Get Current Time in Python
Table of contents
- 1. Introduction to Date and Time in Python
- 2. Using the time Module
- 3. Using the datetime Module
- 4. Formatting Time with strftime
- 5. Getting the Current Time in Different Time Zones
- 6. Using the pytz Module for Time Zones
- 7. Using dateutil for Advanced Time Handling
- 8. Comparing and Manipulating Times
- 9. Practical Examples
- 10. Conclusion
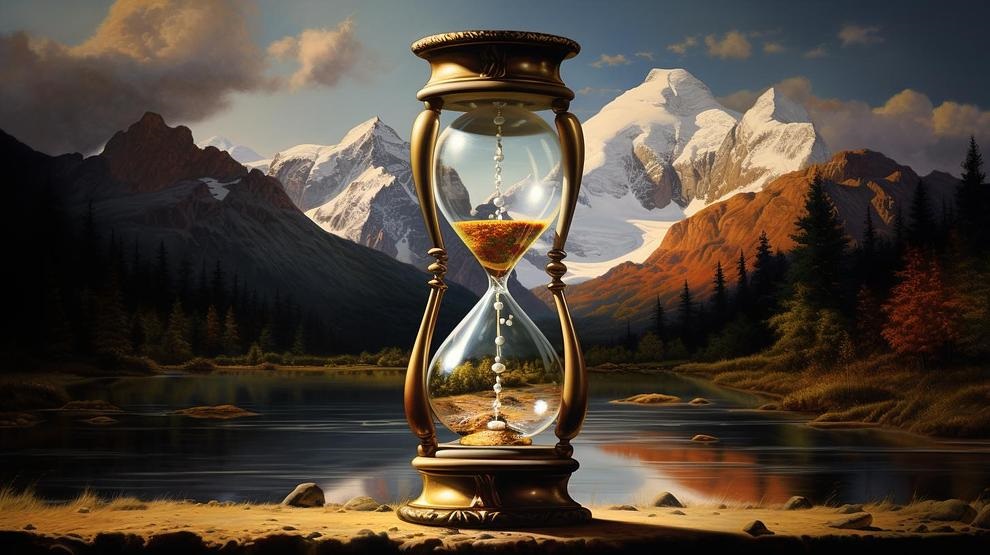
Python provides a wide range of libraries and modules to handle date and time. Whether you're building a simple application or a complex system, understanding how to work with the current time is essential.
In this tutorial, we'll explore various methods to get the current time in Python, along with practical examples and best practices.
1. Introduction to Date and Time in Python
Python has several modules that deal with date and time, such as time
, datetime
, and third-party modules like pytz
and dateutil
. These modules provide various functions and classes to handle dates, times, and time zones efficiently. Understanding these modules is crucial for many applications, including logging, event scheduling, and data analysis.
Date and time are fundamental to many programming tasks. Whether you need to log events, schedule tasks, or handle timestamps in data processing, Python's date and time modules can help you accomplish these tasks effectively.
This tutorial aims to cover the essentials of working with the current time in Python, providing a solid foundation for further exploration and application.
If Python isn't installed on your system, you can download it from the official Python website. Alternatively, you can run your Python code online and follow along with this tutorial.
2. Using the time
Module
The time
module provides functions for working with time-related tasks. The time.time()
function returns the current time in seconds since the Epoch (January 1, 1970, 00:00:00 UTC). This is often used in performance testing or when a simple timestamp is required.
Example:
import time
current_time = time.time()
print("Current time in seconds since the Epoch:", current_time)
This output is useful for understanding the exact point in time in a format that is easy to compare, especially in performance metrics.
However, for most practical applications, you will need more detailed information. The time.localtime()
function converts the time expressed in seconds since the Epoch to a struct_time
in local time.
Example:
import time
local_time = time.localtime()
print("Local time:", local_time)
print("Year:", local_time.tm_year)
print("Month:", local_time.tm_mon)
print("Day:", local_time.tm_mday)
print("Hour:", local_time.tm_hour)
print("Minute:", local_time.tm_min)
print("Second:", local_time.tm_sec)
The struct_time
object provides a more human-readable way to access individual components of the time, which can be useful for creating timestamps, logging, or user interface elements that display the current time.
3. Using the datetime
Module
The datetime
module is more versatile and provides classes for manipulating dates and times in both simple and complex ways. To get the current local date and time, you can use datetime.now()
.
Example:
from datetime import datetime
current_time = datetime.now()
print("Current date and time:", current_time)
The datetime
object provides a comprehensive way to handle both date and time information. It includes attributes for the year, month, day, hour, minute, second, and microsecond, which can be accessed individually.
To get the current UTC date and time, use datetime.utcnow()
. This can be particularly useful when working with systems that require time coordination across different geographic regions.
Example:
from datetime import datetime
current_time_utc = datetime.utcnow()
print("Current UTC date and time:", current_time_utc)
The datetime
module also allows for arithmetic operations on date and time objects, enabling easy manipulation and comparison of time values.
4. Formatting Time with strftime
The strftime
method in the datetime
module allows you to format dates and times into readable strings. This is particularly useful for generating logs, reports, or user interface displays that require a specific date and time format.
Example:
from datetime import datetime
current_time = datetime.now()
formatted_time = current_time.strftime("%Y-%m-%d %H:%M:%S")
print("Formatted date and time:", formatted_time)
Common format codes include:
%Y
: Year with century (e.g., 2023)%m
: Month as a zero-padded decimal number (e.g., 01, 12)%d
: Day of the month as a zero-padded decimal number (e.g., 01, 31)%H
: Hour (24-hour clock) as a zero-padded decimal number (e.g., 00, 23)%M
: Minute as a zero-padded decimal number (e.g., 00, 59)%S
: Second as a zero-padded decimal number (e.g., 00, 59)
By using strftime
, you can easily customize the format of the output to match the requirements of your application or system.
5. Getting the Current Time in Different Time Zones
Handling different time zones can be challenging, but Python's datetime
module can help simplify this process. Working with time zones is essential for applications that operate across multiple regions, ensuring that time-sensitive data is accurate and consistent.
To convert the current time to a different time zone, you can use the timezone
class in the datetime
module. This approach is useful for applications that need to display local times for users in different regions or synchronize events across time zones.
Example:
from datetime import datetime, timezone, timedelta
# Get current UTC time
current_time_utc = datetime.now(timezone.utc)
print("Current UTC date and time:", current_time_utc)
# Convert UTC time to a different time zone (e.g., New York)
ny_time_zone = timezone(timedelta(hours=-4)) # EDT is UTC-4
current_time_ny = current_time_utc.astimezone(ny_time_zone)
print("Current New York date and time:", current_time_ny)
This example shows how to convert UTC time to Eastern Daylight Time (EDT). By using the timezone
class and timedelta
, you can easily handle different time zones without relying on external libraries.
6. Using the pytz
Module for Time Zones
The pytz
module allows for more advanced timezone handling. It includes a comprehensive list of time zones and provides more accurate conversions, especially during Daylight Saving Time changes. pytz
is particularly useful for applications that require precise time zone conversions and handling of various local time rules.
To install pytz
, use:
pip install pytz
Example:
from datetime import datetime
import pytz
# Get current UTC time
current_time_utc = datetime.now(pytz.utc)
print("Current UTC date and time:", current_time_utc)
# Convert UTC time to a different time zone (e.g., New York)
ny_time_zone = pytz.timezone('America/New_York')
current_time_ny = current_time_utc.astimezone(ny_time_zone)
print("Current New York date and time:", current_time_ny)
By using pytz
, you can ensure that your application correctly handles time zones and daylight saving changes. This is critical for applications that operate globally and need to maintain accurate time records.
7. Using dateutil
for Advanced Time Handling
The dateutil
module provides powerful extensions to the datetime
module. It can handle parsing of date strings, complex date manipulations, and provides utilities for working with time zones. This module is particularly useful for applications that require advanced date and time operations, such as scheduling, parsing user input, and handling complex time calculations.
To install dateutil
, use:
pip install python-dateutil
Example:
from datetime import datetime
from dateutil import parser, tz
# Parse a date string into a datetime object
date_str = "2023-06-15T12:30:00Z"
parsed_date = parser.parse(date_str)
print("Parsed date:", parsed_date)
# Convert to a different time zone
ny_time_zone = tz.gettz('America/New_York')
parsed_date_ny = parsed_date.astimezone(ny_time_zone)
print("Parsed date in New York time zone:", parsed_date_ny)
The dateutil
module simplifies many common date and time tasks, making it a valuable addition to your toolkit when working with complex date and time requirements.
8. Comparing and Manipulating Times
Python allows you to compare and manipulate date and time objects easily. You can perform operations like addition and subtraction with timedelta
, which is useful for scheduling tasks, calculating durations, and handling time-based conditions.
Example:
from datetime import datetime, timedelta
current_time = datetime.now()
print("Current date and time:", current_time)
# Add 5 days to the current time
future_time = current_time + timedelta(days=5)
print("Date and time 5 days from now:", future_time)
# Subtract 2 hours from the current time
past_time = current_time - timedelta(hours=2)
print("Date and time 2 hours ago:", past_time)
These operations allow you to handle a variety of time-based scenarios, such as setting expiration dates, scheduling future events, and calculating the duration between two points in time.
9. Practical Examples
Scheduling a Task
You might need to schedule a task to run at a specific time. Here's an example of how you can calculate the time difference and sleep until the task should run. This approach is useful for applications that require timed execution of functions, such as reminders, alerts, and automated tasks.
Example:
import time
from datetime import datetime, timedelta
def schedule_task(task_time):
current_time = datetime.now()
wait_time = (task_time - current_time).total_seconds()
if wait_time > 0:
time.sleep(wait_time)
print("Task is running now!")
else:
print("Scheduled time is in the past!")
# Schedule a task to run 10 seconds from now
task_time = datetime.now() + timedelta(seconds=10)
schedule_task(task_time)
This example demonstrates how to use the time.sleep
function to delay execution until the specified time. It's a simple but effective way to handle scheduled tasks.
Logging Timestamps
When logging events, it's common to include a timestamp. This helps in tracking the sequence of events and debugging issues.
Example:
from datetime import datetime
def log_event(event):
timestamp = datetime.now().strftime("%Y-%m-%d %H:%M:%S")
print(f"[{timestamp}] {event}")
log_event("Application started")
time.sleep(2)
log_event("Performing task")
time.sleep(2)
log_event("Application finished")
By including timestamps in your logs, you can easily monitor the flow of your application and diagnose issues based on the time and order of events.
10. Conclusion
Getting the current time in Python is a fundamental skill that can be used in a variety of applications, from logging events to scheduling tasks and handling time zones. By mastering the time
, datetime
, pytz
, and dateutil
modules, you can efficiently work with date and time in your Python programs.
Whether you are building a simple script or a complex system, understanding how to get and manipulate the current time will enhance your ability to handle real-world scenarios. Experiment with the examples provided and explore further to see how these tools can be applied to your projects.
Subscribe to my newsletter
Read articles from Hichem MG directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Hichem MG
Hichem MG
Python dev, coding enthusiast, problem solver. Passionate about clean code and tech innovation.