Shell Scripting for DevOps | Shell Scripting Zero 2 Hero
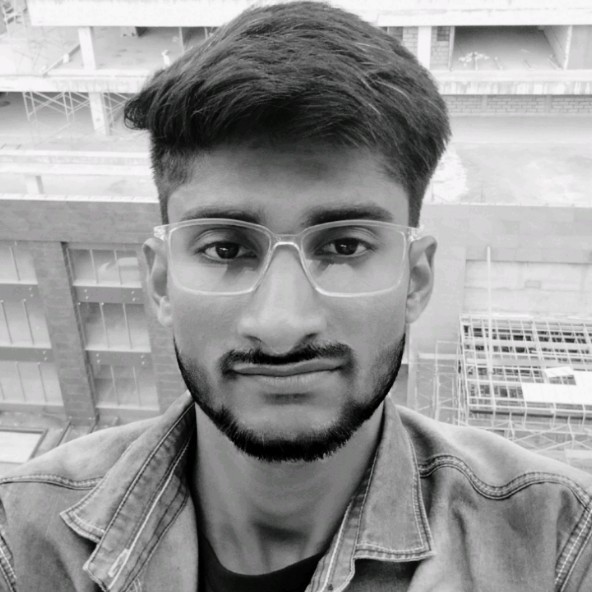
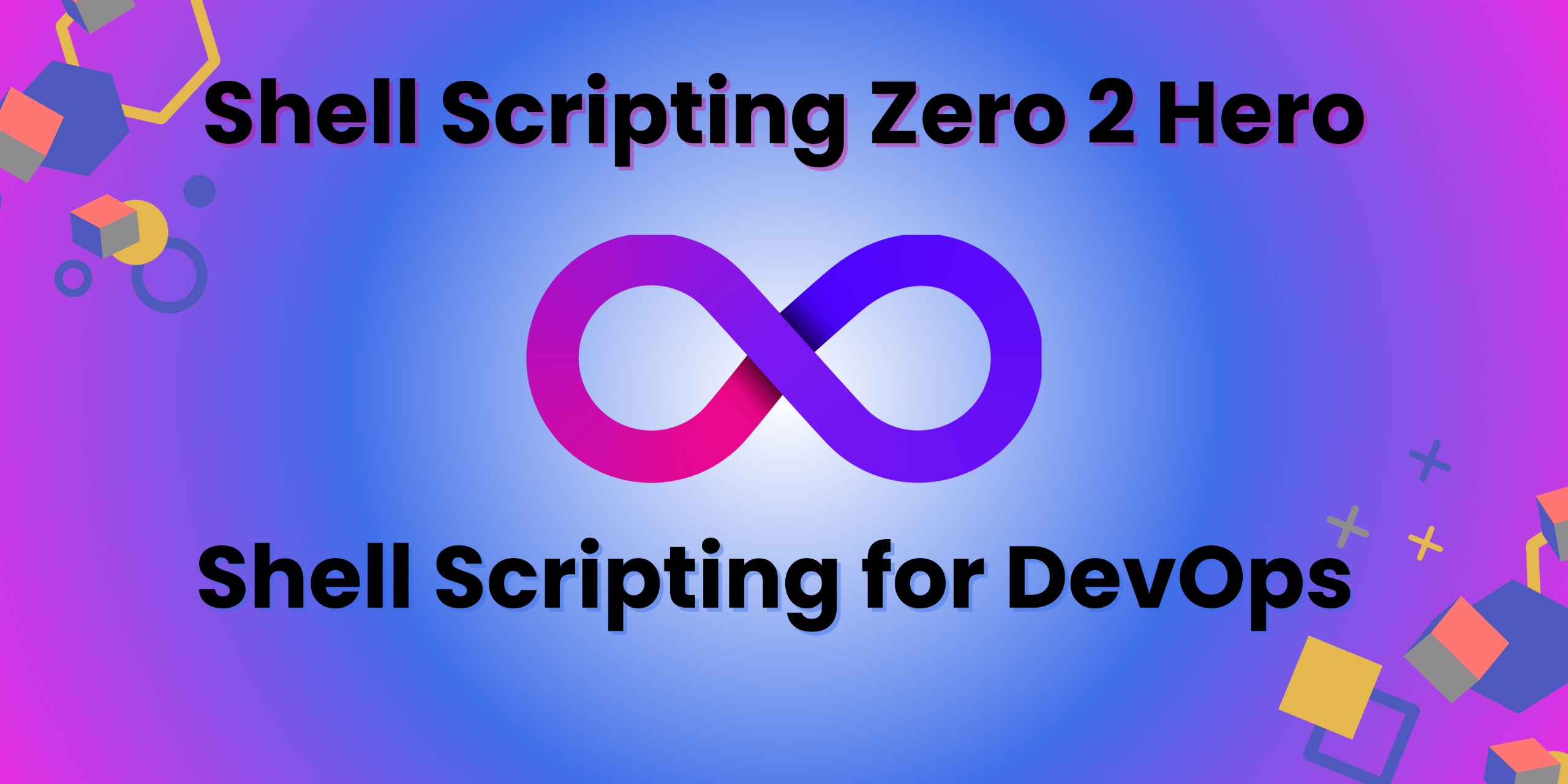
Shell Scripting Day - 1 :
Introduction
Shell scripting is used for automating day-to-day activities on a Linux computer.
It is useful for devops engineers to reduce manual work and increase efficiency.
Basics of Shell Scripting
Shell scripting is a process of automating regular activities on a Linux computer.
To write a shell script, you need to create a file with a
.sh
extension using thetouch
command or a text editor likeVim
.The first line of the shell script should start with
#!
followed by the path to the shell interpreter, such as#!/bin/bash
.Comments in shell scripting start with
#
.Basic Commands
ls
: lists the files and directories in the current directory.ls -ltr
: lists the files and directories in the current directory in long format, sorted by modification time (oldest first).touch
: creates a new empty file or updates the modification time of an existing file.man
: displays the manual page for a command.cat
: concatenates and displays the contents of a file.echo
: prints the given text or variable to the standard output.pwd
: prints the path of the current directory.cd
: changes the current directory.mkdir
: creates a new directory.rm
: removes a file or directory.chmod
: changes the permissions of a file or directory.
Creating a Simple Shell Script
- To create a simple shell script, you can use the following template:
We then wrote a simple shell script that creates a directory and two files inside that directory. The script is as follows:
#!/bin/bash
# Create a directory
mkdir Pratik
# Go inside the directory
cd Pratik
# Create two files
touch firstfile
touch secondfile
Overall, shell scripting is a powerful tool for devops engineers and other Linux users who want to automate their work and increase efficiency. By learning the basics and practicing with simple and advanced scripts, you can become proficient in shell scripting and improve your productivity.
Shell Scripting Day - 2 :
Notes for Advanced Shell Scripting Concepts by Pratik Gote
Introduction
Welcome back to the Article
Focusing on advanced shell scripting concepts
Today's Topic: Custom Node Script to Detect Node Health
Writing a shell script called
node_
health.sh
Using
DF -h
,free -g
, andnproc
commands to output different parameters on the virtual machine
1 : Metadata Information:
Always provide metadata information at the beginning of the script.
Example:
#!/bin/bash # # This script outputs the node health # # Author: Pratik # Date: 1st December # Purpose: Outputs the node health # Version: V1
2 : Debug Mode:
Use
set -x
to print the script in debug mode.Example:
#!/bin/bash set -x
3 : Find Processes:
Use
ps -ef
to print all the processes that are running on the virtual machine.Example:
ps -ef
4 : Filter Output:
Use
grep
to filter the output of any command.Example:
ps -ef | grep Amazon
5 : Get Process IDs:
Use
awk
to get the specific column from the output.Example:
ps -ef | grep Amazon | awk '{print $2}'
6 : Pipe Command:
Use
|
to get the output of the first command and send it to the second command.Example:
ps -ef | grep Amazon | awk '{print $2}'
7 : Exit Script on Error:
Use
set -e
to exit the script when there is an error.Example:
#!/bin/bash set -e
8 : Pipe Fail:
Use
set -o pipefail
to exit the script when there is a pipe failure.Example:
#!/bin/bash set -e set -o pipefail
9 : Log Files:
Use
curl
to retrieve the log files from the internet.Example:
curl https://raw.githubusercontent.com/username/repository/main/logfile.log
10 : Download Files:
Use
wget
to download the files from the internet.Example:
wget https://raw.githubusercontent.com/username/repository/main/logfile.log
11 : Find Command:
Use
find
to search for files in the file system.Example:
find / -name "logfile.log"
12 : If Loops, Else Loops, and For Loops:
Use
if
,else
, andfor
to write conditional statements and loops in the script.Example:
if [ condition ]; then command elif [ condition ]; then command else command fi for i in $(ls); do command done
overall Shell commands :
#!/bin/bash
# Metadata Information
Author: Abhishek
Date: 1st December
Prerequisites: None
Purpose: This script executes and outputs the node health
Version: V1
set -x
set -e
set -o pipefail
ps -ef | grep node | awk '{print $2}'
df -h
free -h
nproc
top
if [ $(ps -ef | grep node | awk '{print $2}') -gt 0 ]; then
echo "Node is running"
else
echo "Node is not running"
fi
curl https://example.com
wget https://example.com/file.txt
find / -name file.txt
# Checking if a Process is Running with if-else
if [ $(ps -ef | grep node | awk '{print $2}') -gt 0 ]; then
echo "Node is running"
else
echo "Node is not running"
fi
# Monitoring Disk Space Usage with if-else
if [ $(df -h | awk '{print $5}' | sed '/%//g') -gt 80 ]; then
echo "Disk space is low"
else
echo "Disk space is okay"
fi
for i in {1..5}; do
echo "Iteration $i"
done
Shell Scripting & Linux Interview Questions for DevOps Engineers
Here are the common interview questions, along with some additional questions that are commonly asked in shell scripting interviews:
Shell Scripting Interview Questions
Basic Questions
What is shell scripting?
- Answer: Shell scripting is a way to automate tasks on a Linux or Unix system by writing a series of commands in a file that can be executed by the shell.
What are the advantages of shell scripting?
- Answer: Shell scripting allows for automation, increased productivity, and ease of maintenance.
What are the different types of shell scripts?
- Answer: There are two types of shell scripts: Bourne shell scripts (sh) and Bash shell scripts (bash).
What is bash?
- Answer: Bash is a type of programming language that you use in the terminal (the command line) on most Linux systems and MacOS. It allows you to write scripts, which are just files with a series of commands. These scripts can do complicated tasks, like making backups of your files, by running several commands automatically. This makes it easier to manage and automate tasks on your computer.
Scripting Questions
Write a script to print "Hello World"?
- Answer:
echo "Hello World"
- Answer:
How do you declare a variable in shell scripting?
- Answer:
variable_name=value
- Answer:
What is the difference between
=
and==
in shell scripting?- Answer:
=
is used for assignment, while==
is used for comparison.
- Answer:
How do you concatenate strings in shell scripting?
- Answer: Using the
+
operator or by using the${parameter}
syntax.
- Answer: Using the
What is the purpose of the
if
statement in shell scripting?- Answer: To execute a block of code if a condition is true.
How do you use the
for
loop in shell scripting?- Answer:
for variable in list; do command; done
- Answer:
What is the purpose of the
while
loop in shell scripting?- Answer: To execute a block of code as long as a condition is true.
How do you use the
case
statement in shell scripting?- Answer:
case variable in pattern1) command1;; pattern2) command2;; esac
- Answer:
File and Directory Management Questions
How do you create a new directory in shell scripting?
- Answer:
mkdir directory_name
- Answer:
How do you delete a file in shell scripting?
- Answer:
rm file_name
- Answer:
How do you copy a file in shell scripting?
- Answer:
cp source_file destination_file
- Answer:
How do you move a file in shell scripting?
- Answer:
mv source_file destination_file
- Answer:
How do you check if a file exists in shell scripting?
- Answer:
if [ -f file_name ]; then echo "File exists"; fi
- Answer:
Error Handling and Debugging Questions
How do you handle errors in shell scripting?
- Answer: Using the
trap
command or by checking the exit status of commands.
- Answer: Using the
How do you debug a shell script?
- Answer: Using the
-x
option or by addingecho
statements to the script.
- Answer: Using the
Advanced Questions
How do you use regular expressions in shell scripting?
- Answer: Using the
grep
command or by using the=~
operator.
- Answer: Using the
How do you use arrays in shell scripting?
- Answer: Declaring an array using
array_name=()
and accessing elements using${array_name[index]}
.
- Answer: Declaring an array using
How do you use functions in shell scripting?
- Answer: Declaring a function using
function_name() { command; }
and calling it usingfunction_name
.
- Answer: Declaring a function using
Common Interview Questions
Can you explain the difference between
sh
andbash
?- Answer:
sh
is the Bourne shell, whilebash
is the Bourne-Again SHell.
- Answer:
How do you schedule a script to run at a specific time?
- Answer: Using the
cron
command.
- Answer: Using the
How do you make a script executable?
- Answer: Using the
chmod
command to set the execute permission.
- Answer: Using the
Can you explain the concept of shell expansion?
- Answer: Shell expansion is the process of replacing special characters and expressions with their values.
How do you use shell scripting to automate system administration tasks?
- Answer: By writing scripts to perform tasks such as backups, user management, and system monitoring.
conclusion :
shell scripting is crucial for DevOps engineers and Linux users because it helps automate tasks and boost productivity. By learning both basic and advanced scripting, you can manage systems efficiently, handle files, and perform complex tasks easily. Knowing common interview questions can also aid in job preparation. With regular practice, anyone can become skilled in shell scripting and improve their workflow.
๐ Thanks for Reading! Let's Connect for More DevOps Wisdom!
Follow Me : Pratik Gote
๐ LinkedIn:Pratik-Gote
Stay tuned for my latest blog posts, professional updates, and exciting insights. Together, we can achieve greatness! ๐๐ก
Subscribe to my newsletter
Read articles from Pratik Gote directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
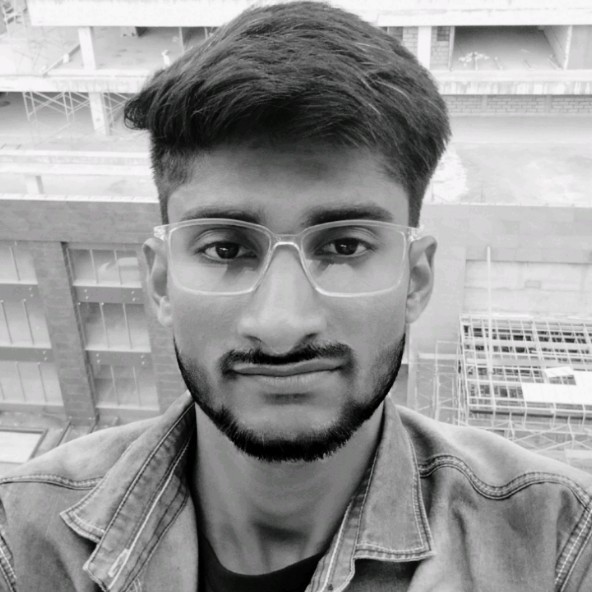
Pratik Gote
Pratik Gote
About Me ๐ Greetings! I'm Pratik Gote, a dedicated software developer with a strong passion for staying at the forefront of technological advancements in the industry. Armed with a solid foundation in Computer Science, I excel in creating innovative solutions that push boundaries and deliver tangible results. What I Do I specialize in: Full-Stack Development: Crafting scalable applications using cutting-edge frameworks such as React, Vue.js, Node.js, and Django. ๐ป Cloud Computing: Harnessing the capabilities of AWS, Azure, and Google Cloud to architect and deploy robust cloud-based solutions. โ๏ธ DevOps: Implementing CI/CD pipelines, Docker containerization, and Kubernetes orchestration to streamline development workflows. ๐ง AI and Machine Learning: Exploring the realms of artificial intelligence to develop intelligent applications that redefine user experiences. ๐ค My Passion Technology is dynamic, and my commitment to continuous learning drives me to share insights and demystify complex concepts through my blog. I strive to: Demystify Emerging Technologies: Simplify intricate ideas into accessible content. ๐ Share Practical Insights: Offer real-world examples and tutorials on state-of-the-art tools and methodologies. ๐ ๏ธ Engage with Fellow Enthusiasts: Foster a collaborative environment where innovation thrives. ๐ค Get in Touch I enjoy connecting with like-minded professionals and enthusiasts. Let's collaborate on shaping the future of technology together! Feel free to connect with me on LinkedIn : https://www.linkedin.com/in/pratik-gote-516b361b3/ or drop me an email at pratikgote69@gmail.com. Let's explore new horizons in technology! ๐