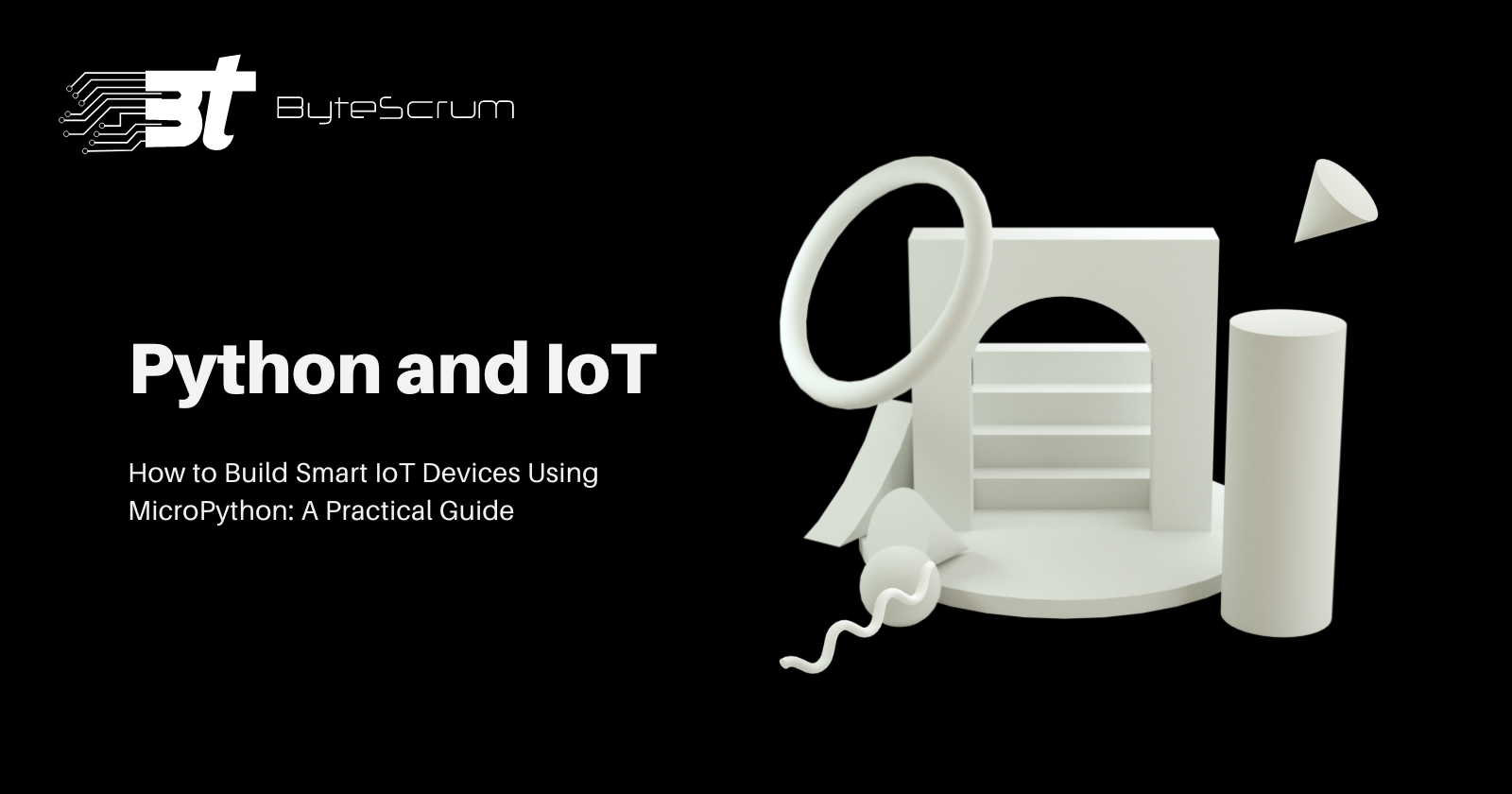
Introduction
The Internet of Things (IoT) is revolutionizing the way we interact with the world around us. From smart homes to industrial automation, IoT devices are becoming increasingly prevalent. In this blog post, we will explore how to use Python, specifically MicroPython, to build smart devices for IoT applications. MicroPython is a lean and efficient implementation of Python3 that is optimized to run on microcontrollers and other resource-constrained devices.
Setting Up MicroPython
Choosing a Microcontroller
Before diving into code, you need a microcontroller that supports MicroPython. Popular choices include:
ESP8266
ESP32
Pyboard
For this tutorial, we'll use the ESP32 due to its robust features and support for Wi-Fi and Bluetooth.
Installing MicroPython on ESP32
Download the MicroPython Firmware:
Visit the MicroPython download page.
Download the latest stable release for ESP32.
Flash the Firmware to the ESP32:
Install the esptool.py using pip:
pip install esptool
Erase the flash memory of the ESP32:
esptool.py --port /dev/ttyUSB0 erase_flash
Flash the MicroPython firmware to the ESP32:
esptool.py --chip esp32 --port /dev/ttyUSB0 --baud 460800 write_flash -z 0x1000 esp32-20220117-v1.18.bin
Writing Your First MicroPython Script
Connecting to the ESP32
To interact with your ESP32, you'll need a serial communication tool. You can use picocom
, minicom
, or the built-in REPL in the Thonny IDE. For this example, we'll use picocom
:
picocom /dev/ttyUSB0 -b 115200
Hello World in MicroPython
Let's start with a simple "Hello, World!" script to ensure everything is set up correctly.
Open the REPL by connecting to your ESP32.
Type the following code:
print("Hello, World!")
Building a Smart Device
Example 1: Controlling an LED
Connecting an LED to the ESP32
Wiring the LED:
Connect the anode (long leg) of the LED to GPIO 2.
Connect the cathode (short leg) of the LED to a resistor (220 ohms).
Connect the other end of the resistor to GND.
Writing the LED Control Script
Open the REPL.
Enter the following code:
from machine import Pin import time led = Pin(2, Pin.OUT) while True: led.on() time.sleep(1) led.off() time.sleep(1)
This script will blink the LED on and off every second.
Example 2: Reading a Temperature Sensor
Connecting a DHT22 Sensor
Wiring the DHT22:
Connect the VCC pin to the 3.3V pin on the ESP32.
Connect the GND pin to the GND pin on the ESP32.
Connect the Data pin to GPIO 4.
Writing the Temperature Sensor Script
Install the DHT library:
import upip upip.install('micropython-umqtt.simple')
Enter the following code:
import dht import machine import time sensor = dht.DHT22(machine.Pin(4)) while True: sensor.measure() temp = sensor.temperature() hum = sensor.humidity() print('Temperature: {}°C Humidity: {}%'.format(temp, hum)) time.sleep(2)
This script will read and print the temperature and humidity every two seconds.
Connecting to Wi-Fi
One of the powerful features of the ESP32 is its Wi-Fi capability. Let's connect our device to a Wi-Fi network.
import network
ssid = 'your_SSID'
password = 'your_password'
station = network.WLAN(network.STA_IF)
station.active(True)
station.connect(ssid, password)
while station.isconnected() == False:
pass
print('Connection successful')
print(station.ifconfig())
Sending Data to the Cloud
Let's send sensor data to a cloud service. For this example, we'll use the Adafruit IO service.
Install the Adafruit IO library:
import upip upip.install('adafruit-io')
Send Data to Adafruit IO:
from umqtt.simple import MQTTClient import ubinascii import machine import micropython import time # Adafruit IO configuration ADAFRUIT_IO_URL = b'io.adafruit.com' ADAFRUIT_USERNAME = b'your_username' ADAFRUIT_IO_KEY = b'your_aio_key' FEED_ID = b'temperature' # ESP32 unique ID CLIENT_ID = ubinascii.hexlify(machine.unique_id()) def connect_to_adafruit(): client = MQTTClient(CLIENT_ID, ADAFRUIT_IO_URL, user=ADAFRUIT_USERNAME, password=ADAFRUIT_IO_KEY) client.connect() return client client = connect_to_adafruit() while True: temperature = 25 # Replace with sensor reading payload = bytes(str(temperature), 'utf-8') client.publish(b'{}/feeds/{}'.format(ADAFRUIT_USERNAME, FEED_ID), payload) time.sleep(10)
💡The information provided in this blog is for educational purposes only. Ensure you follow all safety guidelines and legal regulations when working with electronic components and IoT devices. The author and publisher assume no responsibility for any errors or omissions or for any damages resulting from the use of the information contained herein.
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
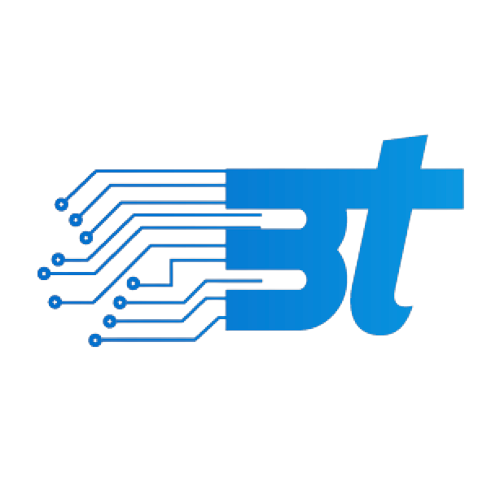
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.