Mastering Java for Interviews: Key Concepts and Questions
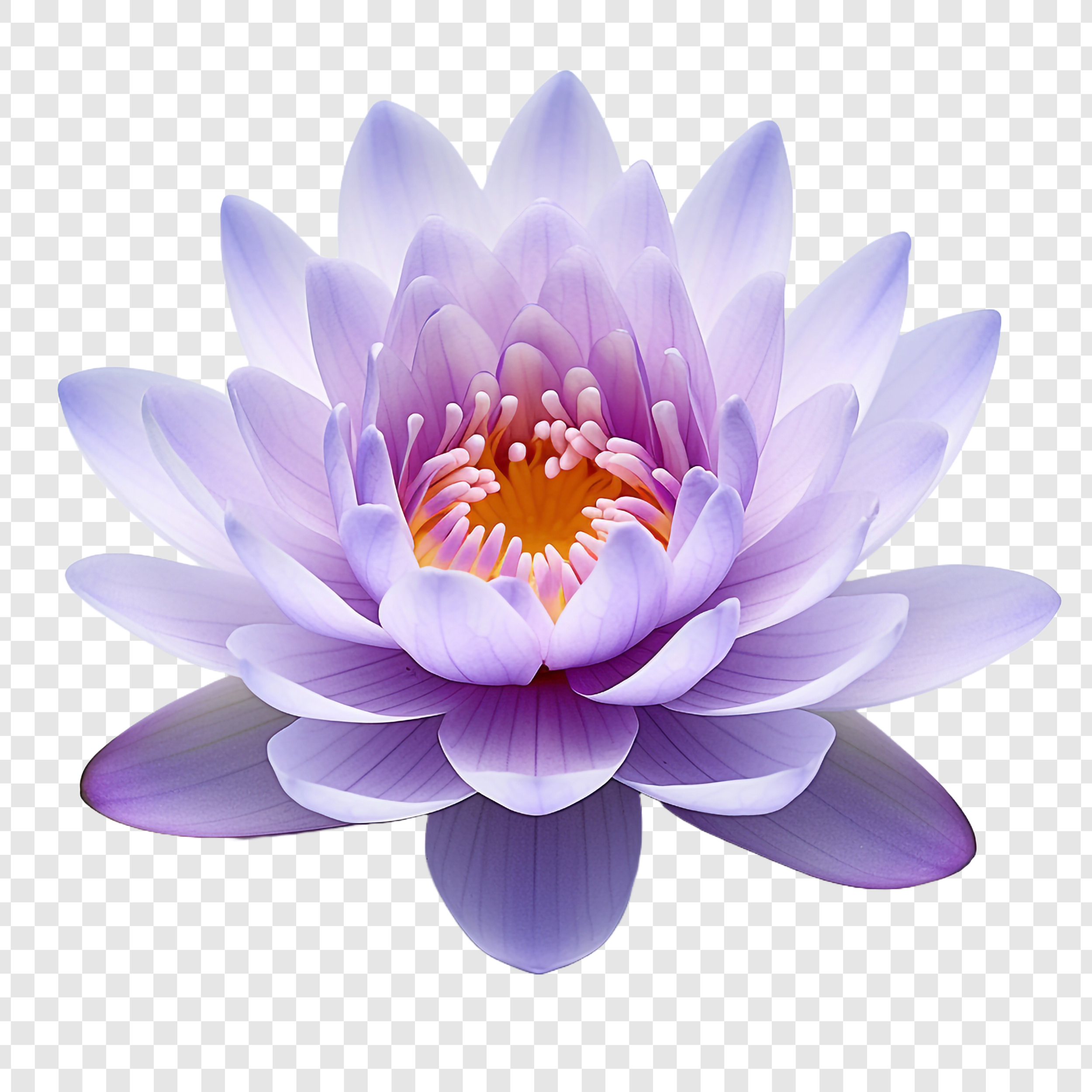
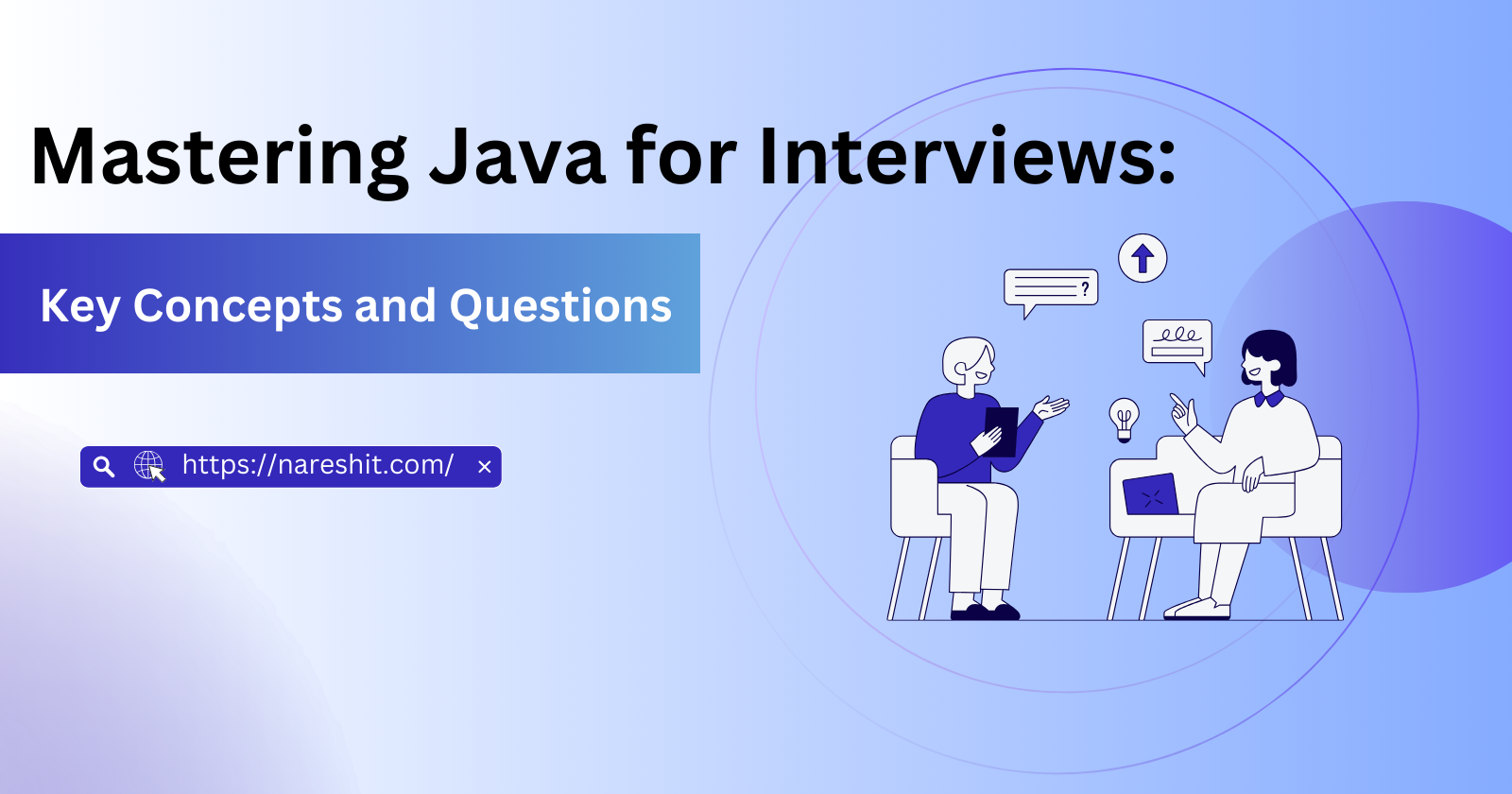
Java remains one of the most widely used programming languages in the industry. Whether you're preparing for a Java developer interview or just brushing up on your skills, it's essential to have a solid understanding of both fundamental and advanced concepts. In this blog, we'll cover key Java topics and provide some common interview questions to help you prepare effectively.
Basic Java Concepts
What is Java?
▪️ Java is a high-level, object-oriented programming language designed to be platform-independent. Its "write once, run anywhere" capability makes it an ideal choice for developing cross-platform applications.Main Features of Java
▪️ Object-Oriented: Java uses an object-oriented programming model that allows for modular and flexible code.
▪️ Platform-Independent: Java code is compiled into bytecode, which can run on any device with a Java Virtual Machine (JVM).
▪️ Secure: Java includes built-in security features, such as the Java security manager, which allows for fine-grained access control.
▪️ Robust: Java's strong memory management, exception handling, and type-checking mechanisms contribute to its robustness. Multithreaded: Java supports multithreading, allowing for concurrent execution of two or more threads for maximum CPU utilization.Object-Oriented Programming (OOP) in Java
▪️ Principles of OOP Encapsulation: Wrapping data (variables) and code (methods) together into a single unit called a class. It restricts direct access to some of an object's components.
▪️ Inheritance: A mechanism where one class inherits the properties and behavior (methods) of another class, promoting code reuse. Polymorphism: Allows objects to be treated as instances of their parent class rather than their actual class. Achieved through method overriding and method overloading.
▪️ Abstraction: Hiding complex implementation details and showing only the necessary features of an object.
Common Interview Questions
- What is the difference between JDK, JRE, and JVM?
▪️ JDK (Java Development Kit): A software development kit used to develop Java applications, includes JRE and development tools.
▪️ JRE (Java Runtime Environment): Provides the libraries, JVM, and other components to run Java applications.
▪️ JVM (Java Virtual Machine): An abstract machine that enables a computer to run Java programs by converting Java bytecode into machine code.
- Explain the concept of 'platform independence' in Java.
▪️ Java code is compiled into bytecode, which can run on any device with a JVM, regardless of the underlying platform, making it platform-independent.
- What is encapsulation in Java?
▪️ Encapsulation is the technique of wrapping data (variables) and code (methods) together as a single unit. It restricts direct access to some of an object's components and can be achieved through access modifiers.
Advanced Java Concepts
- Streams and Lambda Expressions : Java 8 introduced Streams and Lambda expressions, providing a more functional approach to processing collections and handling behaviors.
▪️ Streams: Allow you to process sequences of elements with operations like filter, map, and reduce, enabling efficient, declarative data manipulation.
▪️ Lambda Expressions: Provide a clear and concise way to represent one method interface using an expression, enabling functional programming capabilities.
Common Interview Questions
- What are Java Streams and how do they work?
▪️ Streams in Java provide a functional approach to processing sequences of elements. They support operations like filter, map, and reduce, allowing for efficient, declarative data manipulation.
- Explain the concept of 'lambda expressions' in Java.
▪️ Lambda expressions, introduced in Java 8, provide a clear and concise way to represent one method interface using an expression. They enable functional programming capabilities by allowing you to pass behavior as parameters.
- What are default methods in Java interfaces?
▪️ Default methods are methods defined in interfaces with the default keyword, providing a default implementation. They allow interfaces to evolve without breaking the existing implementation of the classes that implement the interface.
Java Concurrency
Concurrency is a crucial aspect of modern Java applications, enabling them to perform multiple tasks simultaneously.
Common Interview Questions
- What is the difference between synchronized and volatile in Java?
▪️ synchronized: Ensures that only one thread can access a block of code or method at a time. It provides a lock on the object being synchronized.
▪️ volatile: Indicates that a variable's value will be modified by different threads. It ensures visibility of changes to variables across threads but does not provide atomicity.
- What are the main differences between Callable and Runnable?
▪️ Runnable: A functional interface that represents a task to be executed by a thread. It does not return a result or throw a checked exception.
▪️ Callable: A functional interface similar to Runnable but it can return a result and throw a checked exception.
Java Memory Management
Understanding how Java handles memory is crucial for writing efficient and effective code.
Memory Management and Garbage
▪️ Collection Heap and Stack: Java memory is divided into two parts: Heap (for dynamic memory allocation) and Stack (for static memory allocation). Garbage ▪️ Collection: Java automatically manages memory through garbage collection, which reclaims memory used by objects that are no longer reachable.Common Interview Questions
- What is the Java heap space?
▪️ Heap space is the runtime data area from which memory for all class instances and arrays is allocated.
- How does garbage collection work in Java?
▪️Garbage collection in Java automatically deletes objects that are no longer referenced by the application, freeing up memory.
- What is the difference between a stack and a heap?
▪️ Stack: Used for static memory allocation and for storing temporary variables created by functions.
▪️ Heap: Used for dynamic memory allocation, storing objects and arrays created at runtime.
Java Collections Framework
The Java Collections Framework provides a set of interfaces and classes for storing and manipulating groups of data as a single unit.
Collections Framework List:
Ordered collection that allows duplicate elements. Examples include ArrayList, LinkedList.
▪️ Set: Unordered collection that does not allow duplicate elements. Examples include HashSet, TreeSet.
▪️ Map: Collection of key-value pairs. Examples include HashMap, TreeMap.Common Interview Questions
- What is the difference between ArrayList and LinkedList?
▪️ ArrayList: Uses a dynamic array to store elements and provides fast random access. However, inserting and deleting elements is slow due to the need to shift elements.
▪️ LinkedList: Uses a doubly-linked list to store elements. It provides better performance for insertion and deletion operations but slower random access.
- What is a HashMap and how does it work?
▪️ HashMap is a part of the Java Collections Framework that stores data in key-value pairs. It uses a hash table to implement the Map interface, allowing for fast retrieval of elements based on keys.
- What is the difference between HashSet and TreeSet?
▪️ HashSet: Uses a hash table for storage and does not maintain any order of elements.
▪️ TreeSet: Implements the Set interface that uses a tree for storage. It maintains elements in a sorted order.
Java 9 and Beyond
Java has introduced several new features and enhancements in its recent versions, making it more powerful and efficient.
- Module System (Java 9) The module system, introduced in Java 9, provides better modularization and encapsulation of code.
Common Interview Questions
- What is the Java Module System?
▪️ The Java Module System is a new feature in Java 9 that allows developers to modularize their code. It introduces the concept of modules, which are groups of packages.
- How do you define a module in Java?
▪️ A module in Java is defined using the module-info.java file, which specifies the module's name and its dependencies.
- What are the benefits of using the Java Module System?
▪️ Improved encapsulation, better separation of concerns, reduced complexity, and improved application performance.
Java Best Practices
Following best practices ensures that your code is efficient, maintainable, and scalable.
Best Practices
▪️ Use meaningful variable and method names.
▪️ Follow the Single Responsibility Principle (SRP).
▪️ Avoid using magic numbers; use constants instead.
▪️ Prefer composition over inheritance.
▪️ Use streams and lambda expressions for cleaner and more readable code.
Common Interview Questions- What are some Java best practices you follow?
▪️ Writing clean and readable code, using meaningful variable names, following design principles like SOLID, avoiding code duplication, and writing unit tests.
- How do you handle exceptions in Java?
▪️ By using try-catch blocks, throwing exceptions with meaningful messages, and using custom exception classes when necessary.
- What is the importance of immutability in Java?
▪️ Immutability helps to create simple, reliable, and thread-safe code. Immutable objects cannot be changed after creation, reducing the risk of unintended side-effects.
Preparing for Your Java Interview
To excel in your Java interview, focus on:
▪️ Understanding core Java concepts and OOP principles.
▪️ Familiarizing yourself with Java 8 and later features, such as Streams and Lambda expressions.
▪️ Gaining a solid grasp of concurrency and multithreading.
▪️ Practicing coding problems and algorithms in Java.
▪️ Reviewing common Java libraries and frameworks relevant to the job you're applying for.
Conclusion
Java is a powerful and versatile language that remains a staple in the software development industry. By mastering the concepts covered in this blog and practicing common interview questions, you'll be well-prepared to tackle your next Java interview with confidence.
Good luck with your interview preparation, and happy coding!
.
Subscribe to my newsletter
Read articles from Tejaswini Naresh IT directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
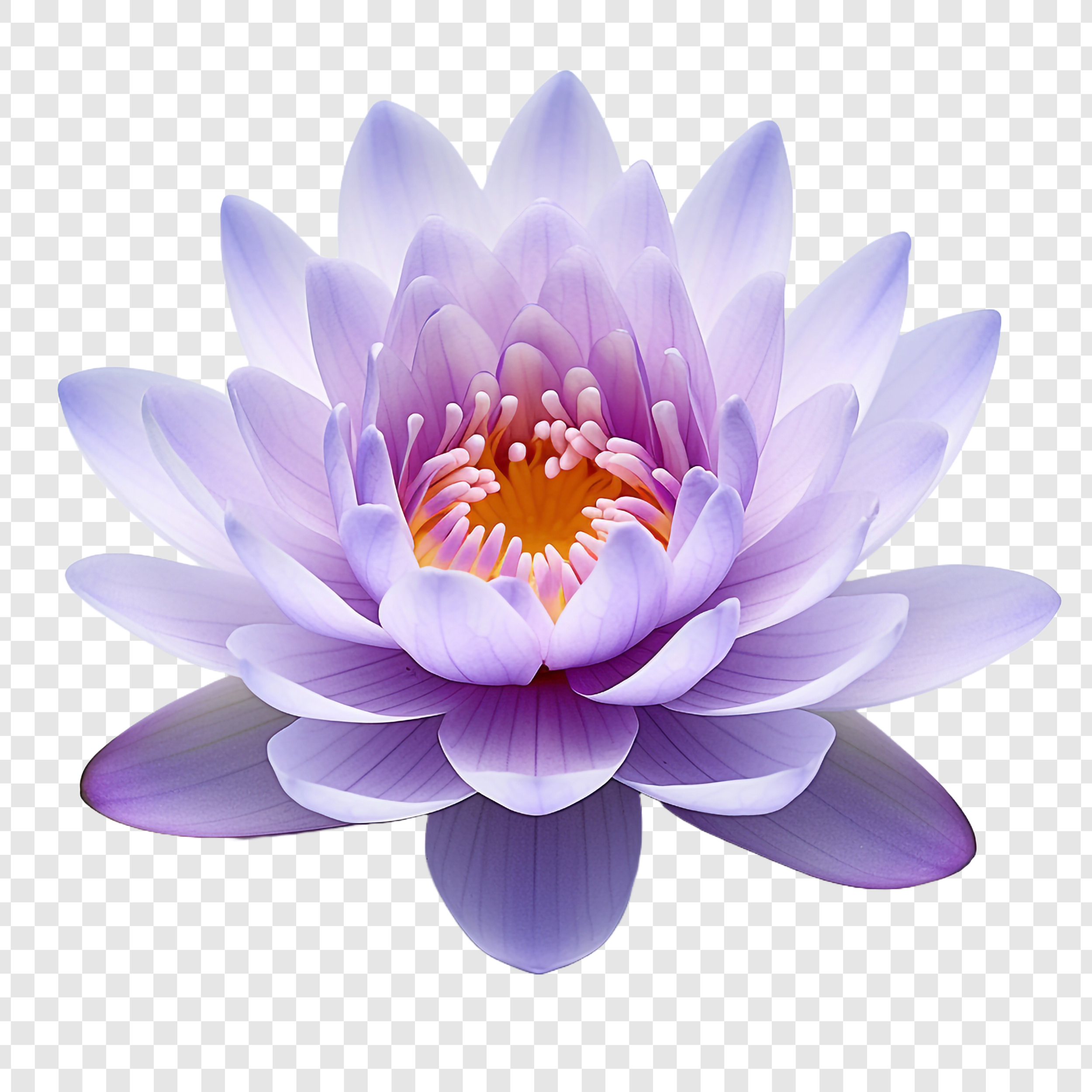
Tejaswini Naresh IT
Tejaswini Naresh IT
I AM A DIGITAL MARKETER