Starting with JavaScript: Must-Know Tips for Newbies
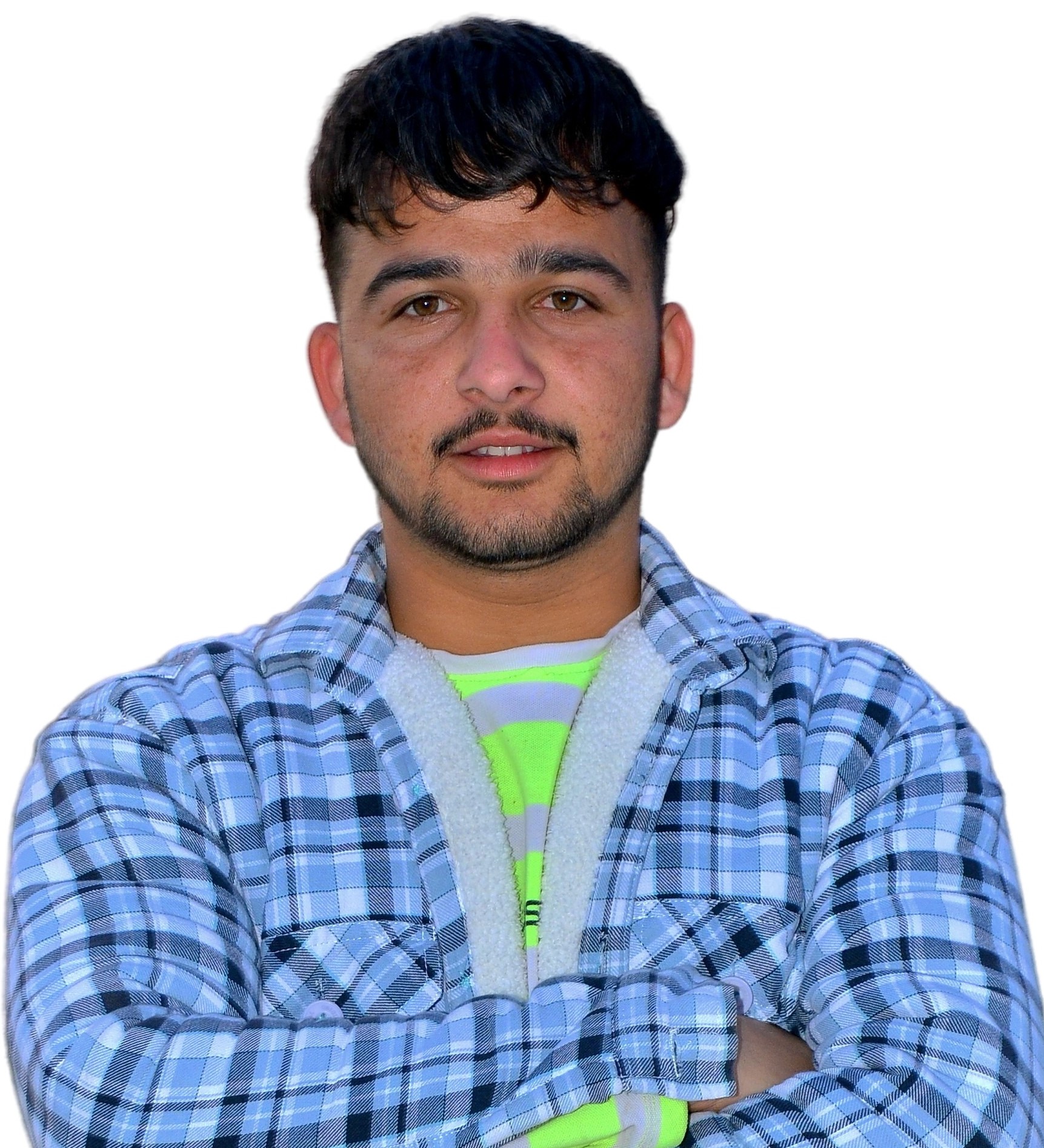

Why is JavaScript So Popular?
JavaScript's popularity can be attributed to several factors, including:
Versatility: JavaScript can be used for various tasks, from front-end web development to back-end development and even mobile app development.
Relatively Easy to Learn: JavaScript is often considered to be a relatively easy language to learn, especially for those who already have some programming experience.
Large Community: JavaScript has a large-scale and active community of developers. This means a lot of resources available online to help you learn the language and solve problems.
Why Do People Hate JavaScript?
Despite its popularity, JavaScript also has its fair share of critics. Some of the most common complaints include:
Confusing Syntax: JavaScript has some rules that can seem confusing or inconsistent, making it hard to learn and write clean code.
Weak Typing: Variables in JavaScript don't have fixed types, which can cause unexpected errors if you're not careful.
Callback Hell: Using callbacks for asynchronous programming can make the code messy and hard to maintain.
If you're new to JavaScript, it's important to remember that there is a learning curve. But with the right foundation and resources, you can overcome the challenges of the language and start to create amazing things.
Understanding JavaScript Execution: How Code Works
JavaScript is a popular and powerful language but to fully harness its potential to understand how it executes code.
Synchronous Single-Threaded Language
- First and foremost, JavaScript is a synchronous, single-threaded language. This means it can only execute one command at a time, in the order the commands are written. Imagine JavaScript as a single-lane road where only one car can drive at a time.
The Execution Context
At the heart of the JavaScript execution is the execution context. Think of it as a big box where the JavaScript engine runs your code. Everything in JavaScript happens inside this context.
An execution context consists of two components :
Memory Allocation (Variable Allocation).
Code execution (Thread of Execution).
Let's understand with an example
var alias = 10;
console.log(alias);
function execute() {
var name = "Gevin Belson");
console.log("name");
return;
}
Here, when code is executed, JavaScript creates a global execution context for the main program. Within this context, the variable alias
is declared and assigned the value 10
. Then, console.log(alias)
is executed.
When execute()
is called, a new execution context is created for the execute
function. Inside this context, this variable name
is declared and assigned a value then console.log(name)
is executed.
Understanding these concepts can help easily learn JavaScript and write efficient and error-free code.
If you are new to JavaScript, understanding its basic syntax, variables, data types, and operators is essential to kick-start your journey. In this beginner's friendly guide, we'll delve into these fundamental concepts to provide you with a solid foundation.
Syntax:
JavaScript syntax refers to the rules that govern how code is written and structured. Let's start with a simple example:
// This is single line comment in JavaScript
/*
This is a
multi-line comment
*/
console.log("Hello World");
Comments: Comments are used to add notes within your code for explanation or clarification. They are ignored by JavaScript interpreters.
Statements: JavaScript code is composed of statements, which are instructions that perform actions. Each statement typically ends with a semicolon(;).
Variables:
Variables in JavaScript are used to store and manipulate data. They are declared using var
let
const
keywords. Here's how you declare variables:
var age = 25;
let name = 'john';
const PI = 3.14;
var: Declares a variable with function or global scope.
let: Declare a block-scoped variable.
const: Declares a blocked-scoped constant variable whose value cannot be reassigned.
Data types:
JavaScript supports various data types, including strings, numbers, boolean, arrays, objects, and more. Let's explore some of the basic data types:
- Strings: Used to represent textual data enclosed in single (
'
) or double ("
) quotes.
let message = "Hello World";
- Numbers: Used to represent numeric data, including integers and floating-point numbers.
let age = 21;
let pi = 3.14;
- Boolean: Represents true or false values.
let isStudent = true;
Basic Operators:
JavaScript supports various operators for performing operations on data. Here are some basic operators:
Arithmetic Operators: Used for arithmetic operations such as addition, subtraction, multiplication, division, and modulus.
let sum = 10 + 5; let difference = 10-5; let product = 10 * 5; let quotient = 10 / 5; let remainder = 10 % 3;
Assignment Operator: Used to assign values to variables.
let x = 10;
Comparison Operators: Used to compare values and return true and false.
let isEqual = 10 ===5;
Logical Operators: Used to perform logical operations.
let result = (10 > 5) && (5 < 3);
Understanding these fundamental concepts is crucial as you embark on your journey to learn JavaScript. Practice writing code examples, experiment with different ideas and gradually build your understanding. In the next part of the blog, we'll delve deeper into control flow and functions in JavaScript.
Subscribe to my newsletter
Read articles from Sunil Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
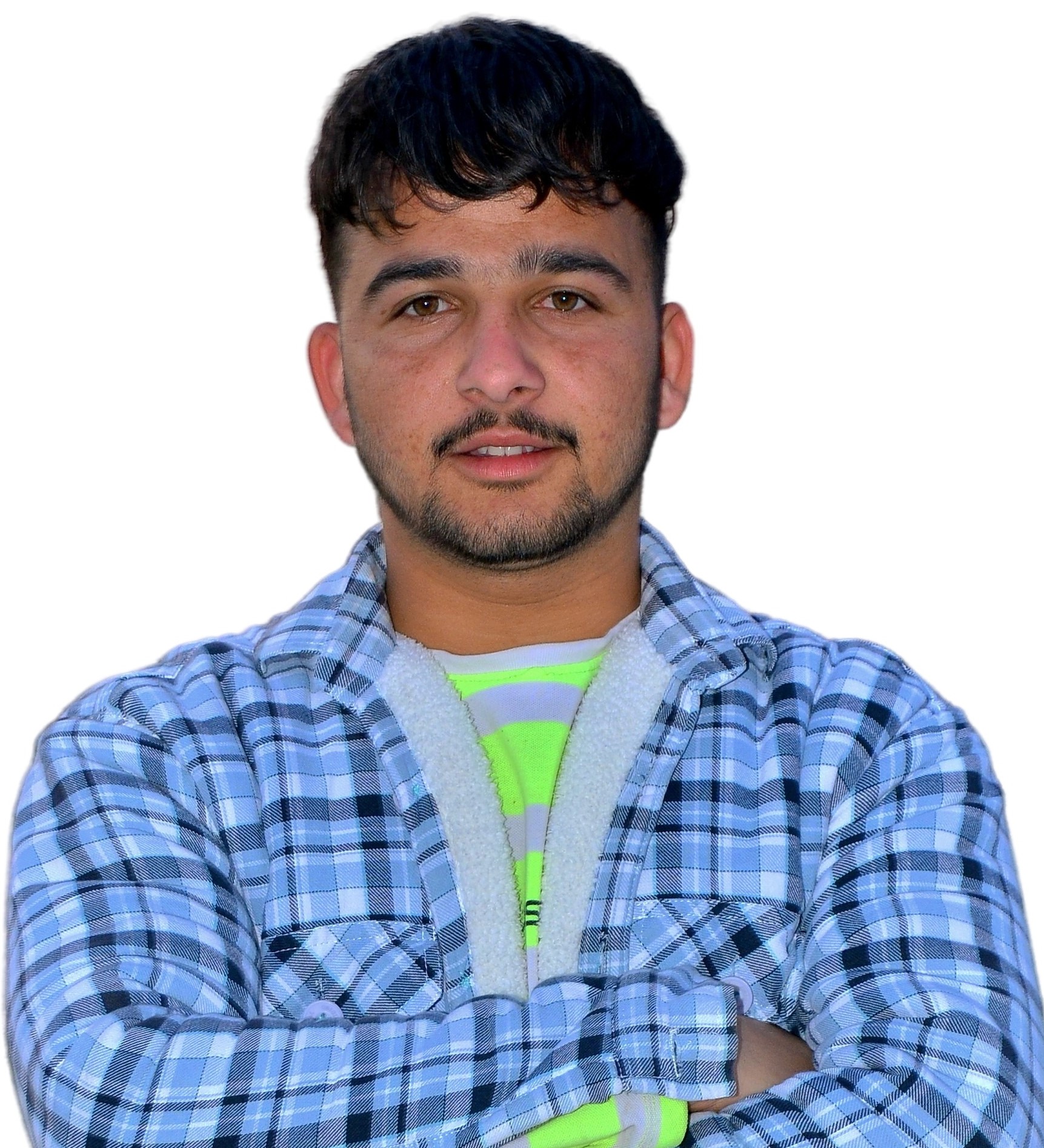
Sunil Kumar
Sunil Kumar
Teaching myself how to code