Yup and Formic

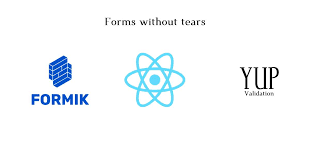
Today, we'll explore using Yup and Formic together in React. Learn how to simplify form validation and state management with these powerful libraries.
What are Yup and Formic?
Yup :-
Yup is a JavaScript schema builder for value parsing and validation. It allows developers to define object schemas with a rich set of validation rules to ensure data integrity. Yup is often used in form handling to validate user inputs and ensure they meet specified criteria before being submitted or processed.
Formic :-
Formic is a popular library for building and managing forms in React applications. It simplifies form state management, validation, and submission, providing developers with easy-to-use components and hooks to create complex forms with minimal
boilerplate code.
Purpose of Yup
Schema-Based Validation: Yup allows developers to create schemas that define the structure and constraints of their data. This schema-based approach makes it easy to handle complex validation logic.
Custom Validations: Yup supports custom validation methods, enabling developers to create tailored validation rules to meet specific application requirements.
Integration with Forms: Yup can be integrated with form libraries like Formic to handle validation logic seamlessly.
Purpose of Formic :-
Form State Management: Formic manages the state of form fields, including their values, errors, and touched status, reducing the need for manual state handling.
Validation Integration: Formic integrates easily with validation libraries like Yup, allowing for declarative validation rules within the form components.
Simplified Form Handling: Formic provides built-in components like
<Formik>
,<Form>
,<Field>
, and<ErrorMessage>
to streamline the creation and management of forms.
Why They Are Used Together :-
Seamless Validation: Using Yup with Formic allows developers to define validation schemas separately from the form logic. Formic’s
validationSchema
prop directly integrates with Yup schemas, providing real-time validation feedback as users interact with the form.Maintainable Code: Combining Yup and Formic results in cleaner and more maintainable code. The separation of concerns between form state management (handled by Formic) and validation logic (handled by Yup) leads to more organized and readable code.
Enhanced User Experience: The integration of Yup and Formic enhances the user experience by providing immediate validation feedback, preventing form submission until all inputs are valid, and ensuring that the data meets the required criteria before processing.
Basic Usage
Creating a Basic Form with Formik and Yup :-
Step 1: Install Dependencies
npm install formik yup
Step 2: Set Up Formik and Yup
Create a form component that utilizes formik
for form state management and yup
for validation.
import React from 'react';
import { Formik, Form, Field, ErrorMessage } from 'formik';
import * as Yup from 'yup';
// Define validation schema using Yup
const validationSchema = Yup.object({
firstName: Yup.string()
.max(15, 'Must be 15 characters or less')
.required('Required'),
lastName: Yup.string()
.max(20, 'Must be 20 characters or less')
.required('Required'),
email: Yup.string()
.email('Invalid email address')
.required('Required'),
});
// Define the initial values for the form fields
const initialValues = {
firstName: '',
lastName: '',
email: '',
};
// Define the onSubmit handler
const onSubmit = (values, { setSubmitting }) => {
setTimeout(() => {
alert(JSON.stringify(values, null, 2));
setSubmitting(false);
}, 400);
};
const SignupForm = () => (
<Formik
initialValues={initialValues}
validationSchema={validationSchema}
onSubmit={onSubmit}
>
{({ isSubmitting }) => (
<Form>
<div>
<label htmlFor="firstName">First Name</label>
<Field name="firstName" type="text" />
<ErrorMessage name="firstName" component="div" />
</div>
<div>
<label htmlFor="lastName">Last Name</label>
<Field name="lastName" type="text" />
<ErrorMessage name="lastName" component="div" />
</div>
<div>
<label htmlFor="email">Email Address</label>
<Field name="email" type="email" />
<ErrorMessage name="email" component="div" />
</div>
<button type="submit" disabled={isSubmitting}>
Submit
</button>
</Form>
)}
</Formik>
);
export default SignupForm;
Quick Catch :-
Import Libraries: We import
Formik
,Form
,Field
, andErrorMessage
fromformik
, and* as Yup
fromyup
.Validation Schema: Using
yup
, we define a schema for the form validation. The schema defines rules such as maximum length and required fields.Initial Values: We specify the initial values of the form fields, which are empty strings in this case.
onSubmit Function: This function is called when the form is submitted. It receives the form values and a set of helper functions. Here, we simulate a form submission with a delay using
setTimeout
.Formik Component: We use the
Formik
component to wrap our form. We passinitialValues
,validationSchema
, andonSubmit
toFormik
.Form Component: Inside
Formik
, we define the form usingForm
,Field
, andErrorMessage
components.1 .Field
: This component connects the form inputs toformik
state.2 .ErrorMessage
: This component displays validation error messages.
Submit Button: The submit button is disabled while the form is submitting to prevent multiple submissions.
How to conditionally validate fields :-
Yup allows conditional validation using the when
method. For example, if you have a form where a password
field should only be required when a hasPassword
checkbox is checked.
const validationSchema = Yup.object({
hasPassword: Yup.boolean(),
password: Yup.string()
.when('hasPassword', {
is: true,
then: Yup.string()
.required('Password is required')
.min(8, 'Password must be at least 8 characters long'),
otherwise: Yup.string(),
}),
});
In this schema, the password
field is required and must be at least 8 characters long only if the hasPassword
field is true
.
How you can customize error messages in Yup :-
You can customize error messages in Yup by passing a message string to the validation method. Each validation method accepts an optional message parameter that will be displayed when the validation fails. Here is an example
import * as Yup from 'yup';
const validationSchema = Yup.object({
name: Yup.string().required('Please enter your name'),
email: Yup.string().email('Enter a valid email').required('Email is required'),
age: Yup.number()
.positive('Age must be a positive number')
.integer('Age must be a whole number')
.required('Please enter your age'),
});
In this example, each validation rule has a custom error message that will be shown to the user if the input does not meet the specified criteria.
Hope this will help you. I tried to cover some important points comment if you have any doubt we'll discuss .
Subscribe to my newsletter
Read articles from Yashaswi Sahu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
