GFG POTD - 21st June 2024 Solution
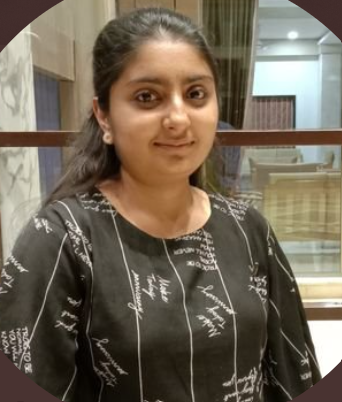
Problem Statement:
You are given a string str containing two fractions a/b and c/d, compare them and return the greater. If they are equal, then return "equal".
Note: The string str contains "a/b, c/d"(fractions are separated by comma(,) & space( )).
Examples:
Input: str = "5/6, 11/45"
Output: 5/6
Explanation: 5/6=0.8333 and 11/45=0.2444, So 5/6 is greater fraction.
Steps to solve problem:
Firstly we know that input is a string containing two fractions separated by comma and space.
So, let's break string from comma to get out two fraction. We will use find() to find out the position of comma and then use substr() to slice string, from position 0 to position of comma(not included) is first fraction and from position of comma+2 (here comma+1 will be include space so we are using comma+2) till last to get second fraction.
size_t commaPos = str.find(','); string first = str.substr(0, commaPos); string second = str.substr(commaPos+2); // str = "5/7, 7/8" // first = "5/7" and second "7/8"
Now fractions are first of all string and in format a/b. So we need to separate out numerator and denominator from string. Again use find('/') to separate numerator and denominator from slash. and then slice out in similar way we done above. Here's code:
size_t firstPos = first.find('/'); int firstNum = stoi(first.substr(0,firstPos)); int firstDenom = stoi(first.substr(firstPos+1)); size_t secondPos = second.find('/'); int secondNum = stoi(second.substr(0,secondPos)); int secondDenom = stoi(second.substr(secondPos+1)); // firstNum = 5 and firstDenom = 7 // secondNum = 7 and secondDenom = 8
Now that we have got numerator and denominator just divide them and compare with each other. If first fraction is bigger then return first else return second and if they are equal then return equal.
float res1, res2; res1 = static_cast<float>(firstNum)/firstDenom; res2 = static_cast<float>(secondNum)/secondDenom; if(res1 == res2){ return "equal"; } else if(res1 > res2){ return first; } else if(res1 < res2){ return second; } else{ return "invalid"; }
Below is the whole code:
class Solution { public: string compareFrac(string str) { size_t commaPos = str.find(','); string first = str.substr(0, commaPos); string second = str.substr(commaPos+2); size_t firstPos = first.find('/'); int firstNum = stoi(first.substr(0,firstPos)); int firstDenom = stoi(first.substr(firstPos+1)); size_t secondPos = second.find('/'); int secondNum = stoi(second.substr(0,secondPos)); int secondDenom = stoi(second.substr(secondPos+1)); float res1, res2; res1 = static_cast<float>(firstNum)/firstDenom; res2 = static_cast<float>(secondNum)/secondDenom; if(res1 == res2){ return "equal"; } else if(res1 > res2){ return first; } else if(res1 < res2){ return second; } else{ return "invalid"; } } };
Thank you ! Keep Learning :)
Subscribe to my newsletter
Read articles from Mahek Gor directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
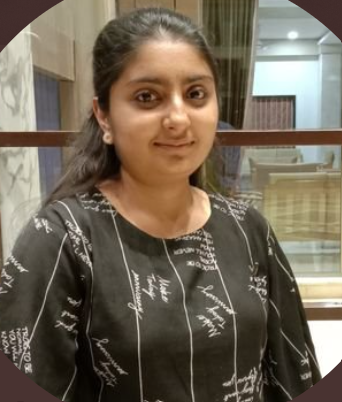