Mastering Iterators in Python: A Fun and Easy Guide
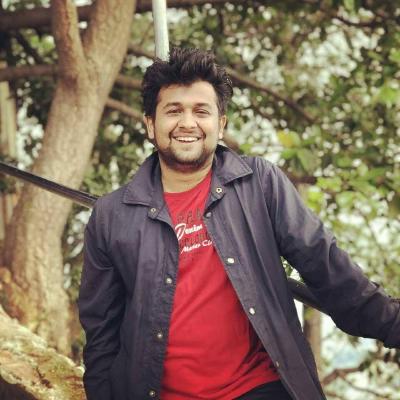

Hey there, Python enthusiasts! Today, we're going to dive deep into the world of iterators in Python. If you've ever felt confused or overwhelmed by iterators, fear not! I'm here to break it down for you in a fun and easy way.
What are Iterators?
In Python, an iterator is an object that allows you to traverse through a sequence of elements. It provides a way to access the elements of a container one by one without needing to know the underlying structure of the container.
Iterators are everywhere in Python. You've probably used them without even realizing it! For example, when you loop through a list using a for
loop, you're actually using an iterator behind the scenes.
How to Create an Iterator
Creating your own iterator in Python is surprisingly simple. All you need to do is define a class that implements the __iter__()
and __next__()
methods.
Let's create a simple iterator that generates the Fibonacci sequence:
class Fibonacci:
def __init__(self):
self.a, self.b = 0, 1
def __iter__(self):
return self
def __next__(self):
result = self.a
self.a, self.b = self.b, self.a + self.b
return result
fib = Fibonacci()
for i in range(10):
print(next(fib))
In this example, we define a class Fibonacci
that implements the __iter__()
and __next__()
methods. The __iter__()
method returns the iterator object itself, and the __next__()
method calculates the next Fibonacci number in the sequence.
Using Iterators with iter()
and next()
In Python, you can also create an iterator from an iterable object using the iter()
function. Once you have an iterator object, you can retrieve the next element using the next()
function.
Let's see an example using the iter()
and next()
functions with a list:
my_list = [1, 2, 3, 4, 5]
my_iter = iter(my_list)
print(next(my_iter)) # Output: 1
print(next(my_iter)) # Output: 2
print(next(my_iter)) # Output: 3
In this example, we create an iterator my_iter
from the list my_list
using the iter()
function. We then use the next()
function to retrieve each element from the iterator.
The iter()
Function and Iterables
In Python, an iterable is an object that can be used with the iter()
function to create an iterator. Common examples of iterables include lists, tuples, dictionaries, and strings.
When you call the iter()
function on an iterable, it returns an iterator object that allows you to traverse through the elements of the iterable.
Let's see an example using the iter()
function with a string:
my_string = "Hello, World!"
my_iter = iter(my_string)
print(next(my_iter)) # Output: 'H'
print(next(my_iter)) # Output: 'e'
print(next(my_iter)) # Output: 'l'
In this example, we create an iterator my_iter
from the string my_string
using the iter()
function. We then use the next()
function to retrieve each character from the iterator.
The StopIteration
Exception
When you reach the end of an iterator and try to retrieve the next element using the next()
function, Python raises a StopIteration
exception. This exception indicates that there are no more elements to retrieve from the iterator.
Let's see an example of catching the StopIteration
exception:
my_list = [1, 2, 3]
my_iter = iter(my_list)
try:
while True:
print(next(my_iter))
except StopIteration:
print("End of iterator reached")
In this example, we create an iterator my_iter
from the list my_list
using the iter()
function. We then use a while
loop to continuously retrieve elements from the iterator until the StopIteration
exception is raised.
Using Iterators in for
Loops
One of the most common ways to use iterators in Python is with for
loops. When you iterate over an iterable object using a for
loop, Python automatically creates an iterator object and retrieves elements from it using the next()
function.
Let's see an example of using an iterator in a for
loop:
my_list = [1, 2, 3, 4, 5]
for num in my_list:
print(num)
In this example, we iterate over the list my_list
using a for
loop. Python creates an iterator object behind the scenes and retrieves each element from it using the next()
function.
Conclusion
Congratulations, you've now mastered iterators in Python! You've learned what iterators are, how to create your own iterators, and how to use iterators with the iter()
and next()
functions.
I hope you found this guide fun and easy to follow. If you have any questions or feedback, feel free to leave a comment below. And don't forget to follow me for more Python tips and tricks!
Happy coding! ๐โจ
Subscribe to my newsletter
Read articles from Pravesh Chapagain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
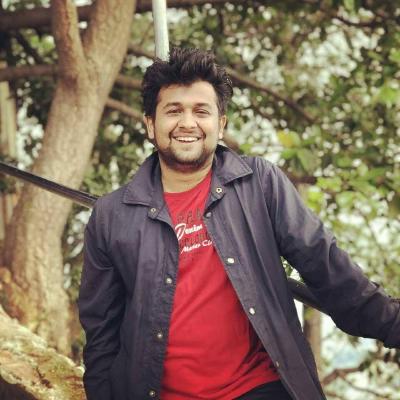