Daily Hack #day78 - Formik React Forms
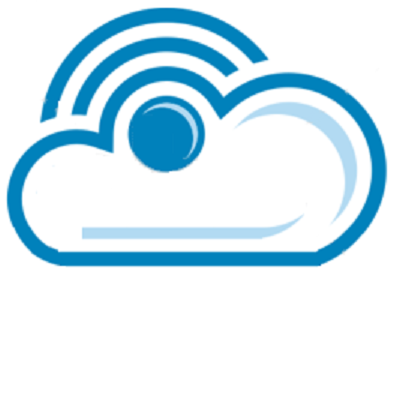
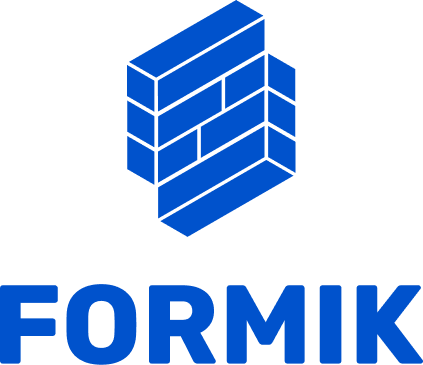
Formik React Forms
Formik is a popular open-source library for building and managing forms in React applications. It simplifies form handling by providing a set of tools and components that manage form state, validation, and submission, making it easier to create complex forms with minimal boilerplate code.
Key Features:
Form State Management: Formik manages the state of form fields, ensuring they are kept in sync with the UI. It handles form values, errors, and touched status, allowing for a smooth user experience.
Validation: Supports both synchronous and asynchronous validation. You can define custom validation logic using JavaScript or integrate with validation libraries like Yup.
Submission Handling: Simplifies form submission by managing the submission process, including handling success and error states.
Field Components: Provides reusable components such as
<Field>
,<Form>
, and<ErrorMessage>
, which streamline the creation of form fields and error messages.Integration: Easily integrates with other React libraries and tools, such as state management solutions and UI component libraries.
Use Cases:
Simple Forms: Quickly create and manage forms with basic validation and state handling.
Complex Forms: Build complex forms with dynamic fields, nested data structures, and custom validation logic.
Reusable Form Components: Develop reusable form components that can be easily integrated into different parts of your application.
Example Usage:
Here is a simple example of a form created with Formik:
import React from 'react';
import { Formik, Form, Field, ErrorMessage } from 'formik';
import * as Yup from 'yup';
const SignupSchema = Yup.object().shape({
username: Yup.string()
.min(2, 'Too Short!')
.max(50, 'Too Long!')
.required('Required'),
email: Yup.string().email('Invalid email').required('Required'),
password: Yup.string()
.min(6, 'Password is too short')
.required('Required'),
});
const SignupForm = () => (
<Formik
initialValues={{ username: '', email: '', password: '' }}
validationSchema={SignupSchema}
onSubmit={(values) => {
console.log(values);
}}
>
{({ isSubmitting }) => (
<Form>
<div>
<label htmlFor="username">Username</label>
<Field type="text" name="username" />
<ErrorMessage name="username" component="div" />
</div>
<div>
<label htmlFor="email">Email</label>
<Field type="email" name="email" />
<ErrorMessage name="email" component="div" />
</div>
<div>
<label htmlFor="password">Password</label>
<Field type="password" name="password" />
<ErrorMessage name="password" component="div" />
</div>
<button type="submit" disabled={isSubmitting}>
Submit
</button>
</Form>
)}
</Formik>
);
export default SignupForm;
Benefits:
Ease of Use: Reduces boilerplate code and simplifies the creation of forms in React applications.
Flexibility: Offers a flexible API that can be customized to fit the needs of any form, from simple to highly complex.
Community and Support: Backed by a large community, with extensive documentation and examples available to help developers get started quickly.
Formik is a powerful tool for managing forms in React applications, providing a robust set of features that make it easier to handle form state, validation, and submission. Whether building simple or complex forms, Formik offers the flexibility and ease of use needed to create effective and efficient form experiences.
Subscribe to my newsletter
Read articles from Cloud Tuned directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
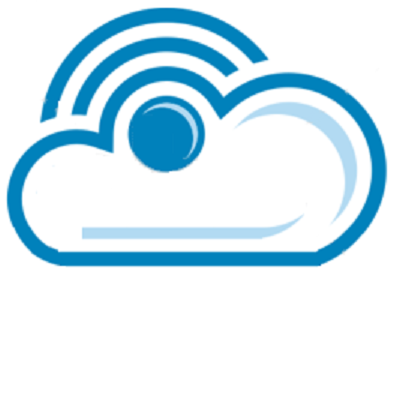