Top 7 Tools You Need to Know to Use AI with Go
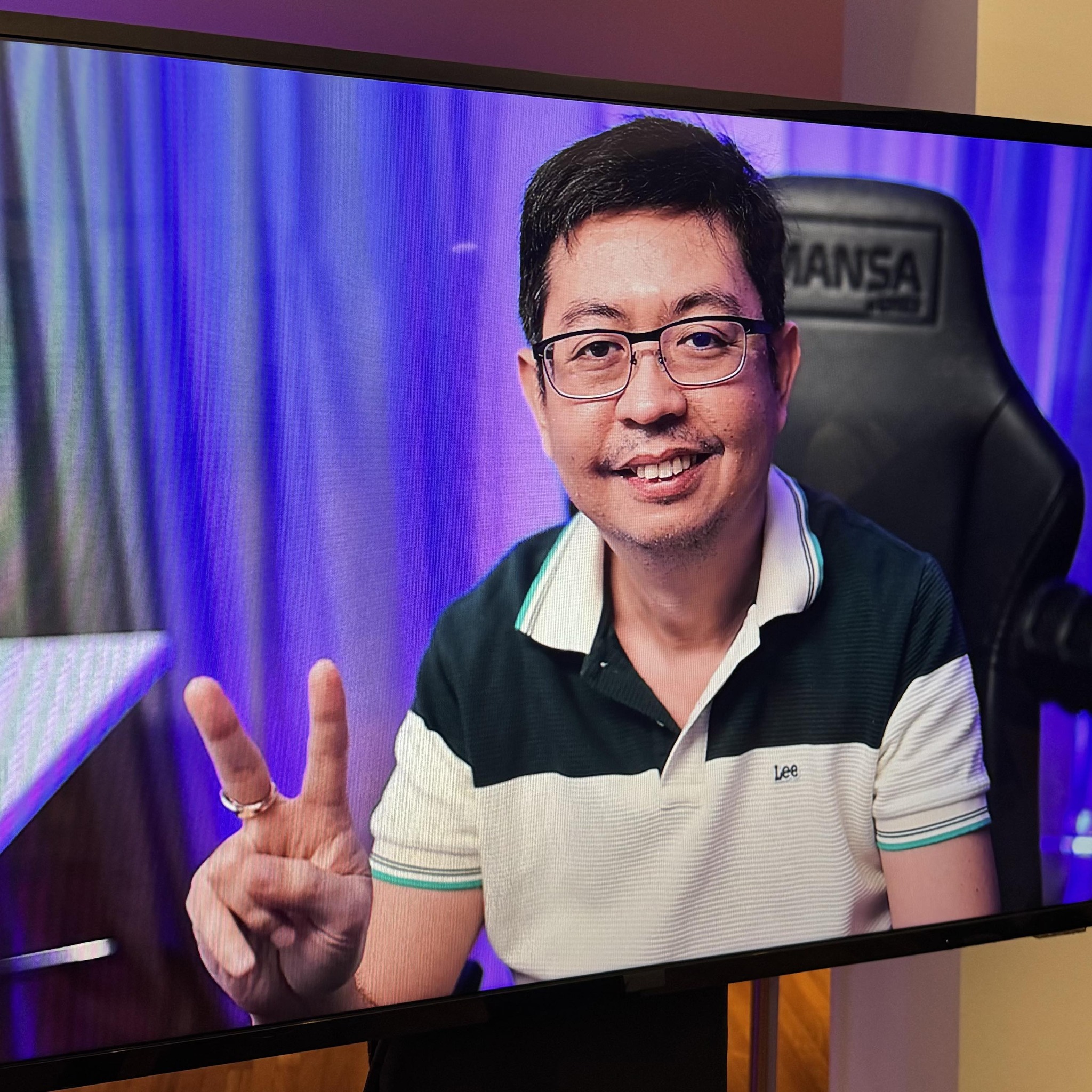
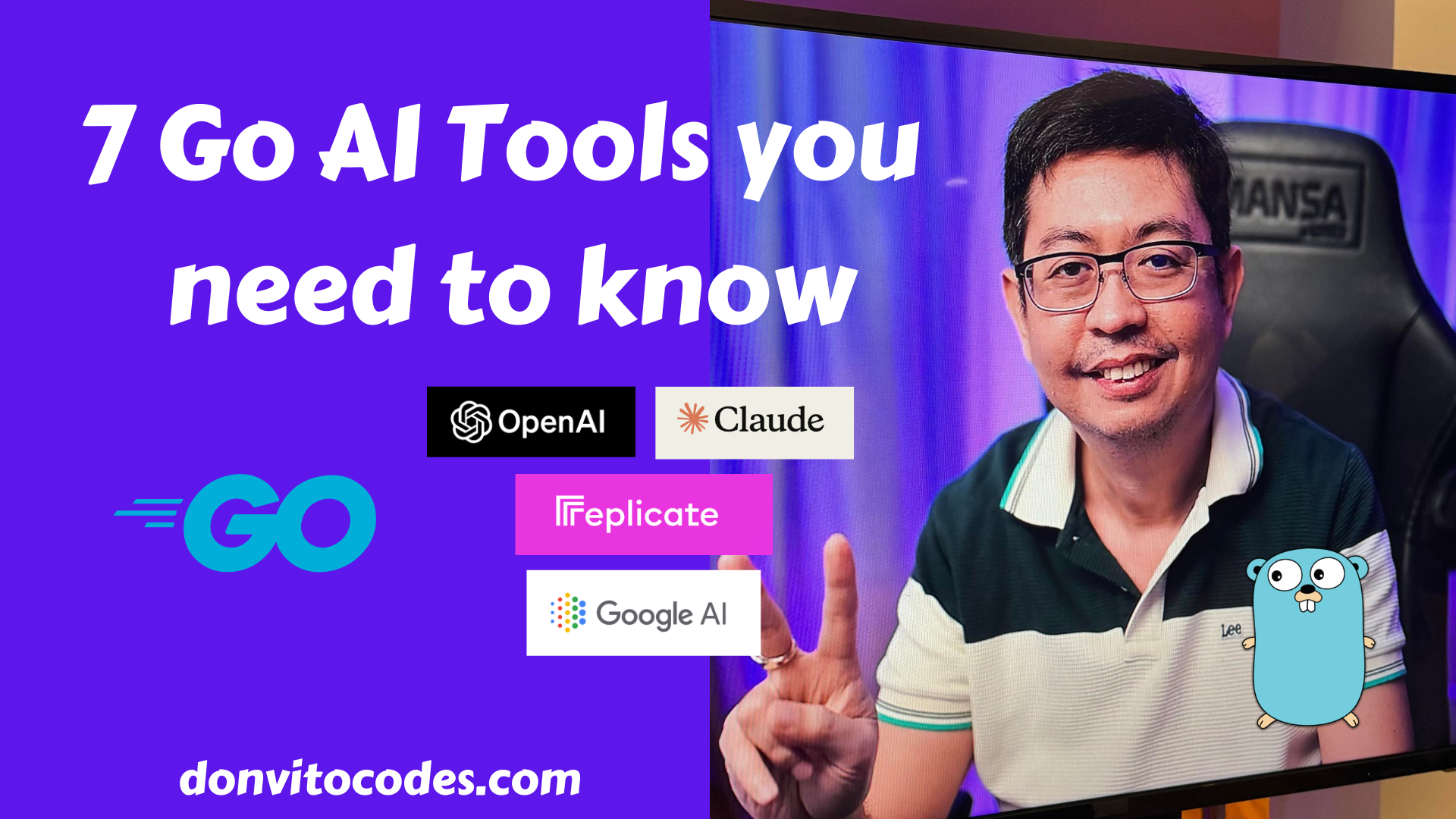
Artificial Intelligence (AI) is rapidly transforming industries by providing intelligent solutions that enhance productivity, efficiency, and innovation. Go developers looking to integrate AI into their applications can find this list useful. In this blog post, we will explore the top 7 tools you need to know to integrate AI with Go.
1. Go OpenAI
Go OpenAI is an unofficial Go client for OpenAI APIs, enabling developers to easily integrate OpenAI's powerful models into their applications. Go OpenAI provides a a great interface to leverage OpenAI's capabilities.
Example usage
package main
import (
"context"
"fmt"
openai "github.com/sashabaranov/go-openai"
)
func main() {
client := openai.NewClient("your token")
resp, err := client.CreateChatCompletion(
context.Background(),
openai.ChatCompletionRequest{
Model: openai.GPT3Dot5Turbo,
Messages: []openai.ChatCompletionMessage{
{
Role: openai.ChatMessageRoleUser,
Content: "Hello!",
},
},
},
)
if err != nil {
fmt.Printf("ChatCompletion error: %v\n", err)
return
}
fmt.Println(resp.Choices[0].Message.Content)
}
2. Go Anthropic
Go Anthropic is an unofficial API wrapper for Anthropic's Claude API, providing a seamless way to integrate Claude's conversational AI capabilities into Go applications. This tool is ideal for developers looking to incorporate advanced conversational agents into their software.
Example Usage (Streaming)
package main
import (
"errors"
"fmt"
"github.com/liushuangls/go-anthropic/v2"
)
func main() {
client := anthropic.NewClient("your anthropic apikey")
resp, err := client.CreateMessagesStream(context.Background(), anthropic.MessagesStreamRequest{
MessagesRequest: anthropic.MessagesRequest{
Model: anthropic.ModelClaudeInstant1Dot2,
Messages: []anthropic.Message{
anthropic.NewUserTextMessage("What is your name?"),
},
MaxTokens: 1000,
},
OnContentBlockDelta: func(data anthropic.MessagesEventContentBlockDeltaData) {
fmt.Printf("Stream Content: %s\n", data.Delta.Text)
},
})
if err != nil {
var e *anthropic.APIError
if errors.As(err, &e) {
fmt.Printf("Messages stream error, type: %s, message: %s", e.Type, e.Message)
} else {
fmt.Printf("Messages stream error: %v\n", err)
}
return
}
fmt.Println(resp.Content[0].Text)
}
3. Go SDK for Google Generative AI
The Go SDK for Google Generative AI allows developers to access Google's cutting-edge generative AI models, such as Gemini, to build innovative AI-powered applications. This SDK is essential for those looking to leverage Google's expertise in AI.
4. LangChain Go
LangChain Go is the Go language implementation of LangChain, a library designed for building language model applications. This tool is perfect for developers looking to integrate advanced language processing capabilities into their Go applications.
Example Usage
package main
import (
"context"
"fmt"
"log"
"github.com/tmc/langchaingo/llms"
"github.com/tmc/langchaingo/llms/openai"
)
func main() {
ctx := context.Background()
llm, err := openai.New()
if err != nil {
log.Fatal(err)
}
prompt := "What would be a good company name for a company that makes colorful socks?"
completion, err := llms.GenerateFromSinglePrompt(ctx, llm, prompt)
if err != nil {
log.Fatal(err)
}
fmt.Println(completion)
}
5. Replicate Go
Replicate Go is a Go client for Replicate, allowing developers to run models from their Go code. This tool simplifies the process of integrating machine learning models into Go applications, providing a straightforward interface to Replicate's API.
Example Usage
ctx := context.TODO()
// You can also provide a token directly with
// `replicate.NewClient(replicate.WithToken("r8_..."))`
r8, err := replicate.NewClient(replicate.WithTokenFromEnv())
if err != nil {
// handle error
}
model := "stability-ai/sdxl"
version := "7762fd07cf82c948538e41f63f77d685e02b063e37e496e96eefd46c929f9bdc"
input := replicate.PredictionInput{
"prompt": "An astronaut riding a rainbow unicorn",
}
webhook := replicate.Webhook{
URL: "https://example.com/webhook",
Events: []replicate.WebhookEventType{"start", "completed"},
}
// Run a model and wait for its output
output, _ := r8.Run(ctx, fmt.Sprintf("%s:%s", model, version), input, &webhook)
6. Go HuggingFace
Go HuggingFace is the Go language client for Hugging Face's Inference API, enabling developers to interact with Hugging Face's extensive model repository. This tool is essential for those looking to utilize state-of-the-art NLP models in their Go applications.
Example Usage
package main
import (
"context"
"fmt"
"log"
"os"
"github.com/hupe1980/go-huggingface"
)
func main() {
ic := huggingface.NewInferenceClient(os.Getenv("HUGGINGFACEHUB_API_TOKEN"))
res, err := ic.ZeroShotClassification(context.Background(), &huggingface.ZeroShotClassificationRequest{
Inputs: []string{"Hi, I recently bought a device from your company but it is not working as advertised and I would like to get reimbursed!"},
Parameters: huggingface.ZeroShotClassificationParameters{
CandidateLabels: []string{"refund", "faq", "legal"},
},
})
if err != nil {
log.Fatal(err)
}
fmt.Println(res[0].Sequence)
fmt.Println("Labels:", res[0].Labels)
fmt.Println("Scores:", res[0].Scores)
}
7. LeonAI Go
LeonAI Go is an unofficial CLI tool for Leonardo AI, providing a command-line interface to interact with Leonardo AI's image and video generation. This tool is perfect for developers who prefer working with CLI tools and want to integrate image AI capabilities into their workflows.
Example Usage
leonai generate --cookie cookie.txt --image car.jpg --output car.mp4 --motion-strength 5
These seven tools are useful when integrating AI with Go, providing developers with powerful capabilities to build intelligent applications. By leveraging these tools, you can enhance your Go projects with state-of-the-art AI features, driving innovation and efficiency. Whether you're building chatbots, content generators, or advanced analytics systems, these tools offer the flexibility and performance you need to succeed in the rapidly evolving field of AI.
I created a Github Repo to collect all Go-based tools and libraries I discover when I work with integrating AI. You can star ⭐ it in Github to get updates.
If you're interested in learning more about integrating Go with Generative AI, follow this blog for more tutorials and insights. This is just the start!
I do live coding in Twitch and Youtube. You can follow me if you'd like to ask me questions when I go live. I also post in LinkedIn, you can connect with me there as well.
Subscribe to my newsletter
Read articles from DonvitoCodes directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
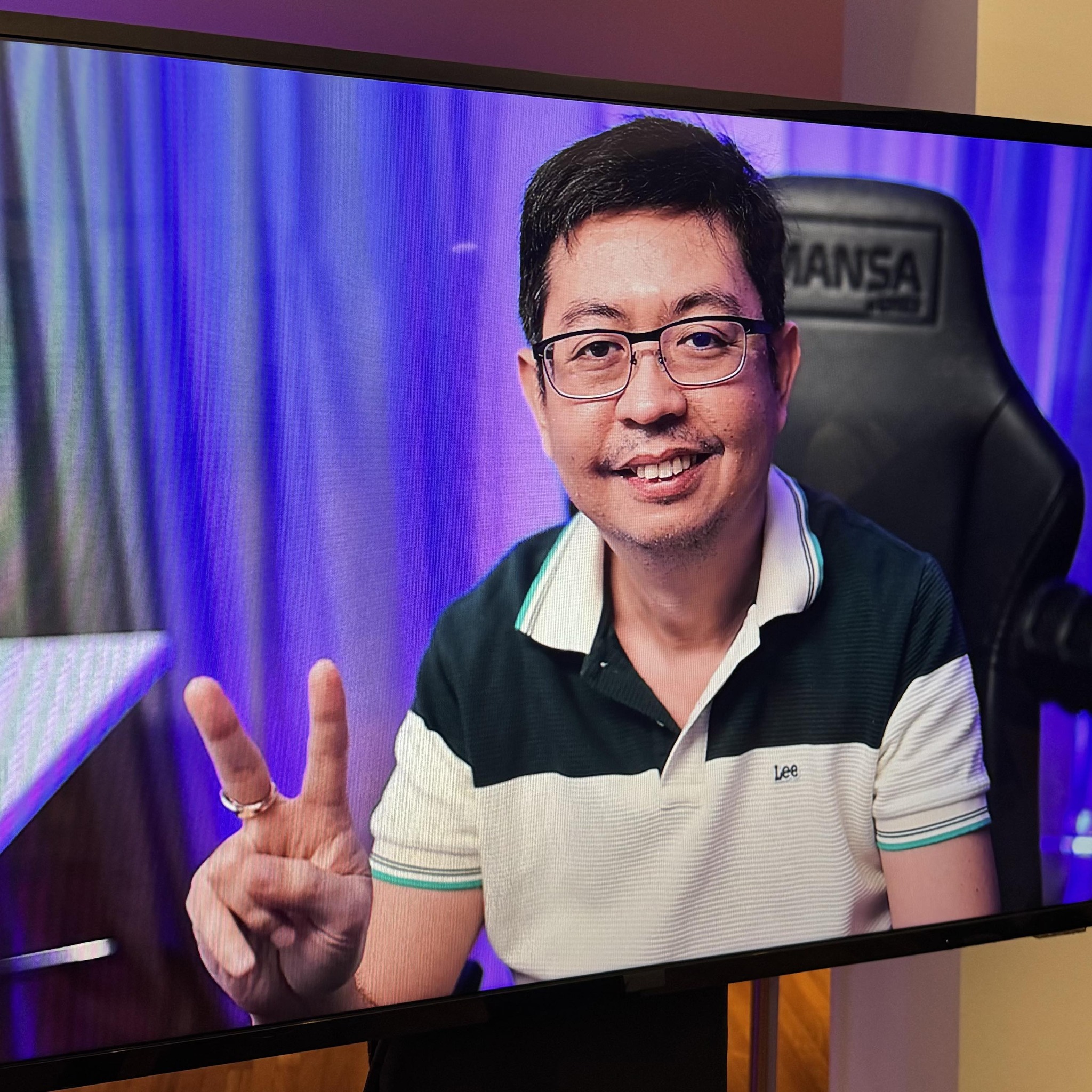
DonvitoCodes
DonvitoCodes
I decided to take a career break and try out building apps using Generative AI. I am also learning NextJS and developing LaunchStack, starter code which I will use for my web projects