Managing Growing Projects with Packages, Crates, and Modules in Rust
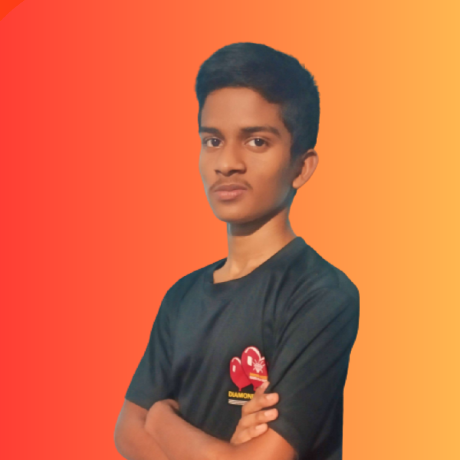
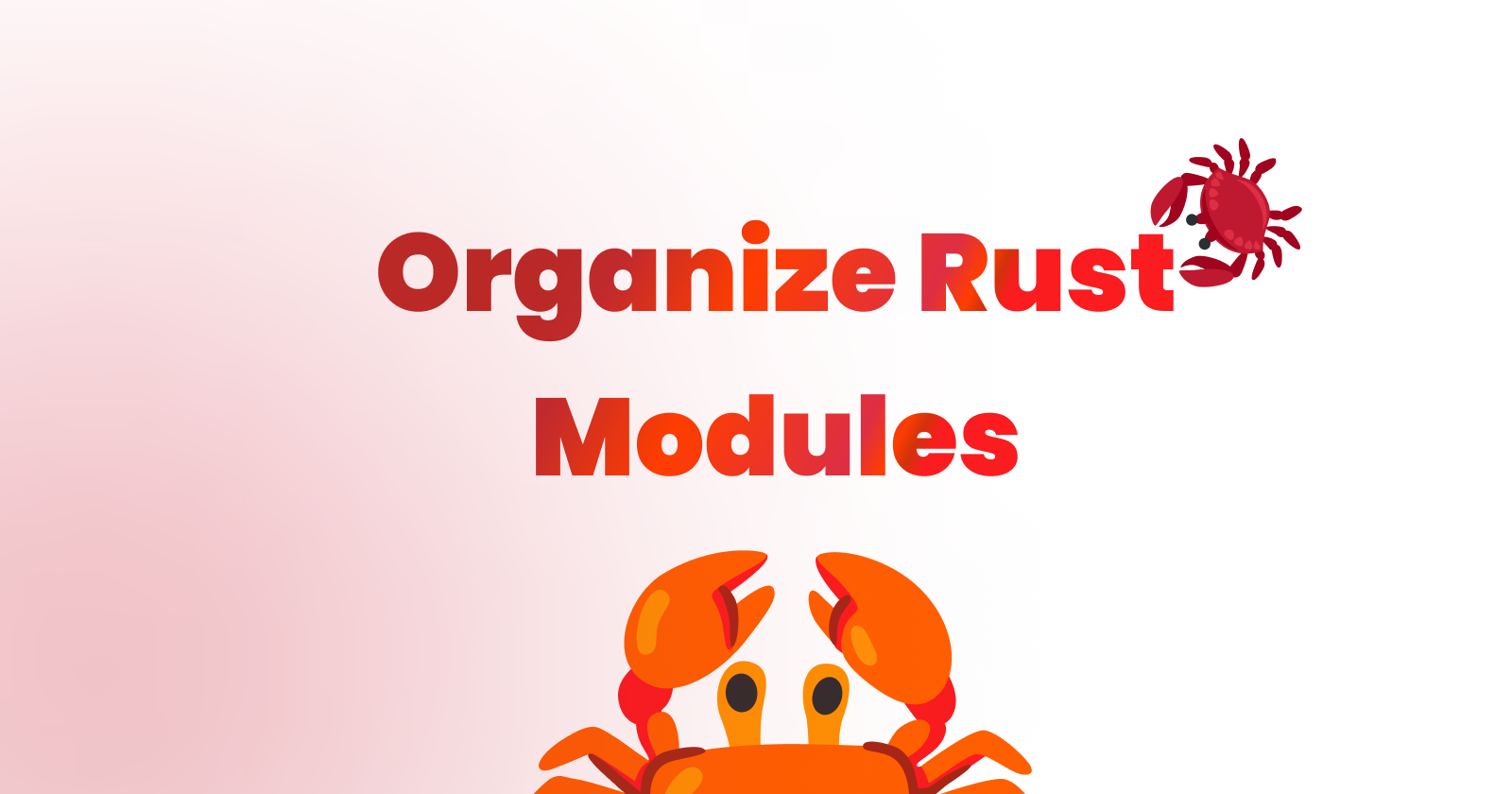
As your Rust projects grow in size and complexity, organizing your code becomes increasingly crucial. This chapter delves into the tools Rust provides to manage this growth effectively, focusing on the concepts of packages, crates, modules, and scope.
Why Organize?
Organizing your code offers several benefits:
Clarity: Grouping related functionality and separating distinct features makes it easier to locate and understand specific code sections.
Maintainability: Well-organized code simplifies modifications and updates, reducing the risk of introducing errors.
Reusability: Encapsulation of implementation details allows you to reuse code components without exposing their inner workings.
Introducing the Module System
Rust offers a powerful module system to manage code organization and scope. Key components include:
Packages: A Cargo feature for building, testing, and sharing crates.
Crates: A tree of modules that produces a library or executable.
Modules and
use
: Mechanisms for controlling the organization, scope, and privacy of paths.Paths: A way to name items like structs, functions, and modules.
Encapsulation and Scope
Encapsulation refers to hiding implementation details and exposing only a public interface. This allows other parts of your code to interact with a component without needing to know its internal workings.
Scope defines the context in which code is written. Each scope has a set of defined names, and the compiler needs to understand whether a name refers to a variable, function, struct, or other item. Rust's module system helps manage scope by defining which names are accessible within a particular context.
This chapter will explore these features in detail, demonstrating how they interact and how to use them to effectively manage your code's organization and scope. By the end, you'll have a solid understanding of the Rust module system and be able to work with scopes confidently.
Packages and Crates
"Rust's package manager, Cargo, makes it incredibly easy to manage dependencies and build projects. It's a huge time-saver for developers."
This section introduces the fundamental concepts of packages and crates within Rust's module system.
Crates: The Building Blocks of Rust Code
"Crates are like building blocks that you can use to create complex applications. They allow you to reuse code and build upon the work of others."
A crate represents the smallest unit of code that the Rust compiler processes at a time. Even a single source file, when compiled with rustc
, is considered a crate. Crates can contain modules, which can be defined in separate files that are compiled together.
Two Types of Crates:
Binary Crates: These crates compile into executable programs, such as command-line tools or servers. They must have a
main
function that defines the program's entry point. All the examples we've seen so far have been binary crates.Library Crates: These crates don't compile to executables. Instead, they define reusable functionality that can be shared across multiple projects. For example, the
rand
crate provides random number generation functionality.
The Crate Root:
The crate root is a source file that the Rust compiler uses as the starting point for a crate. It defines the root module of the crate.
Packages: Bundling Related Crates
"Packages are like containers for crates, allowing you to group related functionality and share it as a cohesive unit."
A package is a collection of one or more crates that work together to provide a set of functionality. Packages are described by a Cargo.toml
file, which outlines how to build the crates within the package.
Package Structure:
Cargo itself: The Cargo tool you use to build and manage Rust projects is a package containing both a binary crate (the command-line tool) and a library crate.
Package Contents: A package can contain multiple binary crates and at most one library crate. It must contain at least one crate.
File Organization:
Creating a Package with Cargo:
The cargo new
command creates a new package. For example:
$ cargo new my-project
Created binary (application) `my-project` package
$ ls my-project
Cargo.toml
src
$ ls my-project/src
main.rs
This creates a package named my-project
with a Cargo.toml
file and a src
directory containing main.rs
. This package contains a single binary crate named my-project
.
Packages with Multiple Crates:
- "Packages make it easier to manage dependencies, as you can specify a single package instead of listing out individual crates."
If a package contains both src/
main.rs
and src/
lib.rs
, it has two crates: a binary crate and a library crate, both with the same name as the package. You can create multiple binary crates by placing additional files within the src/bin
directory.
This section provides a foundational understanding of packages and crates, setting the stage for exploring modules and scope in the following sections.
Defining Modules to Control Scope and Privacy
- "Modules help to enforce encapsulation and prevent unintended access to your code, promoting better code organization and security."
Let's dive into the world of modules! Modules are like containers that organize your code, making it easier to manage and understand. They also help you control which parts of your code are visible to other parts, ensuring a clean and secure structure.
Imagine a Library:
Think of a library with different sections: fiction, non-fiction, children's books, etc. Each section keeps related books together, making it easier to find what you're looking for. Modules are similar: they group related functions, structs, and other items together within your code.
Why Use Modules?
Organization: Modules help you structure your code logically, making it easier to navigate and understand.
Privacy: You can control which parts of your code are accessible from other parts. This is important for keeping implementation details hidden and preventing unintended modifications.
Reusability: Modules can be reused in different parts of your project or even in other projects, promoting code sharing and reducing redundancy.
Defining Modules:
You define a module using the mod
keyword followed by the module name:
mod my_module {
// Code inside the module
}
Accessing Items Within a Module:
To access items (functions, structs, etc.) defined within a module, you use the module name followed by a double colon (::):
mod my_module {
pub fn my_function() {
println!("Hello from my_function!");
}
}
fn main() {
my_module::my_function(); // Calling the function from the module
}
Privacy Control with pub
:
"Rust's module system is designed to make it easy to manage the scope and visibility of your code, promoting better code organization and maintainability."
By default, items within a module are private, meaning they can only be accessed from within the module itself. To make an item public, you use the pub
keyword:
mod my_module {
pub fn my_function() {
// ...
}
fn private_function() {
// ...
}
}
fn main() {
my_module::my_function(); // Allowed - public function
// my_module::private_function(); // Error - private function
}
Nested Modules:
You can create nested modules for even more organized code:
mod my_module {
mod inner_module {
pub fn inner_function() {
// ...
}
}
}
fn main() {
my_module::inner_module::inner_function();
}
Key Points:
Modules are containers that group related code.
pub
makes items accessible from outside the module.Modules can be nested for even better organization.
By using modules, you can create a well-structured and maintainable codebase, making your Rust projects easier to work with and understand.
Let's break down how to use paths in Rust to refer to items within your module tree, making it easier to understand for beginners.
Imagine a Restaurant
Think of a restaurant with different sections:
Front of House: Where customers interact (like ordering).
Back of House: Where the kitchen and staff work (like preparing food).
In Rust, these sections are like modules. Modules help organize your code into logical groups.
Paths: Navigating Your Restaurant
To find something in the restaurant, you need directions, right? Rust uses paths to find items (like functions, structs, or enums) within your code.
Types of Paths:
Absolute Path: Like giving a full address from the restaurant's entrance.
Starts with
crate::
(for your current project) or the name of an external crate.Example:
crate::front_of_house::hosting::add_to_waitlist()
(This means: "Go to thefront_of_house
module, then thehosting
module, and finally call theadd_to_waitlist
function.")
Relative Path: Like giving directions from where you are currently standing.
Starts with a module name or
self
(for the current module) orsuper
(for the parent module).Example:
front_of_house::hosting::add_to_waitlist()
(This means: "Starting from where I am now, go to thefront_of_house
module, then thehosting
module, and finally call theadd_to_waitlist
function.")
Privacy in Your Restaurant
Private: Like the back of the house, where customers can't go. By default, all items in Rust are private to their parent module.
Public: Like the front of the house, open to everyone. Use the
pub
keyword to make items accessible outside their module.
Example:
mod front_of_house {
pub mod hosting {
pub fn add_to_waitlist() {
// ... do something to add a customer to the waitlist
}
}
}
pub fn eat_at_restaurant() {
// Absolute path
crate::front_of_house::hosting::add_to_waitlist();
// Relative path
front_of_house::hosting::add_to_waitlist();
}
Explanation:
mod front_of_house
: Creates a module calledfront_of_house
.pub mod hosting
: Creates a public module calledhosting
insidefront_of_house
.pub fn add_to_waitlist
: Creates a public function calledadd_to_waitlist
insidehosting
.pub fn eat_at_restaurant
: Creates a public function calledeat_at_restaurant
in the main part of your code.crate::front_of_house::hosting::add_to_waitlist()
: Uses an absolute path to call theadd_to_waitlist
function.front_of_house::hosting::add_to_waitlist()
: Uses a relative path to call theadd_to_waitlist
function.
Key Points:
pub
: Makes items visible to code outside their module.crate::
: Starts an absolute path from the root of your project.self
: Refers to the current module.super
: Refers to the parent module.
Remember: Organize your code into modules and use paths to navigate between them. Use pub
to control which parts of your code are accessible from other parts.
Bringing Paths Into Scope with the use
Keyword
Imagine you're at a restaurant and you want to order a specific dish. You could ask the waiter for the "Grilled Salmon with Lemon Butter Sauce" – that's the full name, but it's a bit long! You could also just say "Salmon," because everyone knows what you mean.
In Rust, the use
keyword is like a nickname for a path. It lets you shorten long paths and make your code more readable.
Example:
mod front_of_house {
pub mod hosting {
pub fn add_to_waitlist() {}
}
}
pub fn eat_at_restaurant() {
// Long and repetitive:
crate::front_of_house::hosting::add_to_waitlist();
// With use:
use crate::front_of_house::hosting;
hosting::add_to_waitlist();
}
Explanation:
use crate::front_of_house::hosting;
: This line brings thehosting
module into scope. Now, instead of typing the full pathcrate::front_of_house::hosting
, you can just usehosting
.hosting::add_to_waitlist();
: This calls theadd_to_waitlist
function within thehosting
module.
Benefits of use
:
Shorter Code: Makes your code more concise and easier to read.
Less Repetition: Avoids typing the same long paths over and over.
Improved Organization: Groups related items together, making your code more structured.
Using use
with as
:
You can also use as
to create custom aliases for paths:
use std::collections::HashMap as MyHashMap;
fn main() {
let mut map = MyHashMap::new();
// ...
}
Key Points:
Scope: The
use
keyword brings items into scope within the current module.as
: Lets you create custom aliases for paths.Best Practices: Use
use
to make your code more readable and organized.
Think of use
as a way to create shortcuts in your restaurant. You can use these shortcuts to easily find the items you need, without having to remember the full, long paths.
Separating Modules into Different Files
As your Rust projects grow, you'll find that keeping all your code in a single file becomes cumbersome. Rust provides a way to organize your code by separating modules into different files, making your project more manageable.
Think of it like organizing your restaurant:
Small Restaurant: You can keep everything in one room – the kitchen, dining area, and staff room.
Large Restaurant: You need to separate these areas into different rooms to keep things organized and efficient.
How to Separate Modules:
Create a Module Declaration: In your main file (usually
src/
lib.rs
orsrc/
main.rs
), declare the module you want to move. For example:mod front_of_house;
Create a Separate File: Create a new file with the same name as the module (e.g.,
src/front_of_
house.rs
). Move the code for the module into this file.Organize Submodules: If your module has submodules, create a directory with the same name as the parent module and place the submodule files inside. For example:
src/ front_of_house/ hosting.rs
Example:
Let's say you have a module called front_of_house
with a submodule called hosting
:
src/
lib.rs
(main file):mod front_of_house; pub use crate::front_of_house::hosting; pub fn eat_at_restaurant() { hosting::add_to_waitlist(); }
src/front_of_
house.rs
:pub mod hosting;
src/front_of_house/
hosting.rs
:pub fn add_to_waitlist() {}
Key Points:
mod
: Declares a module in your main file.File Naming: The file name should match the module name.
Directory Structure: Use directories to organize submodules.
pub
: Use thepub
keyword to make items accessible from other modules.
Benefits of Separating Modules:
Improved Organization: Makes your code easier to navigate and understand.
Modularity: Allows you to reuse code in different parts of your project.
Reduced Complexity: Makes it easier to work on individual parts of your project.
Remember: Use mod
to declare modules, create separate files for each module, and use directories to organize submodules. This will help you keep your Rust projects clean and efficient!
Common Beginner Doubts: "Why Do I Need to Separate Modules into Files?"
It's understandable to wonder why you need to separate modules into different files when you're starting out with Rust. After all, it seems like extra work, and you might be thinking, "Can't I just keep everything in one file?"
Here's why separating modules into files is a good idea, even for beginners:
Organization: As your code grows, a single file becomes a tangled mess. Separating modules into files makes your code much easier to navigate and understand.
- Think of it like a library: Would you want to find a specific book in a library where all the books were piled on top of each other? No, you'd want them organized by subject, author, or genre. Modules are like the different sections of a library, helping you find what you need easily.
Modularity: Separating modules allows you to reuse code in different parts of your project without having to copy and paste it.
- Imagine a restaurant menu: You have a section for appetizers, another for main courses, and so on. Each section has its own set of dishes. You can reuse these dishes in different menus (like a lunch menu or a dinner menu) without having to create new dishes each time.
Collaboration: When working with others on a project, it's much easier to manage code changes if each module is in a separate file.
- Think of a team building a house: Each team member might be responsible for a different part of the house (foundation, walls, roof). If everyone is working on the same blueprint, it's easy to get confused and make mistakes. But if each team member has their own blueprint for their specific part of the house, it's much easier to coordinate and avoid problems.
Solution:
Start Small: Don't worry about separating modules into files until your project gets large enough that it becomes difficult to manage. "The journey of a thousand miles begins with a single step." - Lao Tzu
Use
mod
: Start by declaring modules within your main file using themod
keyword. This will help you organize your code even if you don't separate it into files right away.Gradually Migrate: As your project grows, gradually move modules into separate files. Start with the largest or most complex modules first.
Remember: Separating modules into files is a best practice that will help you write cleaner, more maintainable code in the long run. It might seem like extra work at first, but it will pay off as your projects grow!
References
This article draws information from the following sources:
The Rust Programming Language Book: This book is the official guide to learning Rust. It covers a wide range of topics, including modules, organization, and best practices. You can find it here: https://doc.rust-lang.org/book/
Chapter 7: Managing Growing Projects with Packages, Crates, and Modules: This chapter of the Rust Programming Language Book specifically focuses on the concepts of packages, crates, and modules, including how to separate modules into different files. You can find it here: https://doc.rust-lang.org/book/ch07-05-separating-modules-into-different-files.html
Rust Documentation: The official Rust documentation provides comprehensive information about the language, including its syntax, features, and libraries. You can find it here: https://doc.rust-lang.org/
These resources are invaluable for learning and understanding Rust. They provide detailed explanations, examples, and best practices for writing effective Rust code.
Conclusion: Organizing Your Rust Code for Success
As you progress in your Rust journey, you'll find that separating modules into different files becomes an essential practice for managing larger projects. It's not just about keeping your code tidy; it's about creating a structure that promotes clarity, reusability, and collaboration.
Remember, starting small is key. Begin by organizing your code with mod
declarations even if you don't immediately create separate files. As your project grows, gradually migrate modules to their own files, starting with the most complex or frequently used ones.
By embracing modularity, you'll not only improve the readability and maintainability of your code but also lay the foundation for building robust and scalable Rust applications. So, embrace the power of organization and watch your Rust projects flourish!
Enjoyed this article?
❤️ Like it!
🔁 Share it with your fellow Rustaceans!
🤝 Follow me on X/Twitter for more Rust content!
Subscribe to my newsletter
Read articles from Ashfiquzzaman Sajal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
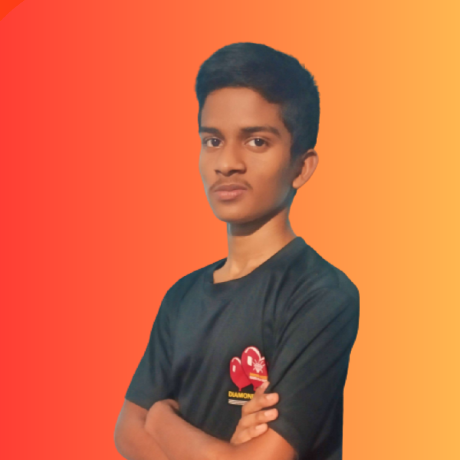