Building a Scalable Node.js Backend: Best Practices for Folder Structure and Code Optimization
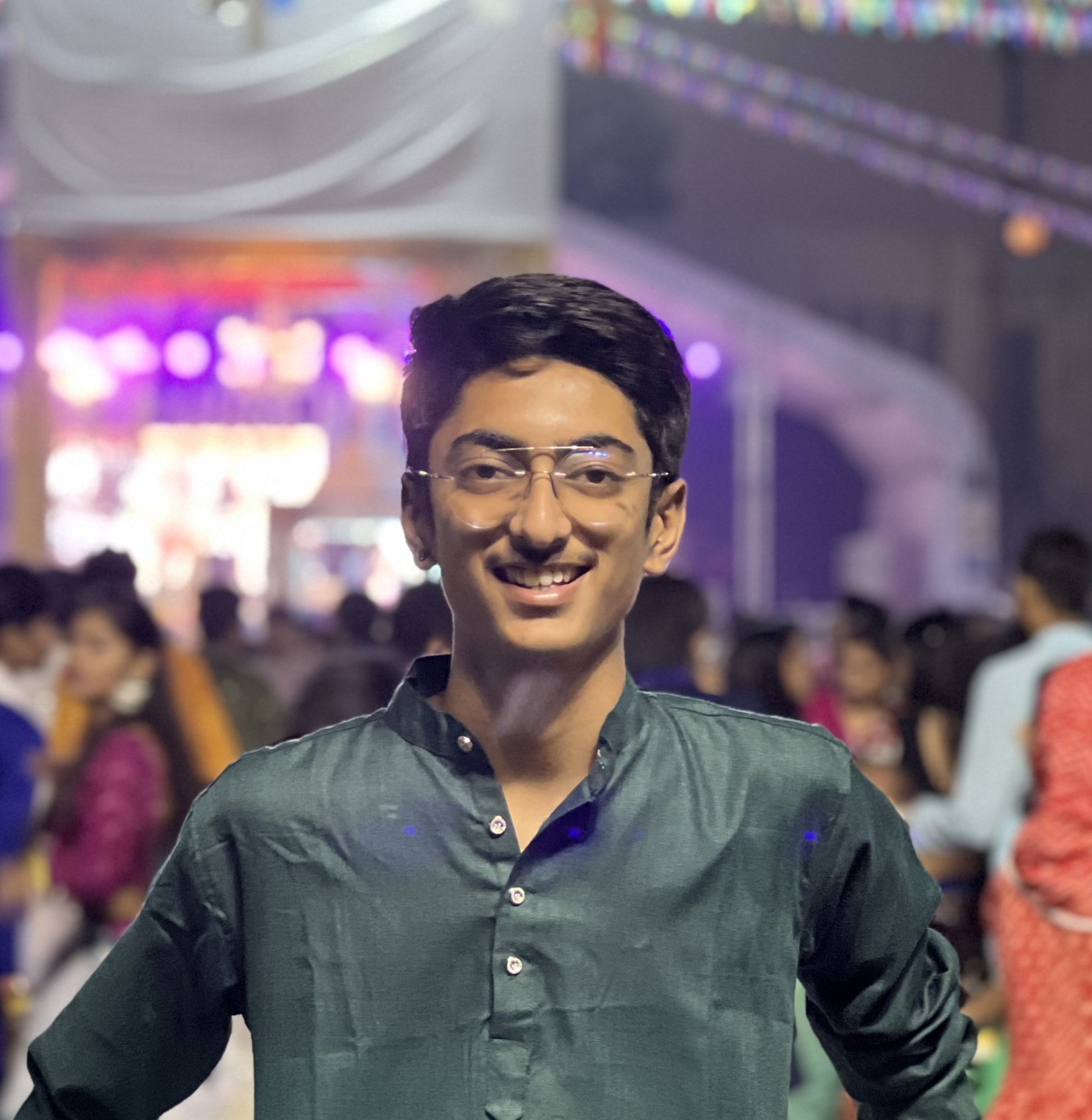
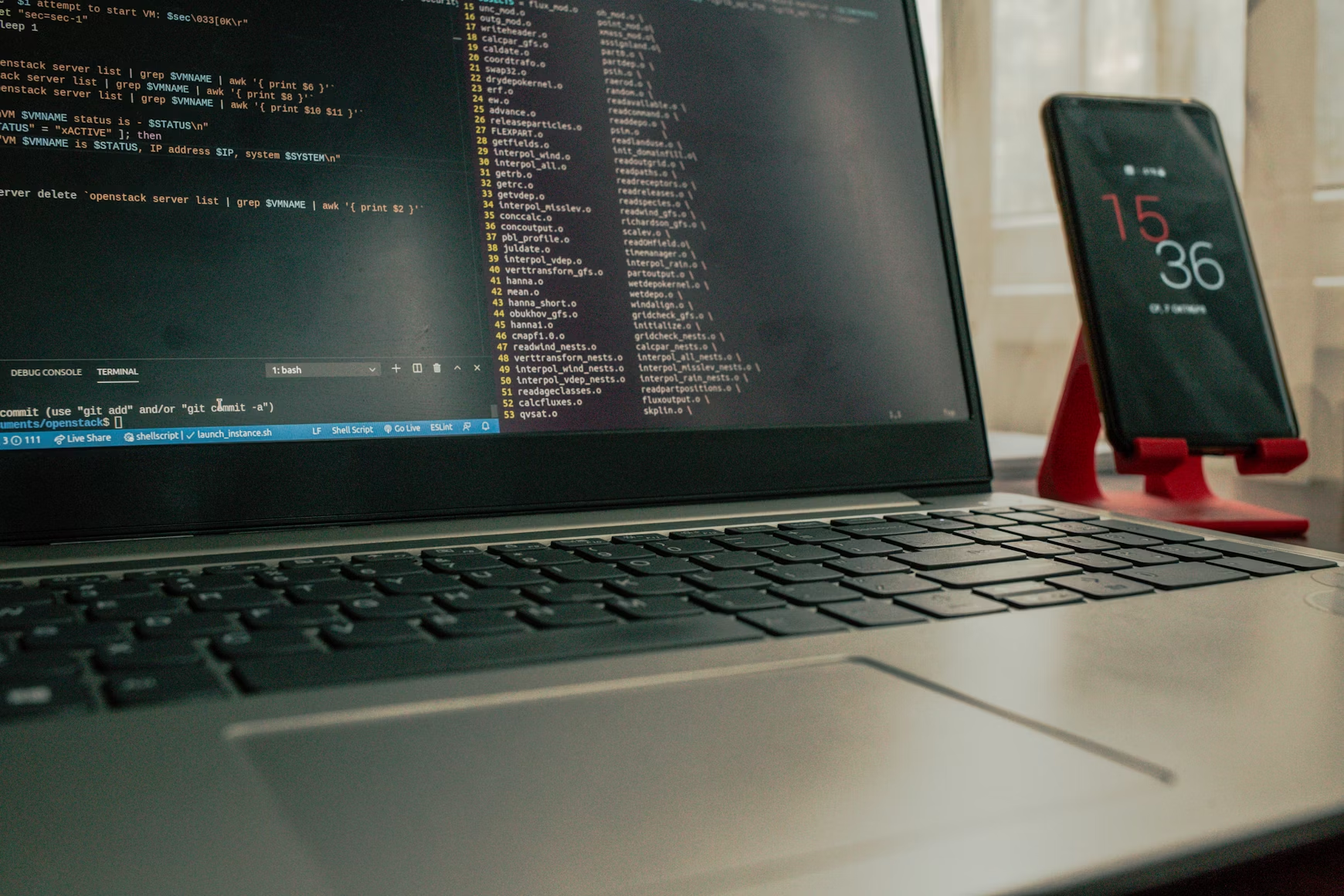
In the realm of modern web development, structuring your Node.js backend effectively is crucial for maintaining scalability, code organization, and developer productivity. This blog post delves into industry-standard practices for organizing your backend codebase using the MERN stack (MongoDB, Express.js, React.js, Node.js), focusing on folder structure, code cleaning, and optimization techniques.
1. Understanding the Folder Structure
A well-organized folder structure not only enhances code readability but also streamlines maintenance and scalability. Here’s a breakdown of the recommended folders and their purposes:
Models: Contains data models that define the schema and structure of your MongoDB collections.
Services: Encapsulates business logic and operations related to data manipulation, serving as an intermediary between controllers and models.
Controllers: Handles incoming requests, executes application logic, and returns appropriate responses by utilizing services and models.
Routes: Defines application endpoints and their corresponding HTTP methods, routing requests to the appropriate controllers.
Middlewares: Implements middleware functions that intercept and process incoming requests before they reach the route handler.
Config: Stores configuration settings such as database connections, environment variables, and other application-wide constants.
Utils: Houses utility functions that are reused across different parts of the application, promoting code reusability and reducing redundancy.
Additional Files:
.prettierrc, .prettierignore: Configuration files for Prettier, a code formatter, ensuring consistent code style across the project.
.env: Environment configuration file for storing sensitive or environment-specific variables.
server.js (or index.js): Entry point of the application where the server is initialized and middleware are applied.
2. Setting Up Your Node.js Backend
To begin, ensure Node.js and npm (Node Package Manager) are installed on your machine. Start by initializing your project and installing necessary dependencies:
mkdir backend
cd backend
npm init -y
npm install express mongoose body-parser dotenv
Express: Fast, unopinionated, minimalist web framework for Node.js.
Mongoose: Elegant MongoDB object modeling for Node.js.
Body-parser: Node.js body parsing middleware.
Dotenv: Loads environment variables from a .env file into process.env.
3. Implementing the Folder Structure
Create the recommended folders and files structure within your project:
/backend
/config
- db.js
- index.js
/controllers
- userController.js
- postController.js
/middlewares
- authMiddleware.js
/models
- User.js
- Post.js
/routes
- userRoutes.js
- postRoutes.js
/services
- userService.js
- postService.js
/utils
- logger.js
.env
.prettierrc
.prettierignore
server.js
4. Optimizing and Cleaning Your Code
To ensure your backend codebase remains maintainable and performs optimally:
Use Async/Await: Utilize asynchronous JavaScript to handle asynchronous operations more cleanly.
Error Handling Middleware: Implement middleware to centralize error handling and improve error messages sent to clients.
Logging: Integrate logging mechanisms to track application behavior and debug efficiently.
Code Reviews: Conduct regular code reviews to enforce coding standards, identify potential issues early, and share knowledge across the team.
Performance Monitoring: Use tools like New Relic, Datadog, or built-in Node.js performance monitoring to identify and resolve performance bottlenecks.
5. Conclusion
In conclusion, adopting a well-structured folder hierarchy and implementing code optimization techniques not only enhances the maintainability and scalability of your Node.js backend but also improves developer productivity and collaboration. By following these industry-standard practices, you can build robust and efficient applications that meet modern web development standards.
Implement these practices in your next MERN stack project to ensure your backend remains scalable, maintainable, and optimized for performance.
By adhering to these guidelines, you can effectively streamline your Node.js backend development process, promoting code cleanliness, scalability, and ease of maintenance. Whether you're starting a new project or refactoring an existing one, applying these practices will undoubtedly contribute to the overall success and longevity of your application.
Happy coding! :)
Subscribe to my newsletter
Read articles from Dev Mankad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
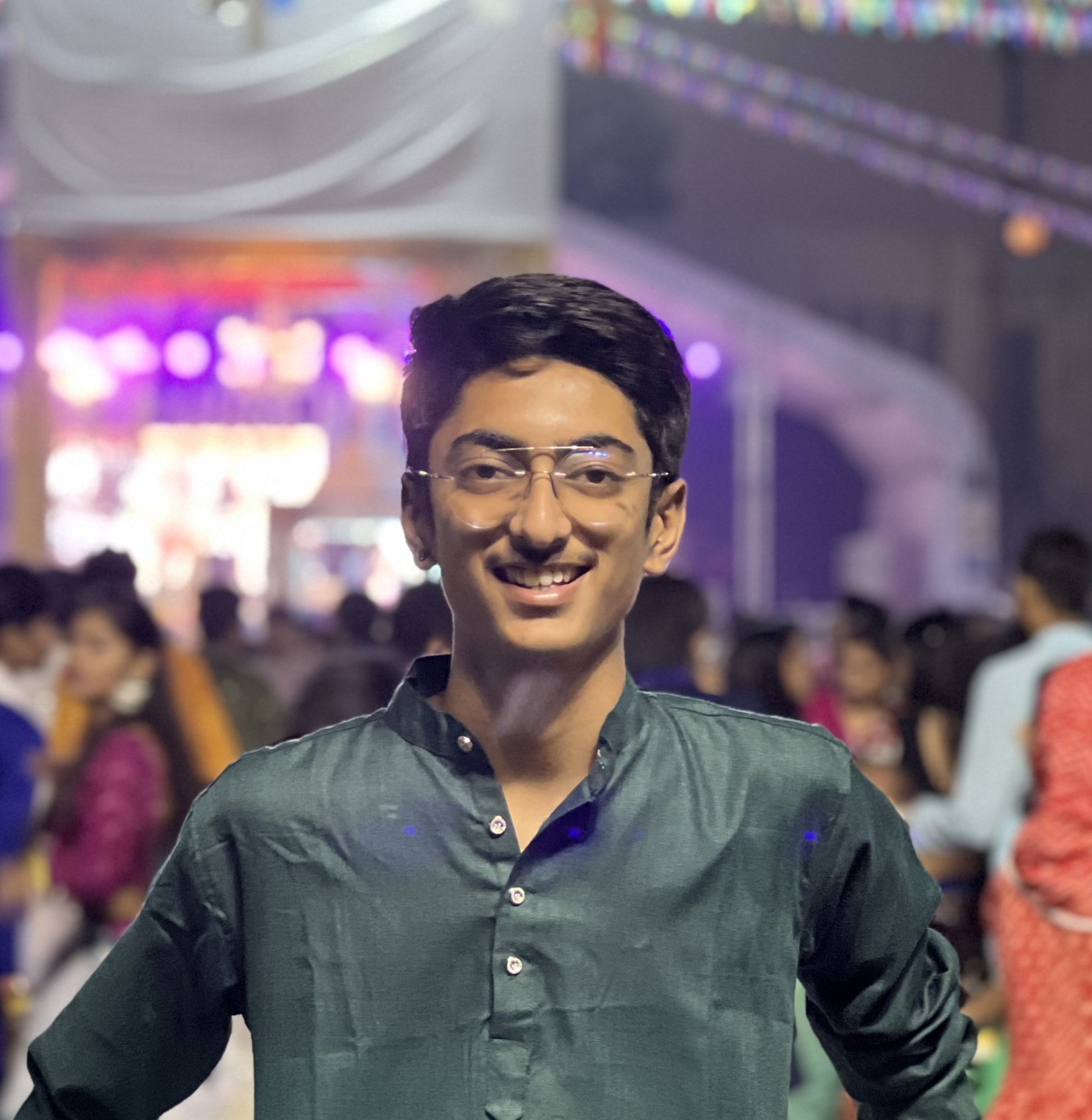
Dev Mankad
Dev Mankad
DevOps enthusiast and cloud aficionado | Sharing insights and expertise on all things DevOps and Cloud | Join me on a journey of continuous learning and innovation | Let's unlock the true potential of modern engineering together | Connect with me to explore the exciting intersection of DevOps and Cloud!