JavaScript Compiler: A Comprehensive Guide to Understanding and Using It

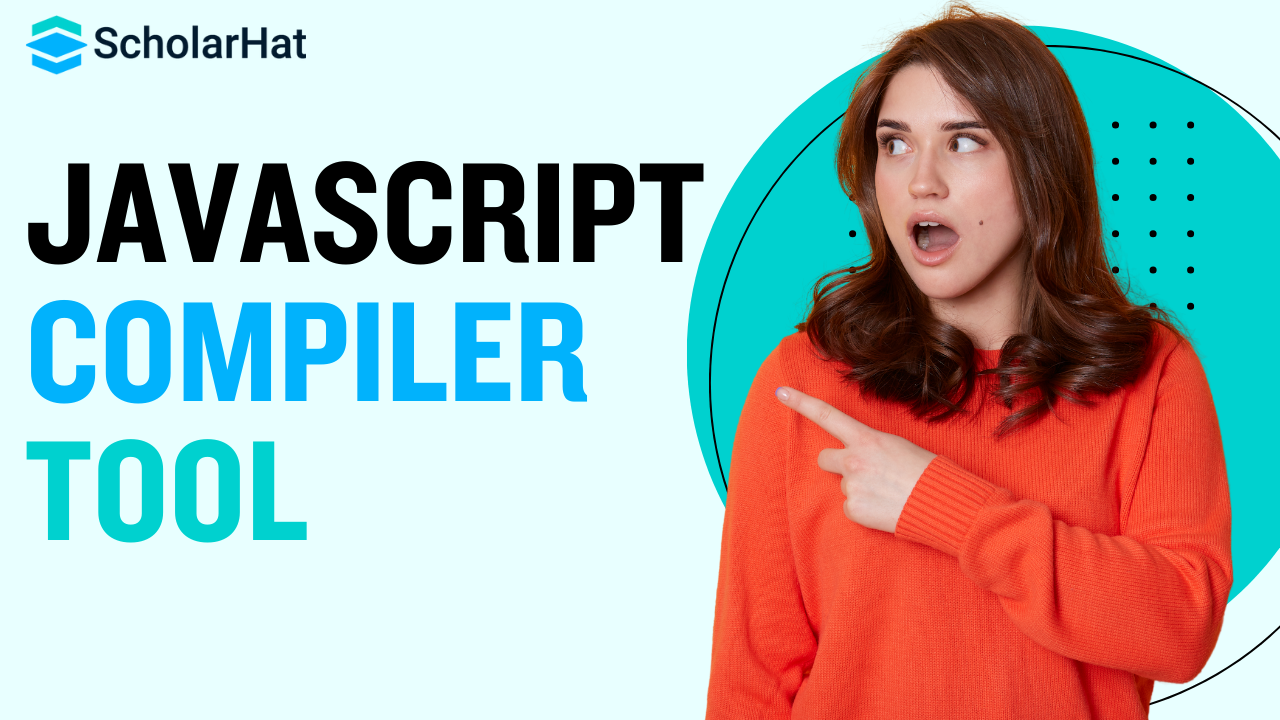
Introduction to JavaScript Compilers
In the ever-evolving world of web development, JavaScript remains a cornerstone language for creating dynamic and interactive websites. As developers strive to optimize their code and enhance performance, tools like JavaScript compilers have become increasingly important. This comprehensive guide will explore what a JavaScript compiler is, how it works, and how you can leverage it to improve your coding workflow.
Before we dive deep into the intricacies of JavaScript compilers, it's worth noting that if you're looking to test your code quickly, you can use a javascript online compiler to experiment with different compiler options. Additionally, for those preparing for technical interviews, brushing up on Javascript interview questions and answers can be incredibly helpful.
What is a JavaScript Compiler?
Understanding the Basics
A JavaScript compiler is a program that transforms JavaScript source code into a more efficient, optimized form. Unlike traditional compiled languages such as C++ or Java, JavaScript is typically considered an interpreted language. However, modern JavaScript engines employ compilation techniques to improve performance.
The Role of Compilation in JavaScript
Compilation in JavaScript serves several purposes:
Code optimization
Error detection
Performance enhancement
Browser compatibility improvement
By using a JavaScript compiler, developers can write more maintainable and efficient code, leading to faster web applications and improved user experiences.
How JavaScript Compilers Work
The Compilation Process
The JavaScript compilation process involves several stages:
Lexical Analysis
Parsing
Abstract Syntax Tree (AST) Generation
Code Generation
Optimization
Each of these stages plays a crucial role in transforming raw JavaScript code into a more efficient form that can be executed by the browser or runtime environment.
Just-In-Time (JIT) Compilation
Modern JavaScript engines often use Just-In-Time (JIT) compilation, a technique that combines the benefits of interpretation and compilation. JIT compilers analyze code as it runs and compile frequently executed parts into machine code for faster execution.
Types of JavaScript Compilers
Popular JavaScript Compilers
There are several JavaScript compilers available, each with its own strengths and use cases:
V8 (used in Chrome and Node.js)
SpiderMonkey (used in Firefox)
JavaScriptCore (used in Safari)
Chakra (used in Microsoft Edge)
These compilers are built into their respective browsers or runtime environments and work behind the scenes to optimize JavaScript execution.
Transpilers: A Special Kind of Compiler
Transpilers, also known as source-to-source compilers, are a unique type of JavaScript compiler. They convert code written in one version of JavaScript (or a JavaScript-like language) into another version or standard JavaScript. Popular transpilers include:
Babel
TypeScript Compiler
CoffeeScript Compiler
Transpilers allow developers to use cutting-edge language features or alternative syntaxes while ensuring compatibility with older browsers or environments.
Benefits of Using a JavaScript Compiler
Enhancing Code Performance
One of the primary advantages of using a JavaScript compiler is improved code performance. Compilers can:
Eliminate dead code
Optimize loops and function calls
Minimize variable lookups
Inline small functions for faster execution
These optimizations can lead to significant speed improvements, especially in complex applications.
Catching Errors Early
JavaScript compilers can detect syntax errors, type mismatches, and other issues before the code is executed. This early error detection helps developers identify and fix problems more quickly, leading to more robust and reliable applications.
How to Use a JavaScript Compiler
Setting Up Your Development Environment
To start using a JavaScript compiler in your projects, you'll need to set up your development environment. Here are the general steps:
Choose a compiler or transpiler based on your project needs
Install Node.js and npm (Node Package Manager) if you haven't already
Install the compiler globally or as a project dependency using npm
Configure the compiler settings in your project
Integrating Compilation into Your Workflow
Once you've set up your compiler, you can integrate it into your development workflow:
Create a configuration file (e.g., .babelrc for Babel)
Set up build scripts in your package.json file
Use task runners like Gulp or Webpack to automate the compilation process
Implement a watch mode for automatic recompilation during development
By integrating compilation into your workflow, you can ensure that your code is always optimized and ready for deployment.
Advanced Compiler Techniques
Optimizing Compiler Performance
As you become more comfortable with JavaScript compilers, you can explore advanced techniques to further optimize your code:
Tree shaking: Eliminating unused code from your final bundle
Code splitting: Breaking your application into smaller chunks for faster loading
Lazy loading: Loading code only when it's needed
Minification: Reducing code size by removing unnecessary characters
These techniques can significantly improve your application's performance and load times.
Debugging Compiled Code
Debugging compiled JavaScript can be challenging, as the compiled code may look different from your original source. To address this:
Use source maps to map compiled code back to the original source
Leverage browser developer tools for step-by-step debugging
Implement logging and error tracking in your compiled code
Mastering these debugging techniques will help you identify and resolve issues more efficiently.
Best Practices for Using JavaScript Compilers
Writing Compiler-Friendly Code
To get the most out of your JavaScript compiler, consider these best practices:
Write modular, reusable code
Avoid global variables and functions
Use const and let instead of var for better scoping
Leverage modern JavaScript features, letting the compiler handle backwards compatibility
Comment your code thoroughly to aid in debugging compiled output
By following these practices, you'll create code that's not only more maintainable but also more efficiently compiled.
Keeping Up with Compiler Updates
JavaScript compilers and the language itself are constantly evolving. To stay current:
Regularly update your compiler and its dependencies
Follow the official documentation and release notes
Participate in community forums and discussions
Experiment with new features in a safe, non-production environment
Staying informed about the latest developments will help you make the most of your JavaScript compiler and keep your skills sharp.
Common Challenges and Solutions
Overcoming Compilation Issues
Even experienced developers can encounter challenges when working with JavaScript compilers. Here are some common issues and their solutions:
Compatibility errors: Use polyfills or adjust your compiler settings
Slow compilation times: Optimize your build process and use incremental compilation
Large bundle sizes: Implement code splitting and tree shaking
Runtime errors in compiled code: Utilize source maps for easier debugging
By anticipating these challenges, you can develop strategies to overcome them efficiently.
Balancing Compilation and Development Speed
Finding the right balance between compilation optimizations and development speed is crucial. Consider:
Using different compilation settings for development and production
Implementing hot module replacement for faster development iterations
Leveraging incremental compilation to reduce build times
Optimizing your project structure for more efficient compilation
Striking this balance will help you maintain productivity while ensuring optimal performance in your final product.
The Future of JavaScript Compilation
Emerging Trends and Technologies
As web development continues to evolve, so do JavaScript compilers. Keep an eye on these emerging trends:
WebAssembly integration for near-native performance
Improved AI-driven code optimization
Enhanced support for modular JavaScript development
Advancements in real-time compilation techniques
Staying informed about these trends will help you prepare for the future of JavaScript development and compilation.
Conclusion
Understanding and effectively using a JavaScript compiler is essential for modern web developers. By leveraging the power of compilation, you can create faster, more efficient, and more maintainable JavaScript applications. Whether you're optimizing code performance, ensuring cross-browser compatibility, or experimenting with cutting-edge language features, a JavaScript compiler is an invaluable tool in your development arsenal.
Remember to regularly practice with a javascript online compiler to hone your skills and experiment with different compilation techniques. Additionally, staying up-to-date with common Javascript interview questions and answers can help you deepen your understanding of the language and its compilation process.
As you continue to explore the world of JavaScript compilation, keep experimenting, learning, and pushing the boundaries of what's possible in web development. With the right tools and knowledge, you'll be well-equipped to create outstanding web applications that perform brilliantly across all platforms and devices.
Subscribe to my newsletter
Read articles from ScholarHat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
