Day 3: Exploring Conditional Statements, Logical Operators, and Interactive Programs
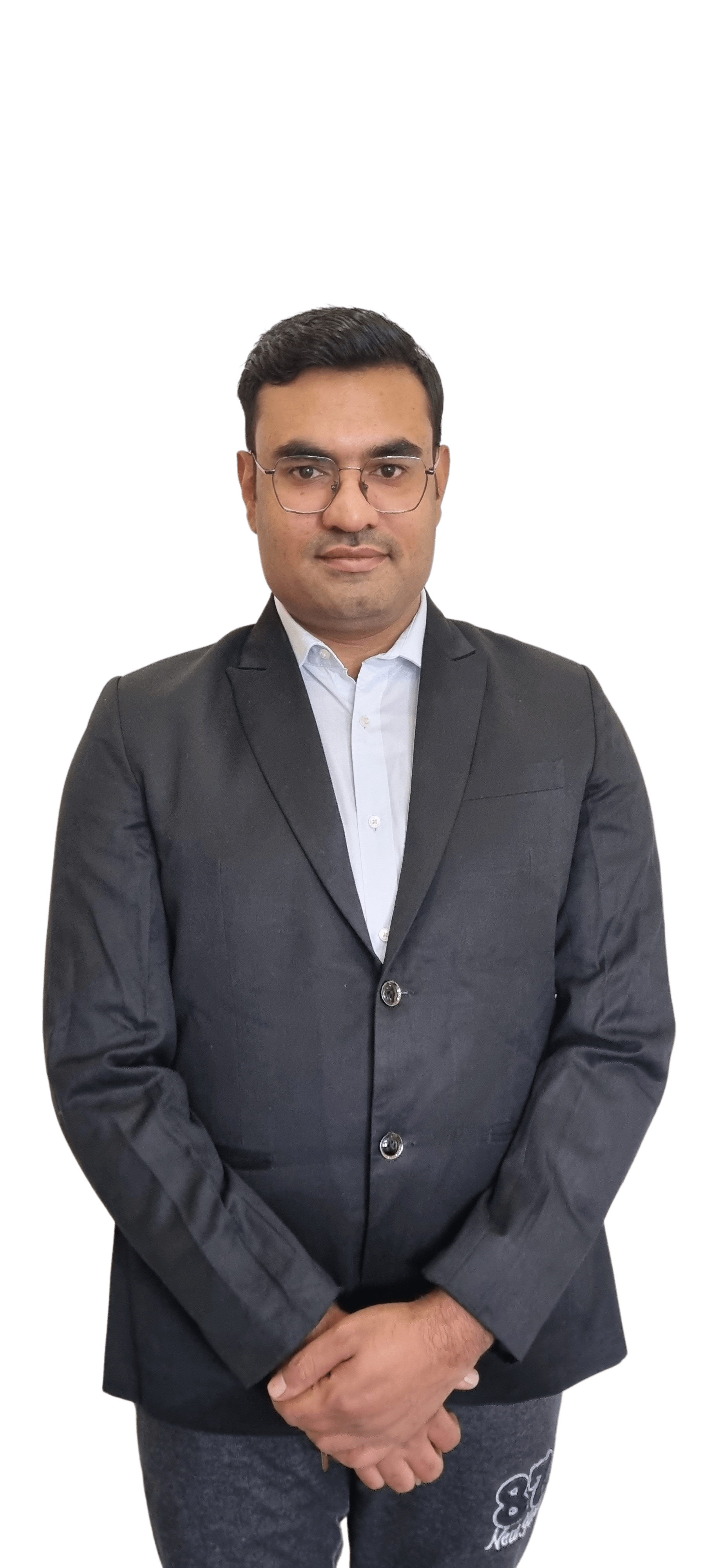
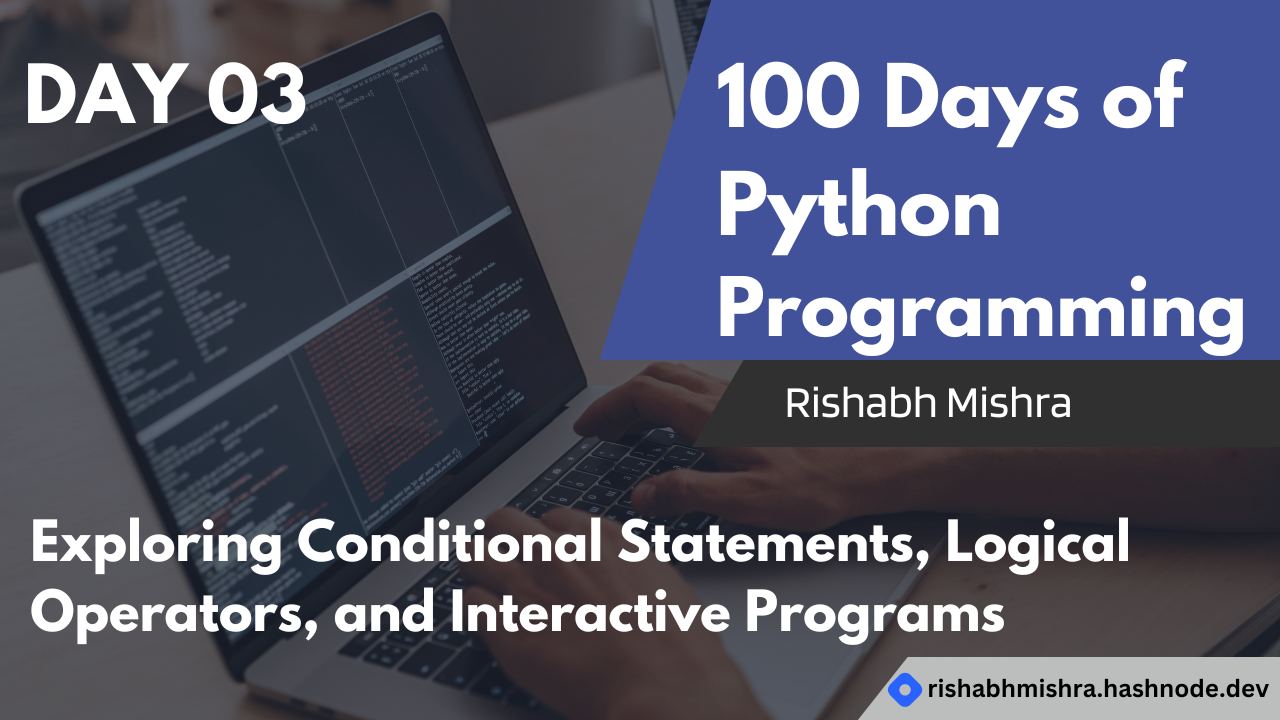
Hello everyone! Today in my journey through Python programming, I delved deeper into conditional statements, logical operators, and created interactive programs that respond dynamically to user inputs. Let’s dive into what I've learned and the exciting programs I built!
Concepts Explored Today:
Conditional Statements (
if
,elif
,else
):- Conditional statements are used to make decisions in programming. They allow the program to execute different blocks of code based on whether a specified condition evaluates to true or false.
num = int(input("Enter a number: "))
if num % 2 == 0:
print(f"The number {num} is even.")
else:
print(f"The number {num} is odd.")
In this example, the program checks if a number is even or odd using the modulus operator (%
). If the remainder when dividing the number by 2 is 0 (num % 2 == 0
), it prints that the number is even; otherwise, it prints that the number is odd.
Logical Operators (
and
,or
,not
):- Logical operators allow combining multiple conditions in conditional statements.
year = int(input("Enter a year: "))
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
print(f"Year {year} is a leap year")
else:
print(f"Year {year} is not a leap year")
Here,
or
is used to check if a year is divisible by 4 and not divisible by 100, or if it is divisible by 400. This determines whether the year is a leap year based on the given rules.Nested Conditionals:
- Nested conditionals involve placing one conditional statement inside another. This allows for more complex decision-making scenarios.
height = int(input("What is your height in cm? "))
if height >= 120:
print("You can ride the rollercoaster!")
age = int(input("What is your age? "))
if age < 12:
print("Child tickets are $5.")
elif age <= 18:
print("Youth tickets are $7.")
else:
print("Adult tickets are $12.")
else:
print("Sorry, you have to grow taller before you can ride.")
This snippet checks if a person is tall enough to ride a rollercoaster based on their height. If they are tall enough (height >= 120
), it further checks their age to determine the ticket price using nested if-else
statements.
Programs Developed:
BMI Calculator:
Weight = int(input("Enter your weight in Kg: ")) Height = float(input("Enter your height in m: ")) BMI = Weight / (Height ** 2) if BMI < 18.5: print(f"Your BMI is {BMI} and You are underweight") elif 18.5 <= BMI < 25: print(f"Your BMI is {BMI} and You have a normal weight") elif 25 <= BMI < 30: print(f"Your BMI is {BMI} and You are slightly overweight") elif 30 <= BMI < 35: print(f"Your BMI is {BMI} and You are obese") elif BMI >= 35: print(f"Your BMI is {BMI} and You are clinically obese")
This program calculates the Body Mass Index (BMI) based on user-provided weight and height, then categorizes the BMI into different health categories using
if-elif-else
statements.Leap Year Checker:
year = int(input("Enter a year: ")) if year % 4 == 0: if year % 100 == 0: if year % 400 == 0: print(f"Year {year} is a leap year.") else: print(f"Year {year} is not a leap year.") else: print(f"Year {year} is a leap year.") else: print(f"Year {year} is not a leap year.")
This program determines whether a given year is a leap year by checking the conditions using nested
if-else
statements.Treasure Island Game:
pythonCopy codeprint("Welcome to Treasure Island.") print("Your mission is to find the treasure.") choice1 = input('You\'re at a crossroad. Where do you want to go? Type "left" or "right"\n').lower() if choice1 == "left": choice2 = input('You\'ve come to a lake. There is an island in the middle of the lake. Type "wait" to wait for a boat. Type "swim" to swim across.\n').lower() if choice2 == "wait": choice3 = input("You arrive at the island unharmed. There is a house with 3 doors. One red, one yellow, and one blue. Which color do you choose?\n").lower() if choice3 == "red": print("It's a room full of fire. Game Over.") elif choice3 == "yellow": print("You found the treasure! You Win!") elif choice3 == "blue": print("You enter a room of beasts. Game Over.") else: print("You chose a door that doesn't exist. Game Over.") else: print("You get attacked by an angry trout. Game Over.") else: print("You fell into a hole. Game Over.")
This interactive game lets users make choices that determine their journey's outcome, demonstrating nested conditional statements for decision-making.
GitHub Repository:
Explore my code examples and follow my Python learning journey on GitHub!
https://github.com/Rishmish94/100daysofpython
Summary:
Today was a productive day learning about conditional statements, logical operators, and creating interactive programs in Python. I explored how to control program flow based on different conditions and built programs like the BMI calculator, leap year checker, rollercoaster ticket price calculator, and a text-based adventure game.
I'm excited to keep improving these skills and using them in more complex projects, so stay tuned for more updates on my Python journey!
Remember, understanding these concepts helps in coding, problem-solving, and creating engaging user experiences, so keep coding and exploring new possibilities!
See you all tomorrow for Day 4!
Subscribe to my newsletter
Read articles from Rishabh Mishra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
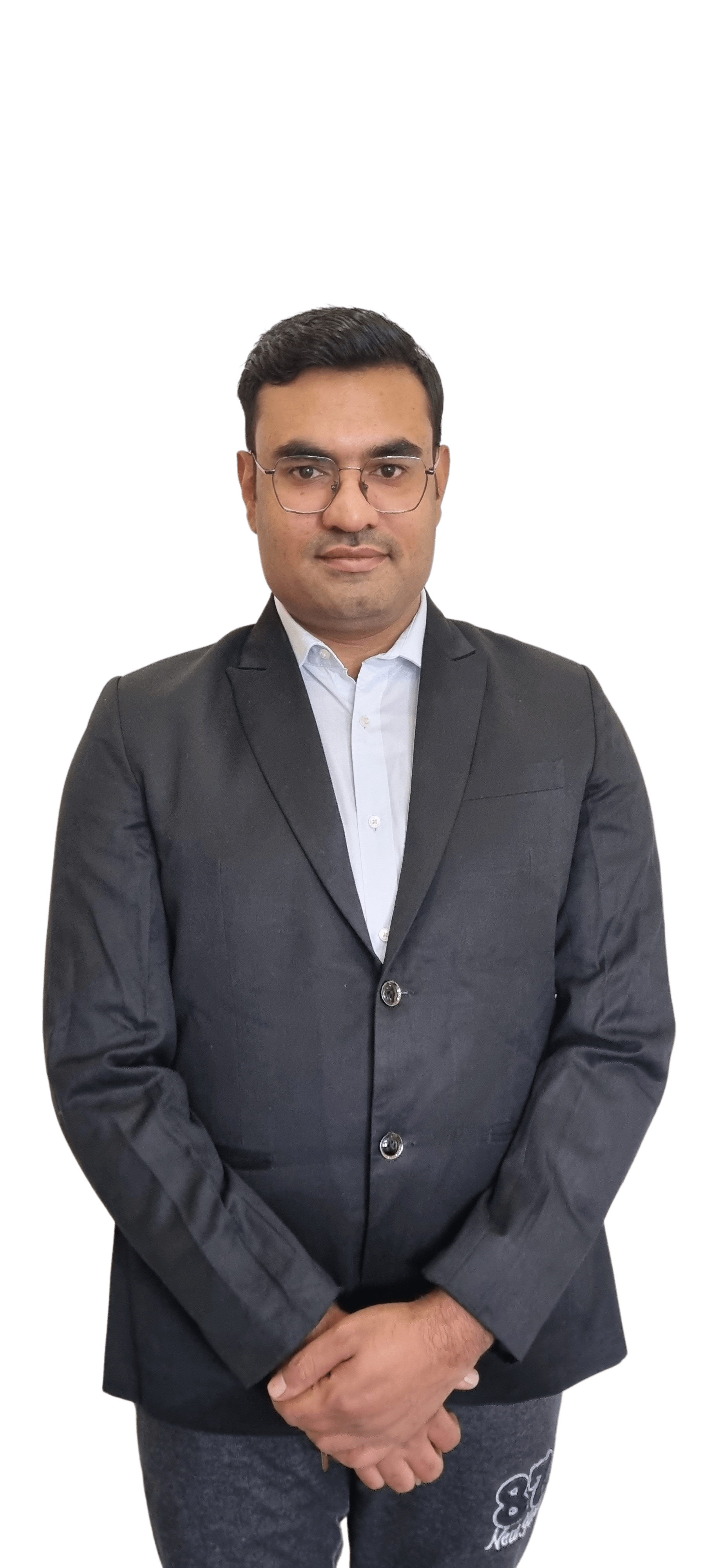
Rishabh Mishra
Rishabh Mishra
I'm Rishabh Mishra, an AWS Cloud and DevOps Engineer with a passion for automation and data analytics. I've honed my skills in AWS services, containerization, CI/CD pipelines, and infrastructure as code. Currently, I'm focused on leveraging my technical expertise to drive innovation and streamline processes. My goal is to share insights, learn from the community, and contribute to impactful projects in the DevOps and cloud domains. Let's connect and collaborate on Hashnode!